var posX = 75; //starting position of tile x direction
var posY = 20;// starting position of tile y direction
var iteration = 0; //lets program know whether its odd or even row
var count = 0; //know when to terminate the program with noLoop
function setup() {
createCanvas(600, 450);
background(0, 0, 75);
}
function draw() {
if(iteration === 0){ //even number rows get 4 tiles
for(var s = 0; s < 4; s++){
tile(posX, posY);
posX += 150;
}
iteration++;
}
else if(iteration === 1){ //odd number rows get 3 tiles to provide hexagonal effect
for(var d = 0; d < 3; d++){
tile(posX + 75, posY);
posX += 150;
}
iteration--;
}
posX = 75;
posY += 100;
if(count > 13){
noLoop();
}
}
function tile(x, y){ //new function actually producing the tile with x, y parameters
stroke(220, 219, 214);
strokeWeight(1);
line(x, y, x - 50, y + 50);
line(x - 50, y + 50, x, y + 100);
line(x, y + 100, x + 50, y + 50);
line(x + 50, y + 50, x, y);
line(x, y, x - 40, y + 50);
line(x - 40, y + 50, x, y + 100);
line(x, y + 100, x + 40, y + 50);
line(x + 40, y + 50, x, y);
line(x, y, x - 30, y + 50);
line(x - 30, y + 50, x, y + 100);
line(x, y + 100, x + 30, y + 50);
line(x + 30, y + 50, x, y);
line(x, y, x - 20, y + 50);
line(x - 20, y + 50, x, y + 100);
line(x, y + 100, x + 20, y + 50);
line(x + 20, y + 50, x, y);
line(x, y, x - 10, y + 50);
line(x - 10, y + 50, x, y + 100);
line(x, y + 100, x + 10, y + 50);
line(x + 10, y + 50, x, y);
count++;
}
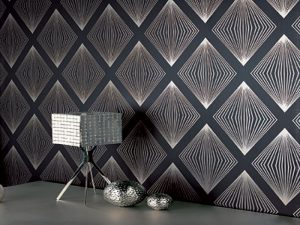
I was inspired by a simple, modern wall paper to create a singular tile that would be repeated in the hexagonal pattern we learned about in Assignment 5B. I created a new function tile(x, y) with two parameters (starting x location and starting y location) to create a set of tiles on a background.