//Christy Zo
//Section C
var nPoints = 400;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(220);
push();
translate(width / 2, height / 2);
drawEpitrochoidCurve();
pop();
push();
translate(width / 2, height / 2);
drawEpicycloidCurve();
pop();
push();
translate(width / 2, height / 2);
rotate(radians(90)); //rotating Epicycloid to create a radial pattern
drawEpicycloidCurve();
pop();
push();
translate(width / 2, height / 2);
rotate(radians(45));
drawEpicycloidCurve();
pop();
push();
translate(width / 2, height / 2);
rotate(radians(135));
drawEpicycloidCurve();
pop();
}
function drawEpicycloidCurve() {
// Epicycloid:
// http://mathworld.wolfram.com/Epicycloid.html
var x;
var y;
var a = constrain(mouseY,0, height);
var b = constrain(mouseX, 0, width);
//var h = constrain(mouseY / 8.0, 0, b);
//var ph = mouseX / 50.0;
noFill();
stroke(0);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = 4 * b * cos (t)* cos (t)* cos (t);
y = a * sin (t) *sin (t)* sin (t);
vertex(x, y);
}
endShape(CLOSE);
}
function drawEpitrochoidCurve() {
// Epicycloid:
// http://mathworld.wolfram.com/Epitrochoid.html
var x;
var y;
var a = mouseX;
var b = a / 2.0;
var h = constrain(mouseY / 8.0, 0, b);
var ph = mouseX / 120.0;
fill(mouseX, mouseY, mouseX);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a + b) * cos(t) - h * cos(ph + t * (a + b) / b);
y = (a + b) * sin(t) - h * sin(ph + t * (a + b) / b);
vertex(x, y);
}
endShape(CLOSE);
}
In this project, I wanted to create something that not only shows horizontal and vertical change, but something that goes diagonally too. I was inspired by how stars twinkle and their strokes/beams of light reduce and increase as it sparkles.

snippets of code:
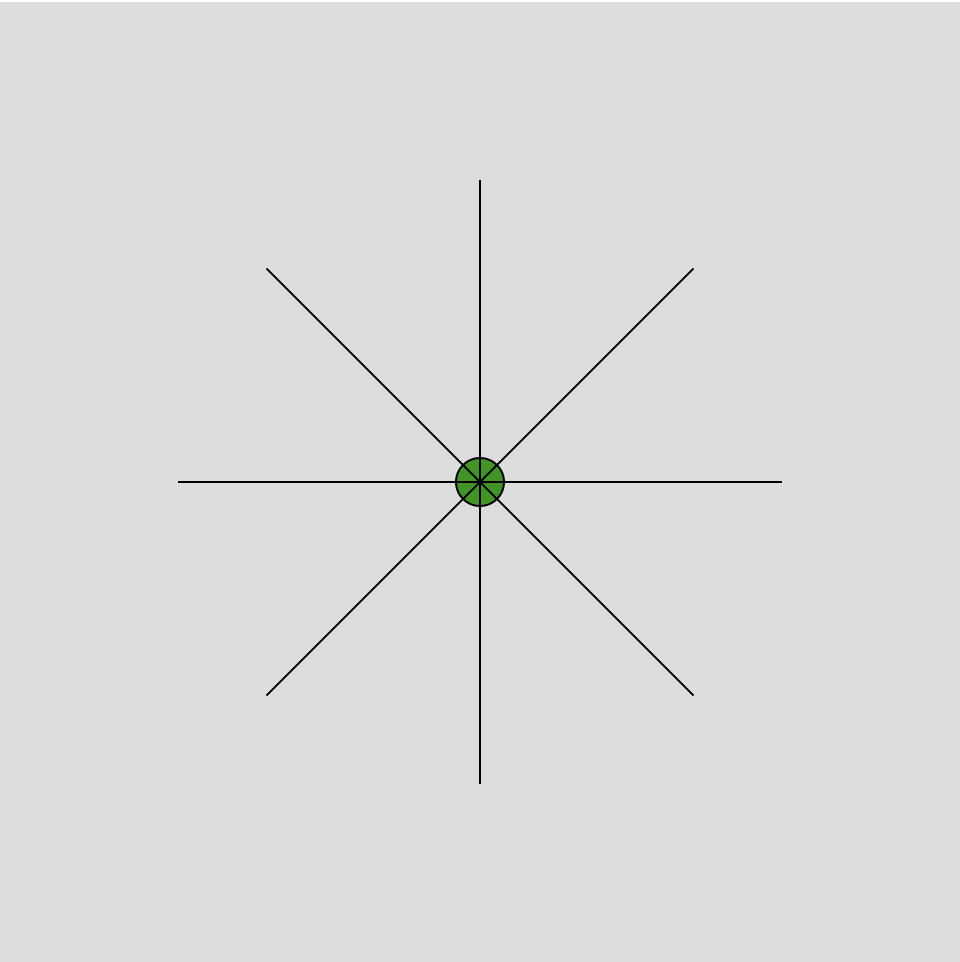
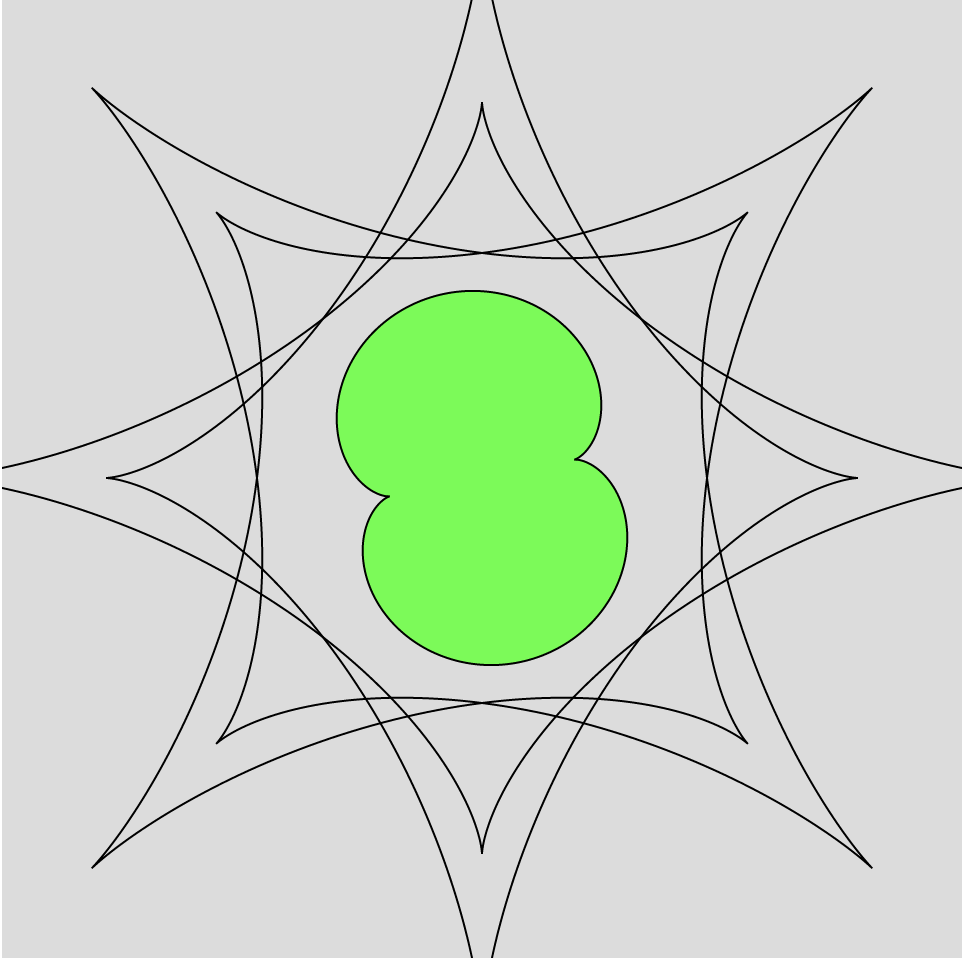
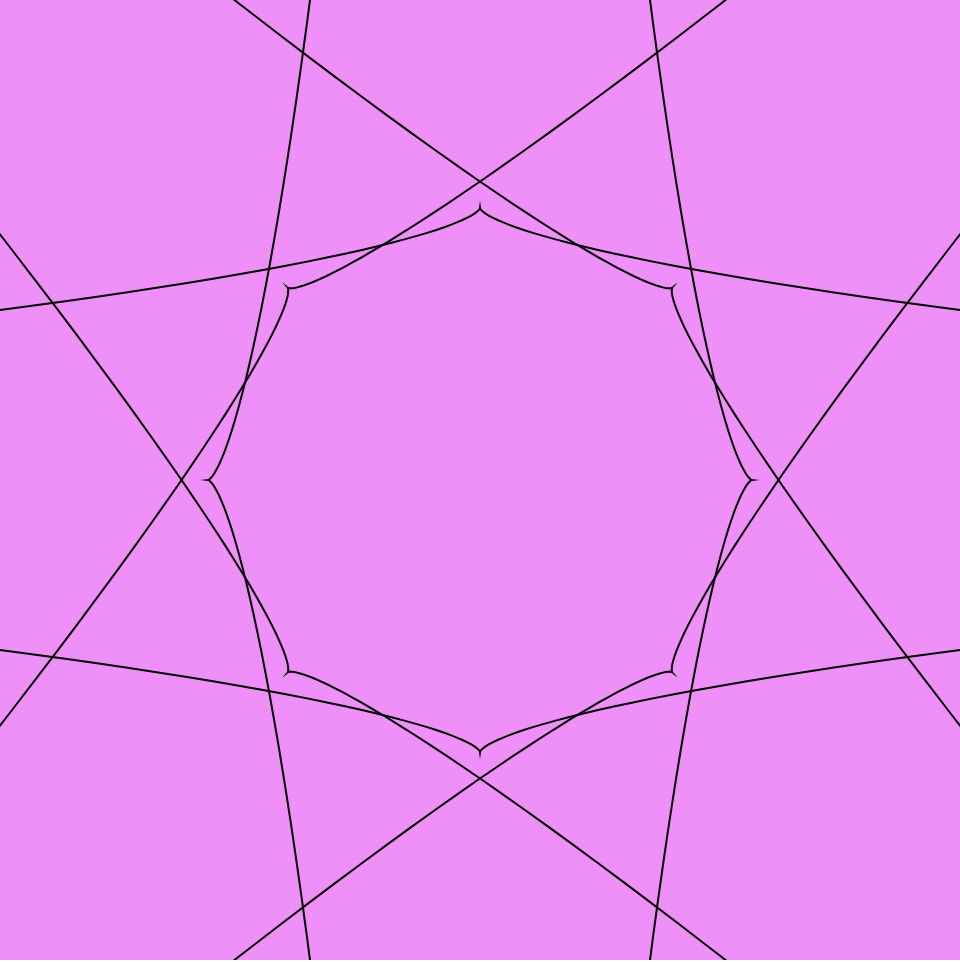
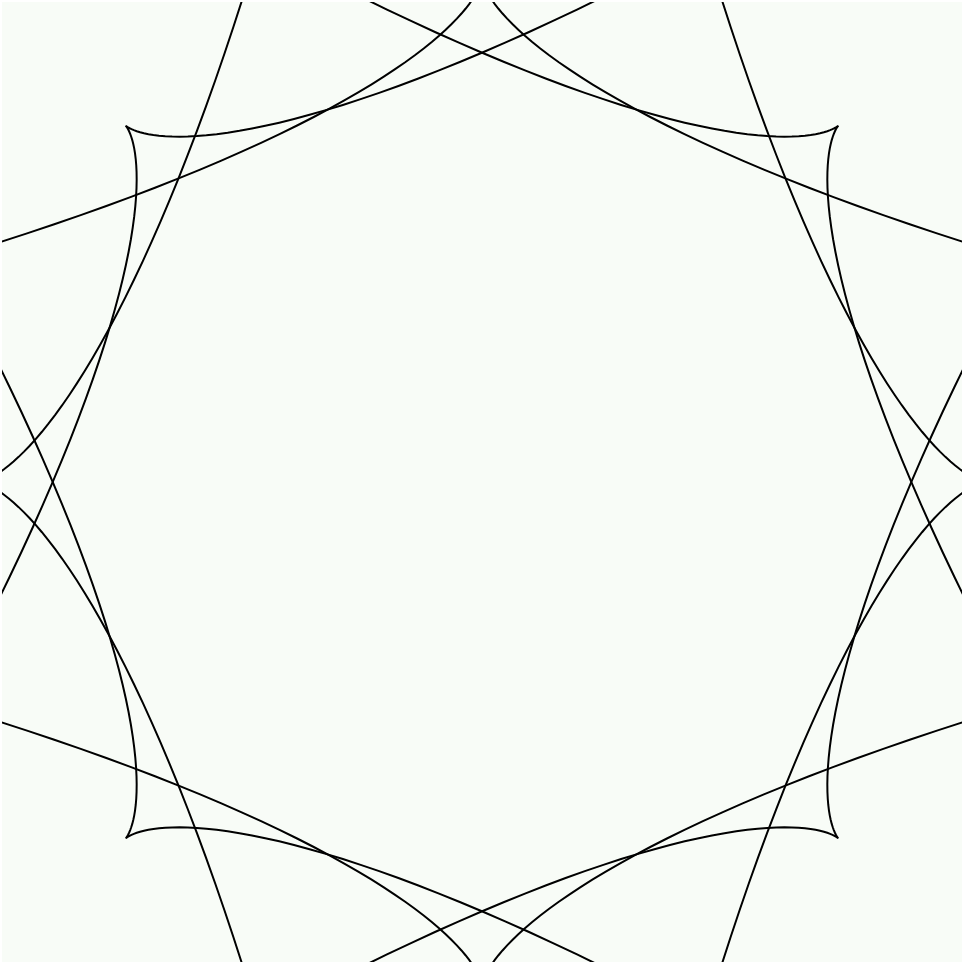
As the cranioid in the center increases in size, the color changes from black-green-pink-white in a gradual scale.