sketch
var cloudShiftX = 200;
var cloudShiftY = 100;
var cloudShift0X = -150;
var cloudShift0Y = 70;
var adjustShift = 100;
var greyWhiteR = 245;
var greyWhiteG = 241;
var greyWhiteB = 230;
var windowsR = 160;
var windowsG = 160;
var windowsB = 150;
var lightsR = 255;
var lightsG = 197;
var lightsB = 107;
var greySkyR = 226;
var greySkyG = 226;
var greySkyB = 214;
var skyR = 182;
var skyG = 222;
var skyB = 228;
var waterR = 162;
var waterG = 222;
var waterB = 208;
var greyWaterR = 160;
var greyWaterG = 160;
var greyWaterB = 150;
function setup() {
createCanvas(640, 480);
}
function draw() {
background(greySkyR, greySkyG, greySkyB);
noStroke();
if ((mouseX >= 640) & (mouseX <= 640)){
mouseX = 640;
}
if ((mouseX > 0) & (mouseX < 640)){
greySkyR = mouseX*((182-226)/640) + 226;
greySkyG = mouseX*((222-226)/640) + 226;
greySkyB = mouseX*((228-214)/640) + 214;
}
if ((mouseX >= 640) & (mouseX <= 640)){
mouseX = 640;
}
if ((mouseIsPressed) && (windowsR = 160)) {
windowsR = lightsR;
windowsG = lightsG;
windowsB = lightsB;
}
else if ((mouseIsPressed) & (windowsR = 225)) {
windowsR = 160;
windowsG = 160;
windowsB = 150;
}
fill(greyWhiteR, greyWhiteG, greyWhiteB);
ellipse(mouseX + 0, 125, 20);
ellipse(mouseX + 20, 120, 35);
ellipse(mouseX + 40, 120, 55);
ellipse(mouseX + 65, 125, 40);
ellipse(mouseX + 90, 120, 45);
ellipse(mouseX + 115, 125, 20);
ellipse(mouseX + 125, 120, 30);
ellipse(mouseX + 145, 125, 20);
ellipse(mouseX + 0 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
ellipse(mouseX + 20 + cloudShiftX + adjustShift, 100 + cloudShiftY, 35);
ellipse(mouseX + 40 + cloudShiftX + adjustShift, 100 + cloudShiftY, 55);
ellipse(mouseX + 65 + cloudShiftX + adjustShift, 105 + cloudShiftY, 40);
ellipse(mouseX + 90 + cloudShiftX + adjustShift, 100 + cloudShiftY, 45);
ellipse(mouseX + 115 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
ellipse(mouseX + 125 + cloudShiftX + adjustShift, 100 + cloudShiftY, 30);
ellipse(mouseX + 145 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
ellipse(mouseX + 0 + cloudShift0X, 105 + cloudShift0Y, 20);
ellipse(mouseX + 20 + cloudShift0X, 100 + cloudShift0Y, 35);
ellipse(mouseX + 40 + cloudShift0X, 100 + cloudShift0Y, 55);
ellipse(mouseX + 65 + cloudShift0X, 105 + cloudShift0Y, 40);
ellipse(mouseX + 90 + cloudShift0X, 100 + cloudShift0Y, 45);
ellipse(mouseX + 115 + cloudShift0X, 105 + cloudShift0Y, 20);
ellipse(mouseX + 125 + cloudShift0X, 100 + cloudShift0Y, 30);
ellipse(mouseX + 145 + cloudShift0X, 105 + cloudShift0Y, 20);
ellipse(mouseX + 0 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 20 + 3*cloudShift0X, 100 + .5*cloudShiftY, 35);
ellipse(mouseX + 40 + 3*cloudShift0X, 100 + .5*cloudShiftY, 55);
ellipse(mouseX + 65 + 3*cloudShift0X, 105 + .5*cloudShiftY, 40);
ellipse(mouseX + 90 + 3*cloudShift0X, 100 + .5*cloudShiftY, 45);
ellipse(mouseX + 115 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 125 + 3*cloudShift0X, 100 + .5*cloudShiftY, 30);
ellipse(mouseX + 145 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 0 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 20 + 5*cloudShift0X, 100 + .5*cloudShiftY, 35);
ellipse(mouseX + 40 + 5*cloudShift0X, 100 + .5*cloudShiftY, 55);
ellipse(mouseX + 65 + 5*cloudShift0X, 105 + .5*cloudShiftY, 40);
ellipse(mouseX + 90 + 5*cloudShift0X, 100 + .5*cloudShiftY, 45);
ellipse(mouseX + 115 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 125 + 5*cloudShift0X, 100 + .5*cloudShiftY, 30);
ellipse(mouseX + 145 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
fill(greyWhiteR, greyWhiteG, greyWhiteB);
rect(40, 322, 562, 10);
rect(104, 310, 433, 15);
triangle(537, 310, 537, 325, 572, 325);
rect(109, 299, 320, 15);
triangle(429, 299, 429, 310, 469, 310);
rect(115, 283, 75, 18);
triangle(190, 283, 190, 301, 223, 301);
rect(169, 234, 120, 80);
rect(290, 255, 100, 60);
rect(390, 276, 130, 40);
bezier(104, 220, 123, 223, 173, 227, 200, 234);
triangle(95, 219, 200, 234, 190, 283);
triangle(95, 219, 121, 237, 121, 258);
triangle(147, 190, 255, 228, 223, 299);
bezier(147, 190, 185, 201, 227, 217, 255, 228);
triangle(147, 190, 162, 229, 185, 232);
triangle(335, 255, 229, 146, 287, 299);
bezier(229, 146, 277, 178, 315, 217, 335, 255);
triangle(237, 222, 229, 146, 287, 299);
triangle(335, 255, 460, 240, 407, 299);
bezier(335, 255, 375, 243, 425, 239, 460, 240);
triangle(395, 253, 472, 276, 429, 299);
bezier(395, 253, 424, 258, 451, 267, 472, 276);
triangle(472, 276, 550, 269, 512, 310);
bezier(472, 276, 498, 270, 525, 268, 550, 269);
fill(windowsR, windowsG, windowsB);
triangle(121, 237, 190, 283, 121, 283);
bezier(190, 283, 192, 270, 208, 270, 223, 299);
bezier(223, 299, 246, 279, 269, 281, 287, 299);
bezier(287, 299, 307, 281, 335, 281, 347, 299);
bezier(347, 299, 362, 279, 384, 282, 407, 299);
triangle(408, 271, 408, 299, 429, 299);
bezier(429, 299, 447, 291, 461, 291, 469, 310);
bezier(469, 310, 480, 294, 496, 294, 512, 310);
triangle(512, 310, 537, 310, 537, 283);
fill(greyWaterR, greyWaterG, greyWaterB);
rect(0, 332, 640, 480);
if ((mouseX > 0) & (mouseX < 640)){
greyWaterR = mouseX*((142-160)/640) + 160;
greyWaterG = mouseX*((222-160)/640) + 160;
greyWaterB = mouseX*((208-150)/640) + 150;
}
var offsetX1 = 100
var offsetY1 = 15
fill(greyWhiteR, greyWhiteG, greyWhiteB);
rect(640-mouseX, 345, 220, 3);
rect(640-mouseX + 280, 345, 160, 3);
rect(640-mouseX + 500, 345, 240, 3);
rect(640-mouseX - 300, 345, 200, 3);
rect(640-mouseX - 600, 345, 220, 3);
rect(640-mouseX + offsetX1, 345 + offsetY1, 220, 3);
rect(640-mouseX + 280 + offsetX1, 345 + offsetY1, 160, 3);
rect(640-mouseX + 500 + offsetX1, 345 + offsetY1, 240, 3);
rect(640-mouseX - 300 + offsetX1, 345 + offsetY1, 200, 3);
rect(640-mouseX - 600 + offsetX1, 345 + offsetY1, 220, 3);
rect(640-mouseX + 2*offsetX1, 345 + 2.5*offsetY1, 220, 3);
rect(640-mouseX + 280 + 2*offsetX1, 345 + 2.5*offsetY1, 160, 3);
rect(640-mouseX + 500 + 2*offsetX1, 345 + 2.5*offsetY1, 240, 3);
rect(640-mouseX - 300 + 2*offsetX1, 345 + 2.5*offsetY1, 200, 3);
rect(640-mouseX - 600 + 2*offsetX1, 345 + 2.5*offsetY1, 220, 3);
rect(640-mouseX + 3*offsetX1, 345 + 5*offsetY1, 220, 3);
rect(640-mouseX + 280 + 3*offsetX1, 345 + 5*offsetY1, 160, 3);
rect(640-mouseX + 500 + 3*offsetX1, 345 + 5*offsetY1, 240, 3);
rect(640-mouseX - 300 + 3*offsetX1, 345 + 5*offsetY1, 200, 3);
rect(640-mouseX - 600 + 3*offsetX1, 345 + 5*offsetY1, 220, 3);
}
I was feeling very ambitious at the start of this project, but after two days of coding simple shapes without yet having added any interactivity, i realized i needed to simplify the design if i was to finish before the deadline. I wanted to originally make the drawing change from soft day-colored gradients to vivid night-colored gradients but time was running short and i settled on a grey-to-saturated fill instead, which i am relatively happy with. Unfortunately, WordPress was not kind to my aspect ratio which i so meticulously planned out in a 640 x 480 arrangement but which was unceremoniously snipped by the WordPress formatting gods. In the future i will try to orient my canvases vertically to avoid cropping. 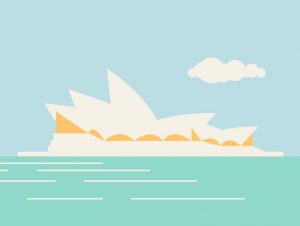