Drawing the pixels of a photo of myself, I set the circles into random sizes. I took out the pixels of the eyes and made them flashing. Basically, it erases the pixels on every other second, and then tries to redraw. The mouse also functions as an eraser by drawing random sizes black circles.
sketch
var original;
function preload() {
var imageLink = "http://i.imgur.com/hfhcgZE.png";
original = loadImage(imageLink);
}
function setup() {
createCanvas(600, 600);
background(0);
original.loadPixels();
}
function draw() {
drawPixel();
eraseEyeL();
eraseEyeR();
drawMouse();
//implied grid
/*line(width/6, 0, width/6, height);
line(width/3, 0, width/3, height);
line(width/2, 0, width/2, height);
line(width/3*2, 0, width/3*2, height);
line(width/6*5, 0, width/6*5, height);
line(0, height/6, width, height/6);
line(0, height/3, width, height/3);
line(0, height/2, width, height/2);
line(0, height/3*2, width, height/3*2);
line(0, height/6*5, width, height/6*5);*/
}
function drawPixel() {
frameRate(120);
var x = random(width);
var y = random(height);
var ix = constrain(floor(x), 0, width-1);
var iy = constrain(floor(y), 0, height-1);
var pixcolor = original.get(ix, iy);
var size = random(10, 30);
fill(pixcolor);
noStroke();
ellipse(x, y, size, size);
}
//erase left eye based on seconds
function eraseEyeL() {
var ex = random(width/3, width/2);
var ey = random(height/2, height/3*2);
var erasecolor = original.get(ex, ey);
if (second() % 2>0){
frameRate(30);
fill(erasecolor);
noStroke();
ellipse(ex, ey, 15, 15);
} else {
//frameRate(300);
fill(10);
noStroke();
ellipse(width/3+50, height/2+50, 120, 120);
print(erasecolor);
}
}
//erase rigt eye based on seconds
function eraseEyeR() {
var ex = random(width/3*2, width/6*5);
var ey = random(height/2, height/3*2);
var erasecolor = original.get(ex, ey);
if (second() % 2>0){
frameRate(20);
fill(erasecolor);
noStroke();
ellipse(ex, ey, 15, 15);
} else {
//frameRate(300);
fill(10);
noStroke();
ellipse(width/3*2+50, height/2+50, 120, 120);
print(erasecolor);
}
}
//erase pixels according to mouse positions
function drawMouse() {
var mx = mouseX;
var my = mouseY;
var msize = random(20,30);
fill(0);
noStroke();
ellipse(mx, my, msize, msize);
}
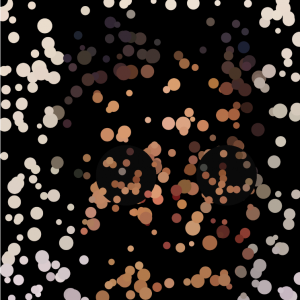
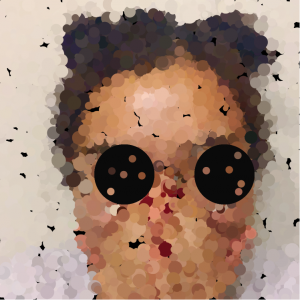