Christine Chen-Project-07-Curves
/*
Christine Chen
Section E
cyc1@andrew.cmu.edu
Project-07-Curves
*/
var nPoints = 100;
var eyeSize = 10;
var pupilSize = 5;
function setup() {
createCanvas(480, 480);
frameRate(10);
}
function draw(){
background(189, 232, 252); //blue
leaves();
push();
translate(width/2, height/2);
drawHeart();
pop();
eye();
blush();
mouth();
}
function drawHeart(){
//color
noStroke();
//peach turns "ripe" when its big
if (mouseY > 130){
fill(255, 178, 182); //light pink
} else {
fill(192, 222, 188); //light green
}
//heart
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = 16 * pow(sin(t), 3);
y = 13 * cos(t) - 5 * cos(2 * t) - 2 * cos(3 * t)
- cos(4 * t) * mouseX/90;
mouseX = constrain(mouseX, 80, 70);
mouseY = constrain(mouseY, 80, 200);
x = map(x, -1, 15, -1, mouseY);
y = map(y, -1, 15, -1, mouseY);
vertex(x, y);
}
endShape(CLOSE);
}
function eye(){
fill(0); //black
var eyeLX = width/2 - 20 - mouseY/10;
var eyeRX = width/2 + 20 + mouseY/10;
ellipse(eyeLX, height/2, eyeSize, eyeSize); //left eye
ellipse(eyeRX, height/2, eyeSize, eyeSize); //right eye
}
function blush(){
noStroke();
fill(229, 51, 60); //red
var blushLX = width/2 - 20 - mouseY/7;
var blushRX = width/2 + 20 + mouseY/7;
ellipse(blushLX, height/2 + 15, eyeSize + 20, eyeSize); //left
ellipse(blushRX, height/2 + 15, eyeSize + 20, eyeSize); //right
}
function mouth(){
fill(232, 91, 98); //dark pink
ellipse(240, 260, mouseY/10, mouseY/10);
}
function leaves(){
noStroke();
fill(112, 230, 134);
//bigger leaves
var leafLX = width/2 - 70;
var leafRX = width/2 + 70;
ellipse(leafLX, height/2 + 100, 150, 100); //left
ellipse(leafRX, height/2 + 100, 150, 100); //right
//smaller shadows on top
fill(107, 221, 129);
ellipse(leafLX + 20, height/2 + 90, 110, 80); //left
ellipse(leafRX - 20, height/2 + 90, 110, 80); //right
}
I was initially just playing with the heart curve. Then when I made it pink, the idea of making it into a peach came to my mind. When the peach is small, it is unripe and so it is green. Once it gets big(once mouseY is larger than a particular point) it becomes ripe(pink). I initially wanted to play around with the curve even more, but I realized that doing so would make the shape not a heart, which wouldn’t work with the idea of a peach. Thus, I just play around with making the curves change “rationally”(so it keeps the shapes of a heart) according to the mouse positions.
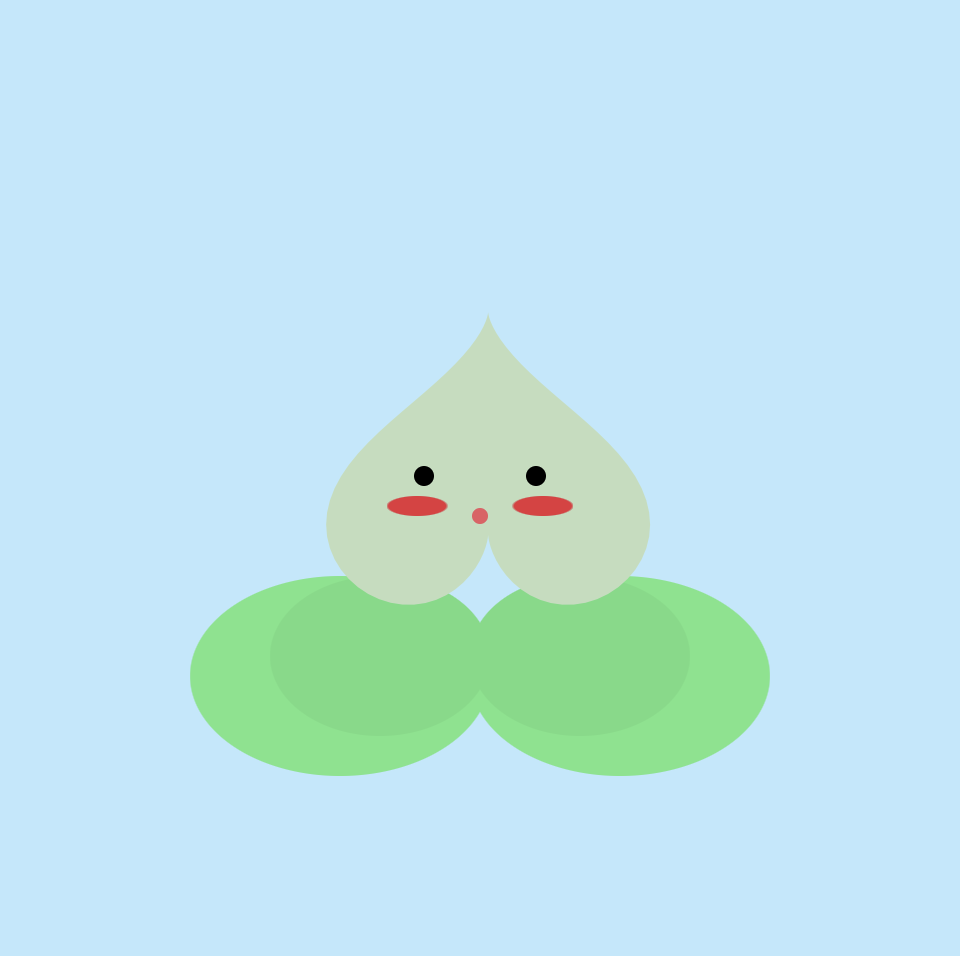
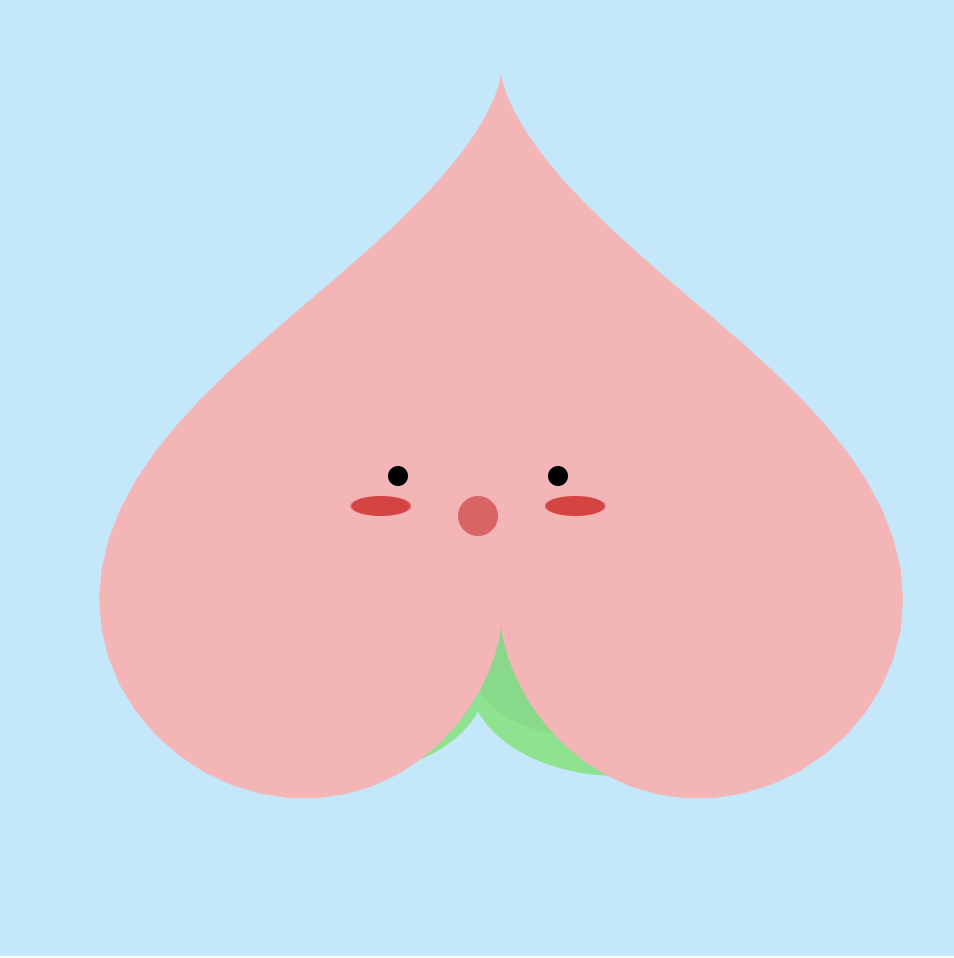