danakim_Project02
//Dana Kim
//danakim@andrew.cmu.edu
//Section D
//Project-02
//Background Colors
var b1= "#E87B3D";
var b2= "#F37C90";
var b3= 265;
var colors= [b1, b2, b3];
//Face colors
var fc1= "#AA723F";
var fc2= "#3A3A3A";
var facecolors= [fc1, fc2];
//eyes
var eyecolor1= "#A91E22";
var eyecolor2= "#2C1A0E";
var eyecolor3= "#FFFFFF";
var eyecolors= [eyecolor1, eyecolor2, eyecolor3];
var eyes= 1;
var eyetype= [1,2];
//ears; iec= interior ear color
var iec1= 0;
var iec2= "#FF8DB0";
var iec3= "#4C330F";
var intearcolors= [iec1, iec2, iec3];
//piercings
var pierce= 1;
var plocation= [1,2];
//Nose
var n1= "#1E1D1D";
var n2= "#70411B";
var nosecolor= [n1, n2];
function setup() {
createCanvas(640, 480);
}
function draw() {
//determines background color
background(b3);
//Head
noStroke();
fill(fc2);
ellipse(315, 232, 278, 232);
beginShape();
curveVertex(401, 208);
curveVertex(439, 225);
curveVertex(458, 246);
curveVertex(465, 267);
curveVertex(466, 283);
curveVertex(464, 304);
curveVertex(457, 318);
curveVertex(446, 334);
curveVertex(440, 343);
curveVertex(433, 352);
curveVertex(423, 369);
curveVertex(418, 381);
curveVertex(410, 402);
curveVertex(397, 419);
curveVertex(377, 432);
curveVertex(359, 438);
curveVertex(327, 443);
curveVertex(311, 442);
curveVertex(288, 441);
curveVertex(264, 435);
curveVertex(241, 423);
curveVertex(223, 401);
curveVertex(217, 386);
curveVertex(211, 371);
curveVertex(196, 347);
curveVertex(180, 329);
curveVertex(172, 317);
curveVertex(167, 295);
curveVertex(167, 277);
curveVertex(180, 244);
curveVertex(207, 223);
endShape(CLOSE);
//Nose
fill(n1);
beginShape();
curveVertex(318, 327);
curveVertex(322, 327);
curveVertex(330, 327);
curveVertex(333, 327);
curveVertex(337, 328);
curveVertex(340, 329);
curveVertex(344, 329);
curveVertex(348, 331);
curveVertex(354, 333);
curveVertex(357, 335);
curveVertex(360, 337);
curveVertex(363, 338);
curveVertex(367, 341);
curveVertex(371, 344);
curveVertex(374, 347);
curveVertex(376, 350);
curveVertex(383, 358);
curveVertex(389, 364);
curveVertex(392, 369);
curveVertex(393, 371);
curveVertex(396, 377);
curveVertex(399, 383);
curveVertex(401, 388);
curveVertex(402, 392);
curveVertex(403, 394);
curveVertex(403, 401);
curveVertex(404, 406);
curveVertex(404, 409);
curveVertex(402, 415);
curveVertex(400, 418);
curveVertex(395, 425);
curveVertex(387, 431);
curveVertex(380, 435);
curveVertex(371, 438);
curveVertex(359, 440);
curveVertex(348, 441);
curveVertex(335, 442);
curveVertex(327, 442);
curveVertex(316, 442);
curveVertex(299, 441);
curveVertex(285, 440);
curveVertex(273, 439);
curveVertex(264, 437);
curveVertex(261, 435);
curveVertex(255, 433);
curveVertex(249, 430);
curveVertex(243, 426);
curveVertex(239, 423);
curveVertex(233, 416);
curveVertex(229, 409);
curveVertex(227, 403);
curveVertex(227, 395);
curveVertex(228, 388);
curveVertex(230, 379);
curveVertex(235, 369);
curveVertex(238, 364);
curveVertex(243, 358);
curveVertex(251, 351);
curveVertex(261, 343);
curveVertex(270, 339);
curveVertex(383, 334);
curveVertex(294, 331);
curveVertex(301, 329);
curveVertex(310, 327);
endShape(CLOSE);
//nostrils
fill(0);
//left nostril
beginShape();
curveVertex(302, 378);
curveVertex(299, 381);
curveVertex(290, 385);
curveVertex(283, 386);
curveVertex(276, 386);
curveVertex(268, 384);
curveVertex(263, 381);
curveVertex(258, 375);
curveVertex(256, 368);
curveVertex(260, 360);
curveVertex(267, 356);
curveVertex(274, 359);
curveVertex(278, 362);
curveVertex(283, 367);
curveVertex(288, 373);
curveVertex(295, 376);
endShape(CLOSE);
//right nostril
beginShape();
curveVertex(336, 378);
curveVertex(337, 377);
curveVertex(340, 377);
curveVertex(343, 376);
curveVertex(346, 375);
curveVertex(348, 374);
curveVertex(350, 373);
curveVertex(352, 371);
curveVertex(354, 369);
curveVertex(356, 366);
curveVertex(357, 364);
curveVertex(361, 361);
curveVertex(364, 358);
curveVertex(366, 357);
curveVertex(367, 356);
curveVertex(369, 356);
curveVertex(371, 356);
curveVertex(374, 357);
curveVertex(375, 358);
curveVertex(377, 359);
curveVertex(378, 361);
curveVertex(379, 362);
curveVertex(381, 365);
curveVertex(381, 366);
curveVertex(381, 370);
curveVertex(381, 373);
curveVertex(380, 375);
curveVertex(378, 377);
curveVertex(376, 380);
curveVertex(374, 381);
curveVertex(372, 383);
curveVertex(370, 384);
curveVertex(366, 384);
curveVertex(362, 386);
curveVertex(358, 386);
curveVertex(354, 386);
curveVertex(349, 384);
curveVertex(345, 384);
curveVertex(342, 383);
curveVertex(339, 381);
endShape(CLOSE);
//eyes
fill(eyecolor1);
//eyes1
if(eyes == 1){
//right eye
ellipse(402, 296, 45, 45);
//left eye
ellipse(227, 297, 45, 45);
//"eyebrows"
stroke(eyecolor1);
strokeWeight(6);
line(374, 283, 432, 264);
strokeWeight(6);
line(256, 284, 198, 266);
}
//eyes2
if(eyes == 2){
//Left eye
beginShape();
curveVertex(261, 299);
curveVertex(254, 297);
curveVertex(247, 297);
curveVertex(237, 301);
curveVertex(229, 302);
curveVertex(222, 300);
curveVertex(217, 296);
curveVertex(214, 290);
curveVertex(213, 283);
curveVertex(215, 277);
curveVertex(219, 271);
curveVertex(224, 267);
curveVertex(231, 265);
curveVertex(237, 264);
curveVertex(243, 266);
curveVertex(249, 270);
curveVertex(254, 276);
curveVertex(259, 286);
curveVertex(261, 295);
endShape(CLOSE);
//right eye
beginShape();
curveVertex(370, 299);
curveVertex(369, 294);
curveVertex(372, 286);
curveVertex(374, 280);
curveVertex(376, 276);
curveVertex(382, 270);
curveVertex(388, 266);
curveVertex(394, 265);
curveVertex(399, 265);
curveVertex(406, 267);
curveVertex(412, 271);
curveVertex(416, 276);
curveVertex(418, 283);
curveVertex(417, 290);
curveVertex(413, 296);
curveVertex(408, 300);
curveVertex(402, 302);
curveVertex(393, 301);
curveVertex(384, 297);
curveVertex(377, 297);
endShape(CLOSE);
}
//horns
fill(265);
stroke(0);
strokeWeight(5);
//right horn
beginShape();
curveVertex(524, 29);
curveVertex(532, 47);
curveVertex(536, 70);
curveVertex(535, 97);
curveVertex(529, 117);
curveVertex(519, 135);
curveVertex(505, 150);
curveVertex(475, 166);
curveVertex(429, 184);
curveVertex(420,185);
curveVertex(412, 182);
curveVertex(397, 169);
curveVertex(384, 144);
curveVertex(384, 130);
curveVertex(391, 124);
curveVertex(434, 125);
curveVertex(464, 122);
curveVertex(490, 115);
curveVertex(506, 106);
curveVertex(513, 98);
curveVertex(517, 91);
curveVertex(522, 67);
curveVertex(522, 41);
endShape(CLOSE);
//left horn
beginShape();
curveVertex(104, 33);
curveVertex(106, 47);
curveVertex(107, 76);
curveVertex(115, 101);
curveVertex(127, 113);
curveVertex(142, 120);
curveVertex(165, 125);
curveVertex(196, 128);
curveVertex(232, 187);
curveVertex(240, 128);
curveVertex(244, 133);
curveVertex(244, 145);
curveVertex(237, 162);
curveVertex(226, 177);
curveVertex(216, 185);
curveVertex(206, 188);
curveVertex(169, 176);
curveVertex(153, 169);
curveVertex(122, 152);
curveVertex(109, 138);
curveVertex(99, 119);
curveVertex(93, 100);
curveVertex(92, 73);
curveVertex(97, 46);
endShape(CLOSE);
//ears
noStroke();
fill(fc2);
beginShape();
//exterior right ear
curveVertex(566, 194);
curveVertex(562, 211);
curveVertex(550, 225);
curveVertex(533, 239);
curveVertex(510, 251);
curveVertex(490, 257);
curveVertex(472, 259);
curveVertex(454, 257);
curveVertex(441, 249);
curveVertex(428, 234);
curveVertex(422, 218);
curveVertex(423, 206);
curveVertex(429, 195);
curveVertex(446, 186);
curveVertex(471, 179);
curveVertex(496, 176);
curveVertex(514, 177);
curveVertex(546, 184);
curveVertex(560, 189);
endShape(CLOSE);
//exterior left ear
beginShape();
curveVertex(67, 202);
curveVertex(75, 216);
curveVertex(88, 229);
curveVertex(101, 239);
curveVertex(114, 247);
curveVertex(140, 256);
curveVertex(162, 259);
curveVertex(176, 258);
curveVertex(193, 249);
curveVertex(205, 236);
curveVertex(212, 218);
curveVertex(211, 206);
curveVertex(206, 198);
curveVertex(195, 189);
curveVertex(162, 179);
curveVertex(143, 176);
curveVertex(126, 176);
curveVertex(88, 184);
curveVertex(75, 188);
curveVertex(67, 194);
endShape(CLOSE);
//interior right ear
fill(iec1);
beginShape();
curveVertex(457, 249);
curveVertex(453, 235);
curveVertex(456, 220);
curveVertex(465, 209);
curveVertex(473, 204);
curveVertex(487, 200);
curveVertex(510, 199);
curveVertex(527, 198);
curveVertex(546, 195);
curveVertex(562, 194);
curveVertex(567, 196);
curveVertex(565, 206);
curveVertex(562, 211);
curveVertex(555, 221);
curveVertex(530, 241);
curveVertex(508, 251);
curveVertex(490, 257);
curveVertex(476, 260);
curveVertex(466, 259);
endShape(CLOSE);
//interior left ear
beginShape();
curveVertex(66, 196);
curveVertex(72, 194);
curveVertex(89, 196);
curveVertex(123, 199);
curveVertex(146, 200);
curveVertex(152, 201);
curveVertex(162, 205);
curveVertex(171, 212);
curveVertex(177, 220);
curveVertex(179, 228);
curveVertex(180, 236);
curveVertex(178, 245);
curveVertex(173, 254);
curveVertex(167, 259);
curveVertex(159, 260);
curveVertex(144, 257);
curveVertex(115, 247);
curveVertex(94, 235);
curveVertex(79, 221);
curveVertex(71, 211);
curveVertex(66, 202);
endShape(CLOSE);
//piercings
fill(239, 202, 44);
//piercing1
if(pierce == 1){
strokeWeight(6);
strokeCap(SQUARE);
stroke(239, 202, 44);
noFill();
arc(317, 403, 69, 69, QUARTER_PI, PI+QUARTER_PI);
}
//piercing2
if(pierce == 2){
triangle(531, 216, 511, 250, 551, 250);
ellipse(531, 216, 20, 20);
}
}
function mousePressed() {
// when the user clicks the mouse, these variables are reassigned
// to preset values at random.
b3= random(colors);
fc2= random (facecolors);
eyecolor1= random(eyecolors);
eyes= random(eyetype);
n1= random(nosecolor);
iec1= random(intearcolors);
pierce= random(plocation);
}
The process took a bit of time because I used curveTexture() to create a lot of the shapes I needed. It was a very inefficient process and I’m sure that there is a more efficient and concise way that I could have executed the script. Overall, the project itself was really fun. I wasn’t able to execute all the features I wanted to put into it but I’m still pleased with the overall outcome. Below are the reference drawings that I had made before starting the script.
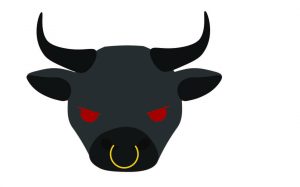
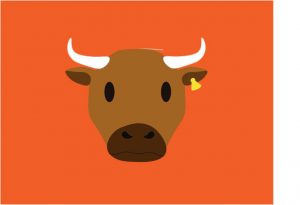