Although I wouldn’t say that this project is polished or complete, I think it was a great learning opportunity for me. While playing around with a turtle, or many turtles, creating hundreds of thin lines, I realized that turtle graphics (or at least that style of turtle graphics) allowed me to create an entirely different aesthetic — one reminiscent of hand drawing.
This is a bit ironic, since the final product is not that reminiscent of hand drawing…
After I had gotten all excited about making these pencil sketch images, I began to play around with more abstract drawings. There were a bunch of these that I was playing around with, and honestly I’m not sure which I like best compositionally, but the last one I worked on was this eyeball-esque shape.
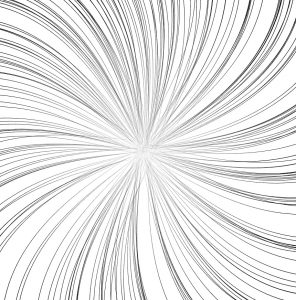
The program takes a bunch of straight lines, and bends them towards the center, creating the illusion of a hard circle filled with a bunch of ellipses.
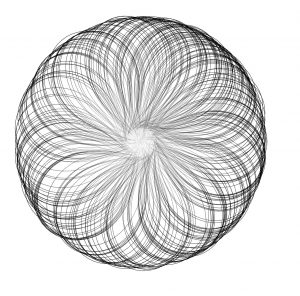
Then, as the line reaches the center, sin() switches from positive to negative, and it bends in the other direction, moving out towards the parameter.
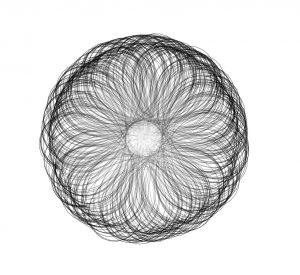
The lines then proceed to curl again towards the center, creating a sort of loose rim around the circle. I enjoy the slowing effect as the line curls, since it creates the feeling of tension or elasticity. I hope you enjoy!
//Jonathan Perez
//Section B
//jdperez@andrew.cmu.edu
//Project 11
var t1; //turtle
var r0 = [] //initialized random radii from center
var face1 = [] //initialized random face
var face2 = [] //second initialzed face before drawing line
var w = .25 //starting angle
function curl(d) {
//function that produces the furling and unfurling effect
//the w variable is incremented to return values from 1 to -1 smoothly
//and the value is scaled by d / 9
return sin(2 * PI * (w)) * d / 9;
}
function drawLine() {
t1.penDown();
for (var i = 0; i < 53; i++) {
var d = t1.distanceTo(240, 240); //turtle distance from center
t1.setWeight(d / 400 + 0.00); //line thickens as it gets further from center
t1.forward(10);
t1.left(curl(d)); //
if (t1.x > width || t1.x < 0 || t1.y > height || t1.y < 0) {
return;
}
}
}
function setup() {
createCanvas(480, 480);
t1 = makeTurtle(240, 240);
for (var i = 0; i < 250; i++) {
//create the starting points for each line
face1.push(random(360));
r0.push(random(0 + random(20)));
face2.push(random(360));
}
}
function draw() {
//background(200, 200, 170);
background(255)
//fill(0);
//ellipse(width/2, height/2, 37, 37)
t1.setColor(0);
t1.setWeight(0.3);
for (var i = 0; i < 250; i++) {
t1.penUp();
//go to respective starting position
t1.goto(width/2, height/2);
t1.face(face1[i]);
t1.forward(r0[i]);
t1.face(face2[i]);
//draw line
drawLine();
}
w += .0025
}
//------------------------------------------
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}