Since this project was so open ended, it took me a while to settle on a design. I was inspired by the spinning squares we learned how to create in the lab and I thought it would be cool to combine many of them to create a shimmer effect. I then decided to sketch out a disco ball utilizing strips composed of rotating squares.
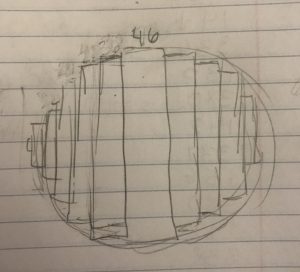
I then found the color scheme from a picture I found via Google. I really wanted to make the click result as unexpected as possible so I decided to stick with white and grey for the pre-clicked image. Then suddenly, with a click of the mouse, the room goes dark and a disco ball appears and begins to spin. All in all, I am satisfied with my product and I really enjoyed this more open-ended project.
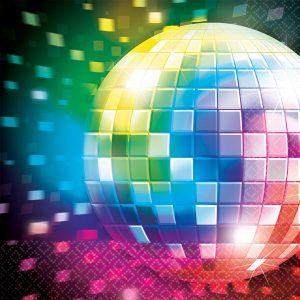
Instructions:
Click to see the squares form into the final design.
Move cursor into left side of the sketch to see it shine.
Move cursor into bottom left corner to see it sparkle.
Click again to see the ball stop “spinning.”
sketch
//Angela Rubin
//Section C
//aerubin@andrew.cmu.edu
//Week 3-Dynamic Drawing
//Rate of Spinning Variables
var angle = 0;
var x = 0;
//Positions of Squares (see bottom for more details)
var a = 23;
var b= 23;
var c= 23;
var d = 391;
var e = 0;
var f = 50;
var g = 391;
var h = 0;
var i = 69;
var j = 23;
var k = 0;
var l = 115;
var m = 0;
var n = 0;
var o = 161;
var p = 0;
var q = 0;
var r = 207;
var s = 0;
var t = 0;
var u = 253;
var v = 0;
var w = 0;
//yellow
var red1 = 255;
var green1 = 255;
var blue1 = 255;
//green
var red2 = 255;
var green2 = 255;
var blue2 = 255;
//blue
var red3 = 255;
var green3 = 255;
var blue3 = 255;
//red
var red4 = 255;
var green4 = 255;
var blue4 = 255;
//Makes Background Black when clicked
var blackBackground = 0;
function setup() {
createCanvas(480, 640);
}
function draw() {
background(230-blackBackground);
fill(255);
noStroke()
//Center 46x46 Squares
push();
translate(a, b);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+150)
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 46));
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+165);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 92));
rotate(radians(angle));
rectMode(CENTER);
fill(red2+150, green2+100, blue2);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 138));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+120, green3+120, blue3+100);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 184));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+40, green3+40, blue3+100);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 230));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+120, blue3+100);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 276));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+200, green3+150, blue3+80);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
push();
translate(a, (b + 322));
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+40, blue4+40);
rect(0, 0, 46, 46);
pop();
angle = angle+x;
//41x41 squares left
push();
translate(c, d);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+110)
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, (d+41));
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+170)
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
//WHITE
push();
translate(c, (d+82));
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, d+123);
rotate(radians(angle));
rectMode(CENTER);
fill (red2+100, green2+100, blue2);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, d+164);
rotate(radians(angle));
rectMode(CENTER);
fill (red3+130, green3+160, blue3+100);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, d+205);
rotate(radians(angle));
rectMode(CENTER);
fill (red3+100, green3+100, blue3+100);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, d+246);
rotate(radians(angle));
rectMode(CENTER);
fill (red3+70, green3+90, blue3+80);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c, d+287);
rotate(radians(angle));
rectMode(CENTER);
fill (red3+180, green3+120, blue3+100);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
//41x41 squares right
//WHITE
push();
translate(c+71, d);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, (d+41));
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+190);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, (d+82));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+180, blue3+130);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, d+123);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+110, green3+120, blue3+60);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, d+164);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+170, blue3+110);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, d+205);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+150, green3+110, blue3+90);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, d+246);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+30, blue4+60);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
push();
translate(c+71, d+287);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+50, blue4+90);
rect(0, 0, (46-e), (46-e));
pop();
angle = angle+x;
//36x36 squares left
push();
translate(f, g);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+60);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+36));
rotate(radians(angle));
rectMode(CENTER);
fill(red2+170, green2+90, blue2+10);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+72));
rotate(radians(angle));
rectMode(CENTER);
fill(red2+110, green2+100, blue2);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+108));
rotate(radians(angle));
rectMode(CENTER);
fill(red2+150, green2+100, blue2);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+144));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+110, green3+140, blue3+100);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
//WHITE
push();
translate(f, (g+180));
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+216));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+60, green3+120, blue3+100);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f, (g+252));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+100, green3+120, blue3+70);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
//36x36 right side
push();
translate(f+140, g);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+160);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+36));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+170, blue3+140);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+72));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+120, blue3+100);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+108));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+110, green3+120, blue3+70);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+144));
rotate(radians(angle));
rectMode(CENTER);
fill(red3+280, green3+180, blue3+130);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+180));
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+60, blue4+30);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+216));
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4, blue4+80);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
push();
translate(f+140, (g+252));
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+60, blue4+40);
rect(0, 0, (46-h), (46-h));
pop();
angle = angle+x;
//31x31 squares left
push();
translate(i, j);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+10);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+31);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+159);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+62);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+100);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+93);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+180, green2+100, blue2);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+124);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+10, green2+40, blue2);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+155);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+30, green3+120, blue3+100);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+186);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+160, green3+160, blue3+110);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i, j+217);
rotate(radians(angle));
rectMode(CENTER);
fill(red3, green3+120, blue3+100);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
//31x31 squares right
push();
translate(i+195, j);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+180)
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
//WHITE
push();
translate(i+195, j+31);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i+195, j+62);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+150, green3+120, blue3+100);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i+195, j+93);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+160, blue3+130);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
//KEEP WHITE
push();
translate(i+195, j+124);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+70, blue4+80);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i+195, j+155);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+90, blue4+100);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i+195, j+186);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+100, blue4+70);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
push();
translate(i+195, j+217);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4, blue4);
rect(0, 0, (46-k), (46-k));
pop();
angle = angle+x;
//26x26 squares left
//WHITE
push();
translate(l, m);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+26);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+52);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+130);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+78);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+180);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+104);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+120, green2+60, blue2);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+130);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+90, green2+130, blue2);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+156);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+16, green3+180, blue3+100);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l, m+182);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+180, green3+170, blue3+100);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
//26x26 squares right
//WHITE
push();
translate(l+238, m);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+26);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+150, green3+120, blue3+110);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+52);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+170, blue3+120);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+78);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+180, green3+120, blue3+70);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+104);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+30, green4, blue4+50);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+130);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+50, blue4+120);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+156);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+20, green4+90, blue4+30);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
push();
translate(l+238, m+182);
rotate(radians(angle));
rectMode(CENTER);
fill (red4, green4+40, blue4+80);
rect(0, 0, (46-n), (46-n));
pop();
angle = angle+x;
// 21x21 sqares left
push();
translate(o, p);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o, p+21);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+150);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
//KEEP WHITE
push();
translate(o, p+42);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o, p+63);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+150, green2+100, blue2);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o, p+84);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+150, green2+170, blue2);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o, p+105);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+40, green2+70, blue2);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
//WHITE
push();
translate(o, p+126);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o, p+147);
rotate(radians(angle));
rectMode(CENTER);
fill(red3, green3+70, blue3+80);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
// 21x21 sqares right
push();
translate(o+270, p);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+170, blue3+120);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+21);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+150, blue3+100);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+42);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+190, green3+120, blue3+120);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
//KEEP WHITE
push();
translate(o+270, p+63);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+84);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+100, blue4+100);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+105);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+50, blue4+70);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+126);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+30, green4+120, blue4+140);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
push();
translate(o+270, p+147);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4, blue4);
rect(0, 0, (46-q), (46-q));
pop();
angle = angle+x;
// 16x16 squares left
push();
translate(r, s);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+100);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+16);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+190);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+32);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+170, green2+100, blue2);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+48);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+100, green2+100, blue2);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+64);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+50, green2+100, blue2);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+80);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+180, green3+180, blue3+100);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+96);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+70, green3+100, blue3+90);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+112);
rotate(radians(angle));
rectMode(CENTER);
fill(red3, green3, blue3+20);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r, s+128);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+120, green3+180, blue3+80);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
// 16x16 squares right
//WHITE
push();
translate(r+300, s);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+16);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+140, green3+120, blue3+120);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+32);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+180, green3+160, blue3+100);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+48);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+40, green4+140, blue4+140);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+64);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+70, blue4+40);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+80);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+20, green4+130, blue4+70);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+96);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4, blue4);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+112);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+60, blue4+120);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
push();
translate(r+300, s+128);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+30, green4+30, blue4+30);
rect(0, 0, (46-t), (46-t));
pop();
angle = angle+x;
// 9x9 squares left
push();
translate(u, v);
rotate(radians(angle));
rectMode(CENTER);
fill(red1, green1, blue1+60);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+9);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+20, green2+100, blue2);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+18);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+30, green2+50, blue2);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+27);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+170, green2+140, blue2);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+36);
rotate(radians(angle));
rectMode(CENTER);
fill(red2+100, green2+90, blue2);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
//WHITE
push();
translate(u, v+45);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+54);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+100, green3+120, blue3+60);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+63);
rotate(radians(angle));
rectMode(CENTER);
fill(red3, green3+120, blue3+60);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+72);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+120, green3+120, blue3+100);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+81);
rotate(radians(angle));
rectMode(CENTER);
fill(red3, green3, blue3+60);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u, v+90);
rotate(radians(angle));
rectMode(CENTER);
fill(red3+120, green3+120, blue3+50);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
// 9x9 squares right
push();
translate(u+323, v);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+50, green4+120, blue4+120);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+9);
rotate(radians(angle));
rectMode(CENTER);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+18);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+30, green4+100, blue4+80);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+27);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+10, green4+60, blue4+20);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+36);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+20, green4+50, blue4+100);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+45);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+60, blue4+100);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+54);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+40, green4+70, blue4+20);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+63);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+80, blue4+90);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+72);
rotate(radians(angle));
rectMode(CENTER);
fill(red4+40, green4+60, blue4+20);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+81);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4+40, blue4+40);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
push();
translate(u+323, v+90);
rotate(radians(angle));
rectMode(CENTER);
fill(red4, green4, blue4);
rect(0, 0, (46-w), (46-w));
pop();
angle = angle+x;
//Sparkles
if(mouseX<width/2) {
stroke(255);
strokeWeight(5);
line(80, 180, 130, 230);
line(130, 180, 80, 230);
line(105, 160-20, 105, 250+20);
line(60-20, 205, 150+20, 205);
}
if(mouseY>height/2) {
line(80+200, 180+200, 130+200, 230+200);
line(130+200, 180+200, 80+200, 230+200);
line(105+200, 160-20+200, 105+200, 250+20+200);
line(60-20+200, 205+200, 150+20+200, 205+200);
}
}
function mouseClicked() {
a = 217; //x position of 46x46 squares
b = 136; //y position of 46x46 squares
c = 184; //x position of 41x41 squares
d = 150; //y position of 41x41 squares
e = 5; //size of 41x41 squares
f = 150; //x position of 36x36 squares
g = 160; //y position of 36x36 squares
h = 10; //size of 36x36 squares
i = 122; //x position of 31x31 squares
j = 170; //y position of 31x31 squares
k = 15; //size of 31x31 squares
l = 100; //x position of 26x26 squares
m = 185; //y position of 26x26 squares
n = 20; //size of 26x26 squares
o = 83; //x position of 21x21 squares
p = 197; //y position of 21x21 squares
q = 25; //size of 21x21 squares
r = 67; //x position of 16x16 squares
s = 205; //y position of 16x16 squares
t = 30; //size of 16x16 squares
u = 55; //x position of 9x9 squares
v = 220; //y position of 9x9 squares
w = 35; //size of 9x9 squares
red1 = 244;
green1 =241;
blue1 = 50;
red2 = 50;
green2 = 130;
blue2 = 82;
red3 = 45;
green3 = 67;
blue3 = 143;
red4 = 212;
green4 = 60;
blue4 = 64;
x = .07 - x; //Makes squares spin
blackBackground = 230;
}