The project that interests me a lot is “4900 Colors” series by Gerhard Richter. The version II consists of 49 plates of 10×10 grids. A computer assigned random colors to each grid. And together they make up a huge color pattern. I find it interesting for it reveals some “pattern”s inside randomness and the weakness of human eye to distinguish colors. For example, each plate contains approximately 4 grids of black and at least one grid of white. If the colors the computer assigned are based on true color system, the chances that there are 4 black grids out of 100 is subtle( that would be 1/256^12). One way to explain this may be our eyes can hardly distinguish a “true” black from dark blue, dark red, etc.
Author: Xiangqi Liu
Liu Xiangqi-Project 06-Abstract Clock
I intended to imitate an hourglass. Use ellipses to represent sands(second), larger ellipse in the bottom to represent the minutes, and the angles of the tilting to represent hours. But I found it extremely difficult to correlate the number of sands with the seconds since there is no suitable arithmetic progression whose sum is 60. So I gave up. Then I realized some abstract shapes can have some fantastic effect as we did in string art. So I designed this clock. The strong contrast in color also makes some interesting effect when they overlap with each other.
sketch
var strokeR = 204;
var strokeG = 230;
var strokeB = 255;
function setup() {
createCanvas(600, 600);
frameRate(1);
}
function draw() {
background(0);
strokeWeight(1);
noFill();
//rotate ellipse to represent seconds
push();
translate(width/2, height/2);
for (var i = 0; i < second(); i ++) {
strokeR = map(i, 0, 60, 255, 51);
strokeG = map(i, 0, 60, 51, 204);
strokeB = map(i, 0, 60, 0, 204);
stroke(strokeR, strokeG, strokeB);
rotate(radians(3));
ellipse(0, 0, 30, 540);
}
pop();
//increase ellipse to represent minutes
for (var j = 0; j < minute(); j ++){
strokeR = map(j, 0, 60, 204, 255);
strokeG = map(j, 0, 60, 153, 255);
strokeB = map(j, 0, 60, 255, 255);
stroke(strokeR, strokeG, strokeB);
ellipse(300, 300, 9*j, 9*j);
}
//increase squares to represent hours
for (var k = 0; k < hour(); k ++){
strokeR = map(k, 0, 60, 255, 255);
strokeG = map(k, 0, 60, 255, 255);
strokeB = map(k, 0, 60, 102, 255);
stroke(strokeR, strokeG, strokeB);
quad(300, 30 +22.5*k, 30 + 22.5*k, 300, 300, 570-22.5*k, 570 - 22.5*k, 300);
}
}
Liu Xiangqi-Looking Outwards-05
Ed Catmull, from Pixar, invented the texture mapping technique in 1974. It is regarded as a breakthrough in 3D Computer Graphic. I admire this because it evokes sense of touching by sight and gives more vivid feeling to a flat object. The technique is widely used in animation. It brings life to millions of cartoon characters. Texture coordinates(generated by some functions) are added to the original vertex. Besides that, colors are changed accordingly. Several techniques are evolved including forward texture mapping, inverse texture mapping, affine texture mapping, etc. It is still in progress for it is not capable of accomplishing some complicated surfaces vividly.
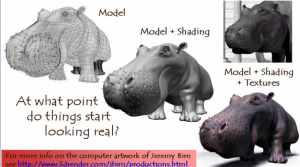
More texture mapping knowledge from CMU
Liu Xiangqi-Project-05
// Liu Xiangqi xiangqil@andrew.cmu.edu Section A Project-05
function setup() {
createCanvas(600, 600);
background(0);
noLoop();
}
function draw() {
var y = 0;
var x = 0;
for (y = 0; y + 50 < height - 50; y += 100){
for (x = 0; x + 50 < width - 50; x += 100){
//draw the roses
fill(255);
ellipse(50+x, 50+y, 10, 10);
stroke(255, 77, 77);
strokeWeight(6);
noFill();
for(var i = 1; i < 11; i ++){
if (i%4 == 1) {
arc(50+x, 50+y, 4*i + 12.5, 4*i + 12.5, 0, HALF_PI);
}else if (i%4 == 2) {
arc(50+x, 50+y, 4*i + 20, 4*i + 20, HALF_PI, PI);
}else if (i%4 == 3) {
arc(50+x, 50+y, 4*i + 27.5, 4*i + 27.5, PI, PI+HALF_PI);
}else{
noFill();
arc(57+x, 45+y, 4*i + 5, 4*i + 5, PI+HALF_PI, TWO_PI+QUARTER_PI);
}
}
//draw the leaves
fill(204, 255, 153);
noStroke();
beginShape();
vertex(50+x, 80+y);
curveVertex(50+x, 80+y);
curveVertex(80+x, 100+y);
curveVertex(90+x, 120+y);
curveVertex(80+x, 110+y);
curveVertex(50+x, 80+y);
vertex(50+x, 80+y);
endShape();
}
}
}
Liu Xiangqi-Project-04-String Art
// Liu Xiangqi xiangqil@andrew.cmu.edu Section-A Project-04
var strokeR = 204;
var strokeG = 230;
var strokeB = 255;
function setup(){
createCanvas(640, 480);
}
function draw() {
background(0);
//draw the outer ring
var x1 = 320;
var y1 = 0;
var x2 = 320-120*sqrt(3);
var y2 = 360;
var x3 = 320+120*sqrt(3);
var y3 = 360;
stroke(255);
noFill();
triangle(x1, y1, x2, y2, x3, y3);
push();
translate(width/2, height/2);
for (var i= 0; i < 60; i ++) {
strokeR = 204;
strokeG = map(i, 0, 30, 230, 153);
strokeB = 255;
stroke(strokeR, strokeG, strokeB);
rotate(radians(2));
triangle(x1-width/2, y1-height/2, x2-width/2, y2-height/2, x3-width/2, y3-height/2);
}
pop();
//draw the inner ring
var x4 = 320;
var y4 = 360;
var x5 = 320 - 60*sqrt(3);
var y5 = 180;
var x6 = 320 + 60*sqrt(3);
var y6 = 180;
stroke(255);
noFill();
triangle(x4, y4, x5, y5, x6, y6);
push();
translate(width/2, height/2);
for (var i= 0; i < 40; i ++) {
strokeR = map(i, 0, 30, 204, 255);
strokeG = map(i, 0, 30, 153, 204);
strokeB = map(i, 0, 30, 255, 204);
stroke(strokeR, strokeG, strokeB);
rotate(radians(3));
triangle(x4-width/2, y4-height/2, x5-width/2, y5-height/2, x6-width/2, y6-height/2);
}
pop();
//draw the innermost ring
var x7 = 320;
var y7 = 180;
var x8 = 320 - 30*sqrt(3);
var y8 = 270;
var x9 = 320 + 30*sqrt(3);
var y9 = 270;
stroke(255);
noFill();
triangle(x7, y7, x8, y8, x9, y9);
push();
translate(width/2, height/2);
for (var i= 0; i < 30; i ++) {
strokeR = 255
strokeG = map(i, 0, 30, 255, 204);
strokeB = map(i, 0, 30, 255, 204);
stroke(strokeR, strokeG, strokeB);
rotate(radians(4));
triangle(x7-width/2, y7-height/2, x8-width/2, y8-height/2, x9-width/2, y9-height/2);
}
pop();
}
Liu Xiangqi-Looking Outwards-05
“Tilt Brush” is launched by Google, which enables users to paint sound. I think this project rather interesting because vision is still the more intuitive sense to human than other forms. Emotions can be expressed more clearly through visual effects. By converting sounds to images, users can understand the sound more.
The qualities of sound can be divided into volume, tone and tune, as images can be expressed by different colors, shapes and composition. I think the developers might have connect these qualities accordingly–use different qualities of sound to control their counterparts in images.
Information in vision can be more prominent than in hearing, so users might experience the sound more thoroughly.
Here is the link for the project.
The Tilt Brush by Google
Liu Xiangqi-Project03
// Liu Xiangqi xiangqil@andrew.cmu.edu Section-A Project-03
var backR = 255;
var backG = 219;
var backB = 77;
var radian = 0;
var size = 150;
var roundR = 255;
var roundG = 102;
var roundB = 0;
function setup() {
createCanvas(640, 480)
}
function draw() {
background(backR, backG, backB);
noStroke();
//change the background color
backR = map(mouseX, 0, width, 255, 0);
backG = map(mouseX, 0, width, 219, 20);
backB = map(mouseX, 0, width, 77, 51);
if (mouseX > width ) {
backR = 0;
backG = 20;
backB = 51;
}
if (mouseX < 0) {
backR = 255;
backG = 219;
backB = 77;
}
//change the position of the moon/sun
push();
radian = map(mouseX, 0, width, 0, 1.57);
translate(320,0);
rotate(radian);
//change the color
roundR = map(mouseX, 0, width, 255, 255);
roundG = map(mouseX, 0, width, 102,255);
roundB = map(mouseX, 0, width, 0, 0);
fill(roundR, roundG, roundB);
ellipse(200, 75, 150, 150);
//change the size of the breach
size = map(mouseX, 0, width, 0, 150);
fill(backR, backG, backB)
ellipse(200, 150, size, size);
pop();
}
I don’t know why the moon stuck in the middle of the canvas. It moves smoothly to the upper-left corner on my browser.
Liu Xiangqi-Lookingoutwards-03
The project “A Unified Approach to Grown Structures” by MIT Media Lab interests me a lot. It utilizes computational approach and associated protocol to imitate growth-a natural process. I admire this a lot because it reminds people of a neglected truth that growth is achieved by duplication of cells and mutations. And also it challenges the definition of life–if life can be artificial? Is life developed randomly? The growth procedure is essentially a deformation and iteration. The initial geometric representation is deformed by data from geometric input representation(phenotype), intermediate representation(genotype) and a coarse implicit representation(developed from two previous representations). As the input representation changes, the deformation is repeated.
A Unified Approach to Grown Structures by MIT Media Lab
Liu Xiangqi-Looking Outwards-02
This work is from Australian Artist Lia. Her work combines the traditional drawing styles and digital techniques and aesthetics. The reason I appreciate her work is that I believe modern art needs to be understood and appreciated by the public. Abstraction is not working well to connect with the audiences.
This work is created for Fabasoft website themes. Through a series, she tries to convey themes of Serenity, Trust and Respect. Her work however, contains some concrete patterns–dandelion in this work. And the texture of color pencil and feather-like strokes can be felt vividly. I feel peacefulness and harmony for consistency in color and non-edge shapes. Variation exists in size of the shape and brightness of the color.
Liu Xiangqi Project-02-Variable-Face
// Liu Xiangqi xiangqil@andrew.cmu.edu Section-A Project-02
var eyeSize = 20;
var faceWidth = 100;
var faceHeight = 150;
var eyeR = 0;
var eyeG = 0;
var eyeB = 0;
var faceR = 255;
var faceG = 230;
var faceB = 230;
var noseX1 = 320;
var noseY1 = 240;
var noseX2 = 310;
var noseY2 = 260;
var noseX3 = 330;
var noseY3 = 260;
var mouthAlpha = 0.5;
var mouthX1 = 310;
var mouthY1 = 280;
var mouthX2 = 315;
var mouthY2 = 275;
var mouthX3 = 320;
var mouthY3 = 280;
var mouthX4 = 325;
var mouthY4 = 275;
var mouthX5 = 330;
var mouthY5 = 280;
var mouthX6 = 325;
var mouthY6 = 290;
var mouthX7 = 315;
var mouthY7 = 290;
var mouthX8 = mouthX1;
var mouthY8 = mouthY1;
function setup() {
createCanvas(640, 480);
}
function draw() {
colorMode(RGB,255,255,255,1);
background(255, 204, 204);
//draw face
noStroke();
fill(faceR, faceG, faceB, 0.3);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
//contour of the eye
fill(255);
ellipse(eyeLX, height / 2, eyeSize+10, eyeSize);
ellipse(eyeRX, height / 2, eyeSize+10, eyeSize);
//change eye color
fill(eyeR, eyeG, eyeB);
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
//contour of the nose
noFill();
stroke(255);
strokeWeight(3.0);
strokeJoin(ROUND);
beginShape();
vertex(noseX1, noseY1);
vertex(noseX2, noseY2);
vertex(noseX3, noseY3);
endShape();
//draw the mouth
fill(255,0,0,mouthAlpha);
noStroke();
beginShape();
curveVertex(mouthX1, mouthY1);
curveVertex(mouthX1, mouthY1);
curveVertex(mouthX2, mouthY2);
curveVertex(mouthX3, mouthY3);
curveVertex(mouthX4, mouthY4);
curveVertex(mouthX5, mouthY5);
curveVertex(mouthX6, mouthY6);
curveVertex(mouthX7, mouthY7);
curveVertex(mouthX8, mouthY8);
curveVertex(mouthX8, mouthY8);
endShape();
}
function mousePressed() {
faceWidth = random(75, 150);
faceHeight = random(130, 200);
eyeSize = random(10, 20);
//randomize eye color;
eyeR = random(0, 250);
eyeG = random(0, 250);
eyeB = random(0, 250);
//random face color according to a research of human skin color
faceR = 168.8 + 38.5*random(-3,3);
faceG = 122.5 + 3.1*random(-3,3);
faceB = 96.7 + 6.3*random(-3,3);
//randomize nose size;
noseX1 = random(317, 323);
noseY1 = random(235, 245);
noseX2 = random(305, 315);
noseY2 = random(255, 265);
noseX3 = random(325, 335);
noseY3 = noseY2;
//randomize mouth color and shape
mouthAlpha = random(0.1, 1);
mouthX1 = random(307, 313);
mouthX2 = random(314, 316);
mouthX3 = random(318, 322);
mouthX4 = random(324, 326);
mouthX5 = random(327, 333);
mouthX6 = random(323, 327);
mouthX7 = random(313, 317);
mouthY1 = random(275, 285);
mouthY2 = random(273, 277);
mouthY3 = mouthY1;
mouthY4 = mouthY2;
mouthY5 = mouthY1;
mouthY6 = random(287, 293);
mouthY7 = mouthY6;
}
I found some problem when I tried to modify the size of the canvas.