//Hanna Jang
//Section B
//hannajan@andrew.cmu.edu;
//Project_10
//background mountian display characteristics
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
var terrainSpeed1 = 0.0008;
var terrainDetail1 = 0.008;
var terrainSpeed2 = 0.0010;
var terrainDetail2 = 0.01;
//moon and car variables
var moon;
var car;
var carx=150;
var carx2=60;
//array for stars
var stars = [];
function preload() {
//loading images of car and moon
moon = loadImage("https://i.imgur.com/EWntReN.png");
car= loadImage("https://i.imgur.com/kx6JqDC.png");
}
function setup() {
createCanvas(480, 480);
frameRate(10);
// create an initial collection of stars
for (var i = 0; i < 15; i++){
var rx = random(width);
stars[i] = makestar(rx);
}
frameRate(10);
}
function draw() {
background(47, 94, 123);
noFill();
//moon image in the sky
image(moon, 320, 60);
//draw background mountian that is farthest from road
beginShape();
for (var x2 = 0; x2 < width; x2++) {
var t = (x2 * terrainDetail2) + (millis() * terrainSpeed2);
var y2 = map(noise(t), 0,1, height/3.5, height/2);
vertex(x2, y2);
stroke(53, 80, 102);
line(x2, height, x2, y2);
}
endShape();
//draw background mountian that is between the other two mountains
beginShape();
for (var x1 = 0; x1 < width; x1++) {
var t = (x1 * terrainDetail1) + (millis() * terrainSpeed1);
var y1 = map(noise(t), 0,1, height/3, height*3/4);
vertex(x1, y1);
stroke(15, 51, 79);
line(x1, height, x1, y1);
}
endShape();
//draw background mountian that is closest to road
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, height/2, height);
vertex(x, y);
stroke(10, 31, 47);
line(x, height, x, y);
}
endShape();
//draw road
fill(0);
rect(0, height-40, width, 20);
//car image driving
image(car, carx, height-carx2);
//stars are displayed
updateAndDisplaystars();
addNewstarsWithSomeRandomProbability();
}
function updateAndDisplaystars(){
// Update the star's positions, and display them.
for (var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function addNewstarsWithSomeRandomProbability() {
// With a very tiny probability, add a new star to the end.
var newstarLikelihood = 0.05;
if (random(0,1) < newstarLikelihood) {
stars.push(makestar(width));
}
}
// method to update position of stars every frame
function starMove() {
this.x += this.speed;
}
// draw the stars
function starDisplay() {
var skyHeight = 50;
var bHeight = this.nsky * skyHeight;
stroke(255);
fill(251, 248, 36);
push();
translate(this.x, height - 100);
rotate(PI/3.0);
rect(0, -bHeight, 5, 5);
pop();
}
//make the stars
function makestar(birthLocationX) {
var str = {x: birthLocationX,
breadth: 60,
speed: -1.0,
nsky: round(random(2,9)),
move: starMove,
display: starDisplay}
return str;
}
For this week’s project, I knew that I wanted to recreate a very specific memory. I wanted to recreate the car drives my family would have in the countryside late at night, when we went on road-trips.
I recreated this by making sure that the car looked like it was continuously moving forward, when it actually was a still image on the canvas. This was done by making sure the background image in the back of the car was moving. The mountains in the background give the image a more 3-D look.
In my memory, there were many bright stars in the sky during those drives. I randomized the position of these stars in the sky. I am overall pleased with the outcome of my project.
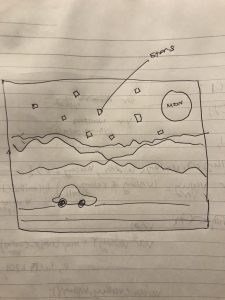
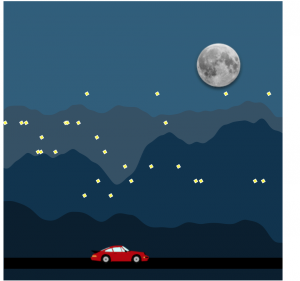