sketch
/* Eunice Choe
Section E
ejchoe@andrew.cmu.edu
Project 06*/
// global variables for wheel position and size
var bigX = 160;
var bigY = 320;
var bigDiam = 200;
var smallX = 370;
var smallDiam = 70;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(172, 201, 232);
angleMode(DEGREES);
var H = hour();
var M = minute();
var S = second();
var cH = map(H, 0, 23, 50, width - 50);
// sidewalk
noStroke();
fill(171, 184, 182);
rect(0, 320, width, 320);
// changing sun position depending on the hour
// changing from sun to moon depending on time
// 7AM to 7PM shows sun while 7PM to 7AM shows moon
if(H < 19 & H > 7){
noStroke();
fill(237, 232, 73);
} else {
fill(88, 92, 94);
}
ellipse(cH, 100, 100, 100);
// Time text
strokeWeight(0.5);
fill(82, 9, 6);
textAlign(CENTER);
text("Hour: " + H, cH, 100);
text("Minute: " + M, smallX, 450);
text("Second: " + S, bigX, 450);
// thin red bike part
strokeWeight(10);
stroke(82, 9, 6)
line(bigX + 110, bigY - 110, bigX + 120, bigY - 130);
// yellow bike parts
stroke(176, 117, 15);
line(bigX + 30, bigY - 200, bigX + 35, bigY - 230);
line(bigX + 35, bigY - 230, bigX, bigY - 250);
line(bigX, bigY - 250, bigX - 20, bigY - 240);
ellipse(bigX, bigY - 250, 10, 10);
// bike seat
fill(176, 117, 15);
line(bigX + 90, bigY - 160, bigX + 120, bigY - 150);
ellipse(bigX + 130, bigY - 150, 20, 15);
// thick red bike parts
stroke(82, 9, 6);
strokeWeight(15);
line(bigX, bigY, bigX + 30, bigY - 200);
line(bigX + 30, bigY - 160, bigX + 120, bigY - 100);
line(bigX + 120, bigY - 100, smallX, bigY + bigDiam / 2 - smallDiam / 2);
// wheels
noFill();
strokeWeight(15);
stroke(255, 250, 202);
ellipse(bigX, bigY, bigDiam, bigDiam);
ellipse(smallX, bigY + bigDiam / 2 - smallDiam / 2, smallDiam, smallDiam);
// the rotating seconds wheel
for(var s = 0; s < S; s++){
push();
translate(bigX, bigY);
rotate(6 * s); // 60 seconds - 60 spokes
stroke(255);
strokeWeight(2);
line(0, 0, 0, -100);
pop();
}
// the rotating minutes wheel
for(var m = 0; m < M; m++){
push();
translate(smallX, bigY + bigDiam / 2 - smallDiam / 2);
rotate(6 * m); // 60 minutes - 60 spokes
stroke(255);
strokeWeight(1);
line(0, 0, 0, -35);
pop();
}
}
My abstract clock is a bicycle with the big wheel representing seconds, the small wheel represent minutes, and the sun/moon representing the hour. For the second and minute wheels, new spokes appear after every second/minute passes. From 7AM to 7PM, the sun is yellow and from 7PM to 7AM the circle turns gray to represent the moon. In addition, the position of the sun/moon changes depending on what time it is.
I came up with the idea of a bike because of the two circular shapes I could work with to represent minutes and seconds. I had to get a little creative to represent the hours, so I chose to use something in the background. The abstract clock was difficult to figure out at times, but overall I feel better about applying rotation and for loops into my code.
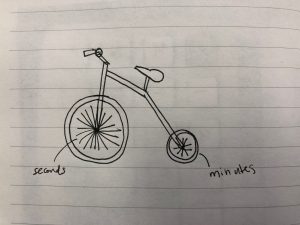
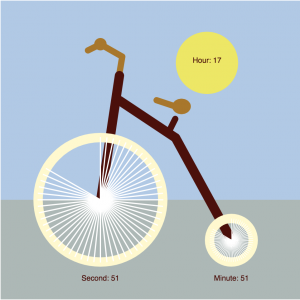
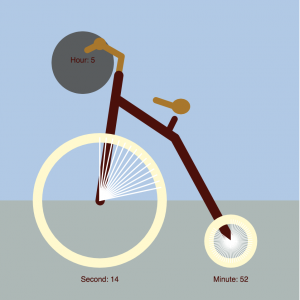
The bike in day time and night time.