var sushi=["https://i.imgur.com/77xfiZT.png", "https://i.imgur.com/LEndRAV.png", "https://i.imgur.com/d1fWjnJ.png", "https://i.imgur.com/UyTqQin.png"];
let belt;
var sushi1;
var sushi2;
var sushi3;
var sushi4;
//var x = 80;
var speed= 7;
var plate=[];
var inChopstick=false;
var nSushi=200;
var x=0;
var speed=7;
function preload() {
belt = loadImage("https://i.imgur.com/zBwKyLa.png")
sushi1=loadImage(sushi[0]);
sushi2=loadImage(sushi[1]);
sushi3=loadImage(sushi[2]);
sushi4=loadImage(sushi[3]);
}
function setup() {
createCanvas(500, 400);
var n = 0;
for (var i = 0; i < 1000; i++){ //continually creates (1000) plates of sushi
plate[i] = makePlate(n);
n -= 200;
}
frameRate(30);
}
function draw() {
background(0);
image(belt,0,0);
plateShow();
}
function plateGo(){
this.x+=this.dx;
}
function plateShow(){
for(var i = 0; i < plate.length; i++){
plate[i].go();
plate[i].show();
// plate[i].mP();
}
}
function makePlate(px){
var plate={x: px, y:180, w:150, h:100,
dx: 7, go:plateGo, show:drawPlate,
sushi: random([sushi1, sushi2, sushi3, sushi4]),
}
return plate;
}
function drawPlate(){
push();
fill(215);
noStroke();
ellipse(this.x, this.y, this.w, this.h);
stroke(185);
noFill();
ellipse(this.x, this.y, 0.75*this.w, 0.75*this.h);
if (this.sushi==sushi1){
image(sushi1, this.x-50, 0);
}
if (this.sushi==sushi2){
image(sushi2, this.x-50, 0);
}
if (this.sushi==sushi3){
image(sushi3, this.x-50, 0);
}
if (this.sushi==sushi4){
image(sushi4, this.x-50, 0);
}
}
Month: November 2021
Looking Outwards 11: Societal Impacts of Digital Art
This week’s looking out is very interesting. Reading about how NFT have a positive impact onto bridging digital divides was a new way to see them. Originally, I thought NFTs had a rather negative impact: influencing careless spending and money onto temporary “tangible” things. The article I read about speaks about how artists bridge the digital divide and reimagined humanity. New digital artists can solve digital divide: the gap between those who benefit from the digital age and those who do not. These artists would appeal to digital capacity-building:harnessing wonder, digital public goods: creating shareable resources, digital inclusion: using the STEMarts Model, etc. The model highlights creating an equitable and a sustainable digital society is essential to the process of digital cooperation. People are brought together from diverse disciplines and cultural perspectives in order to create alternative futures. In a contemporary world of complexity, most approaches are insufficient while the problem is urgent. The experimentation with technologies could foster literacies and bridge social divides.
Window Gazing – Generative Landscape
var trunks = [];
var hills = [];
var mountains = [];
var noiseParam = 0;
var noiseStep = 0.01;
var clouds = [];
function setup() {
createCanvas(640, 240);
//Trunks
for (var i = 0; i < 10; i++){
var rx = random(width);
trunks[i] = makeTrunk(rx);
}
//Hills
for (var i = 0; i <= width; i ++) {
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height*1.5);
hills.push(value-10);
noiseParam += noiseStep;
}
//Mountains
for (var i = 0; i <=width/5+1; i++) {
var n = noise(noiseParam);
var value = map(n,0,1,height*1/5,height*4/5);
mountains.push(value);
noiseParam += noiseStep;
}
//Clouds
for (var i = 0; i < 10; i ++) {
var cloudX = random(width);
var cloudY = random(height/2, height);
clouds[i] = makeClouds(cloudX, cloudY);
}
frameRate(60);
}
function draw() {
background(191,234,255);
drawMountains();
updateAndDisplayClouds();
removeClouds();
addNewClouds();
drawHills();
updateAndDisplayTrunks();
removeTrunks();
addNewTrunks();
drawWindow();
}
function drawWindow() {
//simple code used to draw window that viewer is looking through
var border = 40
stroke(141,171,223);
noFill();
strokeWeight(border);
rect(border/2,border/2,width-border,height-border);
stroke(220);
strokeWeight(border/2)
rect((border/2)+20,(border/2)+20,(width-border)-40,(height-border)-40,20);
}
//Updating position and displaying clouds
function updateAndDisplayClouds() {
for (var i = 0; i < clouds.length; i ++) {
clouds[i].move();
clouds[i].display();
}
}
//if the clouds reach the end of the screen, remove and replace in new array
function removeClouds() {
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep;
}
//based on set probability, add new cloud into array
function addNewClouds() {
var newCloudLikelihood = 0.07;
if (random(0,1) < newCloudLikelihood) {
var newcloudX = 640;
var newcloudY = random(200);
clouds.push(makeClouds(newcloudX, newcloudY));
}
}
function makeClouds(CLOUDX, CLOUDY) {
var cld = {x: CLOUDX,
y: CLOUDY,
speed: -5,
move: cloudMove,
display: cloudDisplay}
return cld;
}
//moving clouds
function cloudMove() {
this.x += this.speed;
}
//drawing of cloud
function cloudDisplay() {
fill(255); // cream color
noStroke();
ellipse(this.x, this.y, 20, 30);
ellipse(this.x, this.y, 20, 30);
ellipse(this.x, this.y, 30, 10);
ellipse(this.x, this.y, 10, 20);
ellipse(this.x, this.y, 40, 20);
}
//hill drawing using Begin and End Shape
function drawHills() {
noStroke();
fill("green");
beginShape();
vertex(0, height);
for (i = 0; i <= width/5 + 1; i += 1) {
vertex(i*5, hills[i]);
vertex((i+1)*5, hills[i+1]);
}
vertex(width, height);
endShape();
hills.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
hills.push(value);
noiseParam += noiseStep;
}
//Mountains is variantion of hill code
function drawMountains() {
noStroke();
fill(161,178,158);
beginShape();
vertex(0, height);
for (i = 0; i <= width/5 + 1; i += 1) {
vertex(i*5, mountains[i]);
vertex((i+1)*5, mountains[i+1]);
}
vertex(width, height);
endShape();
mountains.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
mountains.push(value);
noiseParam += noiseStep;
}
//trunks in front of window being moved and displayed here
function updateAndDisplayTrunks(){
for (var i = 0; i < trunks.length; i++){
trunks[i].move();
trunks[i].display();
}
}
//if trunk leaves screen, remove and replace with another
function removeTrunks(){
var trunksToKeep = [];
for (var i = 0; i < trunks.length; i++){
if (trunks[i].x + trunks[i].breadth > 0) {
trunksToKeep.push(trunks[i]);
}
}
trunks = trunksToKeep;
}
//small probability of adding new trunk
function addNewTrunks() {
var probability = 0.08;
if (random(0,1) < probability) {
trunks.push(makeTrunk(width));
}
}
// moving trunk along window as if "passing"
function TrunkMove() {
this.x += this.speed;
}
// drawing of trunks
function TrunkDisplay() {
noStroke();
var tHeight = 0
fill(102,60,11);
push();
translate(this.x, height);
rect(0, 0, this.breadth, -height);
stroke(200);
pop();
}
function makeTrunk(birthLocationX) {
var trnk = {x: birthLocationX,
breadth: 40,
speed: -7,
move: TrunkMove,
display: TrunkDisplay}
return trnk;
}
This is my project on Generative landscapes. Using what I learned from class, I created an artistic interpretation of what it feels like to look out of the window of a moving vehicle.
Project Landscape
//Yanina Shavialenka
//Section B
/*
The following project was written with an intention to represent world
peace, love, diversity, and care for the planet. I used world map to
represent the world, people in different national outfits to represent
people from around the world, love symbold to represent love, rainbow
peace sign to represent peace in the world and inclusion, recycling
symbol to represent the fact that we need to recycle and save our planet.
*/
//Initial arrays for images and objects
var people = [];
var world = [];
var symbol = [];
var loadPeople = [];
var loadSymbol = [];
var worldmap;
//Value of change of movement
var dx1 = 1.5;
//Preloads images
function preload() {
var file = [];
file[0] = "https://i.imgur.com/PAWmbXw.png";
file[1] = "https://i.imgur.com/t0fgBq2.png";
file[2] = "https://i.imgur.com/SkGPugf.png";
file[3] = "https://i.imgur.com/dbMZm7H.png";
file[4] = "https://i.imgur.com/L1ODD20.png";
file[5] = "https://i.imgur.com/b5hpqxP.png";
for(var i = 0 ; i < file.length; i++) {
people[i] = loadImage(file[i]);
}
var file1 = [];
file1[0] = "https://i.imgur.com/IRxGbDw.png";
file1[1] = "https://i.imgur.com/Xkq7Q0x.png";
file1[2] = "https://i.imgur.com/w9crIqx.png";
for(var i = 0 ; i < file1.length; i++) {
symbol[i] = loadImage(file1[i]);
}
worldmap = loadImage("https://i.imgur.com/LhjqpdM.png");
world.push(makeBackground(0,0,dx1));
}
function setup() {
createCanvas(480, 480);
}
//Object functions that create object
//Below function has an object for background
function makeBackground(cx,cy,cdx) {
var c = { x: cx, y: cy, dx: cdx,
stepFunction: stepBackground,
drawFunction: drawBackground
}
return c;
}
//Below function has an object for characters
function makeCharacter(cx, cdx, num) {
var r = random(320);
var c = { x: cx, y: r, dx: cdx,
imageNum: num,
stepFun: stepCharacter,
drawFun: drawCharacter
}
return c;
}
//Below function has an object for symbols
function makeSymbol(cx, cdx, num) {
var r = random(320);
var c = { x: cx, y: r, dx: cdx,
imageNum: num,
step: stepSymbol,
draw: drawSymbol
}
return c;
}
//Object functions that move objects
function stepBackground() {
this.x -= this.dx;
}
function stepCharacter() {
this.x -= this.dx;
}
function stepSymbol() {
this.x -= this.dx;
}
//Object functions that draw objects
function drawBackground() {
image(worldmap,this.x,this.y);
}
function drawCharacter() {
image(people[this.imageNum],this.x,this.y);
}
function drawSymbol() {
image(symbol[this.imageNum],this.x,this.y);
}
function draw() {
background(220);
//random values
var num = random(people.length - 2);
num = int(num);
var num1 = random(symbol.length);
num1 = int(num1);
var peopleCount = random(100,200);
peopleCount = int(peopleCount);
var symbolCount = random(300);
symbolCount = int(symbolCount);
//moves and draws map
for(var i = 0 ; i < world.length ; i++) {
world[i].stepFunction();
world[i].drawFunction();
}
//when the latest world x postion is 0, a new map is drawn at x coordinate of the world map width
if(world[world.length-1].x <= 0) {
world.push(makeBackground(802,0,dx1));
}
//new position for people and symbols
if(frameCount % peopleCount == 0) {
loadPeople.push(makeCharacter(480,dx1,num));
}
if(frameCount % symbolCount == 0) {
loadSymbol.push(makeSymbol(480,dx1,num1));
}
//moves and draws people and symbols
for(var i = 0 ; i < loadPeople.length ; i++) {
loadPeople[i].stepFun();
loadPeople[i].drawFun();
}
for(var i = 0 ; i < loadSymbol.length ; i++){
loadSymbol[i].step();
loadSymbol[i].draw();
}
}
For this week’s project, I decided to portray the idea of utopia where all people around the world live in peace, love, and inclusion. I used the background of a world map to represent the world; people in different national outfits to represent the people around the world; love symbol to represent love; rainbow peace sign to represent peace and inclusion; recycling symbol to represent the idea that we need to recycle to save the earth and have that ideal utopia.
In my project, I encountered a problem with a background since I used an image instead of drawing it out myself: when the image would end, it would not reappear so I came with an idea to use a double image “glued” together which eventually did the trick. Overall I really enjoyed this assignment and found it really fun to work on!
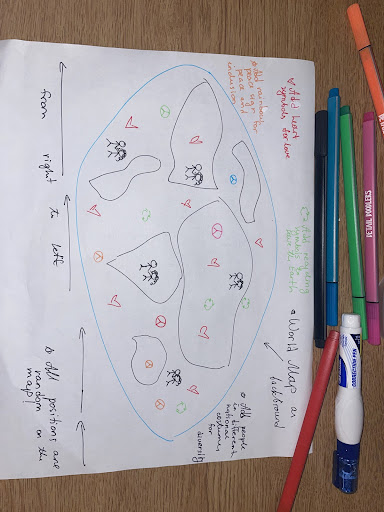
Project 11: Generative Landscape
//Julianna Bolivar
//jbolivar@andrew.cmu.edu
//Section D
//Generative Landscape
var hills = []; //hills array
var trees = [];
var treesShowing = [];
var clouds = [];
var cloudsShowing = [];
var counter = 0;
var cloudCounter = 0;
var noiseParam = 0;
var noiseStep = 0.002;
function setup() {
createCanvas(480, 300);
//trees
for (var i = 0; i < 20;i++){
var trs = random(width);
trees[i] = makeTrees(trs);
}
//hills
for (var i=0; i<width/2+1; i++){
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
noiseParam += noiseStep;
hills.push(value);
}
for (var i = 0; i < 5; i ++) {
var cloudX = random(width);
var cloudY = random(0, 40);
cloudsShowing[i] = makeClouds(cloudX, cloudY);
}
frameRate(20);
}
function draw() {
background(165,182,182);
noStroke();
//sun
fill(204,102,0);
circle(40,50,30);
//hills fill shape
beginShape();
fill(56,87,33);
vertex(0, height);
for (var i=0; i<width/2; i+=1){
vertex(i*5, hills[i]);
}
vertex(width, height);
endShape();
hills.shift();
hills.push(map(noise(noiseParam), 0, 1, 0, height));
noiseParam += noiseStep; //move sideways
updateAndDrawClouds();
removeCloudsOffScreen();
addNewClouds();
updateandDrawTrees();
removeTreesOffScreen();
addNewTrees();
}
function updateandDrawTrees(){
for(var i=0; i < treesShowing.length; i++){
treesShowing[i].move();
treesShowing[i].draw();
}
}
function removeTreesOffScreen(){
var treesToKeep = [];
for (var i = 0; i < treesShowing.length; i++){
if (treesShowing[i].x+20 > 0) {
treesToKeep.push(treesShowing[i]);
}
}
treesShowing = treesToKeep; // remember showing trees
}
function addNewTrees(){
counter+=1;
if (counter % 25 == 0){
treesShowing.push(makeTrees(width, random(150,300)));
}
}
function makeTrees(tx, ty){
var trees = {x:tx, y:ty,
width:random(10, 20),
height:random(50, 90),
r:random(75,200), g:random(75,100), b: 0,
speed: -1.0,
move: treesMove,
draw: drawTrees }
return trees;
}
//draw trees
function drawTrees(){
fill(160,82,45);
rect(this.x, this.y, this.width, this.height);
fill(this.r, this.g, this.b);
circle(this.x-5, this.y-10, 50);
circle(this.x+20, this.y-10, 50);
circle(this.x+5, this.y-20, 50);
}
function treesMove(){
this.x += this.speed;
}
function updateAndDrawClouds(){
for (var i = 0; i < cloudsShowing.length; i++) {
cloudsShowing[i].move();
cloudsShowing[i].draw();
}
}
function removeCloudsOffScreen(){
var cloudsToKeep = [];
for (var i = 0; i < cloudsShowing.length; i++){
if (cloudsShowing[i].cloudX + 10 > 0) {
cloudsToKeep.push(cloudsShowing[i]);
}
}
cloudsShowing = cloudsToKeep;
}
function addNewClouds(){
cloudCounter+=1;
if (cloudCounter % 100 == 0){
cloudsShowing.push(makeClouds(width, random(0,60)));
}
}
function makeClouds(cx, cy){
var c = {cloudX: cx,
cloudY: cy,
cloudSpeed: -1,
move: cloudMove,
draw: cloudDraw}
return c;
}
function cloudMove(){
this.cloudX += this.cloudSpeed;
}
function cloudDraw(){
fill(165);
noStroke();
ellipse(this.cloudX, this.cloudY - 5, 60, 50);
ellipse(this.cloudX - 20, this.cloudY + 10, 60, 50);
ellipse(this.cloudX + 15, this.cloudY - 5, 70, 50);
ellipse(this.cloudX + 5, this.cloudY + 20, 60, 50);
ellipse(this.cloudX + 30, this.cloudY + 10, 80, 50);
}
My landscape is inspired by the autumn. The view from the design studio is very scenic. I think I am most proud of this deliverable. I’ve struggled to make beautiful projects, but I’m pretty satisfied with this one.
LO: Social Impacts of Digital Art
Clearview AI is an app currently being used by hundreds of law enforcement agencies in the US, including the FBI. It can compare a photo of someone’s face to a database of more than 3 billion pictures that Clearview has access to from Facebook, Venmo, YouTube, and other sites. This database includes the FBI’s own database, which contains passport and driver’s license photos. It totals more than 640 million images of US citizens. Clearview AI then returns with matches, along with links to the sites where the database photos were from; it can easily return a name, and possibly a home address as well. What if the general public had access to this app? Police officers and Clearview investors think it’s a possibility for the future. Hopefully this never happens. Personally, I’d never feel safe again. And even in the hands of law enforcement, this technology is controversial. Privacy advocates warn that the app could still return false matches to the police and result in the incarceration of innocent people, and mass implementation and abuse of this technology could be a slippery slope to a surveillance state.
LO-11
I chose to take a look at the article “Art Project Shows Racial Biases in Artificial Intelligence System” written by Meilan Solly. The article talks about an artificial intelligence classification tool called ImageNet created by artist Trevor Paglen and A.I. researcher Kate Crawford. However, the artificial intelligence may be racist. While the program identified white individuals largely in terms of occupation or other functional descriptors, it often classified those with darker skin solely by race. ImageNet is an object lesson, if you will, in what happens when people are categorized like objects. A man who uploaded multiple snapshots of himself in varying attire and settings was consistently labeled “black.” The tool will remain accessible as a physical art installation at Milan’s Fondazione Prada Osservatorio through February 2020.
project-11
this project was pretty interesting. there are birds, clouds, and a landscape going across the screen.
var cloud = []
var bird = []
function setup() {
createCanvas(480, 240)
frameRate(10)
for (i = 0; i < 30; i++) {
birdX = random(width)
birdY = random(height)
bird[i] = makeBird(birdX, birdY)
}
for (i = 0; i < 15; i++) {
cloudX = random(width)
cloudY = random(height/1.5)
cloud[i] = makeCloud(cloudX, cloudY)
}
}
function draw() {
background(140, 200, 255)
strokeWeight(2)
landscape()
showCloud()
showBird()
}
function landscape() {
stroke(86, 125, 70)
beginShape()
for (var i = 0; i < width; i++) {
var x = (i * .01) + (millis() * .0004)
var s = map(noise(x), 0, 1, 150, 200)
line(i, s, i, height)
}
endShape()
}
function makeBird(birdX, birdY) {
var bird = {
fx: birdX,
fy: birdY,
birdspeed: random(-3, -8),
birdmove: moveBird,
birdcolor: color(random(100, 200), random(100, 200), random(100, 200)),
birddraw: drawBird
}
return bird
}
function moveBird() {
this.fx += this.birdspeed
if (this.fx <= -10) {
this.fx += width
}
}
function drawBird() {
stroke(0)
fill(this.birdcolor);
ellipse(this.fx, this.fy, 10, 10)
line(this.fx - 5, this.fy, this.fx - 10, this.fy - 2)
line(this.fx + 5, this.fy, this.fx + 10, this.fy - 2)
}
function showBird() {
for (i = 0; i < bird.length; i++) {
bird[i].birdmove()
bird[i].birddraw()
}
}
function makeCloud(cloudX, cloudY) {
var cloud = {
fx: cloudX,
fy: cloudY,
cloudspeed: random(-1, -2),
cloudmove: moveCloud,
clouddraw: drawCloud
}
return cloud
}
function moveCloud() {
this.fx += this.cloudspeed
if (this.fx <= -10) {
this.fx += width
}
}
function drawCloud() {
noStroke()
fill(255);
ellipse(this.fx, this.fy, 10, 10)
ellipse(this.fx-4, this.fy-1, 10, 10)
}
function showCloud() {
for (i = 0; i < cloud.length; i++) {
cloud[i].cloudmove()
cloud[i].clouddraw()
}
}
Project: Generative Landscape
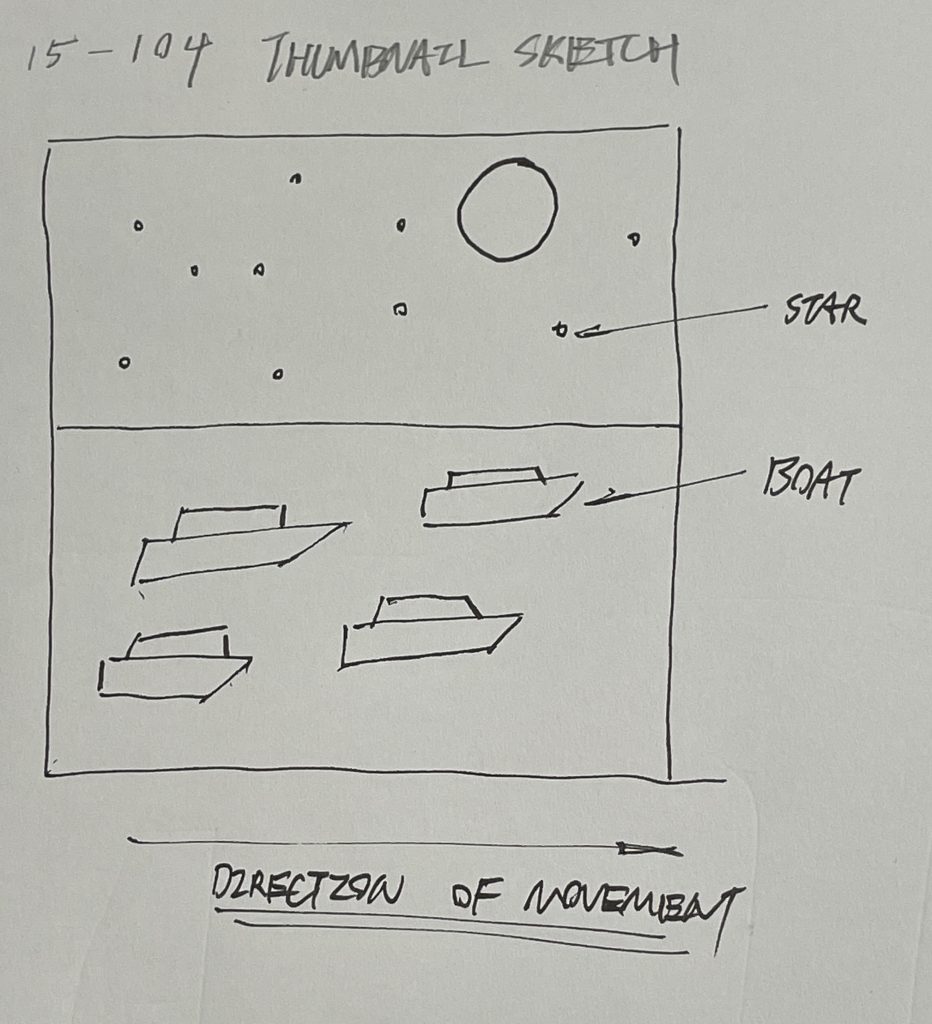
//lucacao
//sectionD
//Project11
var boat = [];//array to store boat number
var speed = 1;
function setup() {
createCanvas(480, 480);
noStroke();
frameRate(5);
for (var i = 0; i < 10;i++){
bx = random(width);//boat x position
}
}
function draw() {
background(220);
sea();
sky();
moon();
for(var i = 0; i < 5; i++){
drawBoat();
boat++;
print(boat);
}
}
function sea() {
fill(0, 64, 108);
rect(0,240,480,240);
}
function sky(){
fill(0, 37, 62);
rect(0,0,480,240);
for (var i = 0; i < 100;i++){
var starx = random(0,480);
var stary = random(0,240);
fill(255,255,255);
ellipse(starx,stary,3,3);
starx += speed;
}
// noLoop();
}
function moon(){
fill(252, 202, 70);
ellipse(350,80,100,100);
}
function drawBoat(){
var blength = random(50,100);
var bheight = random(20,30);
var bx = random(0,480);
var by = random(240,480);
this.bx += this.speed;
fill(0);
rect(bx,by,blength,bheight);
rect(bx+20,by-10,blength/2,bheight/2);
triangle(bx+blength,by+bheight,bx+blength,by,
bx+blength+30,by-10);
}
For my landscape, I wanted to depict a scene with boats and stars moving at night. In my thumbnail sketch, I divided the canvas into halves, with a sky which is the top half, and the sea at the bottom half. I thought it would be more visually interesting with objects moving on all areas of the canvas. During this project, I faced difficulty trying to animate my objects, but I solved the problem by creating multiple functions which helped me clarify my code structure and tackle specific problems. The randomness in my code exists in the size and position of the boat.
Project-11: Generative Landscape
/*Name:Camellia(Siyun) Wang;
Section: C;
Email Address: siyunw@andrew.cmu.edu;*/
//https://imgur.com/DBFXrXh coral2
//https://imgur.com/nyf5GKY coral1
//https://imgur.com/JXohvV2 group
//https://imgur.com/4hO2HW1 weed
//https://imgur.com/N4zWoA4
//https://imgur.com/J8R7qyD beach
var beaches = [];
var corals1 = [];
var corals1n = [];
var corals2 = [];
var corals2n = [];
var corals2b = [];
var groups = [];
var weeds = [];
var weeds2 = [];
var stars = [];
var fishes = [];
var bubbles = [];
var a;
var b;
var c;
var d;
var a1;
var b1;
var c1;
var d1;
function preload(){
backgroundImage = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/back.jpeg");
coral1 = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/coral1.png");
coral2 = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/coral-2.png");
group = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/group.png");
weed = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/weeds.png");
beach = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/beach.png");
star = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/starfish.png");
fish = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/fish.png");
fishschool = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/fish-school.png");
}
function setup() {
a = random(-150,300);
b = random(-150,300);
c = random(-150,300);
d = random(-150,300);
a1 = random(1,-30);
b1 = random(1,-30);
c1 = random(1,-30);
d1 = random(1,-30);
createCanvas(480, 400);
//the beach
var bea = makeBeach(1,400);
beaches.push(bea);
//coral1
var cor1 = makeCoral1(150,200);
corals1.push(cor1);
//coral1n
var cor1n = makeCoral1n(1000,175);
corals1n.push(cor1n);
//coral2
var cor2 = makeCoral2(450,75);
corals2.push(cor2);
//coral2n
var cor2n = makeCoral2n(1300,230);
corals2n.push(cor2n);
//coral2b
var cor2b = makeCoral2b(1330,190);
corals2b.push(cor2b);
//group
var gro = makeGroup(550,140);
groups.push(gro);
//weed
var wee = makeWeed(220,55);
weeds.push(wee);
//weed2
var wee2 = makeWeed2(850,30);
weeds2.push(wee2);
var star = makeStar(1200,325);
stars.push(star);
var fish = makeFish(1220,25);
fishes.push(fish);
var bubble = makeBubble(185,480);
bubbles.push(bubble);
frameRate(30);
}
//the beach
function makeBeach(xb,yb) {
var B = {
x: xb,
y: yb,
move: beachMove,
speed: -1.5,
display: beachDisplay};
return B;
}
function beachMove() {
this.x += this.speed;
}
function beachDisplay(xb) {
image(beach, this.x, 0,1400,400);
}
function updateAndDisplayBeach(){
for(var i = 0; i < beaches.length; i++){
beaches[i].move();
beaches[i].display();
}
}
function removeBeachThatHaveSlippedOutOfView(){
for (var i = 0; i < beaches.length; i++){
if (beaches[i].x < -1440) {
beaches.shift(beaches[i]);
}
}
}
function addNewBeach(){
var lastBeaches = beaches[beaches.length - 1];
if(lastBeaches.x < -900){
var a = makeBeach(480,400);
beaches.push(a);
}
}
//coral1
function makeCoral1(xc1,yc1){
var C1 = {
x: xc1,
y: yc1,
move: coral1Move,
speed: -1.5,
display: coral1Display};
return C1;
}
function coral1Move(){
this.x +=this.speed;
}
function coral1Display(xc1,yc1){
image(coral1, this.x, this.y,200,200);
}
function updateAndDisplayCoral1(){
for(var i = 0; i < corals1.length; i++){
corals1[i].move();
corals1[i].display();
}
}
function removeCoral1ThatHaveSlippedOutOfView(){
for (var i = 0; i < corals1.length; i++){
if (corals1[i].x < -1440) {
corals1.shift(corals1[i]);
}
}
}
function addNewCoral1(){
var lastCoral1 = corals1[corals1.length - 1];
if(lastCoral1.x < -900){
var a = makeCoral1(480,200);
corals1.push(a);
}
}
//coral1n
function makeCoral1n(xc1n,yc1n){
var C1n = {
x: xc1n,
y: yc1n,
move: coral1nMove,
speed: -1.5,
display: coral1nDisplay};
return C1n;
}
function coral1nMove(){
this.x +=this.speed;
}
function coral1nDisplay(xc1,yc1){
image(coral1, this.x, this.y,200,200);
}
function updateAndDisplayCoral1n(){
for(var i = 0; i < corals1n.length; i++){
corals1n[i].move();
corals1n[i].display();
}
}
function removeCoral1nThatHaveSlippedOutOfView(){
for (var i = 0; i < corals1n.length; i++){
if (corals1n[i].x < -1440) {
corals1n.shift(corals1n[i]);
}
}
}
function addNewCoral1n(){
var lastCoral1n = corals1n[corals1n.length - 1];
if(lastCoral1n.x < -900){
var a = makeCoral1n(480,175);
corals1n.push(a);
}
}
//coral2
function makeCoral2(xc2,yc2){
var C2 = {
x: xc2,
y: yc2,
move: coral2Move,
speed: -1.5,
display: coral2Display};
return C2;
}
function coral2Move(){
this.x +=this.speed;
}
function coral2Display(xc2,yc2){
image(coral2, this.x, this.y,200,200);
}
function updateAndDisplayCoral2(){
for(var i = 0; i < corals2.length; i++){
corals2[i].move();
corals2[i].display();
}
}
function removeCoral2ThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2.length; i++){
if (corals2[i].x < -1440) {
corals2.shift(corals2[i]);
}
}
}
function addNewCoral2(){
var lastCoral2 = corals2[corals2.length - 1];
if(lastCoral2.x < -900){
var a = makeCoral2(480,75);
corals2.push(a);
}
}
//coral2n
function makeCoral2n(xc2n,yc2n){
var C2n = {
x: xc2n,
y: yc2n,
move: coral2nMove,
speed: -1.5,
display: coral2nDisplay};
return C2n;
}
function coral2nMove(){
this.x +=this.speed;
}
function coral2nDisplay(xc2n,yc2n){
image(coral2, this.x, this.y,200,200);
}
function updateAndDisplayCoral2n(){
for(var i = 0; i < corals2n.length; i++){
corals2n[i].move();
corals2n[i].display();
}
}
function removeCoral2nThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2n.length; i++){
if (corals2n[i].x < -1440) {
corals2n.shift(corals2n[i]);
}
}
}
function addNewCoral2n(){
var lastCoral2n = corals2n[corals2n.length - 1];
if(lastCoral2n.x < -900){
var a = makeCoral2n(480,230);
corals2n.push(a);
}
}
//coral2b
function makeCoral2b(xc2b,yc2b){
var C2b = {
x: xc2b,
y: yc2b,
move: coral2bMove,
speed: -1.5,
display: coral2bDisplay};
return C2b;
}
function coral2bMove(){
this.x +=this.speed;
}
function coral2bDisplay(xc2b,yc2b){
image(coral2, this.x, this.y,220,220);
}
function updateAndDisplayCoral2b(){
for(var i = 0; i < corals2b.length; i++){
corals2b[i].move();
corals2b[i].display();
}
}
function removeCoral2bThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2b.length; i++){
if (corals2b[i].x < -1440) {
corals2b.shift(corals2b[i]);
}
}
}
function addNewCoral2b(){
var lastCoral2b = corals2b[corals2b.length - 1];
if(lastCoral2b.x < -900){
var a = makeCoral2b(480,190);
corals2b.push(a);
}
}
//group
function makeGroup(xg,yg){
var G = {
x: xg,
y: yg,
move: groupMove,
speed: -1.5,
display: groupDisplay};
return G;
}
function groupMove(){
this.x +=this.speed;
}
function groupDisplay(xg,yg){
image(group, this.x, this.y,350,350);
}
function updateAndDisplayGroup(){
for(var i = 0; i < groups.length; i++){
groups[i].move();
groups[i].display();
}
}
function removeGroupThatHaveSlippedOutOfView(){
for (var i = 0; i < groups.length; i++){
if (groups[i].x < -1440) {
groups.shift(groups[i]);
}
}
}
function addNewGroup(){
var lastGroup = groups[groups.length - 1];
if(lastGroup.x < -900){
var a = makeGroup(480,140);
groups.push(a);
}
}
//weed
function makeWeed(xw,yw){
var W = {
x: xw,
y: yw,
move: weedMove,
speed: -1.5,
display: weedDisplay};
return W;
}
function weedMove(){
this.x +=this.speed;
}
function weedDisplay(xw,yw){
image(weed, this.x, this.y,300,300);
}
function updateAndDisplayWeed(){
for(var i = 0; i < weeds.length; i++){
weeds[i].move();
weeds[i].display();
}
}
function removeWeedThatHaveSlippedOutOfView(){
for (var i = 0; i < weeds.length; i++){
if (weeds[i].x < -1440) {
weeds.shift(weeds[i]);
}
}
}
function addNewWeed(){
var lastWeed = weeds[weeds.length - 1];
if(lastWeed.x < -900){
var a = makeWeed(480,55);
weeds.push(a);
}
}
//weed2
function makeWeed2(xw2,yw2){
var W2= {
x: xw2,
y: yw2,
move: weed2Move,
speed: -1.5,
display: weed2Display};
return W2;
}
function weed2Move(){
this.x +=this.speed;
}
function weed2Display(xw2,yw2){
image(weed, this.x, this.y,300,300);
}
function updateAndDisplayWeed2(){
for(var i = 0; i < weeds2.length; i++){
weeds2[i].move();
weeds2[i].display();
}
}
function removeWeed2ThatHaveSlippedOutOfView(){
for (var i = 0; i < weeds2.length; i++){
if (weeds2[i].x < -1440) {
weeds2.shift(weeds2[i]);
}
}
}
function addNewWeed2(){
var lastWeed2 = weeds2[weeds2.length - 1];
if(lastWeed2.x < -900){
var a = makeWeed2(480,30);
weeds2.push(a);
}
}
//stars
function makeStar(xs,ys){
var S = {
x: xs,
y: ys,
move: starMove,
speed: -1.5,
display: starDisplay};
return S;
}
function starMove(){
this.x +=this.speed;
}
function starDisplay(xs,ys){
image(star, this.x, this.y,75,75);
}
function updateAndDisplayStar(){
for(var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function removeStarThatHaveSlippedOutOfView(){
for (var i = 0; i < stars.length; i++){
if (stars[i].x < -1440) {
stars.shift(stars[i]);
}
}
}
function addNewStar(){
var lastStar = stars[stars.length - 1];
if(lastStar.x < -900){
var a = makeStar(480,325);
stars.push(a);
}
}
//fish school
function makeFish(xf,yf){
var F = {
x: xf,
y: yf,
move: fishMove,
speed: -3.0,
display: fishDisplay};
return F;
}
function fishMove(){
this.x +=this.speed;
}
function fishDisplay(xf,yf){
image(fishschool, this.x, this.y,200,200);
}
function updateAndDisplayFish(){
for(var i = 0; i < fishes.length; i++){
fishes[i].move();
fishes[i].display();
}
}
function removeFishThatHaveSlippedOutOfView(){
for (var i = 0; i < fishes.length; i++){
if (fishes[i].x < -1440) {
fishes.shift(fishes[i]);
}
}
}
function addNewFishRandomly(){
var newFishLikelihood = 0.002;
if (random(0,2) < newFishLikelihood) {
fishes.push(makeFish(480,25));
}
}
//bubbles
function makeBubble(xb,yb){
var B = {
x: xb,
y: yb,
yspeed: -3,
move: bubbleMove,
speed: -1.5,
display: bubbleDisplay};
return B;
}
function bubbleMove(){
this.x +=this.speed;
this.y +=this.yspeed;
}
function bubbleDisplay(xb,yb){
noStroke();
fill(255);
ellipse(this.x,this.y,10,10);
ellipse(this.x+a,this.y+a1,20,20);
ellipse(this.x+b,this.y+b1,17,17);
ellipse(this.x+c,this.y+c1,23,23);
ellipse(this.x+d,this.y+d1,15,15);
}
function updateAndDisplayBubble(){
for(var i = 0; i < bubbles.length; i++){
bubbles[i].move();
bubbles[i].display();
}
}
function removeBubbleThatHaveSlippedOutOfView(){
for (var i = 0; i < bubbles.length; i++){
if (bubbles[i].x < -1440) {
bubbles.shift(bubbles[i]);
}
}
}
function addNewBubbleRandomly(){
var newBubbleLikelihood = 0.02;
if (random(0,2) < newBubbleLikelihood) {
bubbles.push(makeBubble(185,480));
}
}
function draw() {
background(backgroundImage);
updateAndDisplayBubble();
addNewBubbleRandomly();
removeBubbleThatHaveSlippedOutOfView()
updateAndDisplayWeed2();
addNewWeed2();
removeWeed2ThatHaveSlippedOutOfView();
updateAndDisplayBeach();
addNewBeach();
removeBeachThatHaveSlippedOutOfView();
updateAndDisplayWeed();
addNewWeed();
removeWeedThatHaveSlippedOutOfView();
updateAndDisplayCoral2();
addNewCoral2();
removeCoral2ThatHaveSlippedOutOfView();
updateAndDisplayFish();
addNewFishRandomly();
removeFishThatHaveSlippedOutOfView();
updateAndDisplayCoral2b();
addNewCoral2b();
removeCoral2bThatHaveSlippedOutOfView();
updateAndDisplayCoral2n();
addNewCoral2n();
removeCoral2nThatHaveSlippedOutOfView();
updateAndDisplayGroup();
addNewGroup();
removeGroupThatHaveSlippedOutOfView();
image(fish,140,100,200,230);
updateAndDisplayCoral1();
addNewCoral1();
removeCoral1ThatHaveSlippedOutOfView();
updateAndDisplayCoral1n();
addNewCoral1n();
removeCoral1nThatHaveSlippedOutOfView();
updateAndDisplayStar();
addNewStar();
removeStarThatHaveSlippedOutOfView();
}
I find the code of this project not too complicated but rather a bit complex, because I need to create many objects to draw on the canvas. Also, the most difficult part is the first object. After successfully make the first object work, the others are much the same with subtle differences. The landscape I created is an under ocean landscape, narrating a fish’s journey wandering around.
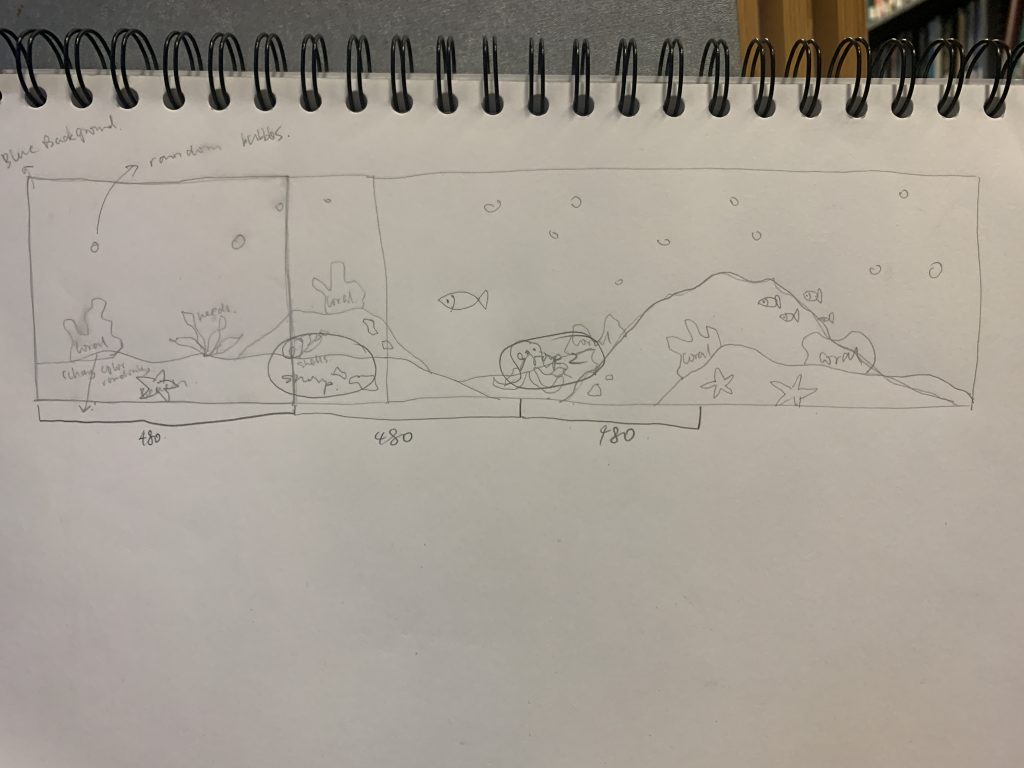