sketch
//Ryu Kondrup
//rkondrup@andrew.cmu.edu
//Section D
//project-03
var cloudShiftX = 200;
var cloudShiftY = 100;
var cloudShift0X = -150;
var cloudShift0Y = 70;
var adjustShift = 100;
//greyWhite
var greyWhiteR = 245;
var greyWhiteG = 241;
var greyWhiteB = 230;
//windows colors
var windowsR = 160;
var windowsG = 160;
var windowsB = 150;
//lights colors
var lightsR = 255;
var lightsG = 197;
var lightsB = 107;
//grey sky colors
var greySkyR = 226;
var greySkyG = 226;
var greySkyB = 214;
//sky colors
var skyR = 182;
var skyG = 222;
var skyB = 228;
//water color
var waterR = 162;
var waterG = 222;
var waterB = 208;
//grey water
var greyWaterR = 160;
var greyWaterG = 160;
var greyWaterB = 150;
function setup() {
createCanvas(640, 480);
}
function draw() {
//colors!!!!!!!!!
background(greySkyR, greySkyG, greySkyB);
noStroke();
//to change background color
if ((mouseX >= 640) & (mouseX <= 640)){
mouseX = 640;
}
if ((mouseX > 0) & (mouseX < 640)){
greySkyR = mouseX*((182-226)/640) + 226;
greySkyG = mouseX*((222-226)/640) + 226;
greySkyB = mouseX*((228-214)/640) + 214;
}
//to change water color
if ((mouseX >= 640) & (mouseX <= 640)){
mouseX = 640;
}
/* if ((mouseX >= 640) & (mouseX <= 640)){
mouseX = 640;
}
if ((mouseX > 0) & (mouseX , 640)){
}
*/
//to turn on the lights
if ((mouseIsPressed) && (windowsR = 160)) {
windowsR = lightsR;
windowsG = lightsG;
windowsB = lightsB;
}
else if ((mouseIsPressed) & (windowsR = 225)) {
windowsR = 160;
windowsG = 160;
windowsB = 150;
//NEEDS SOME WORK
}
//CLOUD
//cloud1
fill(greyWhiteR, greyWhiteG, greyWhiteB);
ellipse(mouseX + 0, 125, 20);
ellipse(mouseX + 20, 120, 35);
ellipse(mouseX + 40, 120, 55);
ellipse(mouseX + 65, 125, 40);
ellipse(mouseX + 90, 120, 45);
ellipse(mouseX + 115, 125, 20);
ellipse(mouseX + 125, 120, 30);
ellipse(mouseX + 145, 125, 20);
//cloud2
ellipse(mouseX + 0 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
ellipse(mouseX + 20 + cloudShiftX + adjustShift, 100 + cloudShiftY, 35);
ellipse(mouseX + 40 + cloudShiftX + adjustShift, 100 + cloudShiftY, 55);
ellipse(mouseX + 65 + cloudShiftX + adjustShift, 105 + cloudShiftY, 40);
ellipse(mouseX + 90 + cloudShiftX + adjustShift, 100 + cloudShiftY, 45);
ellipse(mouseX + 115 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
ellipse(mouseX + 125 + cloudShiftX + adjustShift, 100 + cloudShiftY, 30);
ellipse(mouseX + 145 + cloudShiftX + adjustShift, 105 + cloudShiftY, 20);
//cloud0
ellipse(mouseX + 0 + cloudShift0X, 105 + cloudShift0Y, 20);
ellipse(mouseX + 20 + cloudShift0X, 100 + cloudShift0Y, 35);
ellipse(mouseX + 40 + cloudShift0X, 100 + cloudShift0Y, 55);
ellipse(mouseX + 65 + cloudShift0X, 105 + cloudShift0Y, 40);
ellipse(mouseX + 90 + cloudShift0X, 100 + cloudShift0Y, 45);
ellipse(mouseX + 115 + cloudShift0X, 105 + cloudShift0Y, 20);
ellipse(mouseX + 125 + cloudShift0X, 100 + cloudShift0Y, 30);
ellipse(mouseX + 145 + cloudShift0X, 105 + cloudShift0Y, 20);
//cloud-1
ellipse(mouseX + 0 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 20 + 3*cloudShift0X, 100 + .5*cloudShiftY, 35);
ellipse(mouseX + 40 + 3*cloudShift0X, 100 + .5*cloudShiftY, 55);
ellipse(mouseX + 65 + 3*cloudShift0X, 105 + .5*cloudShiftY, 40);
ellipse(mouseX + 90 + 3*cloudShift0X, 100 + .5*cloudShiftY, 45);
ellipse(mouseX + 115 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 125 + 3*cloudShift0X, 100 + .5*cloudShiftY, 30);
ellipse(mouseX + 145 + 3*cloudShift0X, 105 + .5*cloudShiftY, 20);
//cloud3
ellipse(mouseX + 0 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 20 + 5*cloudShift0X, 100 + .5*cloudShiftY, 35);
ellipse(mouseX + 40 + 5*cloudShift0X, 100 + .5*cloudShiftY, 55);
ellipse(mouseX + 65 + 5*cloudShift0X, 105 + .5*cloudShiftY, 40);
ellipse(mouseX + 90 + 5*cloudShift0X, 100 + .5*cloudShiftY, 45);
ellipse(mouseX + 115 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
ellipse(mouseX + 125 + 5*cloudShift0X, 100 + .5*cloudShiftY, 30);
ellipse(mouseX + 145 + 5*cloudShift0X, 105 + .5*cloudShiftY, 20);
/*
greyWhite = color(245, 241, 230);
greySky = color(200, 200, 185);
greyWater = color(160, 160, 150);
//windows = color(160, 160, 150);
windows = color(window1R, window1G, window1B);
sky = color(124, 35, 60);
//lights = color(225, 193, 96);
lights = color(lightsR, window2G, window2B);
water = color(81, 18, 35);
*/
fill(greyWhiteR, greyWhiteG, greyWhiteB);
//BASE PIECES
//base plate
rect(40, 322, 562, 10);
//2nd base plate
rect(104, 310, 433, 15);
//2nd base plate right triangle
triangle(537, 310, 537, 325, 572, 325);
//3rd base plate
rect(109, 299, 320, 15);
//3rd base plate right triangle
triangle(429, 299, 429, 310, 469, 310);
//4th base plate
rect(115, 283, 75, 18);
//4th base plate triangle
triangle(190, 283, 190, 301, 223, 301);
//opera house FILL HOLES
//left rectangle
rect(169, 234, 120, 80);
//middle rectangle
rect(290, 255, 100, 60);
//right rectangle
rect(390, 276, 130, 40);
//CURVES
//curve 1
bezier(104, 220, 123, 223, 173, 227, 200, 234);
triangle(95, 219, 200, 234, 190, 283);
//curve 1 small
triangle(95, 219, 121, 237, 121, 258);
//curve 2
triangle(147, 190, 255, 228, 223, 299);
bezier(147, 190, 185, 201, 227, 217, 255, 228);
//litle triangle addition
triangle(147, 190, 162, 229, 185, 232);
//curve 3 the big one
//subcurve 3 big
triangle(335, 255, 229, 146, 287, 299);
bezier(229, 146, 277, 178, 315, 217, 335, 255);
//subcurve 3 small
triangle(237, 222, 229, 146, 287, 299);
//curve 4
triangle(335, 255, 460, 240, 407, 299);
bezier(335, 255, 375, 243, 425, 239, 460, 240);
//curve 5
triangle(395, 253, 472, 276, 429, 299);
bezier(395, 253, 424, 258, 451, 267, 472, 276);
//curve 6
triangle(472, 276, 550, 269, 512, 310);
bezier(472, 276, 498, 270, 525, 268, 550, 269);
//WINDOWS
fill(windowsR, windowsG, windowsB);
//window 1
triangle(121, 237, 190, 283, 121, 283);
//window 2
bezier(190, 283, 192, 270, 208, 270, 223, 299);
//window 3
bezier(223, 299, 246, 279, 269, 281, 287, 299);
//window 4
bezier(287, 299, 307, 281, 335, 281, 347, 299);
//winodw 5
bezier(347, 299, 362, 279, 384, 282, 407, 299);
//window 6
triangle(408, 271, 408, 299, 429, 299);
//window 7
bezier(429, 299, 447, 291, 461, 291, 469, 310);
//window 8
bezier(469, 310, 480, 294, 496, 294, 512, 310);
//winodw 9 (end)
triangle(512, 310, 537, 310, 537, 283);
fill(greyWaterR, greyWaterG, greyWaterB);
//WATER BELOW
rect(0, 332, 640, 480);
if ((mouseX > 0) & (mouseX < 640)){
greyWaterR = mouseX*((142-160)/640) + 160;
greyWaterG = mouseX*((222-160)/640) + 160;
greyWaterB = mouseX*((208-150)/640) + 150;
}
var offsetX1 = 100
var offsetY1 = 15
//waterlines
fill(greyWhiteR, greyWhiteG, greyWhiteB);
//row1
rect(640-mouseX, 345, 220, 3);
rect(640-mouseX + 280, 345, 160, 3);
rect(640-mouseX + 500, 345, 240, 3);
rect(640-mouseX - 300, 345, 200, 3);
rect(640-mouseX - 600, 345, 220, 3);
//row2
rect(640-mouseX + offsetX1, 345 + offsetY1, 220, 3);
rect(640-mouseX + 280 + offsetX1, 345 + offsetY1, 160, 3);
rect(640-mouseX + 500 + offsetX1, 345 + offsetY1, 240, 3);
rect(640-mouseX - 300 + offsetX1, 345 + offsetY1, 200, 3);
rect(640-mouseX - 600 + offsetX1, 345 + offsetY1, 220, 3);
//row3
rect(640-mouseX + 2*offsetX1, 345 + 2.5*offsetY1, 220, 3);
rect(640-mouseX + 280 + 2*offsetX1, 345 + 2.5*offsetY1, 160, 3);
rect(640-mouseX + 500 + 2*offsetX1, 345 + 2.5*offsetY1, 240, 3);
rect(640-mouseX - 300 + 2*offsetX1, 345 + 2.5*offsetY1, 200, 3);
rect(640-mouseX - 600 + 2*offsetX1, 345 + 2.5*offsetY1, 220, 3);
//row4
rect(640-mouseX + 3*offsetX1, 345 + 5*offsetY1, 220, 3);
rect(640-mouseX + 280 + 3*offsetX1, 345 + 5*offsetY1, 160, 3);
rect(640-mouseX + 500 + 3*offsetX1, 345 + 5*offsetY1, 240, 3);
rect(640-mouseX - 300 + 3*offsetX1, 345 + 5*offsetY1, 200, 3);
rect(640-mouseX - 600 + 3*offsetX1, 345 + 5*offsetY1, 220, 3);
/*var windows1R = 160;
var window1G = 160;
var window1B = 150;
var lightsR = 225;
var window2G = 193;
var window2B = 96; */
//colors!!!!!!!!!
/* greyWhite = color(245, 241, 230);
greySky = color(200, 200, 185);
greyWater = color(160, 160, 150);
windows = color(160, 160, 150);
sky = color(124, 35, 60);
lights = color(225, 193, 96);
water = color(81, 18, 35);
*/
}
/* //windows colors
var windowsR = 160;
var windowsG = 160;
var windowsB = 150;
//lights colors
var lightsR = 255;
var lightsG = 197;
var lightsB = 107;
function mouseIsPressed (){
if ((windowsR = 160)){
windowsR = 255;
windowsG = 197;
windowsB = 107;
}
else if (true) {
windowsR = 160;
windowsG = 160;
windowsB = 150;
}
}*/
I was feeling very ambitious at the start of this project, but after two days of coding simple shapes without yet having added any interactivity, i realized i needed to simplify the design if i was to finish before the deadline. I wanted to originally make the drawing change from soft day-colored gradients to vivid night-colored gradients but time was running short and i settled on a grey-to-saturated fill instead, which i am relatively happy with. Unfortunately, WordPress was not kind to my aspect ratio which i so meticulously planned out in a 640 x 480 arrangement but which was unceremoniously snipped by the WordPress formatting gods. In the future i will try to orient my canvases vertically to avoid cropping. 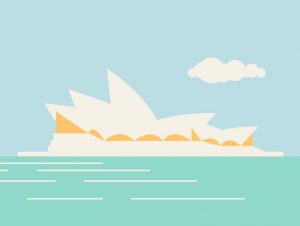