sketch
//Mairead Dambruch
//mdambruc@andrew.cmu.edu
//Section C
//Project-11-A
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
var hotdog = [];//hotdog array
var turtle1;
var startFrame;
var lightening;//sound file
function preload(){
// lightening2 = loadSound("lightening2.wav");//background sound
}
function setup() {
createCanvas(800, 400);
background(0);
// lightening2.play(0, 1, 2);//background sound
for (var i = 0; i < 100; i++){
var hotdogplace = random(width);
hotdog[i]= makehotdog(hotdogplace);
turtle1 = makeTurtle(mouseX, mouseY);//create turtle
turtle1.setColor(color(255));
turtle1.setWeight(2);
turtle1.penDown();//creating lightening turtle
resetCanvas();
frameRate(100);
}
}
function draw() {
//creating striped background
colorMode(RGB);
noStroke();
from = color(48, 138, 191);
to = color(200, 255, 50);
interA = lerpColor(from, to, .33);
interB = lerpColor(from, to, .66);
fill(from);
rect(0, 0, width, 100);
fill(interA);
rect(0, 100, width, 200);
fill(interB);
rect(0, 200, width, 300);
fill(to);
rect(0, 300, width, 400);
sloth();
updateandcreatehotdogs();
removehotdog();
addnewhotdog();
showhotdog();
makehotdog();
//draws lightening
var step = (random(width) - random(height));
turtle1.forward(step);
turtle1.left(1000);
if (turtle1.y > width || turtle1.x > height)
resetCanvas();
}
function resetCanvas(){//creates flashing background after turtle is drawn
background(0);
startFrame = frameCount;
turtle1.penUp();
turtle1.goto(400, 300);
turtle1.penDown();
}
function updateandcreatehotdogs(){
for (var i = 0; i < hotdog.length; i = i + 20){
hotdog[i].move();
hotdog[i].display();
}
}
function removehotdog(){ //removes hotdogs after past canvas
var hotdogToKeep = [];
for (var i = 0; i < hotdog.length; i++){
if (hotdog[i].x + hotdog[i].breadth > 0) {
hotdogToKeep.push(hotdog[i]);
}
}
hotdog = hotdogToKeep;
}
function addnewhotdog() {
var newhotdogProbability = 0.5;
if (random(0, 1) < newhotdogProbability) {
hotdog.push(makehotdog(width));
}
}
function hotdogmove() {
this.x += this.speed;
}
function showhotdog() {
var hotdogHeight = 10;
push();
noStroke();
//draws four lines of hotdogs
translate(this.x, 30);
fill(255, 111, 91);
ellipse(0, hotdogHeight, 25, 7);
rectMode(RADIUS);
fill(240, 182, 66);
rect(0, hotdogHeight+2, 8, 3);
translate(this.x, 80);
fill(255, 111, 91);
ellipse(0, hotdogHeight, 25, 7);
rectMode(RADIUS);
fill(240, 182, 66);
rect(0, hotdogHeight+2, 8, 3);
translate(this.x, 100);
fill(255, 111, 91);
ellipse(0, hotdogHeight, 25, 7);
rectMode(RADIUS);
fill(240, 182, 66);
rect(0, hotdogHeight+2, 8, 3);
translate(this.x, 130);
fill(255, 111, 91);
ellipse(0, hotdogHeight, 25, 7);
rectMode(RADIUS);
fill(240, 182, 66);
rect(0, hotdogHeight+2, 8, 3);
pop();
}
function makehotdog(LocationX) {
var hdog = {x: LocationX,
breadth: 1000,
speed: -1.0,
nstars: round(random(2,8)),
move: hotdogmove,
display: showhotdog}
return hdog;
}
function sloth(x, y){
//draws sloth
x = 400;
y = 350;
noStroke();
fill(140, 70, 0);
ellipse(x, y, 20, 50);
ellipse(x, y - 40, 30, 30);
ellipse(x, y + 10, 35, 30);
//face and body
ellipse(x + 7, y + 20, 10, 20);
ellipse(x - 7, y + 20, 10, 20);
fill(255, 218, 117);
ellipse(x, y - 40, 20, 20);
//innerface
fill(214, 135, 32);
ellipse(x, y - 40, 17, 8);
ellipse(x, y - 37, 10, 10 )
//surrounding eye area
fill(0);
ellipse(x - 5, y - 40, 5, 5);
fill(0);
ellipse(x + 5, y - 40, 5, 5);
//eyes
fill(0);
ellipse(x + 1, y - 37, 2, 2);
fill(0);
ellipse(x - 1, y - 37, 2, 2);
//nostrils
noStroke();
fill(255, 0, 0);
arc(x, y-34, 5, 5, TWO_PI, PI, CHORD);
//mouth
}
For this project, I created a sloth-like creature that is continuously getting electrically shocked in the head by lightening in a hotdog storm. The lightening is created by turtles. I originally had lightening sounds that accompanied this project but was unable to have that due to the need of a local server.
Here is a screenshot of the project
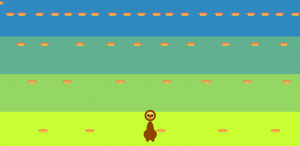