//Hae Wan Park
//15104-A
//haewanp@andrew.cmu.edu
//Project-02-variable-Face
var eyeSize = 15;
var x = 10;
var y = 9;
var t = 5;
var faceY = 5;
var faceX = 3;
var color1 = 0;
var color2 = 150;
var color3 = 240;
var eyeX = 240;
var eyeY = 310;
var n = 10;
var v = 0;
function setup() {
createCanvas(480, 640);
}
function draw() {
background(252);
//hair
noStroke();
fill(255 - color1, 235, 70 + color1);
beginShape();
curveVertex(170, 220);
curveVertex(width / 2 - (faceX+9)*x, 210);
curveVertex(170, height / 2 - 3*y*faceY - 7*faceY); //
curveVertex(230, height / 2 - 3*y*faceY - 7*faceY); //
curveVertex(width / 2 + (faceX+5)*x, height / 2 - 3*y*faceY - 7*faceY);
curveVertex(width / 2 + (faceX+6.5)*x, 195);
curveVertex(305, 230);
curveVertex(270, 215);
curveVertex(265, height / 2 - 20*faceY);
curveVertex(285, height / 2 - 14*faceY);
curveVertex(240, height / 2 - 12*faceY);
curveVertex(220, height / 2 - 18*faceY);
curveVertex(210, 210);
curveVertex(200, 230);
curveVertex(180, 235);
curveVertex(170, 220);
curveVertex(width / 2 - (faceX+9)*x, 210);
curveVertex(170, height / 2 - 3*y*faceY - 7*faceY);
endShape();
//cheek
noStroke();
fill(255 - color1/2, color2 - 50, 210 - color1);
ellipse(width/2 + 70, 350, 8*x, 8*x);
ellipse(width/2 - 80, 350, 8*x, 8*x);
//face
noFill();
stroke(color1, color2 - 40, 255 - color1);
strokeWeight(4.5);
curveTightness(0);
beginShape();
curveVertex(width / 2 - (faceX+5)*x, height / 2 - (faceY+8)*y);
curveVertex(width / 2 - (faceX+1)*x, height / 2 - 3.2*y*faceY);
curveVertex(width / 2 - (faceX-5)*x, height / 2 - 3.2*y*faceY); //
curveVertex(width / 2 - (faceX-10.5)*x, height / 2 - 2.5*y*faceY);
curveVertex(width / 2 + (faceX+7)*x, height / 2 - faceY*y);
curveVertex(width / 2 + (faceX+5)*x, height / 2 + 9*y);
curveVertex(width / 2 + (faceX-2)*x, height / 2 + 15*y);
curveVertex(width / 2 - (faceX+4)*x, height / 2 + 12*y);
curveVertex(width / 2 - (faceX+7)*x, height / 2 + faceY*y);
curveVertex(width / 2 - (faceX+8)*x, height / 2 - faceY*y);
curveVertex(width / 2 - (faceX+6)*x, height / 2 - (faceY+8)*y);
curveVertex(width / 2 - (faceX+1)*x, height / 2 - 3.2*y*faceY);
curveVertex(width / 2 - (faceX-5)*x, height / 2 - 3.2*y*faceY);
endShape();
//ear
ellipse(width / 2 - (faceX+8.5)*x, height / 2 - (faceY-3)*y, 30, 40);
ellipse(width / 2 + (faceX+7)*x, height / 2 - (faceY-3)*y, 30, 40);
//eye
ellipse(205 - faceY, eyeY - 35 + eyeSize/2, eyeSize, eyeSize);
ellipse(275 + faceY, eyeY - 35 + eyeSize/2, eyeSize, eyeSize);
stroke(255, color2 - 55, color2 - 40);
curve(175 + faceY*(faceX/8), eyeY + 60 + 2*faceY,
175 + faceY*(faceX/8), eyeY - 40 + 2*faceY,
225 - faceY, eyeY - 40 + 2*faceY,
225 - faceY, eyeY + 60 + 2*faceY);
curve(255 + faceY, eyeY + 60 + 2*faceY,
255 + faceY, eyeY - 40 + 2*faceY,
305 - faceY*(faceX/8), eyeY - 40 + 2*faceY,
305 - faceY*(faceX/8), eyeY + 60 + 2*faceY);
//eyebrow
line(width/2 - 55, eyeY - 70 + 7*v, width/2 - 25, eyeY - 60 + v);
line(width/2 + 55, eyeY - 70 + 7*v, width/2 + 25, eyeY - 60 + v);
//nose
line(width / 2, 325, 240, 305);
line(width / 2, 325, width / 2 - 2*n, 337);
line(width / 2 - 2*n, 337, width / 2, 351);
//mouth
strokeJoin(ROUND);
beginShape();
vertex(width / 2 + 5*faceX, 390);
vertex(width / 2 - 5*faceX - 6, 390);
vertex(width / 2 - 3*faceX, 380);
vertex(width / 2, 385);
vertex(width / 2 + 10, 380);
vertex(width / 2 + 5*faceX, 390);
vertex(width / 2 - 3, 405);
vertex(width / 2 - 5*faceX - 6, 390);
endShape();
}
function mousePressed() {
eyeSize = random(10, 25);
eyeX = random (220, 250);
eyeY = random (300, 320);
x = random(8,12);
y = random(7,10);
t = random(7,20);
faceY = random(5,7);
faceX = random(3,5);
color1 = random(0, 85);
color2 = random(85, 170);
color3 = random(170, 255);
n = random(6,12);
v = random(-2,4);
}
It is fun to watch the face keeps changing. It was not easy to make everything in right proportion. Also, I sketched on illustrator then tried to figure out how to draw with p5.js. It has to be simplified even though I thought my sketch was not so complicated. Maybe need more practice to control p5.js.
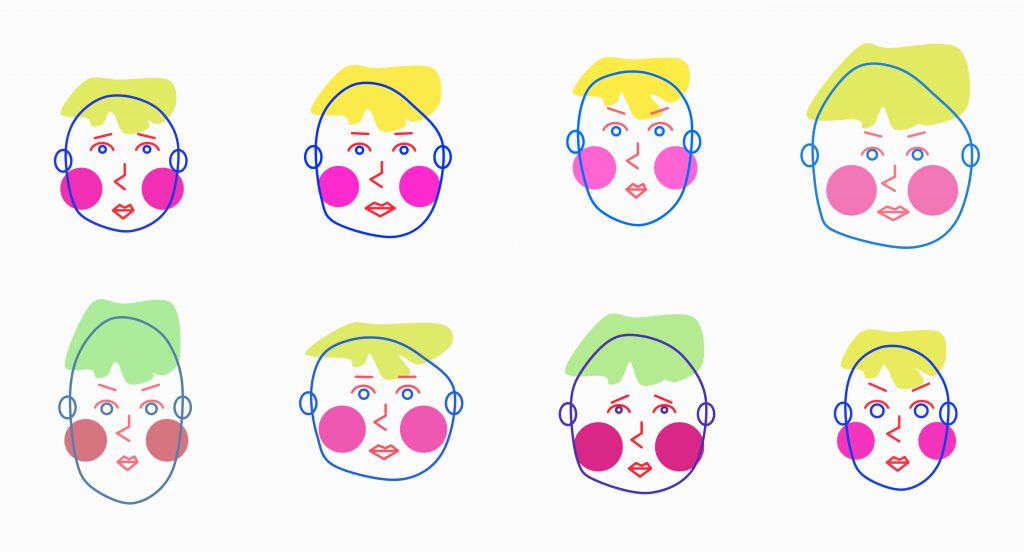
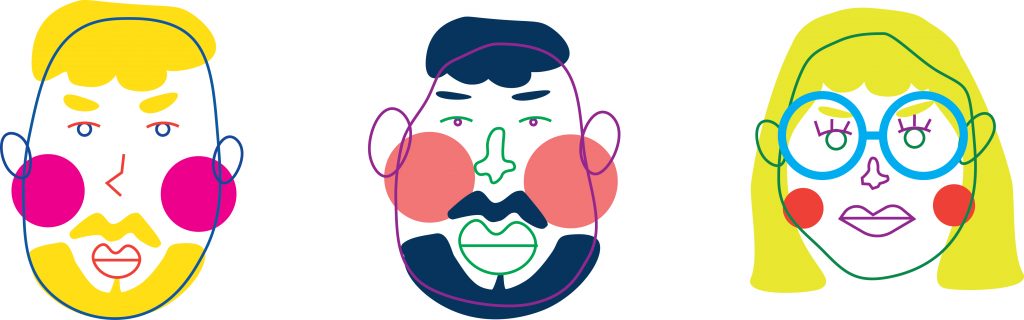