//Selina Lee
//Section C
//selinal@andrew.cmu.edu
//Project-06
var prevSec;
var millisRolloverTime;
var centX = 300; //center coordinates
var centY = 300;
var numcirc = 12; // number of circles to draw
var distanceS = 250; //distance away from center with second pi clock
var distanceM = 225; //distance away from center with minute pi clock
var distanceH = 175; //distance away from center with hour pi clock
var angle = 0; //start angle for circle rotation
function setup() {
createCanvas(600, 600);
millisRolloverTime = 0;
}
function draw() {
background(170, 170, 255);
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
// Reckon the current millisecond,
// particularly if the second has rolled over.
// Note that this is more correct than using millis()%1000;
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
var hourArcAngle = map(H, 0, 23, 0, 360); //angles away from 0 or 12 o'clock
var minuteArcAngle = map(M, 0, 59, 0, 360);
// Make a bar which *smoothly* interpolates across 1 minute.
// We calculate a version that goes from 0...60,
// but with a fractional remainder:
var secondsWithFraction = S + (mils / 1000.0);
var secondsWithNoFraction = S;
//var secondBarWidthChunky = map(secondsWithNoFraction, 0, 60, 0, width);
var secondArcAngleSmooth = map(secondsWithFraction, 0, 60, 0, width);
var strike = radians(270); //so that arcs start at 12:00 placement
var alphaincS = map(S, 0, 59, 0, 155); //increase transparency from full circle
var alphaincM = map(M, 0, 59, 0, 105);
var alphaincH = map(H, 0, 23, 0, 105);
noStroke();
fill(255, 230, 130, 100 + alphaincS);
arc(centX, centY, 500, 500, strike, radians(secondArcAngleSmooth) + strike); //yelllow outside circle for seconds
fill(255, 180, 130, 150 + alphaincM);
arc(centX, centY, 450, 450, strike, radians(minuteArcAngle) + strike); //orange circle for minutes
fill(255, 150, 130, 150 + alphaincH);
arc(centX, centY, 350, 350, strike, radians(hourArcAngle) + strike); //red circle for hours by 24
var angleObjectS = 360/numcirc; //divides circles evenly on outside
for (var i = 0; i < numcirc; i++) //for loops for making circles revolve around center point
{
var posX = centX + distanceS *cos( radians(angleObjectS*i + angle) );
var posY = centY + distanceS *sin( radians(angleObjectS*i + angle) );
fill(255, 0, 0, 50);
ellipse(posX, posY, 30, 30);
}
var angleObjectM = 360/numcirc;
for (var i = 0; i < numcirc; i++)
{
var posX = centX + distanceM *cos( radians(angleObjectM*i + angle) );
var posY = centY + distanceM *sin( radians(angleObjectM*i + angle) );
fill(255, 150, 0, 50);
ellipse(posX, posY, 30, 30);
}
var angleObjectH = 360/numcirc;
for (var i = 0; i < numcirc; i++)
{
var posX = centX + distanceH *cos( radians(angleObjectH*i + angle) );
var posY = centY + distanceH *sin( radians(angleObjectH*i + angle) );
fill(255, 255, 0, 50);
ellipse(posX, posY, 30, 30);
}
}
Category: Project-06-Abstract-Clock
Project 06 – Yugyeong Lee
//Yugyeong Lee
//Section B
//yugyeonl@andrew.cmu.edu
//Project 06
function setup() {
createCanvas(200, 480);
}
function draw() {
//gradient background
var from = color(127, 188, 215);
var to = color(255);
setGradient(0, height, from, to);
//variables for time
var h = hour();
var m = minute();
var s = second();
//mapping ground color based on hour
noStroke();
r = map(h, 0, 24, 190, 0);
g = map(h, 0, 24, 255, 80);
b = map(h, 0, 24, 180, 30);
fill(r, g, b);
rect(0, height-30, width, 30);
//mapping positionY based on minute
var positionY = map(m, 0, 60, 416, 156);
upHouse(positionY);
//balloons increase and move based on second
for (var i = 0; i < s; i++) {
frameRate(1); //frame changes once per second
strokeWeight(.25);
balloonX = random(85, 135);
balloonY = random(65, 120);
line(105, positionY-37, balloonX, positionY-balloonY);
fill(random(249, 255), random(140, 255), random(150, 200));
ellipse(balloonX, positionY-balloonY, 11, 14);
}
}
function setGradient (y, h, from, to) {
// top to bottom gradient
for (var i = y; i <= y+h; i++) {
var inter = map(i, y, y+h, 0, 1);
var c = lerpColor(from, to, inter);
stroke(c);
strokeWeight(2);
line(y, i, y+h, i);
}
}
function upHouse (y) {
//function to create the house
noStroke();
//base
fill(245, 202, 170);
rect(73, y, 62, 38);
fill(126, 176, 200);
rect(73, y, 62, 10);
//roof
fill(51);
quad(74, y-28, 136, y-28, 144, y, 66, y);
//chimney
fill(88, 67, 50);
rect(102, y-36, 6, 10);
//door
fill(78, 60, 45);
rect(95, y+5, 9, 25);
//porch&stair
fill(99, 71, 59);
rect(72, y+27, 18, 11);
for (var i = 0; i < 4; i++) {
fill(255);
stroke(150);
strokeWeight(0.25);
rect(90, y+27+2.75*i, 18.5, 2.75);
}
noStroke();
fill(255);
rect(74, y, 2, 27);
rect(88, y, 2, 27);
rect(76, y+13, 12, 2);
rect(79, y+15, 2, 12);
rect(83, y+15, 2, 12);
//other components of house
fill(187, 202, 94);
rect(108, y, 28, 38);
fill(245, 248, 162);
triangle(104, y+5, 140, y+5, 122, y-38);
rect(84, y-20, 12, 10);
triangle(82, y-18, 98, y-18, 90, y-30);
//outline
stroke(141, 202, 233);
strokeWeight(3.5);
line(140, y, 122, y-38);
line(122, y-38, 104, y);
line(98, y-18, 90, y-30);
line(90, y-30, 82, y-18);
//windows
fill(240, 208, 100);
stroke(265);
strokeWeight(1);
rect(86, y-20, 6, 7);
rect(116, y-14, 10, 10);
rect(115, y+14, 12, 14);
}
*sketch updated to modified code based on question on Piazza
I was inspired by the movie “UP” to create this abstract clock design. The number of balloons increases every second while moving around to random points to illustrate the balloons floating in the air. The number of balloons goes back to 0 when it hits the next minute. The color of the balloons are also randomized within a range to have variety of shades. Each minute is indicated by the house’s Y position as the house moves up. The ground color slowly changes every hour to indicate the current time. A separate function had to be created to generate the house, which uses variable y that is mapped to minute().
cduong-project-06-abstract clock
//Name: Colleen Duong
//Class: Section D
//Email: cduong@andrew.cmu.edu
//Project-06-Abstract Clock
//all of the numbers for the x-coordinates of the falling snow
var snowfallx = [400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350, 250, 150, 50,
400, 300, 200, 100,
450, 350];
//All of the numbers for the y-coordinates of the falling snow
var snowfally = [15, 30, 20, 40, 35,
15, 25, 30, 25,
30, 20, 30, 20, 30,
70, 50, 60, 90,
130, 100, 120, 150, 160,
150, 160, 130, 170,
190, 170, 190, 180, 180,
200, 210, 230, 240,
210, 240, 260, 210, 220,
290, 300, 310, 270,
330, 340, 350, 320, 320,
350, 360, 340, 340,
360, 400, 430, 420, 400]
var snowd = 5; //snow diameter
function setup() {
createCanvas(480, 480);
}
function draw() {
background(240, 235, 231);
var H = hour();
var M = minute();
var S = second();
//Design Code --> The Mountains
noStroke();
//Mountain 5 (Lightest Blue)
fill(160, 201, 218);
triangle(50, 500, 400, 500, 230, 180);
triangle(-50, 500, 300, 500, 110, 100);
triangle(-50, 500, 150, 500, 50, 130);
triangle(0, 500, 300, 500, 150, 100);
//Mountain 4
fill(71, 153, 190);
triangle(180, 500, 300, 500, 250, 280);
triangle(200, 500, 450, 500, 340, 300);
triangle(350, 500, 450, 500, 400, 320);
triangle(350, 500, 460, 500, 420, 320);
triangle(400, 500, 520, 500, 470, 350);
//Mountain 4
fill(51, 129, 175);
triangle(180, 500, 400, 500, 300, 250);
triangle(300, 500, 450, 500, 380, 280);
//Mountain 4
fill(38, 94, 151);
triangle(300, 500, 450, 500, 360, 370);
triangle(350, 500, 500, 500, 450, 300);
//Mountain 3
fill(31, 66, 120);
triangle(-30, 500, 100, 500, 50, 200);
triangle(-30, 500, 100, 500, 10, 250);
triangle(20, 500, 200, 500, 110, 200);
triangle(60, 500, 300, 500, 200, 200);
//Mountain 2
fill(18, 49, 87);
triangle(-30, 500, 100, 500, 50, 300);
triangle(-30, 500, 100, 500, 10, 350);
triangle(20, 500, 200, 500, 110, 370);
triangle(60, 500, 300, 500, 200, 440);
triangle(70, 500, 300, 500, 200, 350);
triangle(90, 500, 330, 500, 300, 440);
triangle(160, 500, 400, 500, 300, 300);
triangle(300, 500, 450, 500, 400, 350);
triangle(400, 500, 500, 500, 450, 400);
//Mountain 1 (Darkest Blue)
fill(0, 7, 18);
triangle(-30, 500, 100, 500, 50, 400);
triangle(20, 500, 200, 500, 130, 420);
triangle(60, 500, 300, 500, 200, 440);
//Design Code --> The mountains
//When it becomes 59 minutes a flag is drawn on the top of the mountain to indicate that the onion has reached the peak (victory!!!)
if(M == 59){
fill(255);
rect(130, 100, 15, 10);
fill(240, 235, 231);
triangle(130, 110, 130, 100, 135, 105)
push()
stroke(0);
line(145, 100, 145, 120);
pop()
}
//Sprout climbs up mountain (Minutes)
var sprout = M * 2
fill(255);
ellipse(140, 240-sprout, 10, 10);
rect(138, 240-sprout, 4, 10, 10);
fill(129, 188, 179-M);
ellipse(136, 235-sprout, 8, 5);
ellipse(144, 235-sprout, 8, 5);
//Changing Moon Shape (Hours)
for(var moon = 1; moon < H + 1; moon++){
fill(196, 219, 230); //blue moon
ellipse(400, 100, 100, 100);
fill(240, 235, 231); //cut moon
ellipse(450, 100, (moon*3)+70, (moon*3)+70); //changes only the part of the moon that cuts the moon
fill(214, 233, 242); //Clouds
ellipse(300, 120, 25, 10);
ellipse(330, 140, 70, 20);
ellipse(360, 130, 70, 20);
ellipse(400, 150, 60, 15);
}
//Making snow fall (Seconds) -- Amount of Snow = Number of Seconds
for(var snowfall = 0; snowfall < S; snowfall++){
fill(255);
ellipse(snowfallx[snowfall], snowfally[snowfall], snowd, snowd);
}
}
I wanted to create some sort of landscape image and I really wanted to create an image that incorporated receding mountains so I tried to sketch something up on illustrator. I noticed that I also had a big chunk of space on the top right so I wanted to add the moon there to use as the hour indicator since it seemed to fit with the overall mountain theme.
The next part of my thought process was that since my mountains were blue colored they seemed as though they were cold, so I wanted to create a snowy looking scenario, which is why I created something that looked like falling snow with a for loop and an array.
My last thing is that I wanted to incorporate something that I’ve been using in all of my projects so far, which is a white onion (the white circle) with a green sprout coming out of its head.
The sprout slowly moves up the mountain as minutes pass and when it finally reaches the peak (59 minutes) it puts a flag on top of it (victory!!).
mstropka-Project-06-E
//Max Stropkay
//Section E
//mstropka@andrew.cmu.edu
//Project-06
function setup() {
createCanvas(480,480);
angleMode(DEGREES);
}
function draw(){
background(225);
//variables for hours, minutes, and seconds
h = hour();
m = minute();
s = second();
//display text under clock
text(h%12 + ':' + m + ':' + s, 220, 400);
//rotate entire canvas -90 degrees so 0 degrees is pointing up
push()
translate(width/2, height/2);
rotate(-90);
//draw arc
strokeWeight(2);
//end of arc is defined by the value of seconds
var sAngle = map(s, 0, 60, 0, 360);
fill(255, 51, 51, 40);
arc(0, 0, 250, 250, 0, sAngle);
//draw a smaller arc where the end is defined by the value of minutes
var mAngle = map(m, 0, 60, 0, 360);
fill(51, 153, 255, 50);
arc(0, 0, 200, 200, 0, mAngle)
//instead of mapping the value of hours, map the value of h%12,
//so 12 hours fits into 360 degrees instead of 24
//draw arc where the end point is defined by h % 12
var hAngle = map(h % 12, 0, 12, 0, 360);
fill(128, 128, 128, 70);
arc(0, 0, 150, 150, 0, hAngle)
pop();
}
For this project, I started with the code I used for assignment C, which drew arcs that formed completed circles as the values for hours, minutes, and seconds increased. In the assignment I hid the arcs and drew lines that pointed to the endpoints to create the hands of a clock. For this, I got rid of the hands and filled in the arcs with transparent colors.
rmanagad-project06-abstractclock
//Robert Managad
//Section E
//rmanagad@andrew.cmu.edu
//Project-06
//color directory
// background and papercrease(2, 53, 61);
// paperlightred(187, 42, 47)
// paperdarkred(160, 42, 56)
// earthocean(65, 200, 225)
// earthland(6, 181, 153);
// earthcloudlight (204, 204, 204)
// earthclouddark (178, 178, 178);
// LightCometStreak(195, 215, 216);
// medCometStreak (129, 166, 176)
// darkCometStreak (37, 60, 84);
//earth parameters
var earthWH = 128;
var earthX = 240;
var earthY = 240;
var landXY1 = 0;
var landXY2 = 0;
var landXY3 = 0;
var landXY4 = 0;
//movement parameters
var dirX = 1;
var dirY = 1;
var dirXComet = 1;
var dirYComet = 1;
var speed = 0.1;
var speedComet = 0.1
function setup() {
createCanvas(480, 480);
}
function draw() {
rectMode(CENTER);
angleMode(DEGREES);
var mil = millis();
var sec = second();
var min = minute();
var h = hour();
var revert = h % 12 // to make hour go back to 0 position at 12:00
background(2, 53, 61);
noStroke();
fill(65, 200, 225);
ellipse(earthX, earthY, earthWH, earthWH);
earthX += dirX * speed/2; // motions the x cooridnate
earthY += dirY * speed/2; // motions the y coordinate
//constraining wiggle movement
if (earthX > width/2 + 0.5 || earthX < width/2 - 0.5) {
dirX = -dirX;
}
else if (earthY > height/2 + 0.5 || earthY < height/2 - 0.5) {
dirY = random(-1, 1);
}
//land masses start
push();
translate(width/2, height/2)
rotate(revert);
push()
rotate(12.45);
fill(6, 181, 153);
ellipse(landXY1-50, landXY1, 16.8, 39.5);
landXY1 += dirX * (speed/2); // speed cut to modify motions
landXY1 += dirY * (speed/2);
pop();
push()
rotate(1);
fill(6, 181, 153);
ellipse(landXY2-10, landXY2-25, 12.725, 21.3);
landXY2 += -dirX * (speed/4); // directions reversed to modify motions
landXY2 += -dirY * (speed/4);
pop();
push()
rotate(20);
fill(6, 181, 153);
ellipse(landXY2-13, landXY2-14, 21.21, 26.1);
pop();
push()
rotate(-10);
fill(6, 181, 153);
ellipse(landXY2-1, landXY2-15, 15.9, 20.4);
pop();
push()
rotate(0);
fill(6, 181, 153);
ellipse(landXY2-3.4, landXY2-7.6, 15.9, 20.4);
pop();
push()
rotate(20);
fill(6, 181, 153);
ellipse(landXY3+36, landXY3+18.7, 30, 46.12);
landXY3 += dirX * (speed/3);
landXY3 += -dirY * (speed/3); //direction reversed to modify motion
pop();
push()
rotate(-20);
fill(6, 181, 153);
ellipse(landXY4+30, landXY4-40, 18, 18.5);
landXY4 += -dirX * (speed/6); // direction reversed to modify motion
landXY4 += dirY * (speed/6);
pop();
pop();
// paper airplane start
push();
translate(width/2, height/2); // translates starting point to center of canvas
for (var i = 0; i < sec/3; i++) {
rotate(mil/30); //motion produced via rapid milliseconds
stroke(160, 42, 56)
strokeWeight(1)
line(230-width/2, 140.3-height/2, 220-width/2, 140.3-height/2);
}
pop();
push();
translate(width/2, height/2); // translates starting point to center of canvas
for (var i = 0; i < 10; i++) {
rotate(mil/30); //motion produced via rapid milliseconds
stroke(160, 42, 56)
strokeWeight(2)
line(230-width/2, 140.3-height/2, 220-width/2, 140.3-height/2);
}
pop();
push();
translate(width/2, height/2); // translates starting point to center of canvas
rotate(sec*6); //Dividing function to rotate in a full revolution in 60 ticks
fill(160, 42, 56)
triangle(240-width/2, 135.8-height/2, 234-width/2, 140.4-height/2, 240-width/2, 144.8-height/2);
fill(187, 42, 47)
triangle(240-width/2, 130.7-height/2, 264-width/2, 140.5-height/2, 240-width/2, 150.43-height/2);
stroke(2, 53, 61)
strokeWeight(1)
line(240-width/2, 140.3-height/2, 251-width/2, 140.3-height/2)
pop();
//comet start
push();
translate(width/2, height/2); // translates starting point to center of canvas
rotate(min); //Dividing function to rotate in a full revolution in 60 ticks
noStroke();
//darkStreaks
fill(129, 166, 176);
rect(247.2-width/2, 67.3-height/2, 34.06, 2, 30);
fill(129, 166, 176);
rect(242.7-width/2, 73.635-height/2, 34.06, 2, 30);
fill(129, 166, 176);
rect(249.16-width/2, 81.05-height/2, 34.06, 2, 30);
//lightStreaks
fill(195, 215, 216);
rect(252.66-width/2, 62.941-height/2, 35.35, 3.05, 30);
fill(195, 215, 216);
rect(230.14-width/2, 69.33-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(243.5-width/2, 78.22-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(233.8-width/2, 82.06-height/2, 38.04, 2, 30);
fill(195, 215, 216);
rect(252.66-width/2, 86.25-height/2, 35.35, 3.05, 30);
//cometBackLayer
fill(195, 215, 216);
ellipse(269.83-width/2, 74.6-height/2, 27.66, 26.07);
//cometMidLayer
fill(129, 166, 176);
ellipse(270.8-width/2, 74.6-height/2, 22.76, 23.33);
//cometBase
fill(37, 60, 84)
ellipse(272.4-width/2, 74.6-height/2, 20.64, 20.64);
push();
//comet craters
translate(272.4-width/2, 74.6-height/2);
rotate(mil/1.5); // rotates craters within the comet
fill(195, 215, 216);
push();
rotate(20);
ellipse(1.2, -7, 6, 1.6)
pop();
push();
rotate(-20);
ellipse(0.75, 8, 6, 2.5)
pop();
push();
rotate(50);
ellipse(-1, 8, 6, 2)
pop();
pop();
pop();
fill(163, 77, 47);
textSize(15);
text(nf(revert, [2], [0]) + " : " + nf(min, [2], [0]) + " : " + nf(sec, [2], [0]), width/2 - 35, 470); // added nf to second function to get two digits.
}
My idea stemmed around a nuanced, playful version of the act of orbiting — I wanted to show the journey of a paper airplane flying around the world, placing it at a scale similar to a handful of satellites. The plane’s movement represents seconds, the comet’s orbit’s minutes, and the rotation of the land masses on the earth represents hours, while the smaller dashed line are a creative play on figuring out how to use milliseconds to display agile, not-jittery movement.
After sketching my initial ideas down, I used Adobe Illustrator to plan out the look of the elements as well as the program’s composition.
thlai-Project-06-Abstract-Clock
I was inspired by both Wassily Kandinsky’s abstract art and the MOMA’s perpetual calendar. I put the two of these together to create a more minimal version of an abstract clock.
The triangle spins using milliseconds, the red arc grows using seconds, the black circle moves using minutes, and the gray circle moves along the black bar using hours.
// Tiffany Lai
// 15-104, Section A
// thlai@andrew.cmu.edu
// Project 06 - Clock
function setup() {
createCanvas(480, 480);
angleMode(DEGREES);
}
function draw() {
background(258, 252, 234);
fill(250, 244, 219);
noStroke();
ellipse(50, 50, 750, 750); // background left circle
// TIME
/* Every millisecond, the background triangle will spin.
Every second, the red circle will be filled in more.
Every minute, the black dot in the red circle will move.
Every hour, the gray circle on the black bar will move. */
milliseconds(); // background triangle
seconds(); // red circle
hours(); // black bar
minutes(); // black circle inside red circle
strokeWeight(2);
stroke(190, 50, 50);
line(350, 285, height, 285); // line indicating 0 seconds
noFill();
stroke(60);
line(235, 64, 301, 50); // decoration line 1
line(242, 84, 304, 62); // decoration line 2
ellipse(width-30, 0, 400, 400); // decoration circle 1
ellipse(width+10, 10, 400, 400); // decoration circle 2
push();
noStroke();
fill(0, 53, 176, 80);
ellipse(370, 190, 100, 100); // blue circle
pop();
}
// HOURS
function hours() {
push();
noStroke();
fill(100);
rect(25, 340, 265, 13); // draw bar
var h = hour() % 12; // 12 hr time instead of 24 hr time
if (h == 0){
h = 12;
}
var hx = map(h, 0, 11, 32, 282); // map hours to length of bar
fill(200);
ellipse(hx, 332, 16, 16); // draw circle
pop();
print(hour());
}
// MINUTES
function minutes(){
var m = minute();
var mx = map(m, 0, 59, 0, 360);
push();
fill(0);
noStroke();
translate(270, 285);
rotate(mx);
ellipse(100, 0, 16, 16);
pop();
}
// SECONDS
function seconds(){
var s = second();
var sx = map(s, 0, 60, 0, 360); // map minutes to circle
if (s==60){
s = 0;
}
// red circle
push();
noFill();
strokeWeight(13);
stroke(223, 97, 97);
ellipse(270, 285, 230, 230); // draw circle
translate(270, 285);
strokeWeight(13);
stroke(190, 50, 50);
strokeCap(SQUARE);
arc(0, 0, 230, 230, 0, sx); // arc grows by the second
pop();
}
// MILLISECONDS
function milliseconds(){
var prevSec = s;
var millisRollover = 0;
var s = second();
if (s != s) {
millisRollover = millis();
}
var mils = floor(millis() - millisRollover);
var milsToSeconds = s + (mils/1000.0);
var milsRotate = map(milsToSeconds, 0, 60, 0, 360);
// triangle
push();
noStroke();
translate(width/2-20, height/2);
rotate(milsRotate);
fill(239, 234, 203);
triangle(0, -195, -167, 97, 167, 98);
pop();
}
gyueunp – Project-06: Abstract Clock
//GyuEun Park
//15-104 E
//gyueunp@andrew.cmu.edu
//Project-06
var maxDiameter = 120;
var theta = 0;
var x = []; // every variable now starts out as an empty array
var y = [];
var dx = []; // velocity in x direction (d for "delta" meaning change or difference)
var dy = []; // velocity in y direction
var col = [];
function setup() {
createCanvas(400, 400);
// the radius of the circle
r = height * 0.3;
speed = 10;
theta = 0;
startTime= second();
for (i = 0; i < 50; i++) {
x[i] = random(width);
y[i] = random(height);
dx[i] = random(-5, 5);
dy[i] = random(-5, 5);
col[i] = color(255,50);
}
frameRate(50);
}
function draw() {
background(117, 2, 21, 10);
push();
var diam = 150 + sin(theta) * maxDiameter ;
stroke(117, 2, 21,80);
strokeWeight(10);
fill(255,13);
ellipse(width/2, height/2, diam, diam);
theta += 10;
pop();
push();
angleMode(DEGREES);
translate(200,200);
rotate(-90);
// time variables
var hr = hour();
var mn = minute();
var sec = second();
stroke(0);
var secAngle = map(sec,0,60,0,360);
stroke(0);
var mnAngle = map(mn,0,60,0,360);
// restart once it gets to 12 and 13 becomes 1 o'clock
stroke(0);
var hrAngle = map(hr%12,0,12,0,360);
// second hand, highlight on iris
push();
rotate(secAngle);
translate(5,5);
stroke(255);
fill(255);
ellipse(0,0,3,3);
pop();
// minute hand, iris
push();
if (mn % 2 == 0){
stroke(255);
ellipse(0,0,40,40);
} else {
stroke(158,3,29);
fill(158,3,29,10);
ellipse(0,0,40,40);
}
pop();
// hour hand, sclera
push();
if (hr % 2 == 0){
stroke(157,8,32);
strokeWeight(10);
noFill();
ellipse(0,0,385,385);
} else {
stroke(157,8,32);
strokeWeight(10);
noFill();
ellipse(0,0,280,280);
}
pop();
pop();
// stagnant pupil in the center of canvas
push();
fill(158,3,29)
stroke(158,3,9,30);
strokeWeight(10,2);
ellipse(width/2,height/2,10,10);
pop();
// swimming rectangles
noStroke();
for (i = 0; i < 20; i++) {
fill(col[i]);
rect(x[i], y[i], 10, 10);
x[i] += dx[i];
y[i] += dy[i];
if (x[i] > width) x[i] = 0;
else if (x[i] < 0) x[i] = width;
if (y[i] > height) y[i] = 0;
else if (y[i] < 0) y[i] = height;
}
}
This abstract clock resembles an eye in that it is composed of various circular forms that are distinct from one another. The rapid blink-like movement and the gliding objects do not represent the time, but instead add a sense of agitation and discomfort. In fact, the subtle alterations of object positions and colors are the components that represent time. In short, this project portrays not only the repetitive nature of time, but also the sense of tension that is created by the limitation of time.
sijings-project06-abstractclock
var eyeballx;
var eyebally;
var mouthp=326;
var hourF=255;
var minuteF=255;
var secondF=255;
var centerS=5;
var eyeballs=30;
var eyelidsx1=480/2; //replaced width
var eyelidsx2=80;
var eyelidsx3=180;
var eyelidsy1=480/2-20;
var eyelidsy2=80;
var eyelidsy3=360;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(250,250,250);
var h = hour();
var m = minute();
var s = second();
//eyeball-second
eyeballx=width/2;
eyebally=height/2-30;
secondF=255-s*2;//transparency decreasing based on minutes seconds and hours
minuteF=255-m*5;
hourF=255-h*10;
angleMode(DEGREES);
noFill();
strokeWeight(3);
//eyes opening-second
if (s%2==0){//for each second, eye will correspondingly open and close
stroke(0,0,0,secondF);
push();
fill(0,0,0,secondF);
ellipse(eyeballx,eyebally,centerS,centerS);
fill(0,20,128,secondF);
ellipse(eyeballx,eyebally,eyeballs,eyeballs);
pop();
arc(eyelidsx1, eyelidsy1, eyelidsx2, eyelidsy2, eyelidsx3, eyelidsy3);
}else{
stroke(0,0,0,secondF);
arc(eyelidsx1, height/2-50, eyelidsx2, eyelidsy2, eyelidsy3, eyelidsx3);
}
fill(0,0,0,secondF);
ellipse(width/2+5,84,20,20);
noFill();
stroke(0,0,0,secondF);
curve(width/2+40, 86, width/2+5, 94, width/2+5, 181, width/2+40, 115);
fill(173,115,66,minuteF); //for colouring fading in minute
stroke(0,0,0,hourF);//for colouring fading in hour
push();
strokeWeight(0);
curve(width/2-110, height/2+6, width/2+5, height/2+4, width/2+5, height/2+71, width/2-310, height/2-80);
strokeWeight(3);
fill(172,36,25,hourF);
curve(width/2+10, 396, width/2-10, mouthp, width/2+37, mouthp, width/2+30, 431);
curve(width/2+10, 121, width/2-10, mouthp, width/2+82, mouthp, width/2+30, 121);
translate(45, 0);//for moving the second lips
curve(width/2+10, 396, width/2-10, mouthp, width/2+37, mouthp, width/2+30, 431);
pop();
//minute
var fp1=width/2;
var fp2=height/2-70;
var offset1=10;
var offset2=15;
for (var y = 0; y < 6; y++) {//nested for loop for drawing the cols and rows
noStroke();
for (var x = 0; x < 5; x++) {
if (((x+1)+(y)*5)<=m){//determing when the next dot appears
fill(168,59,32,minuteF);
var py = fp1 + y * offset1;
var px = fp2 + x * offset2;
ellipse(px, py, 10, 10);
}
}
}
var fp3=width/2;
var fp4=height/2+70;
for (var y1 = 0; y1 < 6; y1++) {
noStroke();
for (var x1 = 0; x1 < 5; x1++) {
if (((x1+1)+(y1)*5)<=(m-30)){//note: need to minus 30 for calculating the second part of the dots
fill(168,59,32,minuteF);
var py1 = fp3 + y1 * offset1;
var px1 = fp4 + x1 * offset2;
ellipse(px1, py1, 10, 10);
}
}
}
//hour
noFill();
stroke(173,115,66,hourF);
arc(width/2-110, height/2-70, 50, 50, 90, 270);
var hourh=height/2-50
for (var i=0;i<h;i++){//number of earings accordings to number of hours
ellipse(width/2-110, hourh,20,20);
hourh+=10;
}
}
For this project, I intended to express an idea of “fading”. The start point of the clock will be a full face with all features visible (not including the earings and the wrinkles, since they are the indication of cumulative time). Then, as time progressed, the eyes will blink (close and open) as an indicator for seconds. The number of wrinkles will increase as minutes increases. So the number of blush will be the number of minutes for each hour. Lastly, the number of earings will be the number of hours. Thus, a way to calculate time is to add the number of earings with number wrinkles and the number of times the eye blinks. At the end, the whole face will fade away.
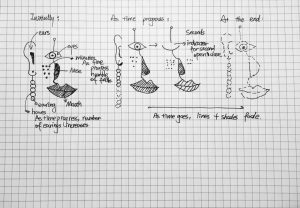
Here are some screenshots for different time periods, so we can see the different representation of time changes.
monicah1-project-06
var prevSec;
var millisRolloverTime;
function setup() {
createCanvas(400, 400);
ellipseMode(CENTER);
fill(255);
noStroke();
}
function draw(){
background(0);
var H = hour();
var M = minute();
var S = second();
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
var rotate1 = TWO_PI * noise(0.005*frameCount + 5);
var rotate2 = TWO_PI * noise(0.005*frameCount + 10);
var rotate3 = TWO_PI * noise(0.005*frameCount + 15);
var rx = 60 * noise(0.005*frameCount + 10);
var tx = 200 * noise(0.005*frameCount + 20);
var volume1 = 200 * noise(0.005*frameCount + 30);
var volume2 = 50 * noise(0.005*frameCount + 30);
var secondsWithFraction = S + (mils / 1000.0);
var secondsWithNoFraction = S;
var secondBarWidthChunky = map(secondsWithNoFraction, 0, 60, 0, width);
var secondBarWidthSmooth = map(secondsWithFraction, 0, 60, 0, width);
translate(width/2, height/2);
for (var i = 0; i < 10; i++) {
push();
rotate(rotate1 + TWO_PI * i / 3);
translate(tx, 0);
fill(0,255,60);
ellipse(0, 0, volume1, volume2);
for (var j = 0; j < 9; j++) {
push();
rotate(rotate2 + TWO_PI * j / 1);
translate(rx, 0);
rotate(rotate3);
fill(250,250,0);
ellipse(rx, 0, volume2, volume2);
pop();
}
translate()
pop();
}
}
I made a clock based on elements of rotation and noise.
katieche-project-06
/*
katie chen
section e
project 06
katieche@andrew.cmu.edu
*/
function setup() {
createCanvas(300, 300);
}
function draw() {
var h = hour();
var m = minute();
var s = second();
angleMode(DEGREES);
// make it 12 hours!
if (h > 11) {
h = h - 12;
}
else {
h=h;
}
background (196, 220, 255);
textAlign(CENTER);
text(h+":"+m+":"+s, width/2, 40);
noStroke();
// minute / rabbit rising
push();
fill (250);
ellipse (150, 370-m, 100, 170);
ellipse (125, 290-m, 20, 90);
ellipse (175, 290-m, 20, 90);
fill (250, 210, 196);
ellipse (120, 325-m, 10, 10);
ellipse (180, 325-m, 10, 10);
fill (0);
ellipse (125, 320-m, 7, 7);
ellipse (175, 320-m, 7, 7);
pop();
//hour / sun
push();
fill (255, 235, 127);
ellipse (260*h/11, 100, 40, 40);
noFill();
stroke(255, 236, 170);
strokeWeight (2);
ellipse (260*h/11, 100, 55, 55);
pop();
//seconds / clouds
push();
fill (250);
ellipse(300*s/59, 100, 30, 30);
ellipse(300*s/59, 105, 50, 20);
ellipse(370*s/59, 140, 40, 30);
ellipse(380*s/59, 145, 80, 20);
ellipse(220*s/59, 125, 60, 20);
ellipse(210*s/59, 120, 40, 20);
ellipse(260*s/59, 140, 40, 10);
pop();
}
I wanted to do something completely different from the traditional rotating clock. I tried to create more of a story, where the sun and clouds move horizontally like in real life and the rabbit rises and sinks.