var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
var birds = [];
var redu;
function preload() { //load birds
birdie = loadImage("https://i.imgur.com/ql6l0Rq.png")
}
function setup() {
createCanvas(480 , 270);
frameRate(24);
for (var i = 0; i < 5; i++){ //set up loop for birds
var location = random(width);
birds[i] = makeBirds(location);
}
}
function draw() {
background(redu, 50, 200);
//call drawings
sun();
drawMountains();
drawRivers();
drawBackground();
updateBirds();
displayBirds();
addBirds();
makeBirds();
moveBirds();
redu = mouseY/5; //let background change depending on y
}
// SUN-----
function sun(){
fill("red");
ellipse(240, 135, 100, 100);
}
// BIRDS -----
function updateBirds(){
for (var i = 0; i < birds.length; i++){
birds[i].move();
birds[i].display();
}
}
function addBirds() {
var a = random(1);
if (a < 0.03) {
birds.push(makeBirds(width));
}
}
function moveBirds() { //change x of birds
this.x += this.speed;
}
function displayBirds() { //put birds on canvas
var birdY = 10;
push();
translate(this.x, this.height);
for(var i=0; i<this.number; i++) {
image(birdie, 10, birdY, random(10, 50), random(10, 50));
}
birdY += 1;
pop();
}
function makeBirds(birthx) { //set up variables for birds
var birds2 = {x: birthx,
number: floor(random(1,2)),
speed: -3,
height: random(90,100),
move: moveBirds,
display: displayBirds}
return birds2;
}
function drawBackground(){ //part of background color
fill(238,238,238,100);
beginShape();
rect(0, 0, width - 1, height - 1);
endShape();
}
//RIVER
function drawRivers(){
//River 1
fill(144,175,197,255);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * 0.003) + (millis() * terrainSpeed * 2);
var y = map(noise(t), 0,1, 0, height * 0.1);
vertex(x, y+225);
}
vertex(width,height);
vertex(0,height);
endShape();
//River 2
fill(51,107,135,255);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * 0.003) + (millis() * terrainSpeed * 2);
var y = map(noise(t), 0,1, 0, height * 0.1);
vertex(x, y+230);
}
vertex(width,height);
vertex(0,height);
endShape();
//River 3
fill(144,175,197,255);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * 0.003) + (millis() * terrainSpeed * 2);
var y = map(noise(t), 0,1, 0, height * 0.1);
vertex(x, y+235);
if(noise(t) > 0.2){
fill(144, 175, 197, 80);
}
else {
fill(144,175,197,120);
}
}
vertex(width,height);
vertex(0,height);
endShape();
//River 4
fill(51,107,135,255);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * 0.003) + (millis() * terrainSpeed * 2);
var y = map(noise(t), 0,1, 0, height * 0.1);
vertex(x, y+240);
}
vertex(width,height);
vertex(0,height);
endShape();
}
function drawMountains() {
noStroke();
fill(118,54,38,255);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed*0.5);
var y = map(noise(t), 0,1, 0, height*1.1);
vertex(x, y-15);
}
vertex(width,height);
vertex(0,height);
endShape();
}
Using the moving landscapes, I wanted to display a landscape of the ocean and mountain moving. For an interesting part of the project, I added a random variation in the birds to make it seem as if the birds were flocking in the sky.
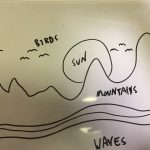