Landscape
// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section D
// Project-10: Generative Landscape
var clouds = [];
var birds = [];
var frames = [];
var mS1 = 0.0003;
var mD1 = 0.03;
var mS2 = 0.0005;
var mD2 = 0.01;
function preload(){
// Images for the bird flight cycle
var filenames = [];
filenames[0] = "https://i.imgur.com/RXatjYL.png";
filenames[1] = "https://i.imgur.com/tUdWerm.png";
filenames[2] = "https://i.imgur.com/4RK7a5B.png";
filenames[3] = "https://i.imgur.com/tUdWerm.png";
filenames[4] = "https://i.imgur.com/RXatjYL.png";
filenames[5] = "https://i.imgur.com/yZgWcpm.png";
filenames[6] = "https://i.imgur.com/wT9v4PU.png";
filenames[7] = "https://i.imgur.com/0Ss2jc0.png";
// Load images into frames array for birds
for(i = 0; i < filenames.length; i++){
frames[i] = loadImage(filenames[i]);
}
}
function setup(){
createCanvas(480, 320);
frameRate(10);
}
function draw(){
Sky(0, 0, width, height);
makeMountain();
makeBird();
updateAndDisplayClouds();
removeCloudsThatHaveSlippedOutOfView();
addNewCloudsWithSomeRandomProbability();
updateAndDisplayBirds();
addNewBirdsWithSomeRandomProbability();
Airplane();
}
function Sky(x, y, w, h) {
//Draws the gradient sky
var c1, c2;
c1 = color(251, 234, 192);
c2 = color(118, 85, 45);
for (var i = y; i <= y + h; i++) {
var inter = map(i, y, y + h, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(x, i, x + w, i);
}
}
function updateAndDisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function updateAndDisplayBirds(){
for (var i = 0; i < birds.length; i++){
birds[i].move();
birds[i].display();
}
}
function removeCloudsThatHaveSlippedOutOfView(){
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep;
}
function addNewCloudsWithSomeRandomProbability(){
// Spawn a new cloud to the right edge of the canvas
var newCloudLikelihood = 0.02; //clouds are spawned relatively often
if (random(0,1) < newCloudLikelihood) {
clouds.push(makeCloud(width));
}
}
function addNewBirdsWithSomeRandomProbability(){
// Spawn a new bird to the right edge of the canvas
var newBirdLikelihood = 0.005; //birds spawn less frequently than the clouds
if (random(0,1) < newBirdLikelihood) {
birds.push(makeBird(width));
}
}
function cloudMove(){
this.x += this.speed;
}
function birdMove(){
this.x += this.speed;
}
function cloudDisplay() {
var cHeight = this.altitude * 25;
push();
translate(this.x, height - 260);
// Draws Clouds of various sizes
fill(191, 175, 159);
// Variables randomize the positioning and size of the clouds
ellipse(0, -cHeight, this.breadth, cHeight-20);
ellipse(-10, -cHeight+10, this.breadth*.8, cHeight-20);
ellipse(25, -cHeight+5, this.breadth, cHeight-20);
ellipse(15, -cHeight+15, this.breadth*1.2, cHeight-20);
pop();
}
function birdDisplay(){
push();
translate(this.x, this.height);
for (i = 0; i< this.size; i++){
var frame = frameCount % 5; //cycles through bird images
image(frames[frame + i],this.x-this.disX*i,0-this.disY*i);
}
pop();
}
function makeCloud(birthLocationX) {
var clouds = {x: birthLocationX,
breadth: 60,
speed: -1.2,
altitude: round(random(-1,2)),
move: cloudMove,
display: cloudDisplay}
return clouds;
}
function makeBird(birthLocationX){
var birds = {x: birthLocationX,
disX: random(15, 40),
disY: random(30),
size:floor(random(1, 5)),
speed: random(-5, -2),
height: round(random(75, 100)),
move: birdMove,
display: birdDisplay}
return birds;
}
function makeMountain(){
// Generates the terrain
// Larger background mountains
noStroke();
fill(98, 89, 79);
beginShape();
for(x1 = 0; x1 < width; x1++){
var t1 = (x1 * mD1) + (millis() * mS1);
var y1 = map(noise(t1), 0, 1, 0, height);
vertex(x1, y1 - 10);
}
vertex(width,height);
vertex(0,height);
endShape();
// Middlegorund mountains
fill(63, 57, 53);
beginShape();
for(x2 = 0; x2 < width; x2++){
var t2 = (x2 * mD2) + (millis() * mS2);
var y2 = map(noise(t2), 0, 1, 0, height);
vertex(x2, y2 + 80);
}
vertex(width,height);
vertex(0,height);
endShape();
}
function Airplane(){
// Wing
noStroke();
fill(193, 173, 146);
quad(401, 247, 176, 124, 208, 121, 454, 175);
fill(67, 63, 52);
quad(401, 247, 200, 146, 228, 143, 444, 232);
fill(73, 58, 47);
triangle(176, 124, 208, 121, 187, 129);
fill(68, 39, 18);
quad(176, 124, 208, 121, 158, 70, 147, 66);
// Window Frame
beginShape();
noStroke();
fill(23, 16, 16);
vertex(0, 0);
vertex(0, 320);
vertex(480, 320);
vertex(480, 0);
vertex(450, 0);
vertex(430, 158);
vertex(426, 177);
vertex(416, 203);
vertex(400, 237);
vertex(373, 270);
vertex(350, 283);
vertex(333, 290);
vertex(295, 300);
vertex(250, 307);
vertex(211, 309);
vertex(163, 302);
vertex(121, 294);
vertex(87, 275);
vertex(56, 245);
vertex(39, 203);
vertex(20, 0);
endShape();
// Window Frame Highlight
beginShape();
noStroke();
fill(86, 66, 59);
vertex(0, 90);
vertex(19, 214);
vertex(31, 255);
vertex(44, 277);
vertex(59, 292);
vertex(67, 299);
vertex(86, 312);
vertex(97, 320);
vertex(81, 320),
vertex(70, 312);
vertex(51, 299);
vertex(43, 292);
vertex(28, 277);
vertex(15, 255);
vertex(0, 214);
endShape();
beginShape();
vertex(480, 52);
vertex(467, 109);
vertex(456, 182);
vertex(447, 226);
vertex(428, 261);
vertex(411, 279);
vertex(378, 304);
vertex(347, 320);
vertex(363, 320);
vertex(394, 304);
vertex(427, 279);
vertex(444, 261);
vertex(463, 226);
vertex(472, 182);
vertex(480, 109);
endShape();
}
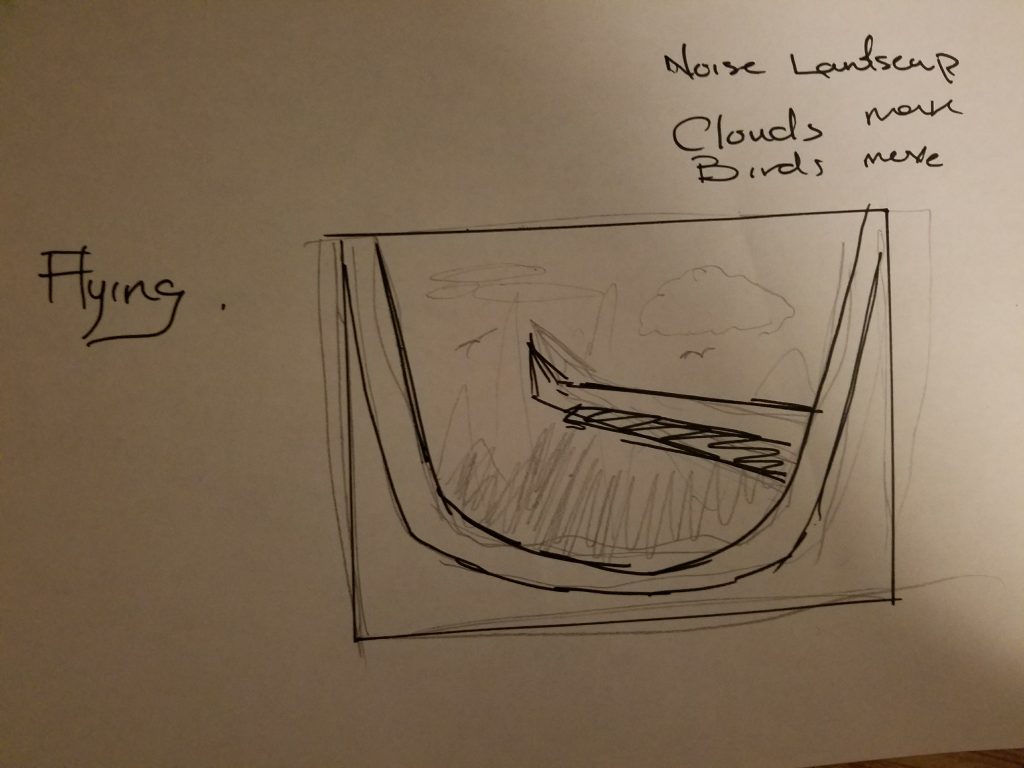
I really wanted to show the view from a plane, because I love flying. I think there is something very calming about being in the air and I wanted my project to encapsulate this idea. Being in the air offers new perspectives, and I would love it if I could fly. Added some birds, clouds and mountains in the background.
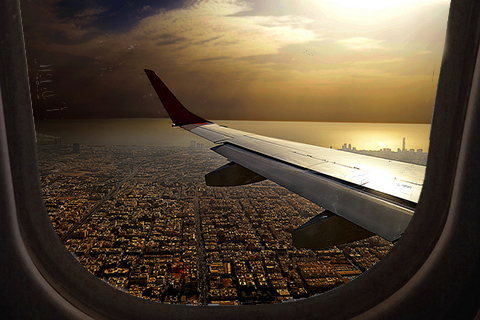