//var smoke = {x:120, y:365, w:35, h:20, d:0.5, a:150}
var dishes=[];
var smokes=[];
var eyelx=220;
var eyerx=260;
var sushiLinks = [
"https://i.imgur.com/dMoEprH.png",
"https://i.imgur.com/69T53Rk.png",
"https://i.imgur.com/LQ3xxUA.png",
"https://i.imgur.com/x19Rvvq.png",
"https://i.imgur.com/d7psp9U.png"]
var sushi = [];
function preload(){
for (var i = 0; i < sushiLinks.length; i++) {
sushi[i]=loadImage(sushiLinks[i]);
}
}
function setup() {
createCanvas(480,480);
for (var i = 0; i < 4; i++) {
dishes[i]=makeDish(-90+i*130);
}
for(var j = 0; j<3; j++){
smokes[j]= makeSmoke(120);
}
}
function draw() {
background(165,199,199);
drawCurtain();
drawChef();
drawEye();
drawBody();
drawBelt()
drawTable();
drawPlate();
drawDishPile();
drawCup();
//smoke
updateAndDisplaySmokes();
removeSmoke();
addNewSmoke();
//dishes on the belt
updateAndDisplayDishes();
removeDish();
addNewDish();
}
function drawCurtain(){
noStroke();
fill(82,106,121)
rect(0,0,width/2-5,90);
rect(width/2+5,0,width/2-5,90)
stroke(106,137,156);
strokeWeight(5);
rect(-15,-15,width/2-5,90);
rect(width/2+20,-15,width/2-5,90)
}
function drawChef(){
push();
noStroke();
rectMode(CENTER);
//hat
fill(129,153,160)
rect(width/2,120,100,30)
//face and neck
fill(235,216,190);
rect(width/2,170,100,70);
rect(width/2,210,50,20);
}
function drawEye(){
push();
fill(37)
ellipseMode(CENTER);
ellipse(eyelx,165,12,7)
ellipse(eyerx,165,12,7)
eyelx += 0.2;
eyerx += 0.2;
if(eyelx>=width/2-5){
eyelx = 205;
eyerx = 245;
}
pop();
}
function drawBody(){
//body
fill(152,178,186);
rect(width/2,300,130,160);
//collar
fill(129,151,158);
triangle(width/2-45,220,width/2+45,220,width/2,280);
fill(212,232,238);
triangle(width/2-30,220,width/2+30,220,width/2,260);
//arms
fill(129,153,160);
quad(width/2-65,220,width/2-90,250,width/2-90,310,width/2-65,310);
quad(width/2+65,220,width/2+90,250,width/2+90,310,width/2+65,310);
fill(235,216,190);
rect(width/2-77,345,24,70)
rect(width/2+77,345,24,70)
pop();
}
function drawBelt(){
noStroke();
fill(152,151,151)
rect(0,350,width,height/3)
fill(133);
rect(0,360,width,5);
fill(183);
rect(0,330,width,30)
}
function drawTable(){
fill(101,92,85);
rect(0,440,width,40);
}
function drawPlate(){
push();
rectMode(CENTER);
fill(222,207,175);
rect(width/2,420,130,15);
rect(width/2-30,428,20,23);
rect(width/2+30,428,20,23);
pop();
}
function drawDishPile(){
fill(240);
rect(width/2+110,389,90,7)
rect(width/2+110,406,90,7)
rect(width/2+110,423,90,7)
fill(218);
rect(width/2+125,396,60,10)
rect(width/2+125,413,60,10)
rect(width/2+125,430,60,10)
}
function drawCup(){
push();
fill(105,108,91);
rect(width/2-155,380,45,60,5)
pop();
}
function drawSmoke(){
fill(255,this.a)
ellipse(this.x,this.y,this.w,this.h);
}
function moveSmoke(){
this.x += this.d;
this.y -= this.d;
}
function scaleSmoke(){
this.w -= 0.2;
this.h -= 0.2;
}
function alphaSmoke(){
this.a -= 2;
}
function makeSmoke(birthLocationX) {
var smoke = {x:birthLocationX,
y:365,
w:35,
h:20,
d:0.3,
a:150,
move:moveSmoke,
scale:scaleSmoke,
alpha:alphaSmoke,
drawS:drawSmoke}
return smoke;
}
function updateAndDisplaySmokes(){
// Update the smoke position, scale, alpha
for (var i = 0; i < smokes.length; i++){
smokes[i].move();
smokes[i].scale();
smokes[i].alpha();
smokes[i].drawS();
}
}
function removeSmoke(){
var smokesToKeep = [];
for (var i = 0; i < smokes.length; i++){
if (smokes[i].a > 0) {
smokesToKeep.push(smokes[i]);
}
}
smokes = smokesToKeep;
}
function addNewSmoke(){
if(frameCount%45==0){
smokes.push(makeSmoke(120))
};
}
function makeDish(dishX) {
var dish = {x:dishX,
speed:1,
move:moveDish,
display:displayDish,
type:1}
return dish;
}
function updateAndDisplayDishes(){
// Update the dishes's positions, and display them.
for (var i = 0; i < dishes.length; i++){
dishes[i].move();
dishes[i].display(dishes[i].x, dishes[i].type);
}
}
function removeDish(){
var dishesToKeep = [];
for (var i = 0; i < dishes.length; i++){
if (dishes[i].x < 480) {
dishesToKeep.push(dishes[i]);
}
}
dishes = dishesToKeep;
}
function addNewDish(){
if(frameCount%120==0){
var newDish = makeDish(-90);
newDish.type = random([0,1,2,3,4]);
dishes.push(newDish)
}
}
//create dish and sushi
function displayDish(x,type){
push();
translate(this.x,314);
fill(240);
rect(0,0,90,7)
fill(218);
rect(15,7,60,10);
pop();
drawSushi(x + 45, type);
}
function moveDish(){
this.x += this.speed;
}
function drawSushi(x, type){
imageMode(CENTER)
image(sushi[type], x, 302);
}
This project, I thought about making a sushi conveyable belt. I thought about making different dishes of sushi moving on the screen and the different sushi need to appear randomly. Therefore, at first, I drew out the scene of a sushi restaurant, where we could see a plate, a cup of tea and some empty dishes on the table. Above the table, there was the conveyable belt with sushi. Behind the belt, there was a sushi chef who kept looking at the sushi. The final result is fun! I liked how I could translate my sketch into a moving webpage 🙂
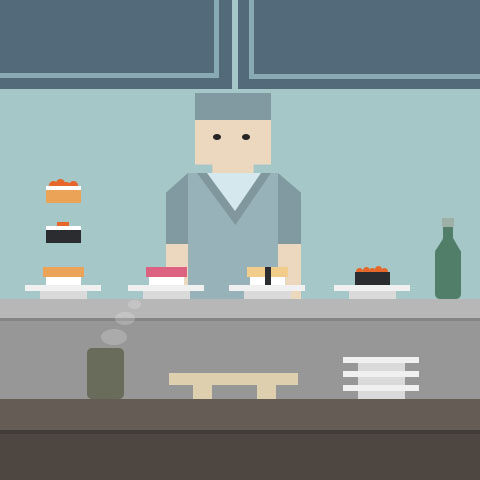