//Scqrlet Tong
//sntong@andrew.cmu.edu
//Section A
// Project 10: Generative Landscape
var sheeps = [];
var sSheeps = [];
function setup() {
createCanvas(480, 480);
// create an initial collection of objects
for (var i = 0; i < 10; i++){
var rx = random(width);
sheeps[i] = makeSheep(rx);
sSheeps[i] = makeSmallSheep(rx);
}
frameRate(10);
}
function draw() {
background(170,215,230);
// changing hillscape
Hill();
// moving sun position
Sun();
// draw existing to new locationlarge sheeps
updateSheeps();
// draw new large sheeps
addNewSheeps();
updateSmallSheeps();
addNewSmallSheeps();
}
function updateSheeps(){
// Update and draw the large sheep positions
for (var i = 0; i < sheeps.length; i++){
sheeps[i].move();
sheeps[i].display();
}
}
function addNewSheeps() {
// With a very tiny probability, add a new building to the end.
var newSheepLikelihood = 0.05;
if (random(0,1) < newSheepLikelihood) {
sheeps.push(makeSheep(width));
}
}
// shift sheeps
function oMove() {
this.x += this.speed;
}
// draw large sheeps
function sheepDisplay() {
push();
translate(this.x+50,height-80+this.scatter);
// legs
stroke(60);
strokeWeight(2);
var loc1 = random (5,7);
line(this.x-10,loc1-10,this.x-10,loc1+12);
line(this.x+10,loc1-10,this.x+10,loc1+12);
//body
strokeWeight(0.5);
fill(255);
stroke(200);
ellipse(this.x, loc1-3, this.fat+20, 20);
fill(150);
//head
ellipse(this.x-18, loc1-6, this.fat-8, 12);
stroke(120);
pop();
}
// object large sheep
function makeSheep(make) {
var sheep = {x: make,
fat: 20,
speed: -1.0,
move: oMove,
scatter: random(5),
display: sheepDisplay}
return sheep;
}
// update location of existing small sheeps
function updateSmallSheeps(){
// Update the small sheep's positions, and draw them.
for (var i = 0; i < sSheeps.length; i++){
sSheeps[i].move();
sSheeps[i].display();
}
}
// generate new small sheeps
function addNewSmallSheeps() {
// add a new small sheep to the end.
var newSheepLikelihood = 0.05;
if (random(0,1) < newSheepLikelihood) {
sSheeps.push(makeSmallSheep(width));
}
}
// draw farer (smaller) sheeps in the field
function smallSheepDisplay() {
push();
translate(this.x+20,height-150+this.scatter);
// legs
stroke(60);
strokeWeight(1);
var loc1 = random (5,6);
line(this.x-5,loc1-5,this.x-5,loc1+6);
line(this.x+5,loc1-5,this.x+5,loc1+6);
//body
strokeWeight(0.5);
fill(255);
stroke(200);
ellipse(this.x, loc1-1.5, this.fat+10, 10);
fill(150);
//head
ellipse(this.x-9, loc1-3, this.fat-4, 6);
stroke(120);
pop();
}
// smalls sheep object
function makeSmallSheep(pop) {
var sSheep = {x: pop,
fat: 10,
speed: -0.5,
move: oMove,
scatter: random(20),
display: smallSheepDisplay}
return sSheep;
}
// function for drawing moving sun
function Sun (){
for (var i = 0; i < width; i++) {
var t = (i * 0.003) + (millis() * 0.0002);
fill(250,200,100);
ellipse(width-t-25,100,50,50);
} noFill();
}
// to creste Landscape
function Hill(){
fill(70,175,100);
noStroke();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * 0.003) + (millis() * 0.00002);
var y = map(noise(t), 0,1, 100, height-100);
vertex(x, y);
vertex(0,height);
vertex(width,height);
}
endShape();
}
I was imagining the view one could get while seating on a train and looking out to the fields in Australia. The larger and smaller sheep suggests their distance to the person. As time passes the sun also moves with the viewer. As I am not familiar with objects, this project is much harder for me as it is working with objects.
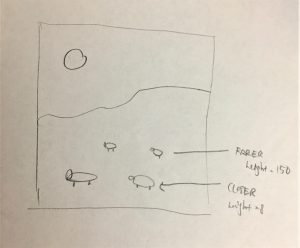