sketch
var cactus = [];var smallCactus = [];
function setup() {
createCanvas(640, 240);
for (var i = 0; i < 6; i++){ var rx = random(width); cactus[i] = makeCactus(rx); }
for(var i = 0; i < 8; i++) { var srx = random(width); smallCactus[i] = makeSmallCactus(srx);
}
frameRate(10);}
function draw() {
background(255, 70, 235);
displayStatusString(); displayHorizon();
updateAndDisplayCactus(); removeCactusThatHaveSlippedOutOfView(); addNewCactusWithSomeRandomProbability();
updateAndDisplaySmallCactus(); removeSmallCactusThatHaveSlippedOutOfView();
addNewSmallCactusWithSomeRandomProbability();
}
function updateAndDisplayCactus(){
for (var i = 0; i < cactus.length; i++){
cactus[i].move();
cactus[i].display();
}
}
function removeCactusThatHaveSlippedOutOfView(){
var cactusToKeep = [];
for (var i = 0; i < cactus.length; i++){
if (cactus[i].x + cactus[i].breadth > 0) { cactusToKeep.push(cactus[i]);
}
}
cactus = cactusToKeep;}
function addNewCactusWithSomeRandomProbability() {
var newCactusLikelihood = 0.007;
if (random(0,1) < newCactusLikelihood) { cactus.push(makeCactus(width)); }
}
function cactusMove() {
this.x += this.speed;
}
function cactusDisplay() {
fill(150, 255, 100); stroke(50, 200, 50); strokeWeight(1); push(); translate(this.x, height - 40);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
for(var i = 0; i < this.nArmsLeft; i++) { beginShape();
curveVertex(this.breadth + 120, -i*30 - 10);
curveVertex(this.breadth/2 - 10, -i*30 - 12);
curveVertex(this.breadth/2 - 35, -i*40 - 50);
curveVertex(this.breadth/2 - 15, -i*40 - 45);
curveVertex(this.breadth/2 - 10, -i*30 - 17);
curveVertex(this.breadth + 60, -i*30 - 15);
endShape();
}
for(var i = 0; i < this.nArmsRight; i++) { beginShape();
curveVertex(this.breadth - 120, -i*30 - 10);
curveVertex(this.breadth/2 + 10, -i*30 - 12);
curveVertex(this.breadth/2 + 35, -i*40 - 50);
curveVertex(this.breadth/2 + 15, -i*40 - 45);
curveVertex(this.breadth/2 + 10, -i*30 - 17);
curveVertex(this.breadth - 60, -i*30 - 15);
endShape();
}
pop();}
function makeCactus(birthLocationX) { var cact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(100, 200), nArmsLeft: round(random(0,5)), nArmsRight: round(random(0,5)), move: cactusMove, display: cactusDisplay} return cact;
}
function updateAndDisplaySmallCactus(){ for (var i = 0; i < smallCactus.length; i++){
smallCactus[i].move();
smallCactus[i].display();
}
}
function removeSmallCactusThatHaveSlippedOutOfView(){ var smallCactusToKeep = [];
for (var i = 0; i < smallCactus.length; i++){
if (smallCactus[i].x + smallCactus[i].breadth > 0) {
smallCactusToKeep.push(smallCactus[i]);
}
}
smallCactus = smallCactusToKeep;}
function addNewSmallCactusWithSomeRandomProbability() {
var newSmallCactusLikelihood = 0.007; if (random(0,1) < newSmallCactusLikelihood) {
smallCactus.push(makeSmallCactus(width));
}
}
function smallCactusMove() {
this.x += this.speed;
}
function smallCactusDisplay() {
fill(130, 240, 120); stroke(50, 210, 90); strokeWeight(1);
push();
translate(this.x, height - 20);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
pop();
}
function makeSmallCactus(birthLocationX) {
var sCact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(20, 80), move: smallCactusMove,
display: smallCactusDisplay}
return sCact;
}
function displayHorizon(){
stroke(255, 210, 80); strokeWeight(20); line (0,height-50, width, height-50); stroke(255, 210, 80, 200);
line(0, height-30, width, height-30); stroke(255, 210, 80, 140);
line(0, height-10, width, height-10);}
function displayStatusString(){
noStroke();
fill(0);
var statusString = "# Cactus = " + cactus.length; var secondString = "# Small Cactus = " + smallCactus.length;
text(statusString, 5,20); text(secondString, 5, 35);
}
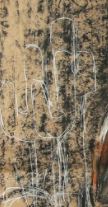
var cactus = [];var smallCactus = [];
function setup() {
createCanvas(640, 240);
for (var i = 0; i < 6; i++){ var rx = random(width); cactus[i] = makeCactus(rx); }
for(var i = 0; i < 8; i++) { var srx = random(width); smallCactus[i] = makeSmallCactus(srx);
}
frameRate(10);}
function draw() {
background(255, 70, 235);
displayStatusString(); displayHorizon();
updateAndDisplayCactus(); removeCactusThatHaveSlippedOutOfView(); addNewCactusWithSomeRandomProbability();
updateAndDisplaySmallCactus(); removeSmallCactusThatHaveSlippedOutOfView();
addNewSmallCactusWithSomeRandomProbability();
}
function updateAndDisplayCactus(){
for (var i = 0; i < cactus.length; i++){
cactus[i].move();
cactus[i].display();
}
}
function removeCactusThatHaveSlippedOutOfView(){
var cactusToKeep = [];
for (var i = 0; i < cactus.length; i++){
if (cactus[i].x + cactus[i].breadth > 0) { cactusToKeep.push(cactus[i]);
}
}
cactus = cactusToKeep;}
function addNewCactusWithSomeRandomProbability() {
var newCactusLikelihood = 0.007;
if (random(0,1) < newCactusLikelihood) { cactus.push(makeCactus(width)); }
}
function cactusMove() {
this.x += this.speed;
}
function cactusDisplay() {
fill(150, 255, 100); stroke(50, 200, 50); strokeWeight(1); push(); translate(this.x, height - 40);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
for(var i = 0; i < this.nArmsLeft; i++) { beginShape();
curveVertex(this.breadth + 120, -i*30 - 10);
curveVertex(this.breadth/2 - 10, -i*30 - 12);
curveVertex(this.breadth/2 - 35, -i*40 - 50);
curveVertex(this.breadth/2 - 15, -i*40 - 45);
curveVertex(this.breadth/2 - 10, -i*30 - 17);
curveVertex(this.breadth + 60, -i*30 - 15);
endShape();
}
for(var i = 0; i < this.nArmsRight; i++) { beginShape();
curveVertex(this.breadth - 120, -i*30 - 10);
curveVertex(this.breadth/2 + 10, -i*30 - 12);
curveVertex(this.breadth/2 + 35, -i*40 - 50);
curveVertex(this.breadth/2 + 15, -i*40 - 45);
curveVertex(this.breadth/2 + 10, -i*30 - 17);
curveVertex(this.breadth - 60, -i*30 - 15);
endShape();
}
pop();}
function makeCactus(birthLocationX) { var cact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(100, 200), nArmsLeft: round(random(0,5)), nArmsRight: round(random(0,5)), move: cactusMove, display: cactusDisplay} return cact;
}
function updateAndDisplaySmallCactus(){ for (var i = 0; i < smallCactus.length; i++){
smallCactus[i].move();
smallCactus[i].display();
}
}
function removeSmallCactusThatHaveSlippedOutOfView(){ var smallCactusToKeep = [];
for (var i = 0; i < smallCactus.length; i++){
if (smallCactus[i].x + smallCactus[i].breadth > 0) {
smallCactusToKeep.push(smallCactus[i]);
}
}
smallCactus = smallCactusToKeep;}
function addNewSmallCactusWithSomeRandomProbability() {
var newSmallCactusLikelihood = 0.007; if (random(0,1) < newSmallCactusLikelihood) {
smallCactus.push(makeSmallCactus(width));
}
}
function smallCactusMove() {
this.x += this.speed;
}
function smallCactusDisplay() {
fill(130, 240, 120); stroke(50, 210, 90); strokeWeight(1);
push();
translate(this.x, height - 20);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
pop();
}
function makeSmallCactus(birthLocationX) {
var sCact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(20, 80), move: smallCactusMove,
display: smallCactusDisplay}
return sCact;
}
function displayHorizon(){
stroke(255, 210, 80); strokeWeight(20); line (0,height-50, width, height-50); stroke(255, 210, 80, 200);
line(0, height-30, width, height-30); stroke(255, 210, 80, 140);
line(0, height-10, width, height-10);}
function displayStatusString(){
noStroke();
fill(0);
var statusString = "# Cactus = " + cactus.length; var secondString = "# Small Cactus = " + smallCactus.length;
text(statusString, 5,20); text(secondString, 5, 35);
}
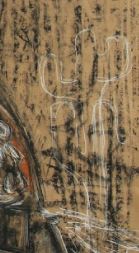
var cactus = [];var smallCactus = [];
function setup() {
createCanvas(640, 240);
for (var i = 0; i < 6; i++){ var rx = random(width); cactus[i] = makeCactus(rx); }
for(var i = 0; i < 8; i++) { var srx = random(width); smallCactus[i] = makeSmallCactus(srx);
}
frameRate(10);}
function draw() {
background(255, 70, 235);
displayStatusString(); displayHorizon();
updateAndDisplayCactus(); removeCactusThatHaveSlippedOutOfView(); addNewCactusWithSomeRandomProbability();
updateAndDisplaySmallCactus(); removeSmallCactusThatHaveSlippedOutOfView();
addNewSmallCactusWithSomeRandomProbability();
}
function updateAndDisplayCactus(){
for (var i = 0; i < cactus.length; i++){
cactus[i].move();
cactus[i].display();
}
}
function removeCactusThatHaveSlippedOutOfView(){
var cactusToKeep = [];
for (var i = 0; i < cactus.length; i++){
if (cactus[i].x + cactus[i].breadth > 0) { cactusToKeep.push(cactus[i]);
}
}
cactus = cactusToKeep;}
function addNewCactusWithSomeRandomProbability() {
var newCactusLikelihood = 0.007;
if (random(0,1) < newCactusLikelihood) { cactus.push(makeCactus(width)); }
}
function cactusMove() {
this.x += this.speed;
}
function cactusDisplay() {
fill(150, 255, 100); stroke(50, 200, 50); strokeWeight(1); push(); translate(this.x, height - 40);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
for(var i = 0; i < this.nArmsLeft; i++) { beginShape();
curveVertex(this.breadth + 120, -i*30 - 10);
curveVertex(this.breadth/2 - 10, -i*30 - 12);
curveVertex(this.breadth/2 - 35, -i*40 - 50);
curveVertex(this.breadth/2 - 15, -i*40 - 45);
curveVertex(this.breadth/2 - 10, -i*30 - 17);
curveVertex(this.breadth + 60, -i*30 - 15);
endShape();
}
for(var i = 0; i < this.nArmsRight; i++) { beginShape();
curveVertex(this.breadth - 120, -i*30 - 10);
curveVertex(this.breadth/2 + 10, -i*30 - 12);
curveVertex(this.breadth/2 + 35, -i*40 - 50);
curveVertex(this.breadth/2 + 15, -i*40 - 45);
curveVertex(this.breadth/2 + 10, -i*30 - 17);
curveVertex(this.breadth - 60, -i*30 - 15);
endShape();
}
pop();}
function makeCactus(birthLocationX) { var cact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(100, 200), nArmsLeft: round(random(0,5)), nArmsRight: round(random(0,5)), move: cactusMove, display: cactusDisplay} return cact;
}
function updateAndDisplaySmallCactus(){ for (var i = 0; i < smallCactus.length; i++){
smallCactus[i].move();
smallCactus[i].display();
}
}
function removeSmallCactusThatHaveSlippedOutOfView(){ var smallCactusToKeep = [];
for (var i = 0; i < smallCactus.length; i++){
if (smallCactus[i].x + smallCactus[i].breadth > 0) {
smallCactusToKeep.push(smallCactus[i]);
}
}
smallCactus = smallCactusToKeep;}
function addNewSmallCactusWithSomeRandomProbability() {
var newSmallCactusLikelihood = 0.007; if (random(0,1) < newSmallCactusLikelihood) {
smallCactus.push(makeSmallCactus(width));
}
}
function smallCactusMove() {
this.x += this.speed;
}
function smallCactusDisplay() {
fill(130, 240, 120); stroke(50, 210, 90); strokeWeight(1);
push();
translate(this.x, height - 20);
beginShape(); curveVertex(this.breadth/2 - 10, 0);
curveVertex(this.breadth/2 - 10, 0);
curveVertex(0, -this.cHeight);
curveVertex(this.breadth, -this.cHeight);
curveVertex(this.breadth/2 + 10, 0);
curveVertex(this.breadth/2 + 10, 0);
endShape();
pop();
}
function makeSmallCactus(birthLocationX) {
var sCact = {x: birthLocationX,
breadth: random(20, 50), speed: -1.0,
cHeight: random(20, 80), move: smallCactusMove,
display: smallCactusDisplay}
return sCact;
}
function displayHorizon(){
stroke(255, 210, 80); strokeWeight(20); line (0,height-50, width, height-50); stroke(255, 210, 80, 200);
line(0, height-30, width, height-30); stroke(255, 210, 80, 140);
line(0, height-10, width, height-10);}
function displayStatusString(){
noStroke();
fill(0);
var statusString = "# Cactus = " + cactus.length; var secondString = "# Small Cactus = " + smallCactus.length;
text(statusString, 5,20); text(secondString, 5, 35);
}
My process for this project was based on a previous drawing I have done with cacti. To me, these are significant structures because they exist in some isolation from each other by their spread and spacing in the desert, away from clusters of other living organisms, so their visual existence is very extraordinary. To create the cacti in p5, I started with creating a curve shape as the base of the cactus. Then, I randomized the number of arms present on the cactus’s body, where I had to play around with trial and error in creating a curve shape which looked like a cactus arm, but also that was attached to the cactus’s main body. I then, played with creating other objects like the smaller, darker cactus and its visual randomization and its positioning on the second horizon line lower than the first, larger, lighter cactus which was on the first horizon line.