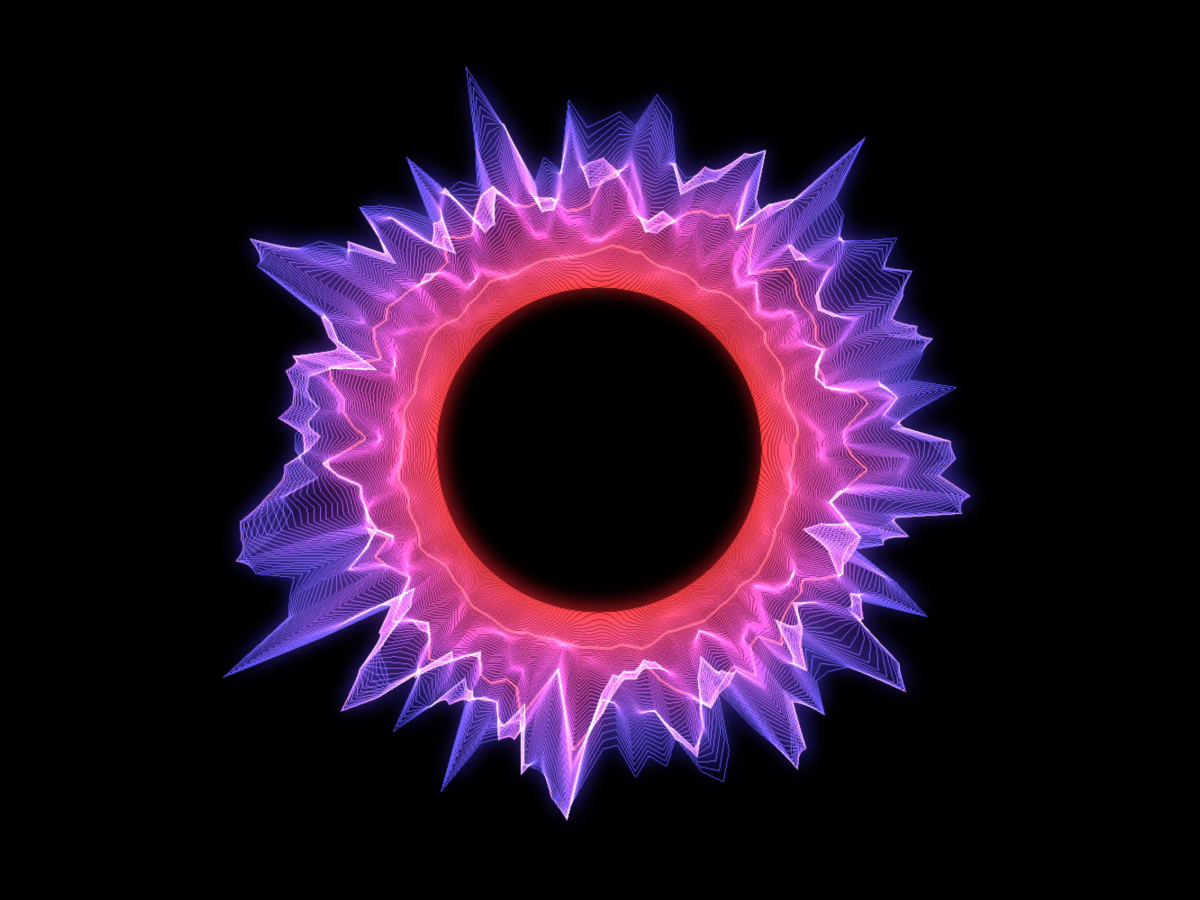
For this week’s Looking Outwards post, I’ll be discussing Gretchen Kupferschmid’s Looking Outwards 07 about the project “Halo.” Like Gretchen, I also appreciate that the project adds an artistic element to data visualization since data, especially in the medical field, is often displayed traditionally through graphs or just listed out as numbers. Being aware of your health data is very important so that you can make informed decisions that will affect your body positively, and I see this visualization as a way of 1. summarizing dense quantitative content into an impression (that you can take in within a glimpse) and 2. engaging users so that they will want to learn more about their own health. While a creative visualization like this by itself may not provide all the necessary details for a holistic report, integrating the two creates an experience where checking your own health can become a visual delight. I also agree with Gretchen that showing data in this visual way allows users to more intuitively and efficiently compare large sets of data without having to process all the numbers in their head.