//Patrick Fisher, Section B, wpf@andrew.cmu.edu Assignment -07-project
var functionState = 1;
function setup() {
createCanvas(480, 480);
frameRate(10);
}
function draw() {
var nPoints = 80;
var radius = 150;
var separation = 120;
if(functionState == 1){ //glitchy circle
background(0);
fill(255, 255, 255, 64);
var mouseXincrease = map(mouseX,0,width,-40,40);
var mouseYincrease = map(mouseY,0,height,-40,40);
var colorXY = map(mouseX + mouseY,0,960,0,255);
push();
translate(2*separation, height / 2);
fill(255,0,colorXY);
beginShape();
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-40, mouseXincrease), py + random(-40, mouseYincrease));
}
endShape(CLOSE);
pop();
}
else if(functionState == 2) {
var mouseXpoints = map(mouseX,0,width/2,1,30);
var oppoY = map(mouseY,0,width,255,0);
background(240);
push();
fill(oppoY)
translate(2*separation, height / 2);
beginShape();
for (var i = 0; i < mouseXpoints; i++) {
var theta = map(i, 0, mouseXpoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px,py);
ellipse(px, py, 3,3);
}
endShape(CLOSE);
pop();
}
}
function mousePressed(){
if(functionState ==1){
functionState = 2;
} else if(functionState ==2){
functionState = 1;
}
}
I am very conflicted on the result of this project. I had a major lack of inspiration when it came to ideas, so I ended up taking some of the shapes shown in the sample and playing around a bit with them. I had difficulty with some of my map functions as they were not working as I had intended for some reason. However, I do like in the end what I came up with. The circle getting more and more glitchy is really fun and I really love how the vertices of the second circle spring out of the original one like a clown car.
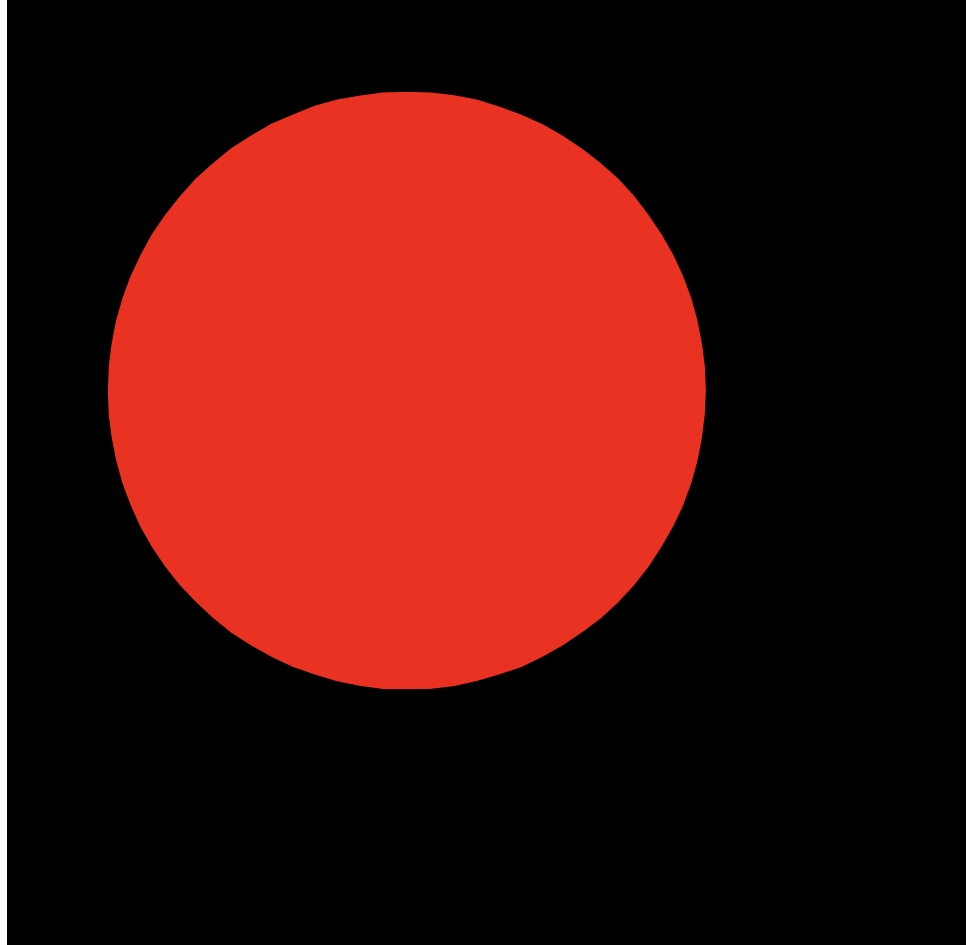
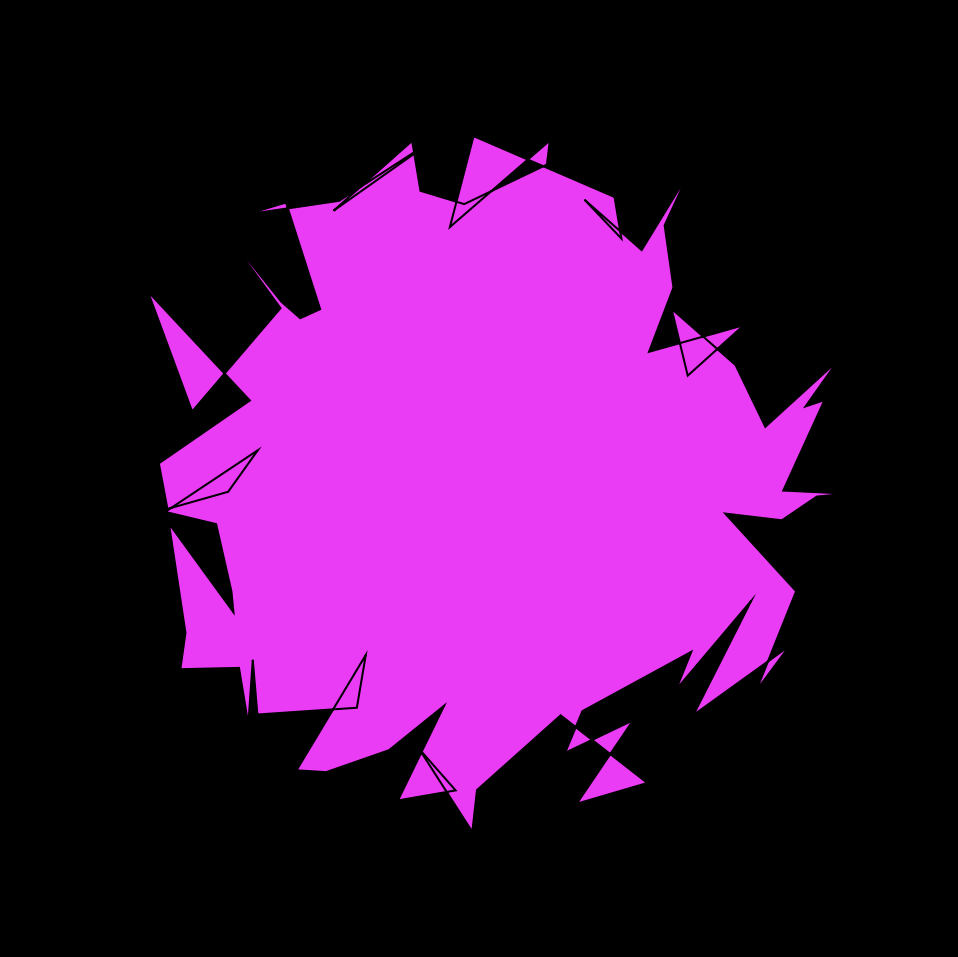
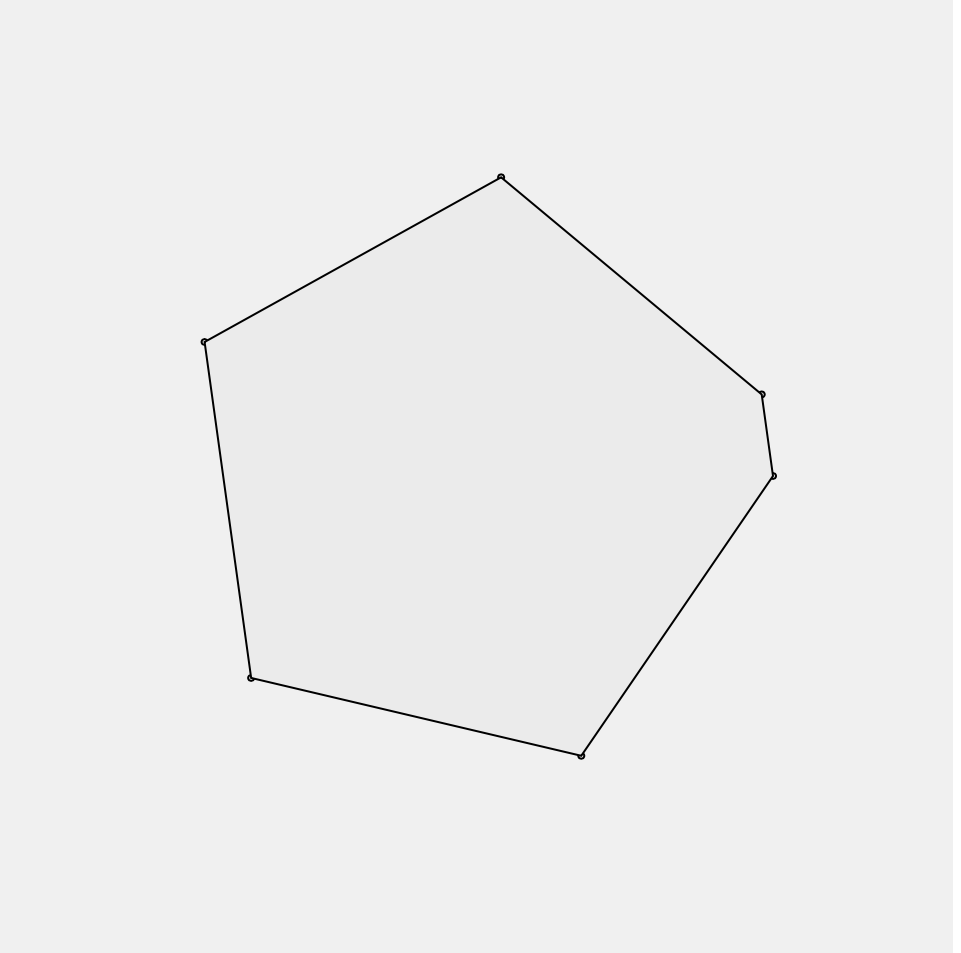
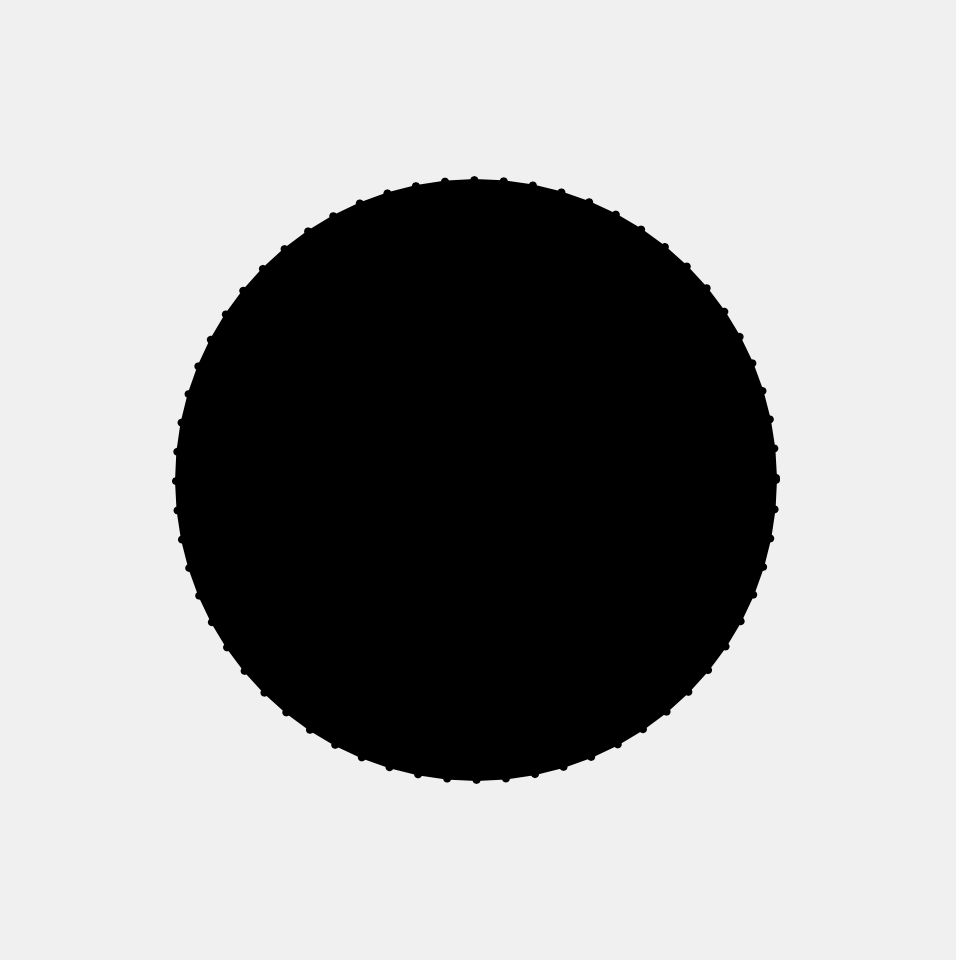