//Jihee Kim
//15-104 MWF 9:30
//jiheek1@andrew.cmu.edu
//Project 10 Landscape
//section D
var stars = []; // an array of all stars
var clouds = []; // an array of all clouds
function setup() {
createCanvas(480, 380);
// initialize stars
for (var s = 0; s < 750; s++){ // loop through stars
var starPositionX = random(width); // the x position of the cloud is
// random along the width of canvas
// the randomness is later set
// in the replaceClouds() function
var starPositionY = random(0, height-120);
stars[s] = makeStars(starPositionX, starPositionY);
}
// initialize clouds
for (var i = 0; i < 5; i++){ // loop through clouds
var cloudPositionX = random(width); // the x position of the cloud is
// random along the width of canvas
// the randomness is later set
// in the replaceClouds() function
var cloudPositionY = random(height/3, height*2/3);
clouds[i] = makeCloud(cloudPositionX, cloudPositionY);
}
}
function draw() {
//WATER
fill(11, 19, 71);
rect(0, height-100, width, 100); // set the very bottom of the canvas as
// water
//gradient background
// draw the gradient background for the pink, night sky
var top = color(198, 96, 113); // pink
var bottom = color(11, 19, 71); // deep blue
Gradient(0, width, top, bottom);
//STARS
animateStars();
hideStars();
addStars();
//MOON
drawMoon();
//CLOUDS (draw the clouds using functions: animate, hide, and add)
animateClouds();
hideClouds();
addClouds();
}
//BACKGROUND
function Gradient (a, b, top, bottom) {
// top to bottom gradient (background)
for (var i = a; i <= height-100; i++) {
var mappedC = map(i, a, a+b, 0, 1);
var c = lerpColor(top, bottom, mappedC);
stroke(c);
strokeWeight(2);
line(a, i, a+b, i);
}
}
//STARS
function drawStars() {
noStroke();
// make stars seem like they're shining by manipulating transparency
var transparency = random(30, 100);
fill(255, transparency);
ellipse(this.x, this.y, 1.2, 1.2);
}
function animateStars() {
for (var s = 0; s < stars.length; s++) {
stars[s].move();
stars[s].draw();
}
}
function hideStars() {
// make the clouds that are actually within the canvas to stay
// if not, hide them
var starsOnCanvas = [];
for (var s = 0; s < stars.length; s++){ // go through all clouds
// if the star is on the canvas
if (0 < stars[s].x < width) {
starsOnCanvas.push(stars[s]); // draw the stars that are on the
// canvas
}
}
stars = starsOnCanvas; // update the stars to the ones that are actually
// on the canvas
}
function addStars() {
var randomValStar = 0.01; // a really small chance of stars randomly
// being added/generated
if (random(0,1) < randomValStar) { // because it is not that likely for a
// randomly chosen value to be less than
// 0.0001, this will be a rare occasion
var starPositionX = random(0, width);
var starPositionY = random(0, height-120);
stars.push(makeStars(starPositionX, starPositionY)); // generate new
// stars along
// the canvas
}
}
function starMove() {
//move stars by updating x coordinate
this.x += this.speed;
}
function makeStars(starPositionX, starPositionY) {
var star = {x: starPositionX,
y: starPositionY,
speed: -random(0, .005),
move: starMove,
draw: drawStars}
return star;
}
//MOON
function drawMoon() {
ellipseMode(CENTER);
noStroke();
fill(252, 202, 202, 80);
ellipse(width/2+100, 150, 80); // overlay another ellipse for slight volume
fill(234, 139, 139, 80);
ellipse(width/2+100, 150, 75); // draw moon
}
// CLOUDS
function animateClouds() {
for (var i = 0; i < clouds.length; i++){ // loop through all clouds
clouds[i].move(); // update the clouds' positions on canvas
clouds[i].display(); // display the clouds
}
}
function hideClouds(){
// make the clouds that are actually within the canvas to stay
// if not, hide them
var cloudsOnCanvas = [];
for (var i = 0; i < clouds.length; i++){ // go through all clouds
if ((clouds[i].x > 0) + (clouds[i].breadth > 0)) { // if the cloud is
// on the canvas
cloudsOnCanvas.push(clouds[i]); // draw the clouds that are on the
// canvas
}
}
clouds = cloudsOnCanvas; // update the clouds to the ones that are actually
// on the canvas
}
function addClouds() {
var randomVal = 0.005; // a really small chance of clouds randomly
// being added/generated
if (random(0,1) < randomVal) { // because it is not that likely for a
// randomly chosen value to be less than
// 0.005, this will be a rare occasion
var cloudPositionX = random(0, width);
var cloudPositionY = random(height/3, height-50);
clouds.push(makeCloud(cloudPositionX, cloudPositionY)); // generate new
// clouds along
// on canvas
}
}
function cloudMove() { // update position of cloud each frame
this.x += this.cloudSpeed;
}
function cloudDisplay() { // draw clouds
var cloudHeightFactor = 7; // factor that determines cloud height
var cloudHeight = this.nFloors * cloudHeightFactor;
ellipseMode(CENTER);
fill(242, 209, 214, this.transparency);
noStroke();
// higher line of clouds (further down on canvas)
push();
translate(this.x, height/2-130);
ellipse(30, -cloudHeight+120, this.breadth, cloudHeight);
pop();
// middle line of clouds (middle on canvas)
push();
translate(this.x, height/2-70);
ellipse(150, -cloudHeight+120, this.breadth, cloudHeight/1.5);
pop();
// lower line of clouds (further down on canvas)
push();
translate(this.x, height/2);
ellipse(110, -cloudHeight+120, this.breadth, cloudHeight);
pop();
}
function makeCloud(cloudPositionX, cloudPositionY) {
var cloud = {x: cloudPositionX, // x position of clouds
y: cloudPositionY, // y position of clouds
transparency: random(10, 80),
breadth: random(120, 250), // width of clouds
cloudSpeed: random(0.07, 0.3), // speed at which clouds move
nFloors: round(random(2,10)),
move: cloudMove,
display: cloudDisplay}
return cloud;
}
For my ever-changing “landscape” I decided to draw a starry night. In order to make the image dynamic, I used components such as the stars and clouds that actually move and generate on it’s own based on a logic that incorporates randomness. I used a color gradient for a more engaging background as well.
I wanted to convey tranquility and I believe that I was able to achieve that goal through employing movements that speak to that.
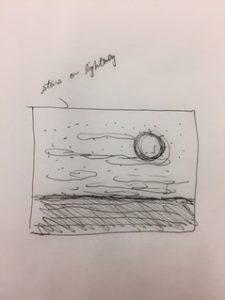