When I saw the project, I was reminded of the 2013 Great Gatsby movie poster, and I wanted to make a version of it using the string art. I thought this project was going to be a huge difficulty for me, but after really looking at it and asking tutors, I got a better grasp of the assignment and was able to make better guesses at figuring coordinates out. I tried to use the x1,y1/x2, y2 concept listed in the instructions as well as the x1 += x1StepSize, because I figured it was probably the simplest way to help generate the “curves,” so I could focus more on creating a better design.
sketch2
//Natalie Schmidt
//nkschmid@andrew.cmu.edu
//Sectiond D
//Project-04-StringArt
var height = 300;
var width = 400;
//coordinates for light gold loops
var x1 = 0;
var y1 = 0;
var x1StepSize = width/20;
var y1StepSize = height/20;
//coordinates for dark gold loops
var x2 = 0;
var y2 = 0;
var x2StepSize = width/20;
var y2StepSize = height/20;
//to change from gold to silver
var colorR1 = 219;
var colorG1 = 180;
var colorB1 = 117;
var colorR2 = 183;
var colorG2 = 129;
var colorB2 = 59;
function setup() {
createCanvas(400, 300);
background(0);
fill(255);
//display text
textSize(15);
text("The Great", 168, 135);
textSize(50);
text("GATSBY", 105, 180);
}
function draw() {
stroke(colorR2, colorG2, colorB2);
//change the colors from gold to silver
if (mouseX <= height/2) {
colorR2 = 183;
colorG2 = 129;
colorB2 = 59;
}
else {
colorR2 = 109;
colorG2 = 111;
colorB2 = 106;
}
strokeWeight(10);
//how far apart each line is from each other
var spacing = 20;
//lines down left
for (var x = 65; x <= 115; x += spacing) {
line(x, 0, x, height/2.3);
}
//lines down right
for (var x3 = 300; x3 <= 350; x3 += spacing) {
line(x3, 0, x3, height/2.3);
}
//lines across left
line(0, height/2.3, width/4, height/2.3);
line(0, height/2.6, width/4, height/2.6);
line(0, height/3, width/4, height/3);
//lines across right
line(width - width/4.2, height/2.3, width, height/2.3);
line(width - width/4.2, height/2.6, width, height/2.6);
line(width - width/4.2, height/3, width, height/3);
//lines going down left
for (var x4 = 45; x4 <= 102; x4 += spacing) {
line(x4, height/2.3, x4, height);
}
//lines going down right
for (var x5 = 320; x5 <= 360; x5 += spacing) {
line(x5, height/2.3, x5, height);
}
//light gold set of "curves"/loops
for (var i = 0; i <= 400; i++) {
strokeWeight(1);
stroke(colorR1, colorG1, colorB1);
line(0, y1, x1, height);
line(0, height - y1, x1, 0);
line(width, y1, width - x1, height);
line(width, height - y1, width - x1, 0);
x1 += x1StepSize;
y1 += y1StepSize;
}
//dark gold set of "curves"/loops
for (var j = 0; j <= 400; j++) {
strokeWeight(1);
stroke(colorR2, colorG2, colorB2);
line(0, y2, x2, height);
line(0, height - y2, x2, 0);
line(width, y2, width - x2, height);
line(width, height - y2, width - x2, 0);
x2 += 1.3*x2StepSize;
y2 += 1.5*y2StepSize;
}
//top triangle
for (var k = 0; k < 5; k++) {
stroke(colorR1, colorG1, colorB1);
line(width/2, 110, k * 20, 50);
line(width/2, 110, 60 + k * 20 , 50);
line(width/2, 110, 120 + k * 20, 50);
line(width/2, 110, 180 + k * 20, 50);
line(width/2, 110, 240 + k * 20, 50);
line(width/2, 110, 300 + k * 20, 50);
}
//bottom triangle
for (var l = 0; l < 5; l++) {
stroke(colorR1, colorG1, colorB1);
line(width/2, height - 110, l * 20, height - 50);
line(width/2, height - 110, 60 + l * 20 , height - 50);
line(width/2, height - 110, 120 + l * 20, height - 50);
line(width/2, height - 110, 180 + l * 20, height - 50);
line(width/2, height - 110, 240 + l * 20, height - 50);
line(width/2, height - 110, 300 + l * 20, height - 50);
}
if (mouseX <= width/2) {
colorR1 = 219;
colorG1 = 170;
colorB1 = 91;
}
else {
colorR1 = 180;
colorG1 = 177;
colorB1 = 172;
}
}
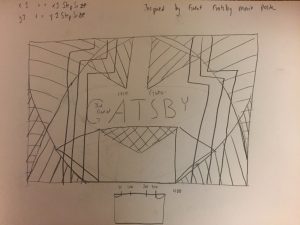
A really rough sketch of my initial idea. It ended up changing, because I felt like it originally looked too busy. I did have help from the CS pedagogy tutors in trying to figure out how to approach this assignment.