jmmedenb-10c
//Jessica Medenbach
//jmmedenb@andrew.cmu.edu
//Tuesdays at 1:30PM
//Assignment-10c
var balloons = [];
var tSpeed = 0.0001;
var tDetail = 0.003;
function setup() {
createCanvas(600, 400);
// create an initial collection of fish
for (var i = 0; i < 10; i++){
balloons[i] = makeBalloons(random(width),random(height-100));
}
frameRate(12);
}
function draw() {
var cB = 20;
x = map(cB,0,height,255,0);
background(255);
//sea
beginShape();
fill(100,255,255);
vertex(0,height);
for (var x = 0; x < width; x++) {
var t = (x * tDetail) + (millis() * tSpeed);
var y = map(noise(t), 1,0,100, height);
vertex(x, y);
}
vertex(width,height);
endShape(CLOSE);
updateAndDisplayBalloons();
removeBalloons();
addNewBalloons();
}
//boats
function updateAndDisplayBalloons(){
for (var i = 0; i < balloons.length; i++){
balloons[i].move();
balloons[i].display();
}
}
function removeBalloons(){
var balloonsToKeep = [];
for (var i = 0; i < balloons.length; i++){
if (balloons[i].x + balloons[i].bodySize > 0) {
balloonsToKeep.push(balloons[i]);
}
}
balloons = balloonsToKeep; // only keeps ballons on screen
}
function addNewBalloons() {
// adds new ballons
var newBalloonsLikelihood = 0.02;
if (random(0,1) < newBalloonsLikelihood) {
balloons.push(makeBalloons(width,random(2,height-100)));
}
}
// updates of balloons
function balloonsFloat() {
this.x += this.speed;
}
// balloons
function balloonsDisplay() {
noStroke();
push();
fill(255,100,100);
ellipse(this.x, this.y, this.bodySize, this.bodyWidth);
rect(this.x, this.y+10, 10, 20);
pop();
fill(0);
rect(this.x+5, this.y+30, 1, 30);
}
function makeBalloons(LocationX,LocationY) {
var balloons = {x: LocationX+300,
y: LocationY+275,
bodyWidth: (50,50),
bodySize: (50,50),
speed: random(-3.0,-1.0),
colorR: random(130,240),
colorG: random(20,80),
move: balloonsFloat,
display: balloonsDisplay}
return balloons;
}
I started out wanting to make boats for this project but then started thinking about things that get lost at sea, such as bottles or objects. Then I started thinking of when people accidentally let go of balloons, or events for children where everyone is given a balloon and they all inevitably accidentally let go of them and you just see a sea of balloons in the sky. I remember as a kid wondering where they went. I put together these two thoughts and decided to make a sea of balloons in the sea. I then decided to create a moving image of watching the sea of balloons floating together, perhaps people see them, perhaps they don’t, but they’re there. Maybe the sea is where all the lost balloons end up. 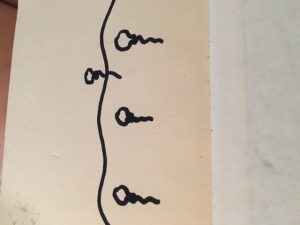