The idea for this project was to try and represent the time of day by paralleling it with the subsequent way someone feels during the day, and match the way they look as a day progresses. The result was a person who looks tired in the morning, starts to liven up around noon and in the afternoon, and then gradually gets tired again and falls asleep. The background also matches the color of the sky as time passes by.
Here are photos of all the different stages: 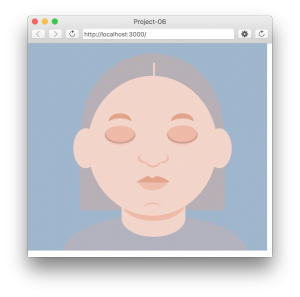
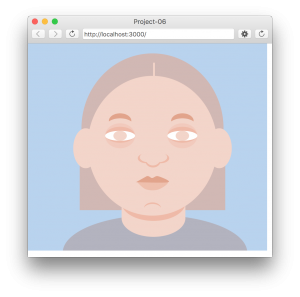
dawn morning
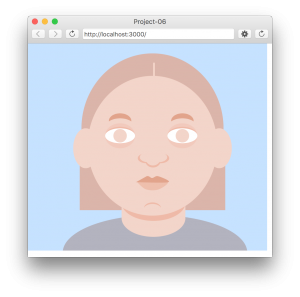
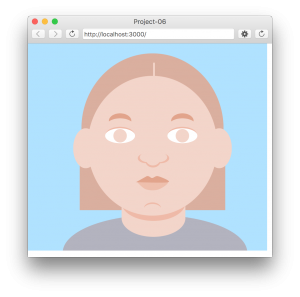
noon afternoon
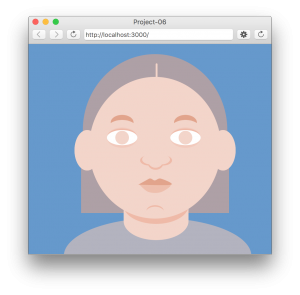
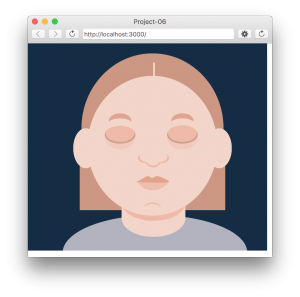
evening dusk
sketch
var r = (0);
var g = (0);
var b = (0);
var dawn = (5);
var morning = (7);
var noon = (12);
var afternoon = (13);
var evening = (18);
var dusk = (22);
skinbaseR = (243);
skinbaseG = (213);
skinbaseB = (202);
function setup() {
createCanvas(480,415);
}
function draw() {
var h = hour();
var m = minute();
var s = second();
background(r,g,b);
if (dawn <= h & h < morning){
dawnImage();
}
else if (morning <= h & h < noon){
morningImage();
}
else if (noon <= h & h < afternoon){
noonImage();
}
else if (afternoon <= h & h < evening){
afternoonImage();
}
else if (evening <= h & h < dusk){
eveningImage();
}
else if (dusk <= h & h < 24 ){
duskImage();
}
else if (0 <= h < dawn){
duskImage();
}
}
function dawnImage() {
r = (159);
g = (182);
b = (205);
var m = minute();
var s = second();
var ms = millis();
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28)
ellipse(252,350,128,75)
fill(239,186,168)
ellipse(252,318,129,75)
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140)
ellipse(310,155,45,30)
ellipse(185,155,45,30)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,165,55,30)
ellipse(185,165,55,30)
fill(239,186,168,100);
ellipse(185,185,60,40);
ellipse(310,185,60,40);
fill(239,186,168)
stroke(207,159,143);
ellipse(185,183,58,32);
noStroke();
ellipse(185,180,58,30);
stroke(207,159,143)
ellipse(310,183,58,32);
noStroke();
ellipse(310,180,58,32);
fill(255)
ellipse(185,185,60,0)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(185,185,27,0)
fill(255)
ellipse(310,185,60,0)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,0)
fill(239,186,168)
ellipse(251,236,30,25)
ellipse(238,230,35,20)
ellipse(266,230,35,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(242,228,35,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(262,228,35,20)
ellipse(251,230,30,29)
fill(239,186,168)
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250,333,36,23);
push();
translate(-52,50)
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
if (ms%3===0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
function morningImage() {
r = (185);
g = (211);
b = (238);
m = minute();
s = second();
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28)
ellipse(252,350,128,75)
fill(239,186,168)
ellipse(252,318,129,75)
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140)
ellipse(310,155,45,30)
ellipse(185,155,45,30)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,165,55,30)
ellipse(185,165,55,30)
fill(239,186,168,100);
ellipse(185,185,60,50);
ellipse(310,185,60,50);
fill(239,186,168)
ellipse(185,183,58,32);
ellipse(310,183,58,32);
fill(255)
ellipse(185,185,60,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(185,185,27,20)
fill(255)
ellipse(310,185,60,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,20)
fill(239,186,168)
ellipse(251,236,30,25)
ellipse(238,230,35,20)
ellipse(266,230,35,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(242,228,35,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(262,228,35,20)
ellipse(251,230,30,29)
fill(239,186,168)
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250,333,36,23);
push();
translate(-52,50)
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
function noonImage() {
r = (198);
g = (226);
b = (255);
m = minute();
s = second();
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
fill(239,186,168);
ellipse(252,318,129,75);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140);
ellipse(310,155,45,30);
ellipse(185,155,45,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
ellipse(185,165,55,30);
fill(239,186,168,100);
ellipse(185,185,60,50);
ellipse(310,185,60,50);
fill(239,186,168);
ellipse(185,183,58,32);
ellipse(310,183,58,32);
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,27);
fill(239,186,168);
ellipse(251,236,30,25);
ellipse(238,230,35,20);
ellipse(266,230,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
ellipse(251,230,30,29);
fill(239,186,168);
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(0.65,0.65);
translate(27,-10);
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
function afternoonImage() {
r = (176);
g = (226);
b = (255);
m = minute();
s = second();
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
fill(239,186,168);
ellipse(252,318,129,75);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140);
ellipse(310,155,45,30);
ellipse(185,155,45,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
ellipse(185,165,55,30);
fill(239,186,168);
ellipse(185,183,58,32);
ellipse(310,183,58,32);
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,27);
fill(239,186,168);
ellipse(251,236,30,25);
ellipse(238,230,35,20);
ellipse(266,230,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
ellipse(251,230,30,29);
fill(239,186,168);
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(0.65,0.65);
translate(27,-10);
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
function eveningImage() {
r = (102);
g = (153);
b = (204);
m = minute();
s = second();
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
fill(239,186,168);
ellipse(252,318,129,75);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140);
ellipse(310,155,45,30);
ellipse(185,155,45,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
ellipse(185,165,55,30);
fill(239,186,168,100);
ellipse(185,185,60,40);
ellipse(310,185,60,40);
fill(239,186,168);
ellipse(185,183,58,32);
ellipse(310,183,58,32);
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,27);
fill(239,186,168);
ellipse(251,236,30,25);
ellipse(238,230,35,20);
ellipse(266,230,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
ellipse(251,230,30,29);
fill(239,186,168);
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
function duskImage() {
r = (21);
g = (44);
b = (69);
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
fill(226,164,140,90); ellipse(250, 170, 285, 300);
rect(104,180,292,154);
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
fill(239,186,168);
ellipse(252,318,129,75);
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
ellipse(384, 210, 50, 80);
ellipse(116, 210, 50, 80);
fill(226,164,140);
ellipse(310,155,45,30);
ellipse(185,155,45,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
ellipse(185,165,55,30);
fill(239,186,168,100);
ellipse(185,190,60,45);
ellipse(310,190,60,45);
fill(239,186,168);
stroke(207,159,143);
ellipse(185,183,58,32);
noStroke();
ellipse(185,180,58,30);
stroke(207,159,143);
ellipse(310,183,58,32);
noStroke();
ellipse(310,180,58,32);
fill(255)
ellipse(185,185,60,0);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,0);
fill(255);
ellipse(310,185,60,0);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,0);
fill(239,186,168);
ellipse(251,236,30,25);
ellipse(238,230,35,20);
ellipse(266,230,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
ellipse(251,230,30,29);
fill(239,186,168);
ellipse(250,330,34,23);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
}