sketch
var earthWH = 128;
var earthX = 240;
var earthY = 240;
var landXY1 = 0;
var landXY2 = 0;
var landXY3 = 0;
var landXY4 = 0;
var dirX = 1;
var dirY = 1;
var dirXComet = 1;
var dirYComet = 1;
var speed = 0.1;
var speedComet = 0.1
function setup() {
createCanvas(480, 480);
}
function draw() {
rectMode(CENTER);
angleMode(DEGREES);
var mil = millis();
var sec = second();
var min = minute();
var h = hour();
var revert = h % 12
background(2, 53, 61);
noStroke();
fill(65, 200, 225);
ellipse(earthX, earthY, earthWH, earthWH);
earthX += dirX * speed/2; earthY += dirY * speed/2; if (earthX > width/2 + 0.5 || earthX < width/2 - 0.5) {
dirX = -dirX;
}
else if (earthY > height/2 + 0.5 || earthY < height/2 - 0.5) {
dirY = random(-1, 1);
}
push();
translate(width/2, height/2)
rotate(revert);
push()
rotate(12.45);
fill(6, 181, 153);
ellipse(landXY1-50, landXY1, 16.8, 39.5);
landXY1 += dirX * (speed/2); landXY1 += dirY * (speed/2);
pop();
push()
rotate(1);
fill(6, 181, 153);
ellipse(landXY2-10, landXY2-25, 12.725, 21.3);
landXY2 += -dirX * (speed/4); landXY2 += -dirY * (speed/4);
pop();
push()
rotate(20);
fill(6, 181, 153);
ellipse(landXY2-13, landXY2-14, 21.21, 26.1);
pop();
push()
rotate(-10);
fill(6, 181, 153);
ellipse(landXY2-1, landXY2-15, 15.9, 20.4);
pop();
push()
rotate(0);
fill(6, 181, 153);
ellipse(landXY2-3.4, landXY2-7.6, 15.9, 20.4);
pop();
push()
rotate(20);
fill(6, 181, 153);
ellipse(landXY3+36, landXY3+18.7, 30, 46.12);
landXY3 += dirX * (speed/3);
landXY3 += -dirY * (speed/3); pop();
push()
rotate(-20);
fill(6, 181, 153);
ellipse(landXY4+30, landXY4-40, 18, 18.5);
landXY4 += -dirX * (speed/6); landXY4 += dirY * (speed/6);
pop();
pop();
push();
translate(width/2, height/2); for (var i = 0; i < sec/3; i++) {
rotate(mil/30); stroke(160, 42, 56)
strokeWeight(1)
line(230-width/2, 140.3-height/2, 220-width/2, 140.3-height/2);
}
pop();
push();
translate(width/2, height/2); for (var i = 0; i < 10; i++) {
rotate(mil/30); stroke(160, 42, 56)
strokeWeight(2)
line(230-width/2, 140.3-height/2, 220-width/2, 140.3-height/2);
}
pop();
push();
translate(width/2, height/2); rotate(sec*6); fill(160, 42, 56)
triangle(240-width/2, 135.8-height/2, 234-width/2, 140.4-height/2, 240-width/2, 144.8-height/2);
fill(187, 42, 47)
triangle(240-width/2, 130.7-height/2, 264-width/2, 140.5-height/2, 240-width/2, 150.43-height/2);
stroke(2, 53, 61)
strokeWeight(1)
line(240-width/2, 140.3-height/2, 251-width/2, 140.3-height/2)
pop();
push();
translate(width/2, height/2); rotate(min); noStroke();
fill(129, 166, 176);
rect(247.2-width/2, 67.3-height/2, 34.06, 2, 30);
fill(129, 166, 176);
rect(242.7-width/2, 73.635-height/2, 34.06, 2, 30);
fill(129, 166, 176);
rect(249.16-width/2, 81.05-height/2, 34.06, 2, 30);
fill(195, 215, 216);
rect(252.66-width/2, 62.941-height/2, 35.35, 3.05, 30);
fill(195, 215, 216);
rect(230.14-width/2, 69.33-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(243.5-width/2, 78.22-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(233.8-width/2, 82.06-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(252.66-width/2, 86.25-height/2, 35.35, 3.05, 30);
fill(195, 215, 216);
ellipse(269.83-width/2, 74.6-height/2, 27.66, 26.07);
fill(129, 166, 176);
ellipse(270.8-width/2, 74.6-height/2, 22.76, 23.33);
fill(37, 60, 84)
ellipse(272.4-width/2, 74.6-height/2, 20.64, 20.64);
push();
translate(272.4-width/2, 74.6-height/2);
rotate(mil/1.5); fill(195, 215, 216);
push();
rotate(20);
ellipse(1.2, -7, 6, 1.6)
pop();
push();
rotate(-20);
ellipse(0.75, 8, 6, 2.5)
pop();
push();
rotate(50);
ellipse(-1, 8, 6, 2)
pop();
pop();
pop();
fill(163, 77, 47);
textSize(15);
text(nf(revert, [2], [0]) + " : " + nf(min, [2], [0]) + " : " + nf(sec, [2], [0]), width/2 - 35, 470);}
My idea stemmed around a nuanced, playful version of the act of orbiting — I wanted to show the journey of a paper airplane flying around the world, placing it at a scale similar to a handful of satellites. The plane’s movement represents seconds, the comet’s orbit’s minutes, and the rotation of the land masses on the earth represents hours, while the smaller dashed line are a creative play on figuring out how to use milliseconds to display agile, not-jittery movement.
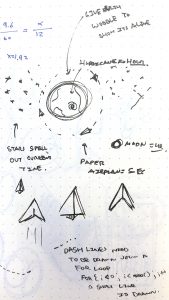
After sketching my initial ideas down, I used Adobe Illustrator to plan out the look of the elements as well as the program’s composition.
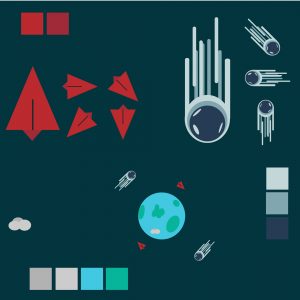