// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section D
// Assignment-11: Freestyle Turtles
// Global Variables
var d = 150;
var x = 50;
var y = 300;
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
function setup(){
createCanvas(400, 400);
background(0);
var t1 = makeTurtle(x, y);
var t2 = makeTurtle(x, y);
var t3 = makeTurtle(x, y);
var t4 = makeTurtle(x, y);
var t5 = makeTurtle(x, y);
// Controls the color and lineweight of the meanders
t1.setColor(255);
t1.setWeight(2);
t2.setColor(255);
t2.setWeight(2);
t3.setColor(255);
t3.setWeight(2);
t4.setColor(255);
t4.setWeight(2);
t5.setColor(255);
t5.setWeight(2);
// Drwas the different meanders
// meander1(t1);
// meander2(t2);
meander3(t3);
meander4(t4);
meander5(t5);
}
// Creates path of first stage
function meander1(t1){
t1.left(60);
t1.forward(d);
t1.right(60);
t1.forward(d);
t1.right(60);
t1.forward(d);
}
// Creates path of second stage
function meander2(t2){
t2.forward(d/2);
t2.left(60);
t2.forward(d/2);
t2.left(60);
t2.forward(d/2);
t2.right(60);
t2.forward(d/2);
t2.right(60);
t2.forward(d/2);
t2.right(60);
t2.forward(d/2);
t2.right(60);
t2.forward(d/2);
t2.left(60);
t2.forward(d/2);
t2.left(60);
t2.forward(d/2);
}
// Creates path of third stage
function meander3(t3){
t3.left(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4)
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.left(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
t3.right(60);
t3.forward(d/4);
}
// Creates path of fourth stage
function meander4(t4){
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8)
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.right(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
t4.left(60);
t4.forward(d/8);
}
// Creates path of fifth stage
function meander5(t5){
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16)
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.left(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
t5.right(60);
t5.forward(d/16);
}
I was inspired the Sierpinski Triangle, and the result of my code is meant to be an abstracted version, with a similar idea. I wanted to challenge myself, so I was originally looking into ideas of recursion to draw the Sierpinski Triangle, along with the turtle graphics, but unfortunately after a while it went beyond what I was capable of. I chose to keep going with the idea, and abstract it, in the hope that sometime in the future I will learn (which I did), and possibly rework the code some day to make what I originally set out to. However, I think this is still a pretty interesting graphic. I commented out the first two meanders.
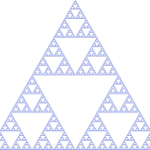