sketch
//Natalie Schmidt
//nkschmid@andrew.cmu.edu
//Sectiond D
//Project-05
var x = 0;
var y = 0;
function setup() {
createCanvas(400, 480);
background(102, 159, 166);
}
function draw() {
for (var y = -460; y < height - 35; y += 140) {
for (var x = -50; x < width - 50; x += 130) {
leaf(x, y);
push();
fill(191, 158, 57);
triangle(x + 5, y + 55, x + 18, y + 40, x + 30, y + 55);
triangle(x + 5, y + 55, x + 18, y + 70, x + 30, y + 55);
noFill();
stroke(191, 158, 57);
strokeWeight(2);
triangle(x, y + 55, x + 18, y + 35, x + 35, y + 55);
triangle(x, y + 55, x + 18, y + 75, x + 35, y + 55);
pop();
}
}
noLoop();
}
function leaf(x, y) {
stroke(47, 87, 53);
strokeWeight(1);
fill(65, 121, 73);
// top leaf 1 - right
beginShape();
curveVertex(x + 25, y + 400);
curveVertex(x + 25, y + 400);
curveVertex(x + 35, y + 403);
curveVertex(x + 45, y + 408);
curveVertex(x + 50, y + 420);
curveVertex(x + 45, y + 430);
curveVertex(x + 45, y + 430);
endShape();
//top leaf 1 - left
beginShape();
curveVertex(x + 25, y + 400);
curveVertex(x + 25, y + 400);
curveVertex(x + 23, y + 406);
curveVertex(x + 23, y + 409);
curveVertex(x + 26, y + 420);
curveVertex(x + 30, y + 425);
curveVertex(x + 35, y + 428);
curveVertex(x + 45, y + 430);
curveVertex(x + 45, y + 430);
endShape();
// top leaf 2 - right
beginShape();
curveVertex(x + 45, y + 388 - 8);
curveVertex(x + 45, y + 388 - 8);
curveVertex(x + 55, y + 391 - 8);
curveVertex(x + 65, y + 396 - 8);
curveVertex(x + 70, y + 408 - 8);
curveVertex(x + 65, y + 418 - 8);
curveVertex(x + 65, y + 418 - 8);
endShape();
//top leaf 2 - left
beginShape();
curveVertex(x + 45, y + 388 - 8);
curveVertex(x + 45, y + 388 - 8);
curveVertex(x + 43, y + 394 - 8);
curveVertex(x + 43, y + 397 - 8);
curveVertex(x + 46, y + 408 - 8);
curveVertex(x + 49, y + 413 - 8);
curveVertex(x + 55, y + 416 - 8);
curveVertex(x + 65, y + 418 - 8);
curveVertex(x + 65, y + 418 - 8);
endShape();
// top leaf 3 - right
beginShape();
curveVertex(x + 51 + 9, y + 388 - 29);
curveVertex(x + 51 + 9, y + 388 - 29);
curveVertex(x + 61 + 9, y + 391 - 29);
curveVertex(x + 71 + 9, y + 396 - 29);
curveVertex(x + 76 + 9, y + 408 - 29);
curveVertex(x + 71 + 9, y + 418 - 29);
curveVertex(x + 71 + 9, y + 418 - 29);
endShape();
//top leaf 3 - left
beginShape();
curveVertex(x + 51 + 9, y + 388 - 29);
curveVertex(x + 51 + 9, y + 388 - 29);
curveVertex(x + 49 + 9, y + 394 - 29);
curveVertex(x + 49 + 9, y + 397 - 29);
curveVertex(x + 52 + 9, y + 408 - 29);
curveVertex(x + 55 + 9, y + 413 - 29);
curveVertex(x + 61 + 9, y + 416 - 29);
curveVertex(x + 71 + 9, y + 418 - 29);
curveVertex(x + 71 + 9, y + 418 - 29);
endShape();
// top leaf 4 - right
beginShape();
curveVertex(x + 51 + 24, y + 388 - 52);
curveVertex(x + 51 + 24, y + 388 - 52);
curveVertex(x + 61 + 24, y + 391 - 52);
curveVertex(x + 71 + 24, y + 396 - 52);
curveVertex(x + 76 + 24, y + 408 - 52);
curveVertex(x + 71 + 24, y + 418 - 52);
curveVertex(x + 71 + 24, y + 418 - 52);
endShape();
//top leaf 4 - left
beginShape();
curveVertex(x + 51 + 24, y + 388 - 52);
curveVertex(x + 51 + 24, y + 388 - 52);
curveVertex(x + 49 + 24, y + 394 - 52);
curveVertex(x + 49 + 24, y + 397 - 52);
curveVertex(x + 52 + 24, y + 408 - 52);
curveVertex(x + 55 + 24, y + 413 - 52);
curveVertex(x + 61 + 24, y + 416 - 52);
curveVertex(x + 71 + 24, y + 418 - 52);
curveVertex(x + 71 + 24, y + 418 - 52);
endShape();
// bottom leaf 1 - right
beginShape();
curveVertex(x + 51 + 49, y + 388 - 25);
curveVertex(x + 51 + 49, y + 388 - 25);
curveVertex(x + 61 + 49, y + 391 - 25);
curveVertex(x + 71 + 49, y + 396 - 25);
curveVertex(x + 76 + 49, y + 408 - 25);
curveVertex(x + 71 + 49, y + 418 - 25);
curveVertex(x + 71 + 49, y + 418 - 25);
endShape();
//bottom leaf 1 - left
beginShape();
curveVertex(x + 51 + 49, y + 388 - 25);
curveVertex(x + 51 + 49, y + 388 - 25);
curveVertex(x + 49 + 49, y + 394 - 25);
curveVertex(x + 49 + 49, y + 397 - 25);
curveVertex(x + 52 + 49, y + 408 - 25);
curveVertex(x + 55 + 49, y + 413 - 25);
curveVertex(x + 62 + 49, y + 416 - 25);
curveVertex(x + 71 + 49, y + 418 - 25);
curveVertex(x + 71 + 49, y + 418 - 25);
endShape();
// bottom leaf 2 - right
beginShape();
curveVertex(x + 51 + 33, y + 388 - 1);
curveVertex(x + 51 + 33, y + 388 - 1);
curveVertex(x + 61 + 33, y + 391 - 1);
curveVertex(x + 71 + 33, y + 396 - 1);
curveVertex(x + 76 + 33, y + 408 - 1);
curveVertex(x + 71 + 33, y + 418 - 1);
curveVertex(x + 71 + 33, y + 418 - 1);
endShape();
//bottom leaf 2 - left
beginShape();
curveVertex(x + 51 + 33, y + 388 - 1);
curveVertex(x + 51 + 33, y + 388 - 1);
curveVertex(x + 49 + 33, y + 394 - 1);
curveVertex(x + 49 + 33, y + 397 - 1);
curveVertex(x + 52 + 33, y + 408 - 1);
curveVertex(x + 55 + 33, y + 413 - 1);
curveVertex(x + 62 + 33, y + 416 - 1);
curveVertex(x + 71 + 33, y + 418 - 1);
curveVertex(x + 71 + 33, y + 418 - 1);
endShape();
// bottom leaf 3 - right
beginShape();
curveVertex(x + 51 + 15, y + 388 + 18);
curveVertex(x + 51 + 15, y + 388 + 18);
curveVertex(x + 61 + 15, y + 391 + 18);
curveVertex(x + 71 + 15, y + 396 + 18);
curveVertex(x + 76 + 15, y + 408 + 18);
curveVertex(x + 71 + 15, y + 418 + 18);
curveVertex(x + 71 + 15, y + 418 + 18);
endShape();
//bottom leaf 3 - left
beginShape();
curveVertex(x + 51 + 15, y + 388 + 18);
curveVertex(x + 51 + 15, y + 388 + 18);
curveVertex(x + 49 + 15, y + 394 + 18);
curveVertex(x + 49 + 15, y + 397 + 18);
curveVertex(x + 52 + 15, y + 408 + 18);
curveVertex(x + 55 + 15, y + 413 + 18);
curveVertex(x + 62 + 15, y + 416 + 18);
curveVertex(x + 71 + 15, y + 418 + 18);
curveVertex(x + 71 + 15, y + 418 + 18);
endShape();
// bottom leaf 4 - right
beginShape();
curveVertex(x + 51 - 2, y + 388 + 35);
curveVertex(x + 51 - 2, y + 388 + 35);
curveVertex(x + 61 - 2, y + 391 + 35);
curveVertex(x + 71 - 2, y + 396 + 35);
curveVertex(x + 76 - 2, y + 408 + 35);
curveVertex(x + 71 - 2, y + 418 + 35);
curveVertex(x + 71 - 2, y + 418 + 35);
endShape();
//bottom leaf 3 - left
beginShape();
curveVertex(x + 51 - 2, y + 388 + 35);
curveVertex(x + 51 - 2, y + 388 + 35);
curveVertex(x + 49 - 2, y + 394 + 35);
curveVertex(x + 49 - 2, y + 397 + 35);
curveVertex(x + 52 - 2, y + 408 + 35);
curveVertex(x + 55 - 2, y + 413 + 35);
curveVertex(x + 62 - 2, y + 416 + 35);
curveVertex(x + 71 - 2, y + 418 + 35);
curveVertex(x + 71 - 2, y + 418 + 35);
endShape();
fill(73, 135, 82);
stroke(191, 158, 57);
strokeWeight(1);
//center line for leaf
push();
noFill();
stroke(47, 87, 53);
strokeWeight(3);
beginShape();
curveVertex(x + 30, y + 310);
curveVertex(x + 30, y + 435);
curveVertex(x + 100, y + 360);
curveVertex(x + 50, y + 360)
endShape();
pop();
}
I really wanted to do something with plant leaves and gold, and started at first to sketch out what I wanted. That ended up changing quite a lot, as I couldn’t for the life of me figure out how to write a loop that would loop the leaf shape rather than having to figure out the coordinates for each one individually. I asked multiple people for help, including a TA and some students in other CS courses, but none of us could really make it work. I wish I could’ve figured that out and implemented it for this project, because it would’ve saved a lot of time. Otherwise, figuring out how to loop the whole plant was relatively easy, since we had learned a version of how to do so in class.
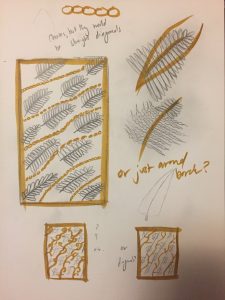
The original ideas for my wallpaper. I wanted to do some sort of fern, and did a rough sketch of what that might look like.
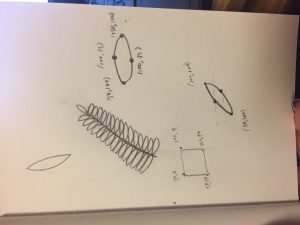
Trying to figure out coordinates for the leaves. Figuring out the first leaf took most of my time, as I was pretty new to using curveVertex().
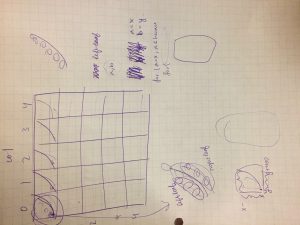
A friend (from another CS course) tried to help me figure out how to write a loop for the leaves by drawing it out on paper. In the end, we couldn’t figure it out.