function setup() {
createCanvas(480, 480);
}
function draw() {
background(56, 136, 249);
stroke(66, 229, 253);
strokeWeight(5);
noFill();
//shaky box
rect(width/2 - random(118,120), height/2 - random(118,120),
random(238,240), random(235,240));
//draw the hypcocylcoids
push();
translate(width/2, height/2);
drawHypocyclo();
drawCyclo2();
pop();
}
function drawHypocyclo(){ //hypocycloid
var x;
var y;
//big radius
var a = map(mouseX, 0, 480, 50 *.01 * mouseX, 25 * .01 * mouseX);
//lil radius
var b = map(mouseY, 0, 480, 1 * .01 * mouseY, 10 * .01 * mouseY);
var r; //angle
beginShape();
stroke(255);
strokeWeight(1);
for (var r = 0; r < TWO_PI * 10; r += 0.01){
x = (a - b) * cos(r) - b * cos(((a-b)/(b))* r);
y = (a - b) * sin(r) + b * sin(((a-b)/(b))* r);
vertex(x,y);
}
endShape();
}
function drawCyclo2(){
var a = map(mouseX, 0, 480, 50 *.01 * mouseX, 80 * .01 * mouseX);
var b = map(mouseY, 0, 480, 10 * .01 * mouseY, 90 * .01 * mouseY);
beginShape();
stroke(66, 229, 253);
strokeWeight(3);
for (var r = 0; r < TWO_PI * 50; r += 0.01){
x = (a - b) * cos(r) - b * cos(((a-b)/(b))* r);
y = (a - b) * sin(r) + b * sin(((a-b)/(b))* r);
vertex(x,y);
}
endShape();
}
I had a surprising amount of fun with this project once I was able to figure out the math and which variables to apply to which mouse function. It was also a good opportunity for me to finally figure out how the map(); function works, which I really shouldn’t have saved for until now.
While I was playing around on the Mathworld website (wow) I found myself in love with the Hypocycloid and all the different patterns you could get out of it, so I drew two of them. I added the shaky box as a sort of center piece, and I like how it acts as a containment unit for the inner curve.
Here are a couple of states that I really enjoyed from my project.
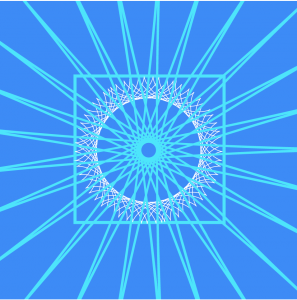
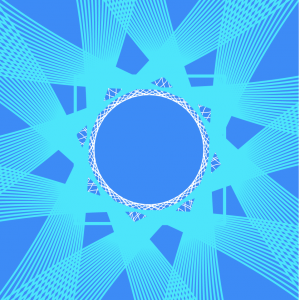
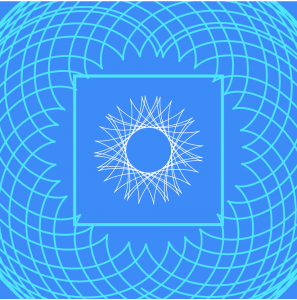
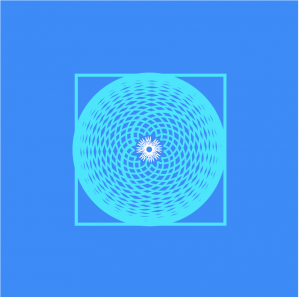