I definitely learned the usefulness of turtles when it comes to both dashes and curves.
After fiddling around with turtle movement, I accidentally created rotating clock hands and decided to base my experiment on it.
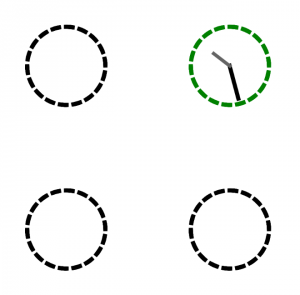
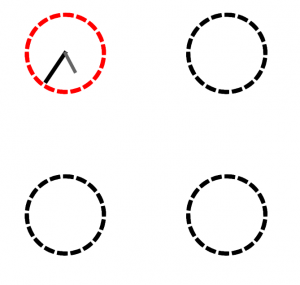
sketch
var t1;
var t2;
//Two turtles used for clock hands
var tC1;
var tC2;
var tC3;
var tC4;
//Four turtles used for the circles
var t = 6;
//Simple incremental distance for convenience
function setup() {
createCanvas(480, 480);
t1 = makeTurtle(0, 0);
t2 = makeTurtle(0, 0);
//initialize both clock hand turtles
tC1 = makeTurtle(0, 0);
tC2 = makeTurtle(0, 0);
tC3 = makeTurtle(0, 0);
tC4 = makeTurtle(0, 0);
//initialize the four circle turtles
}
function draw() {
background(255);
strokeJoin(MITER);
strokeCap(PROJECT);
makeCirclesAndHands(1, 1, "red", tC1);
makeCirclesAndHands(3, 1, "green", tC2);
makeCirclesAndHands(1, 3, "blue", tC3);
makeCirclesAndHands(3, 3, "yellow", tC4);
//Makes all four circles and the conditional clock hand situations
}
function hand(turtle, length, speed, x, y, color, thick) {
turtle.penUp();
turtle.goto(x, y);
turtle.penDown();
turtle.forward(length);
turtle.right(speed);
turtle.setColor(color);
turtle.setWeight(thick);
//Makes a clock hand that rotates around x,y and has
//a bunch of other variables tha control the appearance and movement
}
function circley(turtle, x, y, color) {
//Makes a dashed circle at x,y with a
// color, using a specific turtle by
// moving a given distance on the curve with
// the pen down, then again with the pen up
// then repeating that penUp penDown process
// until the full circle is completed
turtle.setColor(color);
turtle.face(0);
turtle.setWeight(6);
turtle.goto(x,y);
for (var p = 0; p < 18; p++) {
for (var l = 0; l < 10; l++) {
turtle.penDown();
turtle.forward(1);
turtle.right(1);
}
for (var i = 0; i < 10; i++) {
turtle.penUp();
turtle.forward(1);
turtle.right(1);
}
}
}
function distance(x1,y1,x2,y2) {
return sqrt((x2-x1)*(x2-x1) + (y2-y1)*(y2-y1));
}
//Simple distance formula for use in calculation
function makeCirclesAndHands(widthMultiplier, heightMultiplier, colorChange, circleTurtle) {
//Creates four dashed line circles that, if you put the mouse
//inside them, change color depending on the circle and place
//spinning clock hands within the circle
if (distance(mouseX, mouseY, widthMultiplier*width/4+7, heightMultiplier*height/4+7) < 50) {
circley(circleTurtle, widthMultiplier*width/4, heightMultiplier*height/4 - 50, colorChange);
hand(t1, 50, 1, widthMultiplier*width/4,heightMultiplier*height/4+7, 0, t);
hand(t2, 30, 0.5, widthMultiplier*width/4,heightMultiplier*height/4+7, 100, t-1);
} else {
circley(circleTurtle, widthMultiplier*width/4, heightMultiplier*height/4 - 50, 0);
}
}
//Compressed turtle stuff
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}