The Computer Composer game, which is featured on the website of The Mathenæum, allows you to choose to generate a short musical composition with the assistance of a computer. For every input of notes and rhythms, you can either manually input values or choose to have the computer randomly assign them for you. It is interesting to see the limitations of randomness within music; for example, this program specifically only writes in C major or A natural minor, which eliminates 5 of the possible notes in each octave. It is also limited to one and a half octaves, as opposed to the 7 usually present on a piano. Compositionally, the creation of rhythms is always much more variable, as is the case even more clearly in this program.
Month: October 2021
Project-06: Abstract Clock
var x = [];
var y = [];
function setup() {
createCanvas(450, 380);
var hr = hour();
var min = minute();
var sec = second();
//set range for displaying sec location
for (i = 0; i <= 60; i ++) {
x[i] = random(315, 345);
y[i] = random(255, 285);
}
}
function draw() {
background(7, 55, 99);
var hr = hour();
var min = minute();
var sec = second();
var mapHr = map(hr, 0, 20, 0, width);
var mapMin = map(min, 0, 50, 0, width);
var mapSec = map(sec, 0, 50, 0, width);
//flowerpot
fill(76, 32, 1);
strokeWeight(8);
stroke(102, 42, 0);
ellipse(110, 110, 110, 110);
//succulent leaf [represents hour]
if (hr > 12) {
hr = hr % 12;
}
noStroke();
fill(114, 196, 143);
for (var i = 0; i < hr; i ++) {
push();
translate(110, 110);
rotate(radians(i * 30));
ellipse(0, -30, 20, 50);
pop();
}
//flower
fill(244, 204, 204);
ellipse(110, 97, 19, 19);
ellipse(95, 108, 19, 19);
ellipse(125, 108, 19, 19);
ellipse(101, 125, 19, 19);
ellipse(119, 125, 19, 19);
ellipse(110, 111, 21, 21);
fill(255, 229, 153);
ellipse(110, 112, 13, 13);
//coffee plate
fill(220, 200, 186);
stroke(255, 248, 244);
strokeWeight(2);
ellipse(330, 270, 100, 100);
//coffee handle [represents minute]
noStroke();
push();
translate(330, 270);
rotate(radians(min * 6));
fill(255, 248, 244);
rect(-6, -60, 12, 60, 4);
pop();
//coffee cup
strokeWeight(3);
fill(220, 200, 186);
stroke(255, 248, 244);
ellipse(330, 270, 70, 70);
//coffee
fill(78, 34, 0);
stroke(120, 52, 0);
strokeWeight(2);
ellipse(330, 270, 53, 53);
//coffee bubble [represents second]
for (i = 0; i < sec; i ++) {
fill(120, 52, 0);
ellipse(x[i], y[i], 2, 2);
}
//keyboard
noStroke();
fill(188, 188, 188);
rect(280, 30, 300, 140, 7);
fill(238, 238, 238);
rect(280, 30, 300, 10, 1);
//keyboard keys
fill(255, 255, 255);
stroke(145,145,145);
strokeWeight(1);
rect(288, 48, 19, 11, 2); //first row keys
rect(310, 48, 19, 11, 2);
rect(332, 48, 19, 11, 2);
rect(354, 48, 19, 11, 2);
rect(376, 48, 19, 11, 2);
rect(398, 48, 19, 11, 2);
rect(420, 48, 19, 11, 2);
rect(442, 48, 19, 11, 2);
rect(288, 63, 19, 17, 2); //second row keys
rect(310, 63, 19, 17, 2);
rect(332, 63, 19, 17, 2);
rect(354, 63, 19, 17, 2);
rect(376, 63, 19, 17, 2);
rect(398, 63, 19, 17, 2);
rect(420, 63, 19, 17, 2);
rect(442, 63, 19, 17, 2);
rect(288, 84, 30, 17, 2); //third row keys
rect(321, 84, 19, 17, 2);
rect(343, 84, 19, 17, 2);
rect(365, 84, 19, 17, 2);
rect(387, 84, 19, 17, 2);
rect(409, 84, 19, 17, 2);
rect(431, 84, 19, 17, 2);
rect(288, 105, 37, 17, 2); //fourth row keys
rect(328, 105, 19, 17, 2);
rect(350, 105, 19, 17, 2);
rect(372, 105, 19, 17, 2);
rect(394, 105, 19, 17, 2);
rect(416, 105, 19, 17, 2);
rect(438, 105, 19, 17, 2);
rect(288, 126, 49, 17, 2); //fifth row keys
rect(340, 126, 19, 17, 2);
rect(362, 126, 19, 17, 2);
rect(384, 126, 19, 17, 2);
rect(406, 126, 19, 17, 2);
rect(428, 126, 19, 17, 2);
rect(450, 126, 19, 17, 2);
rect(288, 147, 19, 17, 2); //sixth row keys
rect(310, 147, 19, 17, 2);
rect(332, 147, 19, 17, 2);
rect(354, 147, 19, 17, 2);
rect(376, 147, 100, 17, 2);
//iPad
fill(45, 45, 45);
stroke(255, 255, 255);
strokeWeight(10);
translate(90, 230);
rotate(PI / 5.0);
rect(0, 0, 130, 200, 3);
fill(0);
noStroke();
ellipse(65, 0, 3, 3);
}
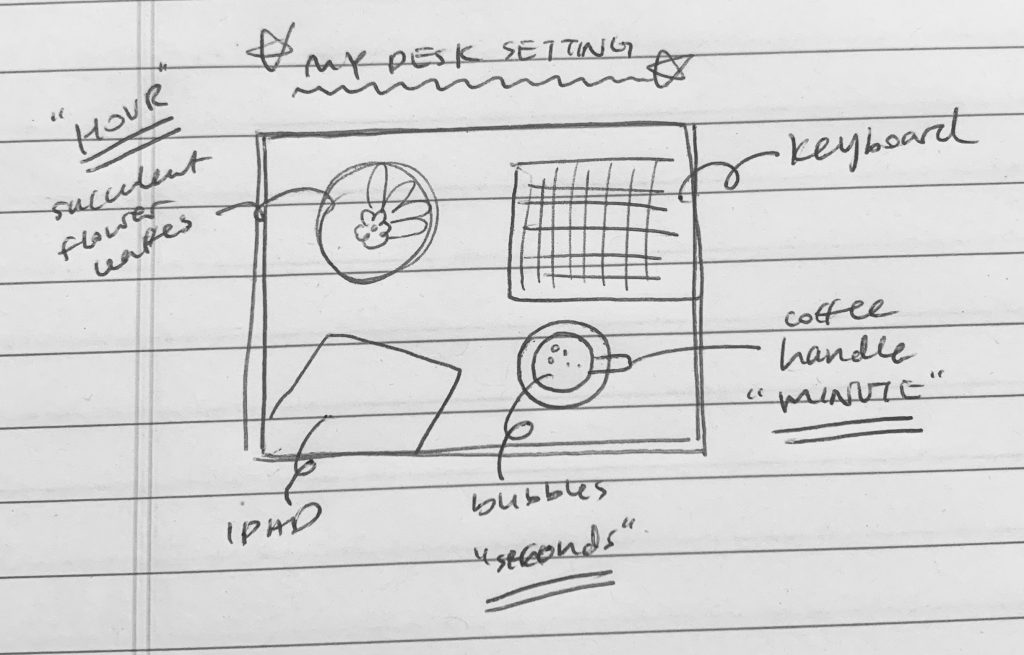
For this project, I wanted to create a 12-hour clock illustration using a top-view desk setting. To represent the numerical value of ‘hour’, I used the leaves of the flower. To represent the ‘minute’, I used the handle of the coffee cup to direct actual minute. Last but not least, I used the bubbles of coffee to represent ‘second’. In addition, I added details of my desk of an iPad along with my MacBook keyboard. It was super fun to depict my desk surrounding into this project.
LO 06: Randomness
Geoffrey Bradway’s mesmerizing yet hallucinatory patterns are created with repeated overlays of dynamic lines using machine learning and artificial intelligence. Bradway generates powerful computational art that is beyond one’s imagination, specifically with a program called Python and a plotter called Axidraw V3 A3. When Bradway produces a collection of lines that structures using [A, B, C, D, …], the robot can merely translate this list of points into mechanical operations. For instance, a line [A, B, C] is rendered as “PENUP, GOTO(A), PENDOWN, GOTO(B), GOTO(C), PENUP.” I found his particular style of generating art really fascinating as he uses AI and robots to create beautiful, magnetic piece of art. I admire how he exploits the aspects of rapidness and accuracy in computers, and brilliant intelligence human beings have. Bradway’s artistic sensibility arises as he uses his intuition to direct his artworks the way he wants to shape it with multiple lines of codes.
Reference: https://www.chromatocosmos.com/pop-art
Looking Outwards – 06
I have always been very interested in quantum computers. Out of all the quantum projects that are in progress right now, one of the most interesting is probably the quantum processor being built at Google. Their goal is to use this quantum processor to create “pure randomness”. The main idea around this randomness is derived from the fundamental concept in quantum physics, known as superposition. In quantum physics, superposition is referred to when the Qbits (quantum particles) exist in a state of being both 0 and 1 at the same time. While you are able to use quantum theory to calculate the probability of the bit being either 0 or 1, ultimately the particles in superposition are fundamentally random. With this level of pure randomness, it can help us make big strides in cybersecurity and encryption. In a world basically run by technology, it is important that we have a way of protecting ourselves from cyberattacks, and quantum processors may be the answer.
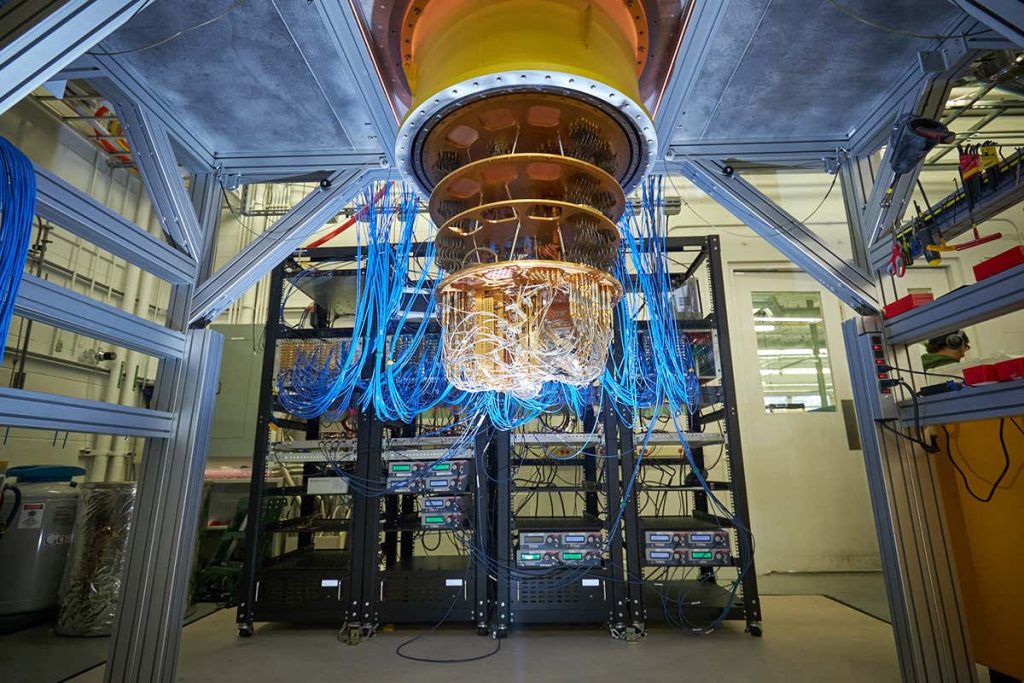
Clock Project
var fall;
var spring;
var winter;
var summer;
function setup() {
createCanvas(480, 480);
angleMode(DEGREES);
textAlign(CENTER);
rectMode(CENTER);
fall = {r1:108, g1:12, b1:12,
r2:75, g2:28, b2:0,
r3:189, g3:81, b3:19};
winter = {r1:253, g1:249, b1:231,
r2:202, g2:213, b2:255,
r3:21, g3:54, b3:186};
spring = {r1:177, g1:244, b1:249,
r2:253, g2:217, b2:73,
r3:255, g3:204, b3:255};
summer = {r1:123, g1:227, b1:243,
r2:255, g2:145, b2:145,
r3:250, g3:244, b3:228};
}
function draw() {
background(220);
translate(240, 240);
date();
rotate(-90);
let hr = hour();
let mn = minute();
let sc = second();
strokeWeight(30);
noFill();
if (m==3 || m==4 || m==5) {
secondscircle(sc, spring);
minutescircle(mn, spring);
hourscircle(hr, spring);
}
else if (m==6 || m==7 || m==8) {
secondscircle(sc, summer);
minutescircle(mn, summer);
hourscircle(hr, summer);
}
else if (m==9 || m==10 || m==11) {
secondscircle(sc, fall);
minutescircle(mn, fall);
hourscircle(hr, fall);
}
else {
secondscircle(sc, winter);
minutescircle(mn, winter);
hourscircle(hr, winter);
}
}
function date() {
stroke(0);
strokeWeight(9);
fill(255);
rect(0,0, width/2,height/3,20);
m = month();
d = day();
y = year();
textSize(50);
fill(0);
strokeWeight(1);
stroke(0);
textFont('Orbitron')
text(m + '.' + d + '.' + y, 0,15);
}
function secondscircle(sc, coloring) {
stroke(coloring.r1,coloring.g1,coloring.b1);
let secondsAngle = map(sc, 0, 60, 0, 360);
arc(0, 0, 450, 450, 0, secondsAngle);
}
function minutescircle(mn, coloring) {
stroke(coloring.r2,coloring.g2,coloring.b2);
let minutesAngle = map(mn, 0, 60, 0, 360);
arc(0, 0, 385, 385, 0, minutesAngle);
}
function hourscircle(hr, coloring) {
stroke(coloring.r3,coloring.g3,coloring.b3);
let hoursAngle = map(hr % 24, 0, 24, 0, 360);
arc(0, 0, 320, 320, 0, hoursAngle);
}
Some challenges I faced were trying to use objects as a system of carrying the different color schemes for the different seasons in the year. Once I got the hang of using objects, I realized how useful and helpful they can be.
Looking Outwards 06: Randomness
This week I took a look at Tyler Hobbs, a generative artist who utilizes randomness in his work. His project “Waves” is a generative piece made for one of four interior murals for a single client. This piece focuses on water and its fragmented nature. Each shape is a different color, but they come together as a very cohesive piece. I suppose that he uses a program to create these different lines that curve and bend on different generated paths. I would assume that at certain coordinates within the canvas, he changes the shades of blue and uses some random function to create spots/highlights of pink. The curves seem to resemble that of a sin or cos wave. The quality of water is very apparent in this piece, and I think that the artist was able to create a very clear abstract representation of water.
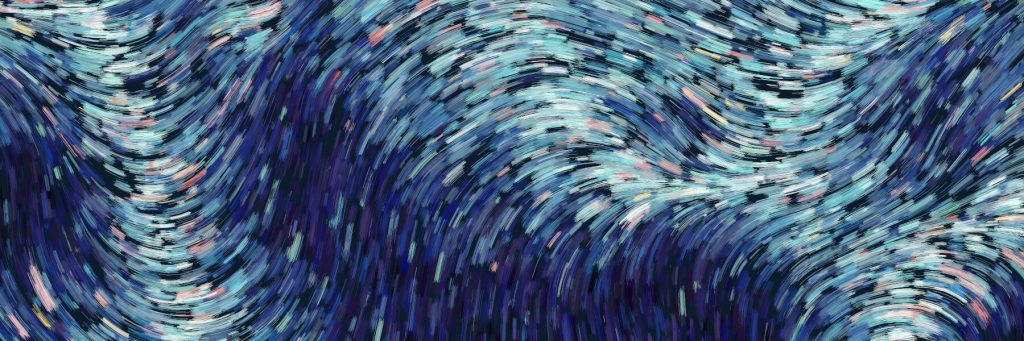
Project 06: Abstract Clock
//Anthony Pan
//Section C
var d = 0; //diameter for 24 hr circles
var d1 = 0; //diameter for 24 hr circles
//var d2; //first circle diameter
//var d3; //first cirlce diameter
var c1 = []; //color array for the 24 hrs of a day
var hours = []; //hours in a day
function setup() {
createCanvas(480, 480);
for(var i = 0; i <= 23; i++) {
hours[i] = i;
if(i >= 0 & i <= 8){
c1[i] = color(0, random(256), random(256)); //random green + blue, no red first 8 hrs
}
if(i > 8 & i <= 16) {
c1[i] = color(random(256), 0 , random(256)); //random red + blue, no green between 8-16 hrs
}
if(i >16 & i<=23) {
c1[i] = color(random(256), random(256), 0); //random red + green, no blue 16-24 hrs
}
}
}
function draw() {
background(0);
draw_circle(d, d1);
//fill_circle(d2, d3);
noStroke();
fill(c1[hour()]); //fill color based on the hour
//draw a circle that grows based on the number of minutes that has passed
ellipse(240, 240, 20*hour() + minute()/3, 20*hour() + minute()/3);
//display clock
textSize(15);
strokeWeight(1);
fill(255);
text(hour() + ":" + minute() + ":" + second(), 10, 470); //display time on 24 hr clock at bottom left corner
}
function draw_circle(d, d1){
for(var i = 1; i <= 25; i++) {
noFill();
stroke(255); //create why circle stroke
ellipse(240, 240, d, d1); //create 24 concentric cirlces for 24 hrs in a day
d += 20;
d1 += 20; //d and d1 grow each cirlce's radius
}
}
//process for creating fill circle
/*
function fill_circle(d2, d3) { //fill circle based on minute
for(var i = 0; i <= hour(); i++) {
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, 10*hour() + d2*minute(), 10*hour() + d3*minute());
d2 += 1/6; //rate of growth based on time /proportions
d3 += 1/6;
print(d2);
}
}
*/
/*
function fill_circle(d2, d3) { //fill circle based on minute
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, 20*hour() + minute()/3, 20*hour() + minute()/3);
}
/*
function draw_circle(d, d1){
for(var i = 0; i <= 23; i++) {
noFill();
stroke(255);
ellipse(240, 240, d, d1); //create 24 concentric cirlces for 24 hrs in a day
d += 20;
d1 += 20; //d and d1 grow each cirlce's radius
}
}
*/
/*
function fill_circle(d2, d3) { //fill circle based on minute
for(var i = 0; i <= minute(); i++) {
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, d2, d3);
d2 += 0.33; //rate of growth based on time /proportions
d3 += 0.33;
}
}
*/
Project Description:
This clock has 24 concentric circles representing the 24 hours in a day. A second function creates a circle that is filled with a random color based on the range of time: 0-8, 8-16, and 16-24. The circle fills the concentric circles slowly, growing based on each minute passed. There is a display of the time on a 24-hour clock at the bottom of the canvas to show the exact time as the circle fills the concentric circles very slowly.
Process/Ideation:
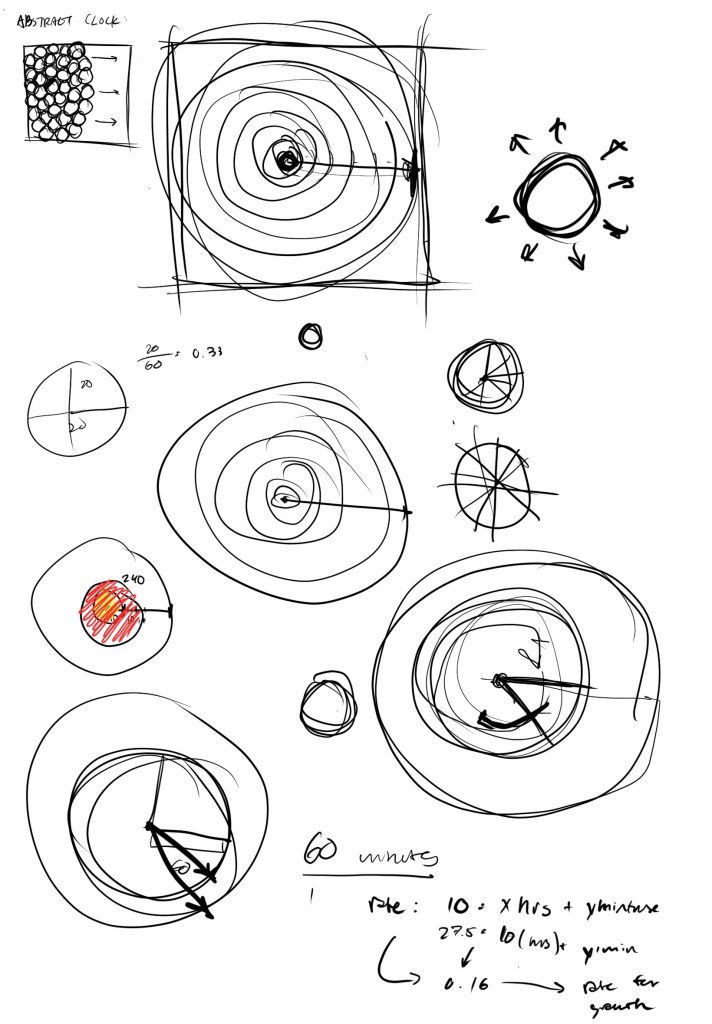
This was some of my process working on creating this clock.
Project-06-Abstract-Clock
function setup() {
createCanvas(400, 400);
angleMode(DEGREES);
}
function draw() {
background(240, 217, 156);
fill(220);
strokeWeight(2);
ellipse(200,200,395);
fill('magenta');
stroke(150,255,100);
ellipse(200,180,50);
fill(183, 156, 240);
strokeWeight(6);
arc(200,250,200,150,0,180,CHORD);
let hr = hour();
let mn = minute();
let sc = second();
strokeWeight(4);
stroke(255,100,150);
noFill();
let end1 = map(sc,0,60,0,360);
arc(120,120,35,35,0,end1);
arc(280,120,35,35,0,end1);
stroke(150,100,255);
let end2 = map(mn,0,60,0,360);
arc(120,120,28,28,0,end2);
arc(280,120,28,28,0,end2);
stroke(150,255,100);
let end3 = map(hr % 12,0,12,0,360);
arc(120,120,20,20,0,end3);
arc(280,120,20,20,0,end3);
stroke("orange");
let s = map(second(),0,60,0,360) - 180;
line(200,180,200 + cos(s)*50, 180 + sin(s)*50);
strokeWeight(2);
text('hehe: \n' + s, 100, 190);
text('hehe: \n' + s, 300, 190);
strokeWeight(1);
stroke('red');
text('Hey_time: \n' + hr + 'hours' + mn + 'minutes', 100, 50);
}
function mouseIsPressed() {
if (s % 5 == 0){
fill('blue');
strokeWeight(6);
stroke(0);
arc(200,250,200,150,0,180,CHORD);
} else {
fill(183, 156, 240);
strokeWeight(6);
stroke(0);
arc(200,250,200,150,0,180,CHORD);
}
}
This project was quite challenging. I had to search through the internet to learn how to call the numbers using javascript. It turned out to be easy. However, contextualizing my concepts to code was again very difficult. I tried to create a cute face using functions that implement the numbers of the clock. However, as always, it turned out quite differently than my sketch. I used lines on the nose and used the print function to print the time on the top left corner of the canvas.
Project 6 Abstract Clock
It was alot of fun making this peice. It was really hard to figure out where to start, but when I decided to split the hour, minute, and second into different parts, I was able to better figure out what to do.
var lengt = 10;
var hr;
var min;
var sec;
function setup() {
createCanvas(480, 480, WEBGL);
hr = hour();
min = minute();
sec = second();
text("p5.js vers 0.9.0 test.", 10, 15);
ellipse(240,240,480);
}
// drawing a plant that grows and dies at 24 hours but then grows from the stump again
function draw() {
background(220);
sec = second();
min = minute();
sec = second();
thePlant(hr);
theSky(min,hr);
theLeaves(sec);
}
function theLeaves(second){ //the leaves or flower changes based on the second
fill("purple");
cone(10+second,65,16,3);
}
function thePlant (hr){ //each hour adds length to the plant
fill("brown");
translate(0,100);
cylinder(60, 100, 16, 16);
//the actual plant
translate(0,-70);
fill("green");
cylinder(20, lengt*hr, 16, 16);
}
function theSky (min,hr){ //changes based on the 12 hr system
if (hr <= 12){
fill(0,0,0.3472*(min + (60*hr)));
}else{
fill(0,0,255 - 0.3472*(min + (60*hr)));
}
rect(-480,-480,960,960);
}
Looking Outward 06
A particular work that I find interesting is e4708 by Mark Wilson. To describe the artwork, it looks like a collection of shapes like circles and squares that are repeated many times in neon colors. Some circles are on top of each other, while some circles are on top of squares. The colors make it seem like it’s a lucid dream just about to happen. The fact that I can’t exactly pinpoint what it is inspires me as it really does leave it up to the viewer to interpret the collection of shapes and spaces. I am unsure about the algorithms that the author uses, but he uses it well. Mark Wilson artistic sensibilities manifest in this piece as he is trying to leave it up to a larger audience. He created the piece by purchasing a personal computer and writing his own software. He mixes repetition, careful curation and randomness by the machine to create the piece. In conclusion, I really enjoy looking at this piece and the way it was created.
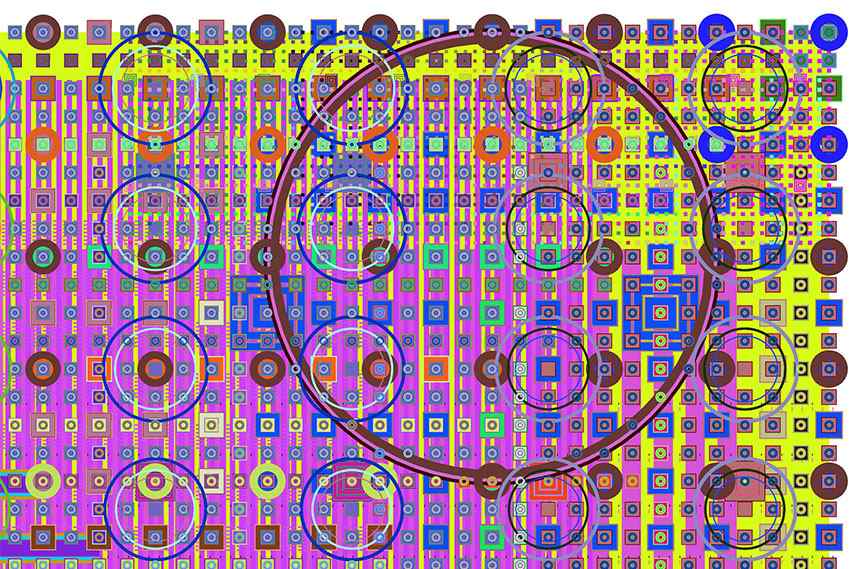