var img;
var gScale = 0.14; // Scale the image size down
var size = 5; // Size of all the circles and squares
function preload() {
var portrait = "https://i.imgur.com/xb4QYap.jpg";
img = loadImage(portrait);
}
function setup() {
createCanvas(img.width * gScale, img.height * gScale);
background(255);
frameRate(60);
img.loadPixels();
}
function draw() {
//Draws small circles and squares. The circles are tinted red and the squares are blue
var cx = random(img.width);
var cy = random(img.height);
var dotColor = img.get(cx, cy);
var db = brightness(dotColor); // Gets the grayscale value of the pixel
noStroke();
fill(db + 150, db + 100, db + 100); // Turns all of the circles a red/brown
circle(cx * gScale, cy * gScale, size);
var sx = random(img.width);
var sy = random(img.height);
var squareColor = img.get(sx, sy);
var sb = brightness(squareColor);
fill(sb + 100, sb + 100, sb + 150); // Turns the squares blue
square(sx * gScale, sy * gScale, size);
var gx = random((mouseX - 15) / gScale, (mouseX + 15) / gScale); // creates a circle within 30 pixels of the mouse
var gy = random((mouseY - 15) / gScale, (mouseY + 15) / gScale);
greenDotColor = img.get(gx, gy);
var gb = brightness(greenDotColor);
fill(gb + 100, gb + 150, gb + 100); // Turns these circles green
circle(gx * gScale, gy * gScale, size);
}
For this project I used a quick picture I took of myself as the base. I played around a bit with what I could do with the colors. Using the brightness feature I basically turned the picture black and white, then added in contrasting colors. Red circles and blue squares will randomly appear to start filling in the picture. Green circles will begin to fill in the picture in an area close to your mouse.
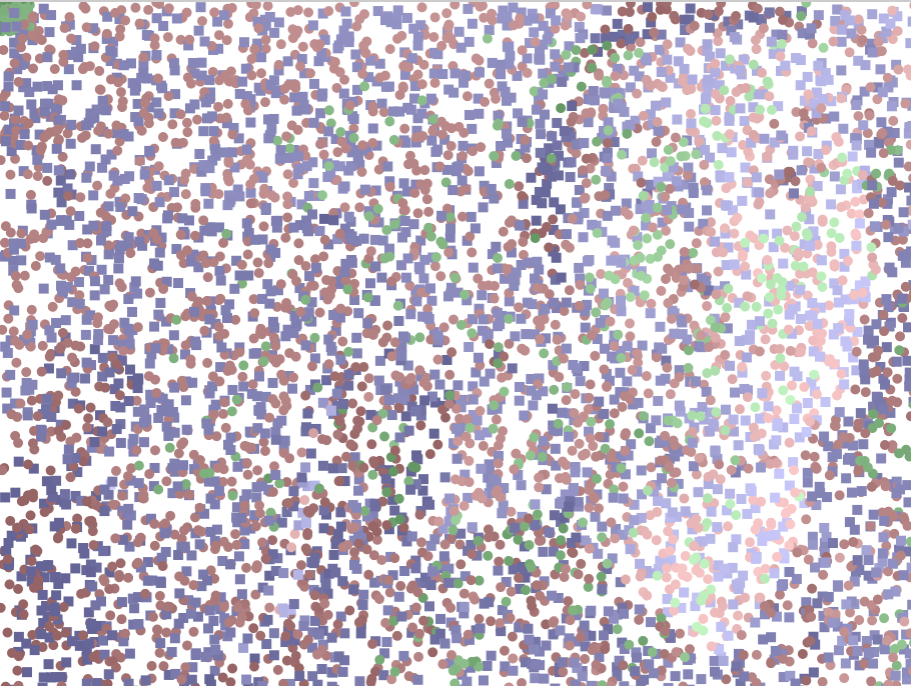
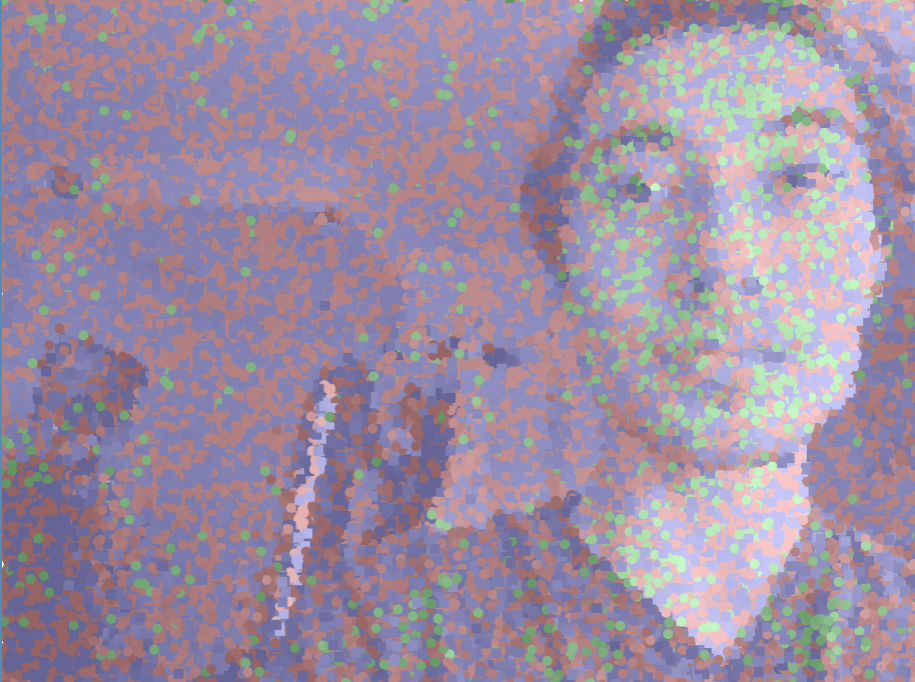
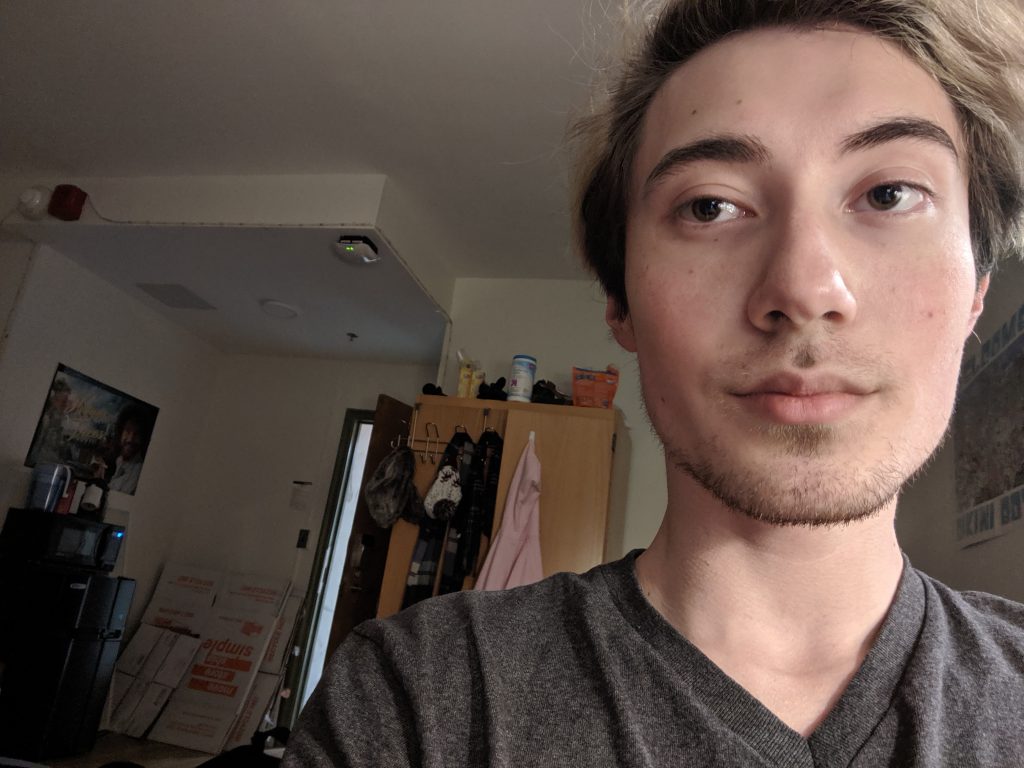