//Audrey Zheng
//Section A
//audreyz@andrew.cmu.edu
//Project 9
var img;
function preload() {
var me = "https://i.imgur.com/iiz3eb4.jpg";
img = loadImage(me);
}
function setup() {
createCanvas(480, 480);
background(0);
img.loadPixels();
frameRate(100);
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width);
var iy = constrain(floor(py), 0, height);
var color = img.get(ix,iy);
noStroke();
fill(color);
shape(px,py,random(4,9),random(9,15));
}
function shape(x, y, r, n) {
var angle = TWO_PI / n;
beginShape();
for (var a = 0; a < TWO_PI; a += angle) {
var sx = x + cos(a) * r;
var sy = y + sin(a) * r;
vertex(sx, sy);
}
endShape(CLOSE);
}
Category: Section A
Portrait 9 rrandell
/* Rani Randell
rrandell@andrew.cmu.edu
Section A
Project 9 */
var underImage;
function preload() {
var ImageURL = "https://i.imgur.com/lmhDtNm.jpg?3";
underImage = loadImage(ImageURL);
}
function setup() {
createCanvas(480, 480);
background(0);
underImage.loadPixels();
frameRate(1000);
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width-1);
var iy = constrain(floor(py), 0, height-1);
var theColorAtLocationXY = underImage.get(ix, iy);
noStroke();
fill(theColorAtLocationXY);
//text('bff', px, py); part of my experimentation
rect(px, py, 5, 5);
ellipse(px, py, 10, 10);
//ellipse(px + 6, py, 5, 5)
}
Although Georges Seurat’s paintings were not provided as examples for this project I immediately thought of his work, that I am sure is familiar to many of you. I was really inspired by the painterly quality of his work and tried to include that in my portrait using teardrop shapes to stimulate paint blobs. I included one of Seurat’s paintings below:
This is the original underlying picture
This image was me playing around with shapes
Jonathan Liang – Project 9 – Computational Portrait
//Jonathan Liang
//jliang2
//section A
var springy = 0.7; // how much velocity is retained after bounce
var np = 50; // how many particles
var underlyingImage;
function preload() {
var myImageURL = "https://i.imgur.com/obcoL1tl.jpg";
underlyingImage = loadImage(myImageURL);
}
function particleStep() {
var ix = constrain(floor(this.x), 0, width-1);
var iy = constrain(floor(this.y), 0, height-1);
var theColorAtLocationXY = underlyingImage.get(ix, iy);
noStroke();
fill(theColorAtLocationXY)
ellipse(this.x, this.y, 5);
this.x += this.dx;
this.y += this.dy;
if (this.x > width) { // bounce off right wall
this.x = width - (this.x - width);
this.dx = -this.dx * springy;
} else if (this.x < 0) { // bounce off left wall
this.x = -this.x;
this.dx = -this.dx * springy;
}
if (this.y > height) { // bounce off bottom
this.y = height - (this.y - height);
this.dy = -this.dy * springy;
} else if (this.y < 0) { // bounce off top
this.y = -this.y;
this.dy = -this.dy * springy;
}
}
// create a "Particle" object with position and velocity
function makeParticle(px, py, pdx, pdy) {
p = {x: px, y: py,
dx: pdx, dy: pdy,
step: particleStep,
}
return p;
}
var particles = [];
var randx = [];
var randy = [];
function setup() {
createCanvas(380, 480);
background(0);
underlyingImage.loadPixels();
for (i = 0; i < np; i++) {
randx[i] = random(-50, 50);
randy[i] = random(-50, 50);
}
frameRate(5);
}
// draw all particles in the particles array
//
function draw() {
background(230);
for (var i = 0; i < np; i++) {
// make a particle
var p = makeParticle(200, 350,
randx[i], randy[i]);
// push the particle onto particles array
particles.push(p);
}
for (var i = 0; i < particles.length; i++) { // for each particle
var p = particles[i];
p.step();
}
}
I chose a picture of my friend from high school. I wanted the ellipses that generated the image to first start from his mouth and then bounce everywhere to generate the image. Kind of like vomiting, but with a more artistic result. The ellipses slow down after they hit the walls so that it is clearer that they are bouncing and not just randomly generating wherever they want. Below is a few screenshots of the image being generated over time.
Looking Outwards – 09 Min Lee
“Vektron Modular” by Niklas Roy, 2010
The Looking Outwards assignment that I found interesting is Katherine Hua’s assignment on Niklas Roy’s “Vektron Modular”. This project by Roy stores algorithmic sound compositions within microcontroller modules that can be played back on a compatible device. The attached synthesizer on the device can alter parameters of the algorithm that produce the sounds, which is why the audio becomes distorted as Roy plays with the joystick in the video above.
What stood out to me about this project was the inspiration and technical applications that are possible through it. The device was inspired by Sony’s Playstation, which Katherine very accurately describes in her assignment. The application that Niklas Roy found for his device came through the musical exploration possibilities that follow the device. Through various switches on the device, the user is able to explore the binary structures within music and compare different rhythmic patterns and number systems for counting beats. An interesting and productive assessment of this device could be made from a strictly musical production standpoint by using it to create new and interesting instrumentals.
Source: https://courses.ideate.cmu.edu/15-104/f2018/2018/09/21/katherine-hua-looking-outwards-04/
Katherine Hua – Looking Outwards-09
I really liked Min Lee’s looking outwards blog post on this project called Skyline. Skyline is a code-based generative music video that uses Voronoi tessellation. Skyline’s systems have seeds that are categorized into different types of agents that represent a certain behavior or trigger transformations in appearance. The song’s audio spectrum or the animation sequence of the artist acts as the driver of these motions, creating beautiful patterns that come together to make a complex visual conclusion. I agree with how Min that this project is amazing in the way that the artist was able to create a visual representation of musical behavior through patterns of geometric shapes and inkblot-like figures. I’d like to add that I think it’s very interesting that throughout this music video, the shapes come together to form a figure of the vocalist or the face of the vocalist while the visual elements are moving with the sound of the instrumentals.
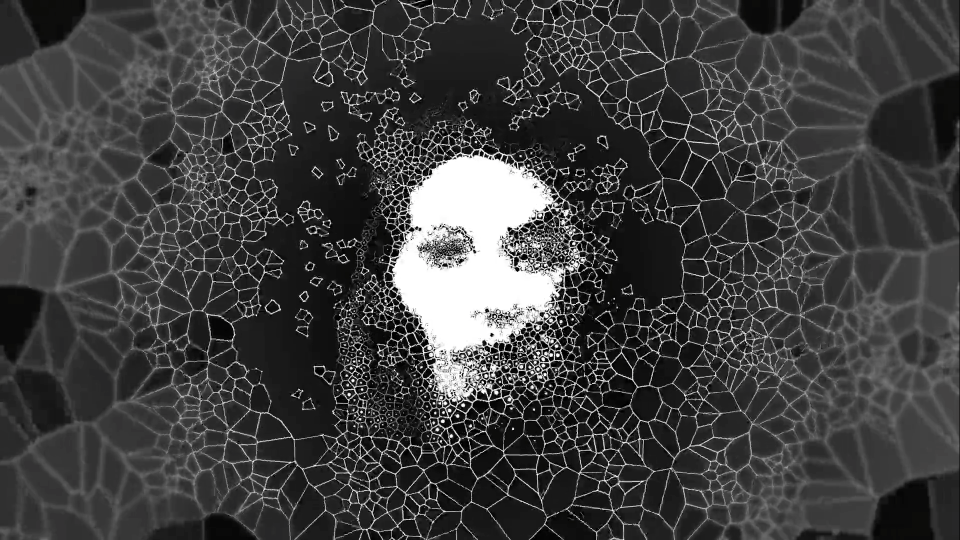
Curran Zhang- Project 09- Portrait
/*Curran Zhang
curranz
Project 9
Section A
*/
var Ball = [];
var img = ['https://i.imgur.com/6PlJkcb.jpg',
'https://i.imgur.com/MIU3YvB.jpg']
var dir;
var picIndex = 1;
var currentIndex = 0;
function preload(){
pic = loadImage(img[picIndex]);
}
function setup(){
createCanvas(480,480);
pic.loadPixels();
background(50);
dir = random(5,10);
}
function properties(px,py,pdy){
b = { x: px,
y: py,
dy: pdy,
speed: ballSpeed,
ball: ballMake,
bSize: random(3,6)
}
return b;
}
function ballMake(){
var xx = constrain(floor(this.x),0,width-1);
var yy = constrain(floor(this.y),0,height-1);
var pix = pic.get(this.x, this.y);
fill(pix)
stroke(0);
strokeWeight(0.1);
ellipse(this.x, this.y, this.bSize, this.bSize);
}
function ballSpeed(){
this.y += this.dy * random(.1,.3);
}
function draw(){
//Creating an array of all the individual balls
newBall =[];
for (var i = 0; i <Ball.length; i++) {
var b = Ball[i];
b.speed();
b.ball();
newBall.push(b);
}
Ball = newBall;
//Applying all the drawn properties onto the canvas
var bb = properties(random(width), 0, dir);
Ball.push(bb);
}
/*
//Failed attempt to shuffle through the array to switch the image
function mouseClicked(){
picIndex = int(random(0,1))
while (currentIndex == picIndex){
picIndex = int(random(0,1))
}
currentIndex = bodyIndex;
}
*/
For this project, I used pictures from my friend Kelly. I tried to create a snow falling effect of the individual pixels. I tried to place the pictures into an array which would eventually shuffle through with mouseClicked(), however it would just freeze up. Even though it didn’t work, I am satisfied with the glittery effect that it produces.
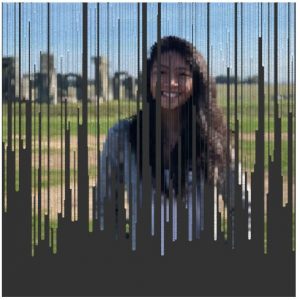
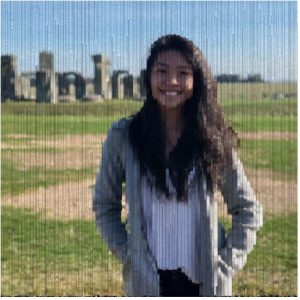
Katherine Hua – Project-09 – Portraits
/* Katherine Hua
Section A
khua@andrew.cmu.edu
Project-09-Portrait */
var klaire;
var x;
var y;
var dx;
var dy;
var color;
function preload() {
var imageLink = "https://i.imgur.com/DijwpWY.jpg"; //link of image
klaire = loadImage(imageLink); //loading image onto canvas
}
function setup() {
createCanvas(500, 500);
background(42, 57, 70);
klaire.loadPixels(); //loading pixels of image
frameRate(500); //making it draw faster
x = random(width); //randomizing x between constraints of width
y = random(height); //randomizing y between constraints of height
dx = random(-1,1); //randomizing direction
dy = random(-1,1); //randomizing direction
}
function draw() {
var ix = constrain(floor(x), 0, width-1);
var iy = constrain(floor(y), 0, height-1);
color = klaire.get(ix, iy); //getting pixel color according pixel position in comparison to pixel in image
fill(color); //making color at this pixel position the same color as in the image's pixel position
textSize(10);
text("loading", x, y);
x += dx * 5 ;
y += dy * 5 ;
//when the word "loading" hits the edge of the canvas, direction is changed randomly
if (x > width || x < 0) {
dx = -dx;
dy = random(-1, 1);
}
if (y > height || y < 10) {
dy = -dy;
dx = random(-1, 1);
}
}
For this project, I chose my portrait to be of my twin sister, Klaire (because I miss her). She goes to school on the west coast in California, so the only times we can see each other now are breaks. I chose to use text to create compute the portrait of her. I like how when all the text builds up, it begins to look like strokes of paint instead of text.
This is what is looks like at the very beginning. The text “loading” is drawn randomly across the canvas. Each time it hits a wall, it will change direction at a random angle and speed.
As more and more build on top of each other, the image starts coming together to create this.
This is the original portrait of Klaire. <3
Project 09 – Portrait
var underlyingImage;
var textChoice;
function preload() {
var me = "https://i.imgur.com/UDfjk6L.jpg";
underlyingImage = loadImage(me);
}
function setup() {
createCanvas(500, 500);
background(0);
underlyingImage.loadPixels();
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width);
var iy = constrain(floor(py), 0, height);
var theColorAtLocationXY = underlyingImage.get(ix, iy);
//randomizes text between three choices
textChoice = random(0, 1000)
noStroke();
//size of text changes as mouse moves to right
textSize(mouseX / 50)
fill(theColorAtLocationXY);
if (textChoice > 600) {
text("bruh", px, py);
} else if (textChoice < 600 & textChoice > 400) {
text("wow", px, py);
} else {
text("very", px, py);
}
}
//press mouse to reset
function mousePressed() {
background(0);
}
For this project, I wanted to channel the energy of memes to shape an appropriate photo of myself. I was very surprised to learn how simple it is to use images and extract colors for certain pixels, which will be helpful in creating future projects.
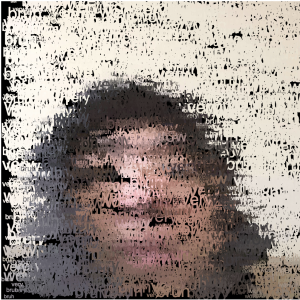
Anthony Ra – Project 09 – Portrait
/* Anthony Ra
Section-A
ahra@andrew.cmu.edu
Project-09 */
var heaven;
var start = 100;
/* spelling of heaven as arrays to fill the image */
var name = ["H", "E", "A", "V", "E", "N"];
function preload() {
var heavenUrl = "http://i.imgur.com/ILpL0Fu.jpg" /* uploading picture */
heaven = loadImage(heavenUrl);
}
function setup() {
createCanvas(400, 400);
background(0);
imageMode(CENTER);
heaven.resize(400, 400);
heaven.loadPixels();
frameRate(5000);
}
function draw() {
/* series of sizes for the letters */
var size = random(20, 35);
/* starting point */
var px = randomGaussian(width/2, start);
var py = randomGaussian(height/2, start);
/* values constrained to the picture */
var heavenX = constrain(floor(px), 0, width);
var heavenY = constrain(floor(py), 0, height);
/* getting colors from the picture */
var col = heaven.get(heavenX, heavenY);
/* induces the speed of pixels array */
var i = floor(random(5));
push();
translate(px, py);
noStroke();
fill(col);
textSize(size);
text(name[i], 0, 0);
pop();
}
I don’t have any pictures of myself because .. I don’t. So, I used a personally funny picture of a dear friend who is always salty. Using a play on his name (Evan), I randomized the letters in the word “HEAVEN” and given each color based on the image. The origin of “Heaven” came from a typo from Au Bon Pain. I increased the rate of the letters appearing to an astronomical amount because I just want to see this face haha.
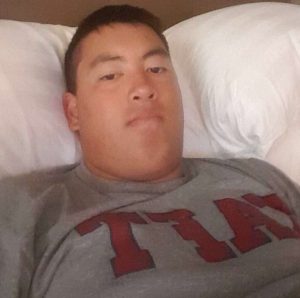
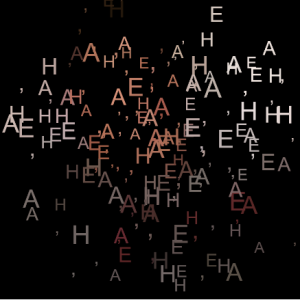
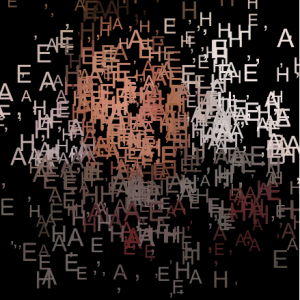
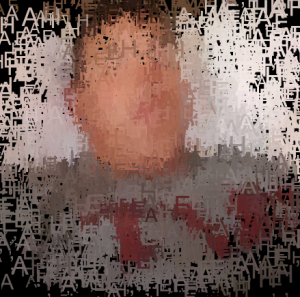
Anthony Ra – Looking Outwards 09
For this looking outwards post, I looked into the post of randomness that Curran Zhang wrote. An art installation by Kling Klang Klong in 2018, this piece that spans through multiple screens utilizes sound composition from the audience to generate parcels of data arranged to create unpredictable currents.
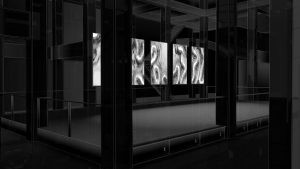
I guess a part of the common ground in which Curran and I were impressed with this work is the involvement of the audience into an already finished final work. As if we, the people, contribute to this well-generated piece.
One thing that is difficult in choosing this project is that I only see articles on this installation and no videos of it in the works. As much as the photos that are available are intriguing and eye-catching, I think that watching its movement and its randomness with a video will allow me to appreciate this installation much more.
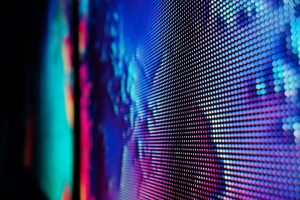
What Curran said at the end is very important to the direction of where art and design should go. The importance of collaboration and the uses of different fields in ways that people would think be impossible.