/* Cathy Dong
Section D
yinhuid
Project 5-wallpaper
*/
var ox = 50;
var oy = 75;
var xSpace = 80;
var ySpace = 120;
function setup() {
createCanvas(420, 600);
background(244, 239, 223);
}
function draw() {
for (var i = 0; i < 5; i++) {
for (var j = 0; j < 5; j++) {
//avocado outer variable
var outX1 = ox + i * xSpace - 33;
var outY1 = oy + j * ySpace;
var outX4 = ox + i * xSpace - 25;
var outY4 = oy + j * ySpace - 30;
var outX2 = ox + i * xSpace - 8;
var outY2 = oy + j * ySpace - 60;
var outX3 = ox + i * xSpace;
var outY3 = oy + j * ySpace + 35;
//avocado
stroke(29, 59, 16);
strokeWeight(2);
fill(203, 223, 180);
beginShape();
curveVertex(outX4, outY4);
curveVertex(outX2, outY2);
curveVertex(outX2 + 7, outY2 - 2); //center point
curveVertex(outX2 + 16, outY2);
curveVertex(outX4 + 50, outY4);
curveVertex(outX1 + 66, outY1);
curveVertex(outX3 + 20, outY3 - 10);
curveVertex(outX3, outY3);
curveVertex(outX3 - 20, outY3 - 10);
curveVertex(outX1, outY1);
endShape(CLOSE);
//avocado inner
noStroke();
fill(235, 234, 188);
beginShape();
curveVertex(outX4 + 10, outY4 - 5);
curveVertex(outX2 + 10, outY2 + 10);
curveVertex(outX2 + 17, outY2 + 8); //top center point
curveVertex(outX2 + 20, outY2 + 10);
curveVertex(outX4 + 40, outY4 - 10);
curveVertex(outX1 + 60, outY1 - 10);
curveVertex(outX3 + 15, outY3 - 15);
curveVertex(outX3, outY3 - 5); //bottom center point
curveVertex(outX3 - 20, outY3 - 15);
curveVertex(outX1 + 10, outY1 - 10);
endShape(CLOSE);
// avocado shell
if ((i % 2 == 0 & j % 2 == 1) || (i % 2 == 1 && j % 2 == 0)){
drawShell(i,j);
}
// avocade without shell
if ((i % 2 == 1 & j % 2 == 1) || (i % 2 == 0 && j % 2 == 0)){
drawNoShell(i,j);
}
}
}
noLoop();
}
function drawShell(i,j) {
//avocado center
stroke(131, 98, 66);
strokeWeight(2);
fill(198, 140, 102);
ellipse(ox + i * xSpace, oy + j * ySpace, 34, 36);
//avocado center mid
noStroke();
fill(215, 158, 124);
ellipse(ox + i * xSpace, oy + j * ySpace, 20, 23);
//avocado center light
stroke(240, 217, 174);
strokeWeight(5);
noFill();
arc(ox + i * xSpace, oy + j * ySpace, 20, 20, 0, QUARTER_PI);
}
function drawNoShell(i,j) {
//avocado center
stroke(248, 241, 224);
strokeWeight(1);
fill(227, 217, 114);
ellipse(ox + i * xSpace, oy + j * ySpace, 35, 38);
//avocado center light
stroke(202, 198, 75);
strokeWeight(10);
noFill();
arc(ox + i * xSpace, oy + j * ySpace, 20, 20, 0, HALF_PI);
}
My project is about avocado patterns. To help coding, I first calculated important point x and y axis, using shell center as the origin.
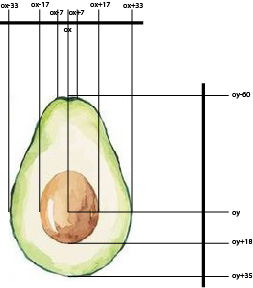