For this project, it took me a while to figure out what I wanted to do. At first I was stuck on the idea of a clock being circular and thought of creating a flower with petals to represent hours, minutes and seconds. However, I thought this wasn’t abstract enough. After pondering for a bit, I came up with the idea of creating a landscape with certain parts that would gradually change over time. At first, I thought of just creating a natural landscape with a sun, river, and mountains, but I felt this wasn’t personalized enough.
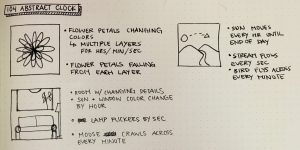
While thinking of what to do, I was siting at my kitchen table staring at the couch across from me and out my window. I finally decided to take inspiration from my own room and create an image of this view of the room. I decided to add a few different fun features to the actual clock.
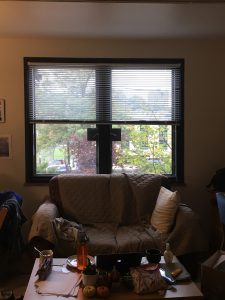
In the abstract clock, the light turns on and off by the second, the sky and sun shift by the hour, and the mouth of the couch gradually opens by the minute.
sketch
function setup() {
createCanvas(480, 400);
}
function draw() {
background(164, 158, 141);
var h = hour();
var windowM = 80; var windowW = 320; var windowH = 240;
if(h > 0 & h < 13){ var skyR = h * (167/12); var skyG = h * (206/12); var skyB = h * (214/12); } else { var skyR = 167 - (h - 12) * (167/12); var skyG = 206 - (h - 12) * (206/12); var skyB = 214 - (h - 12) * (214/12); }
noStroke();
fill(skyR, skyG, skyB);
rect(windowM, 0, windowW, windowH);
strokeWeight(10);
stroke(60, 61, 59);
line(windowM, 0, windowM, windowH); line(windowM + windowW, windowH, windowM + windowW, 0);
var sunDiam = 100; var sunX = width/2;
if(h > 0 & h < 13){ var sunY = (windowH + sunDiam/2) - h * ((windowH + sunDiam)/12);
} else { var sunY = -sunDiam/2 + (h - 12) * ((windowH + sunDiam)/12); }
if(h > 0 & h < 13){ var sunR = h * (211/12); var sunG = h * (130/12); var sunB = h * (115/12); } else { var sunR = 211 - (h - 12) * (211/12); var sunG = 130 - (h - 12) * (130/12); var sunB = 115 - (h - 12) * (115/12); }
noStroke();
fill(sunR, sunG, sunB);
ellipse(sunX, sunY, sunDiam, sunDiam);
var couchR = 228; var couchG = 231; var couchB = 189; var cushionW = 180 - minute() * (140/60); var cushionR = 240 + minute() * (140/60);
strokeWeight(5);
stroke(couchR - 10, couchG - 10, couchB - 10);
fill(couchR, couchG, couchB);
rect(60, windowH - 10, 360, height - windowH + 10, 40);
noStroke();
fill(211, 130, 115);
rect(60, windowH + 65, 360, 75); strokeWeight(30);
stroke(261, 80, 65);
line(120, 345, 360, 345); fill(255);
noStroke();
rect(210, 330, 20, 20); rect(250, 330, 20, 20); ellipse(140, 270, 30, 40); ellipse(340, 270, 30, 40); fill(0);
ellipse(340, 275, 20, 20); ellipse(140, 275, 20, 20);
strokeWeight(5);
stroke(couchR - 10, couchG - 10, couchB - 10);
fill(couchR, couchG, couchB);
rect(60, windowH + 65, cushionW, 75, 20); rect(cushionR, windowH + 65, cushionW, 75, 20); rect(50, 260, 40, 200, 40); rect(width - 90, 260, 40, 200, 40); noStroke();
if(second() % 2 == 0){ fill(242, 239, 199);
rect(440, 60, 50, 100);
} else {
fill(100);
rect(440, 60, 50, 100);
}
fill(60, 61, 59);
rect(470, 160, 20, 400);}