var x;
var y;
function setup() {
createCanvas(480,480);
}
function draw (){
background(247, 173, 209);
drawGrid();
}
function drawGrid(){
//create tile through looping x and y values no more than height and width of canvas
for (y= -6; y<height; y+=94){
for(x=-6; x<width ; x+=94){
//translate the grid to the new x/y position each loop
push();
translate(x,y);
drawShadowPink();
drawWhites();
drawShadowEgg();
drawYolk();
pop();
}
}
}
//create egg white shape
function drawWhites(){
fill(255);
strokeWeight(3);
beginShape();
curveVertex(41.77, 38.02);
curveVertex(82.95, 38.2);
curveVertex(96.47, 39.8);
curveVertex(101.98, 41.56);
curveVertex(103.88, 46.61);
curveVertex(97.46, 51.37);
curveVertex(96.27, 59.94);
curveVertex(92.47, 67.03);
curveVertex(88.92, 66.25);
curveVertex(72.82, 68.51);
curveVertex(55.28, 73.71);
curveVertex(47, 71.42);
curveVertex(42.5, 67.12);
curveVertex(40.78, 63.32);
curveVertex(21.58, 53.57);
curveVertex(20.22, 51.85);
curveVertex(24.11, 47.02);
curveVertex(30.22, 44.07);
curveVertex(31.38, 41.25);
curveVertex(35.3, 38.36);
curveVertex(41.93, 38.2);
curveVertex(41.77, 38.02);
endShape();
}
//create yolk shape
function drawYolk(){
fill(250, 212, 25);
strokeWeight(1);
beginShape();
curveVertex(41.38, 41.62);
curveVertex(47.15, 28.01);
curveVertex(57.11, 23.11);
curveVertex(68.59, 23.89);
curveVertex(79.61, 31.47);
curveVertex(82.02, 35.39);
curveVertex(82.61, 40.38);
curveVertex(71.89, 47.39);
curveVertex(57.46, 48.21);
curveVertex(41.38, 41.62);
curveVertex(47.15, 28.01);
curveVertex(57.11, 23.11);
endShape();
}
//shadow underneath whites
function drawShadowPink(){
fill(224, 112, 168);
strokeWeight(0);
ellipse(66.77, 60.15, 82.86, 37.3);
}
//shadow underneath egg yolk
function drawShadowEgg(){
fill(219, 150, 0);
strokeWeight(0);
ellipse(65.05, 43.14, 47.3, 18.7);
}
I used the pattern of an egg, which is a little doodle/drawing I constantly draw. I even named my website eggontoast.store because of the eggs I draw
I used this simple egg drawing and added some shadows to give it dimension and a more interesting factor.
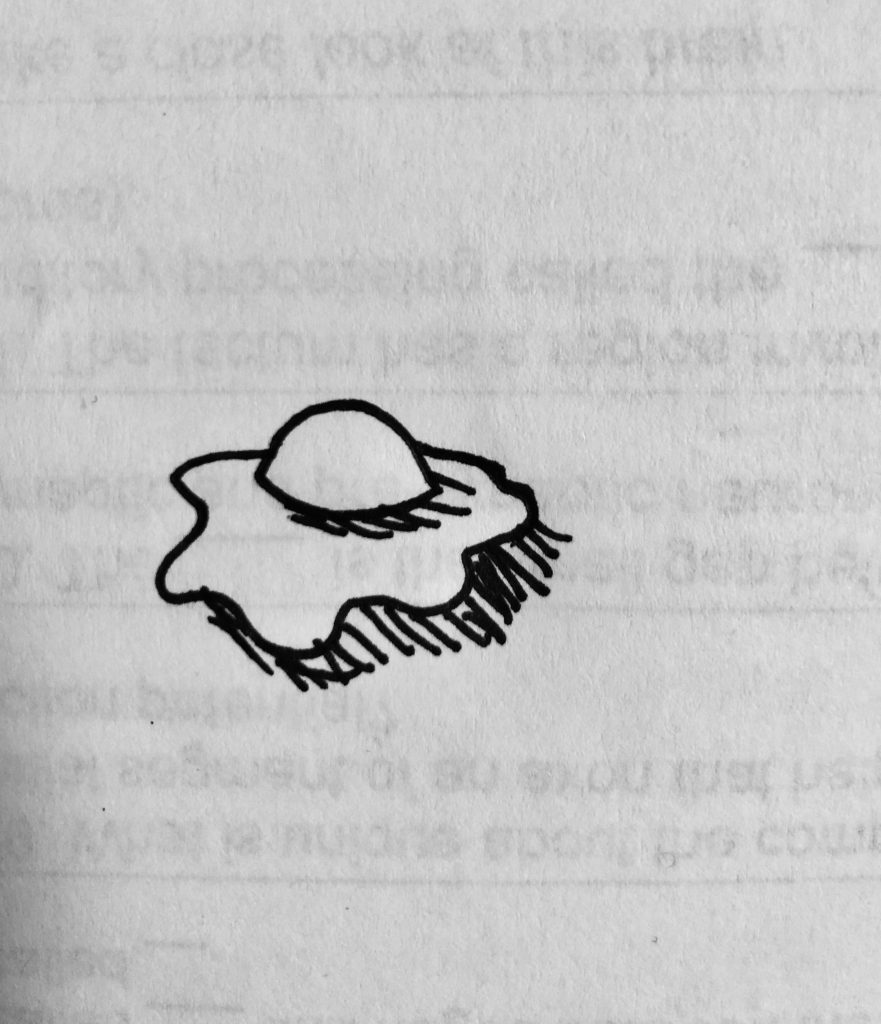