sketch
// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section
// Project-06- Abstract Clock
var bgColmin = (0, 255, 255);
var bgColmax = (92, 26, 102);
// X and Y positions of the lights controlled by the minutes
var xarray = [62, 73, 98, 86, 150, 170, 175, 102, 95, 62, 293, 298, 287, 367, 31, 70, 177, 203, 265, 278, 82, 55, 85, 94, 142, 151, 176, 83, 59, 60, 147, 177,
93, 80, 68, 64, 373, 320, 296, 270, 315, 309, 267, 200, 98, 84, 40, 98, 108, 293, 301, 377, 354, 257, 197, 169, 205, 292, 232, 173];
var yarray = [47, 78, 116, 50, 202, 217, 191, 104, 88, 280, 195, 252, 155, 253, 320,303, 235, 270, 296, 312, 132, 110, 75, 97, 289, 251, 319, 149, 150, 176, 230, 261,
172, 192, 248, 194, 230, 261, 324, 310, 174, 184, 217, 216, 235, 211, 312, 287, 293, 324, 292, 280, 298, 317,267, 260, 283, 290, 313, 329, 318];
function setup() {
createCanvas(400, 400);
}
function draw() {
var milli = map(millis(), 0, 999, -35, width + 35);
var sec = map(second(), 0, 59, -35, width + 35);
var min = map(minute(), 0, 58, 0, 255);
var hrND = map(hour(), 0, 11, bgColmax, bgColmin);
var hrDN = map(hour(), 12, 23, bgColmin, bgColmax);
// var hrMoon = map(hour(), 0, 11, 250, Sun);
// var hrSun = map(hour(), 12, 23, Sun, 250);
var timeND = {r:min, g:sec, b:hrND};
var timeDN = {r:min, g:sec, b:hrDN};
// var timeSun = {r:min, g:sec, b:hrSun};
// var timeMoon = {r:min, g:sec, b:hrMoon};
var hrNDColor = color(0, 0, timeND.b);
var hrDNColor = color(0, 0, timeDN.b);
// var hrSunColor = color(timeSun.b, timeSun.b, 0);
// var hrMoonColor = color(timeMoon.b, timeMoon.b, 0);
var gray = color(240);
// Maps the sky/background color to the hours of the day
// Simulating dark to light, and light to dark depending on the time of day
for(var c = 0; c <= height; c+=5){
var amt = map(c, 0, height, 0, 1);
var background = lerpColor(hrNDColor, gray, amt);
fill(background);
rect(0, c, width, 5);
if(int(hour()) >= 12){
hrNDColor = hrDNColor;
}
}
// Draws Sun/Moon
fill(200, 170, 0);
ellipse(width, 0, 175, 175);
// Calls blimp function; Blimp movement contolled by seconds
blimp(sec, height/10);
// Draws Skyline
fill(0);
noStroke();
strokeJoin(MITER);
beginShape();
vertex(0, 400); vertex(0, 306); vertex(4, 306);
vertex(4, 292); vertex(31, 292); vertex(31, 282);
vertex(50, 282); vertex(50, 30); vertex(60, 30);
vertex(60, 20); vertex(103, 20); vertex(103, 30);
vertex(116, 30); vertex(116, 282); vertex(128, 282);
vertex(128, 304); vertex(132, 304); vertex(132, 268);
vertex(140, 268); vertex(140, 190); vertex(169, 190);
vertex(169, 185); vertex(189, 185); vertex(189, 226);
vertex(202, 226); vertex(206, 185); vertex(208, 226);
vertex(216, 226); vertex(216, 216); vertex(226, 216);
vertex(226, 226); vertex(232, 226); vertex(232, 306);
vertex(244, 306); vertex(244, 199); vertex(252, 199);
vertex(252, 139); vertex(260, 139); vertex(260, 127);
vertex(295, 127); vertex(295, 139); vertex(305, 139);
vertex(305, 170); vertex(330, 170); vertex(330, 306);
vertex(365, 306); vertex(365, 250); vertex(390, 250);
vertex(390, 306); vertex(400, 306); vertex(400, 400);
endShape();
// Draw a new light every minute
for(var i = 0; i < int(minute()); i++){
fill(200, 200, 0);
rect(xarray[i], yarray[i], 7, 7);
}
}
// Draws Blimp
function blimp(x, y){
fill(200, 75, 175);
quad(x - 20, y, x + 15, y, x + 7, y + 23, x -17, y + 23);
fill(75)
ellipse(x, y, 70, 35);
triangle(x - 45, y -15, x - 25, y, x - 45, y + 15);
fill(200, 75, 175);
ellipse(x, y, 68, 15);
}
For this project I was aiming for something seemingly realistic. The background/sky color is controlled by the hours, moving from dark to light and vice versa as you move throughout the day. Every minute a light switches on in the building, and a blimp moves across the screen a certain distance every second.
It was somewhat challenging, but I learnt a lot from the experience.
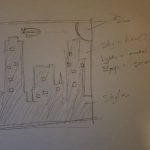
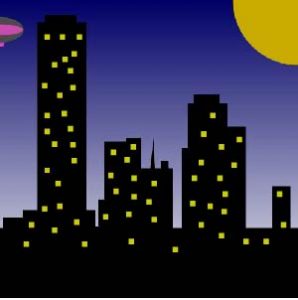
// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section
// Project-06- Abstract Clock
var bgColmin = (0, 255, 255);
var bgColmax = (92, 26, 102);
var xarray = [55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70,
100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305];
var yarray = [306, 306, 306, 285, 285, 285, 285, 285, 270, 270, 285, 285, 285, 270, 265, 275, 250, 250, 250, 270, 190, 190, 190, 200, 200, 215, 235, 235, 180, 180, 50, 50,
50, 265, 300, 290, 255, 285, 175, 175, 100, 100, 110, 255, 235, 240, 295, 260, 160, 160, 145, 132, 149, 240, 272, 300, 306, 210, 150, 150];
function setup() {
createCanvas(400, 400);
}
function draw() {
var milli = map(millis(), 0, 999, -35, width + 35);
var sec = map(second(), 0, 59, -35, width + 35);
var min = map(minute(), 0, 58, 0, 255);
var hrND = map(hour(), 0, 11, bgColmax, bgColmin);
var hrDN = map(hour(), 12, 23, bgColmin, bgColmax);
// var hrMoon = map(hour(), 0, 11, 250, Sun);
// var hrSun = map(hour(), 12, 23, Sun, 250);
var timeND = {r:min, g:sec, b:hrND};
var timeDN = {r:min, g:sec, b:hrDN};
// var timeSun = {r:min, g:sec, b:hrSun};
// var timeMoon = {r:min, g:sec, b:hrMoon};
var hrNDColor = color(0, 0, timeND.b);
var hrDNColor = color(0, 0, timeDN.b);
// var hrSunColor = color(timeSun.b, timeSun.b, 0);
// var hrMoonColor = color(timeMoon.b, timeMoon.b, 0);
var gray = color(240);
// Maps the sky/background color to the hours of the day
// Simulating dark to light, and light to dark depending on the time of day
for(var c = 0; c <= height; c+=5){
var amt = map(c, 0, height, 0, 1);
var background = lerpColor(hrNDColor, gray, amt);
fill(background);
rect(0, c, width, 5);
if(int(hour()) >= 12){
hrNDColor = hrDNColor;
}
}
// Draws Sun/Moon
fill(200, 170, 0);
ellipse(width, 0, 175, 175);
// Calls blimp function; Blimp movement contolled by seconds
blimp(sec, height/10);
// Draws Skyline
fill(0);
noStroke();
strokeJoin(MITER);
beginShape();
vertex(0, 400); vertex(0, 306); vertex(4, 306);
vertex(4, 292); vertex(31, 292); vertex(31, 282);
vertex(50, 282); vertex(50, 30); vertex(60, 30);
vertex(60, 20); vertex(103, 20); vertex(103, 30);
vertex(116, 30); vertex(116, 282); vertex(128, 282);
vertex(128, 304); vertex(132, 304); vertex(132, 268);
vertex(140, 268); vertex(140, 190); vertex(169, 190);
vertex(169, 185); vertex(189, 185); vertex(189, 226);
vertex(202, 226); vertex(206, 185); vertex(208, 226);
vertex(216, 226); vertex(216, 216); vertex(226, 216);
vertex(226, 226); vertex(232, 226); vertex(232, 306);
vertex(244, 306); vertex(244, 199); vertex(252, 199);
vertex(252, 139); vertex(260, 139); vertex(260, 127);
vertex(295, 127); vertex(295, 139); vertex(305, 139);
vertex(305, 170); vertex(330, 170); vertex(330, 306);
vertex(365, 306); vertex(365, 250); vertex(390, 250);
vertex(390, 306); vertex(400, 306); vertex(400, 400);
endShape();
for(var i = 0; i < int(minute()); i++){
fill(200, 200, 0);
rect(xarray[i], yarray[i], 7, 7);
}
}
// Draws Blimp
function blimp(x, y){
fill(200, 75, 175);
quad(x - 20, y, x + 15, y, x + 7, y + 23, x -17, y + 23);
fill(75)
ellipse(x, y, 70, 35);
triangle(x - 45, y -15, x - 25, y, x - 45, y + 15);
fill(200, 75, 175);
ellipse(x, y, 68, 15);
}
// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section
// Project-06- Abstract Clock
var bgColmin = (0, 255, 255);
var bgColmax = (92, 26, 102);
var xarray = [55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70,
100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305];
var yarray = [306, 306, 306, 285, 285, 285, 285, 285, 270, 270, 285, 285, 285, 270, 265, 275, 250, 250, 250, 270, 190, 190, 190, 200, 200, 215, 235, 235, 180, 180, 50, 50,
50, 265, 300, 290, 255, 285, 175, 175, 100, 100, 110, 255, 235, 240, 295, 260, 160, 160, 145, 132, 149, 240, 272, 300, 306, 210, 150, 150];
function setup() {
createCanvas(400, 400);
}
function draw() {
var milli = map(millis(), 0, 999, -35, width + 35);
var sec = map(second(), 0, 59, -35, width + 35);
var min = map(minute(), 0, 58, 0, 255);
var hrND = map(hour(), 0, 11, bgColmax, bgColmin);
var hrDN = map(hour(), 12, 23, bgColmin, bgColmax);
// var hrMoon = map(hour(), 0, 11, 250, Sun);
// var hrSun = map(hour(), 12, 23, Sun, 250);
var timeND = {r:min, g:sec, b:hrND};
var timeDN = {r:min, g:sec, b:hrDN};
// var timeSun = {r:min, g:sec, b:hrSun};
// var timeMoon = {r:min, g:sec, b:hrMoon};
var hrNDColor = color(0, 0, timeND.b);
var hrDNColor = color(0, 0, timeDN.b);
// var hrSunColor = color(timeSun.b, timeSun.b, 0);
// var hrMoonColor = color(timeMoon.b, timeMoon.b, 0);
var gray = color(240);
// Maps the sky/background color to the hours of the day
// Simulating dark to light, and light to dark depending on the time of day
for(var c = 0; c <= height; c+=5){
var amt = map(c, 0, height, 0, 1);
var background = lerpColor(hrNDColor, gray, amt);
fill(background);
rect(0, c, width, 5);
if(int(hour()) >= 12){
hrNDColor = hrDNColor;
}
}
// Draws Sun/Moon
fill(200, 170, 0);
ellipse(width, 0, 175, 175);
// Calls blimp function; Blimp movement contolled by seconds
blimp(sec, height/10);
// Draws Skyline
fill(0);
noStroke();
strokeJoin(MITER);
beginShape();
vertex(0, 400); vertex(0, 306); vertex(4, 306);
vertex(4, 292); vertex(31, 292); vertex(31, 282);
vertex(50, 282); vertex(50, 30); vertex(60, 30);
vertex(60, 20); vertex(103, 20); vertex(103, 30);
vertex(116, 30); vertex(116, 282); vertex(128, 282);
vertex(128, 304); vertex(132, 304); vertex(132, 268);
vertex(140, 268); vertex(140, 190); vertex(169, 190);
vertex(169, 185); vertex(189, 185); vertex(189, 226);
vertex(202, 226); vertex(206, 185); vertex(208, 226);
vertex(216, 226); vertex(216, 216); vertex(226, 216);
vertex(226, 226); vertex(232, 226); vertex(232, 306);
vertex(244, 306); vertex(244, 199); vertex(252, 199);
vertex(252, 139); vertex(260, 139); vertex(260, 127);
vertex(295, 127); vertex(295, 139); vertex(305, 139);
vertex(305, 170); vertex(330, 170); vertex(330, 306);
vertex(365, 306); vertex(365, 250); vertex(390, 250);
vertex(390, 306); vertex(400, 306); vertex(400, 400);
endShape();
for(var i = 0; i < int(minute()); i++){
fill(200, 200, 0);
rect(xarray[i], yarray[i], 7, 7);
}
}
// Draws Blimp
function blimp(x, y){
fill(200, 75, 175);
quad(x - 20, y, x + 15, y, x + 7, y + 23, x -17, y + 23);
fill(75)
ellipse(x, y, 70, 35);
triangle(x - 45, y -15, x - 25, y, x - 45, y + 15);
fill(200, 75, 175);
ellipse(x, y, 68, 15);
}
// Emmanuel Nwandu
// enwandu@andrew.cmu.edu
// Section
// Project-06- Abstract Clock
var bgColmin = (0, 255, 255);
var bgColmax = (92, 26, 102);
var xarray = [55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70,
100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305, 55, 70, 100, 150, 175, 190, 207, 228, 262, 305];
var yarray = [306, 306, 306, 285, 285, 285, 285, 285, 270, 270, 285, 285, 285, 270, 265, 275, 250, 250, 250, 270, 190, 190, 190, 200, 200, 215, 235, 235, 180, 180, 50, 50,
50, 265, 300, 290, 255, 285, 175, 175, 100, 100, 110, 255, 235, 240, 295, 260, 160, 160, 145, 132, 149, 240, 272, 300, 306, 210, 150, 150];
function setup() {
createCanvas(400, 400);
}
function draw() {
var milli = map(millis(), 0, 999, -35, width + 35);
var sec = map(second(), 0, 59, -35, width + 35);
var min = map(minute(), 0, 58, 0, 255);
var hrND = map(hour(), 0, 11, bgColmax, bgColmin);
var hrDN = map(hour(), 12, 23, bgColmin, bgColmax);
// var hrMoon = map(hour(), 0, 11, 250, Sun);
// var hrSun = map(hour(), 12, 23, Sun, 250);
var timeND = {r:min, g:sec, b:hrND};
var timeDN = {r:min, g:sec, b:hrDN};
// var timeSun = {r:min, g:sec, b:hrSun};
// var timeMoon = {r:min, g:sec, b:hrMoon};
var hrNDColor = color(0, 0, timeND.b);
var hrDNColor = color(0, 0, timeDN.b);
// var hrSunColor = color(timeSun.b, timeSun.b, 0);
// var hrMoonColor = color(timeMoon.b, timeMoon.b, 0);
var gray = color(240);
// Maps the sky/background color to the hours of the day
// Simulating dark to light, and light to dark depending on the time of day
for(var c = 0; c <= height; c+=5){
var amt = map(c, 0, height, 0, 1);
var background = lerpColor(hrNDColor, gray, amt);
fill(background);
rect(0, c, width, 5);
if(int(hour()) >= 12){
hrNDColor = hrDNColor;
}
}
// Draws Sun/Moon
fill(200, 170, 0);
ellipse(width, 0, 175, 175);
// Calls blimp function; Blimp movement contolled by seconds
blimp(sec, height/10);
// Draws Skyline
fill(0);
noStroke();
strokeJoin(MITER);
beginShape();
vertex(0, 400); vertex(0, 306); vertex(4, 306);
vertex(4, 292); vertex(31, 292); vertex(31, 282);
vertex(50, 282); vertex(50, 30); vertex(60, 30);
vertex(60, 20); vertex(103, 20); vertex(103, 30);
vertex(116, 30); vertex(116, 282); vertex(128, 282);
vertex(128, 304); vertex(132, 304); vertex(132, 268);
vertex(140, 268); vertex(140, 190); vertex(169, 190);
vertex(169, 185); vertex(189, 185); vertex(189, 226);
vertex(202, 226); vertex(206, 185); vertex(208, 226);
vertex(216, 226); vertex(216, 216); vertex(226, 216);
vertex(226, 226); vertex(232, 226); vertex(232, 306);
vertex(244, 306); vertex(244, 199); vertex(252, 199);
vertex(252, 139); vertex(260, 139); vertex(260, 127);
vertex(295, 127); vertex(295, 139); vertex(305, 139);
vertex(305, 170); vertex(330, 170); vertex(330, 306);
vertex(365, 306); vertex(365, 250); vertex(390, 250);
vertex(390, 306); vertex(400, 306); vertex(400, 400);
endShape();
for(var i = 0; i < int(minute()); i++){
fill(200, 200, 0);
rect(xarray[i], yarray[i], 7, 7);
}
}
// Draws Blimp
function blimp(x, y){
fill(200, 75, 175);
quad(x - 20, y, x + 15, y, x + 7, y + 23, x -17, y + 23);
fill(75)
ellipse(x, y, 70, 35);
triangle(x - 45, y -15, x - 25, y, x - 45, y + 15);
fill(200, 75, 175);
ellipse(x, y, 68, 15);
}