/*
Alice Fang
Section E
acfang@andrew.cmu.edu
Project-10-Generative Lanscape
*/
var boats = [];
var clouds = [];
var terrainDetail = 0.001; // small waves
var terrainSpeed = 0.0005;
function setup() {
createCanvas(400, 200);
noStroke();
for (var i = 0; i < 5; i++) {
// populate arrays
var boatX = random(width);
var boatY = random(height / 2, height - 20);
boats[i] = makeBoat(boatX, boatY);
var cloudX = random(width);
var cloudY = random(0, height / 3);
clouds[i] = makeCloud(cloudX, cloudY);
}
}
function draw() {
background(210, 235, 250);
// sun
fill('Gold');
ellipse(350, 20, 20, 20);
// ocean
fill(180, 215, 230);
rect(0, height / 2, width, height);
// top current
stroke(170, 205, 220);
beginShape();
for (var w = 0; w < width; w++) {
var t = (w * terrainDetail) + (millis() * terrainSpeed);
var Y = map(noise(t), 0, 1, height / 2, height);
vertex(w, Y);
}
endShape();
// bottom current
stroke(170, 205, 220);
beginShape();
for (var w = 0; w < width; w++) {
var t = (w * terrainDetail) + (millis() * terrainSpeed);
var Y = map(noise(t), 0, 1, height / 2 + 20, height);
vertex(w, Y);
vertex(w, Y+10);
}
endShape();
// draw boats
for (var j = 0; j < boats.length; j++) {
boats[j].draw();
boats[j].move();
}
// draw clouds
for (var k = 0; k < clouds.length; k++) {
clouds[k].draw();
clouds[k].move();
}
}
// boats
function drawBoat() {
// mast
var mastX = this.xPos + (this.rWidth * 2 / 3);
stroke(10, 30, 20);
strokeWeight(3);
line(mastX, this.yPos - 3 * this.rHeight, mastX, this.yPos);
// boat
noStroke();
fill('LightSalmon');
quad(this.xPos, this.yPos, this.xPos + this.rWidth, this.yPos,
this.xPos + this.rWidth - this.xOff, this.yPos + this.rHeight, this.xPos + this.xOff, this.yPos + this.rHeight);
// sail
noStroke();
fill(255, 245, 238);
triangle(mastX, this.yPos - 5, mastX - this.rSail, this.yPos - 5, mastX, this.yPos - 4 * this.rHeight);
triangle(mastX, this.yPos - 5, mastX + this.rSail / 2, this.yPos - 5, mastX, this.yPos - 4 * this.rHeight);
}
function moveBoat() {
this.xPos += this.speed;
//if boat reaches right side, restart at left; randomize speed, width, height, offset
if (this.xPos > width) {
this.xPos = 0;
this.xOff = random(5, 15);
this.rWidth = random(40, 60);
this.rHeight = random(8, 12);
this.speed = random(0.1, 0.5);
}
}
function makeBoat(x, y) {
var boat = { xPos: x,
yPos: y,
xOff: random(5, 10), // offset of trapezoid
rSail: random (10, 20),
rWidth: random(30, 50),
rHeight: random(8, 12),
speed: random(0.1, 0.5),
draw: drawBoat,
move: moveBoat}
return boat;
}
// clouds
function drawCloud(x, y) {
fill(240, 245, 250, 120);
ellipse(this.xPos, this.yPos, this.rWidth, this.rWidth);
ellipse(this.xPos + this.rWidth/2, this.yPos, this.rWidth * 2, this.rWidth * 2);
ellipse(this.xPos + this.rWidth, this.yPos, this.rWidth, this.rWidth);
}
function moveCloud() {
this.xPos += this.speed;
//if cloud reaches right side, restart at left; randomize speed
if (this.xPos > width) {
this.xPos = 0;
this.speed = random(0.1, 0.4);
}
}
function makeCloud(x, y) {
var cloud = { xPos: x,
yPos: y,
rWidth: random(10, 20),
speed: random(0.05, 0.3),
draw: drawCloud,
move: moveCloud}
return cloud;
}
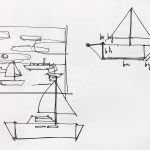
I wanted to create a calm, cute landscape that wasn’t exactly too urban, so when I asked my roommate and she offered the suggestion of beaches, I thought of an ocean populated by little boats. I struggled with implementing objects, because this was the first time I had to code it personally instead of just editing certain elements, but doing this really helped me understand objects better.