//Yanina Shavialena
//Section B
//My portrait
var image;
//Places I've been/Want to go to
var words = ['Belarus','USA','Bulgaria','Poland','Spain','France','South Korea'];
//The state of mouse pressed
let pressedState = false;
//This function loads the image
function preload() {
var imagelink= "https://i.imgur.com/CQ38vbw.jpg";
image = loadImage(imagelink);
}
//This function created canvas and makes sure the picture is in dimensions of the canvas
function setup() {
createCanvas(480, 480);
image.resize(480, 480);
background(0);
image.loadPixels();
frameRate(10000000);
}
function draw() {
//random x and y positions and get the color from that x and y from my portrait
var x = random(width);
var y = random(height);
var c = image.get(x, y);
if( pressedState == false){
//random start controlling point
var x1 = x + random(-25,25);
var y1 = y + random(-25,25);
//random final point
var x3 = x + random(-15,15);
var y3 = y + random(-15,15);
//random final controlling point
var x4 = x3 + random(-25,25);
var y4 = y3 + random(-25,25);
stroke(color(c));
curve(x1, y1, x, y, x3, y3, x4, y4);
//hearts at the beginning and final point
heart(x,y,5,c);
heart(x3,y3,5,c);
}
//puts words at random points
else{
noStroke();
fill(c);
textSize(random(10));
text(random(words),x,y)
}
}
//when mouse is pressed, mouseState is updated and the background is reset
function mousePressed(){
if(pressedState == false) {
pressedState = true;
}
else {
pressedState = false;
}
background(0);
}
//creates hearts to use for the ends of the lines
function heart(x, y, size, color) {
fill(color);
beginShape();
vertex(x, y);
bezierVertex(x - size / 2, y - size / 2, x - size, y + size / 3, x, y + size);
bezierVertex(x + size, y + size / 3, x + size / 2, y - size / 2, x, y);
endShape(CLOSE);
}
I really enjoyed this project because I could finally implement the idea of pixels and creativity. It was hard to come up with something at first but then I thought about what could describe me in my portrait so I decided to use an emoji of a heart because I think love is one of the strongest feelings on Earth and after the click of a mouse multiple countries would pop up randomly to make up an image: one of the countries is where I was born, one of the countries is where I live right now, then the remaining countries are where I visited or where I want to visit.
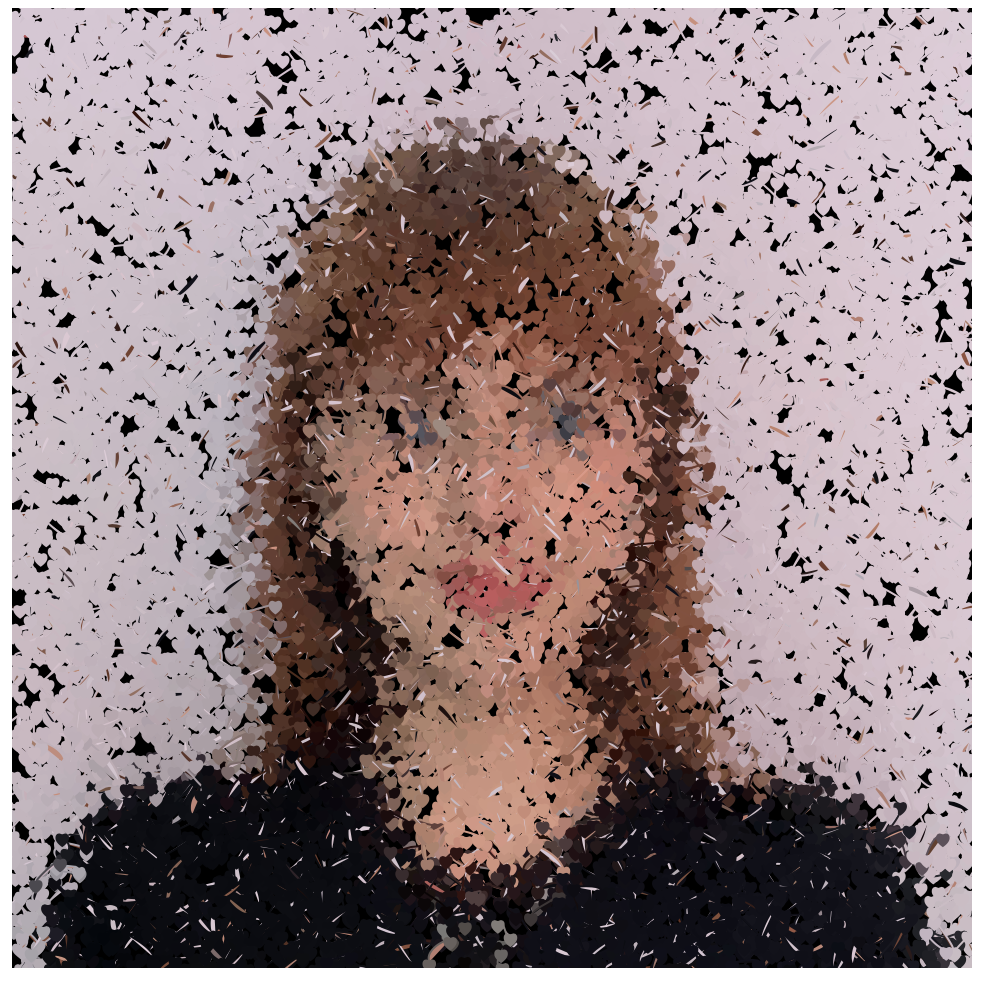
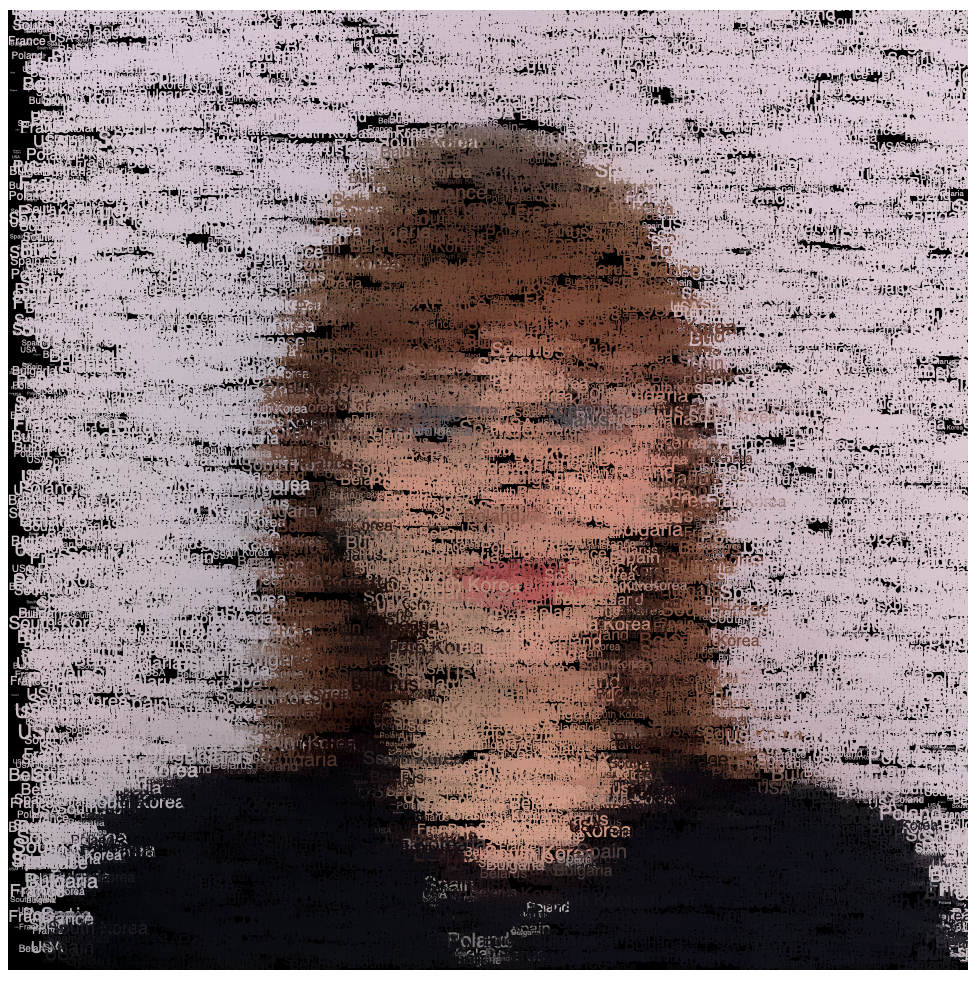
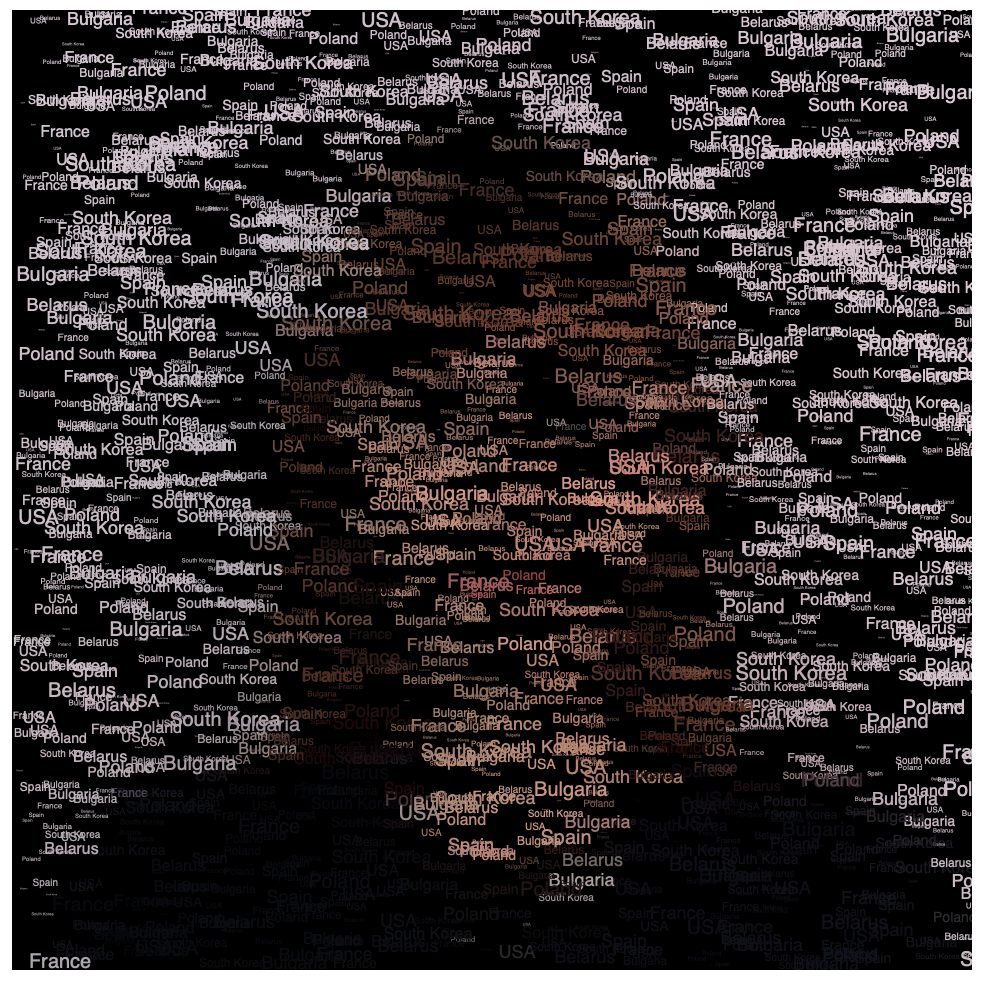