project6
var A = [196,210,227,141,156,168,183,245,260,204,190,177,222,239, 163, 150, 250, 199, 186, 213, 231, 172, 243, 159, 195, 209, 229, 182, 239, 168, 204, 220, 190, 176, 233, 205, 221, 191, 177, 235,195, 211, 181, 224,199, 213, 184, 226, 210, 198, 184, 202, 190, 217, 186, 199, 213, 191, 206, 200]
var B = [327,327,327,327,327,327,327,327,327,324,324,324,324,324,324,324,324, 319,319,319,319,319,319,319, 314, 314,314,314,314,314, 310,310,310,310,310, 305,305,305,305,305, 303,303,303,303, 298,298,298,298, 294,294,294, 290,290,290, 285,285,285, 280,280, 275];
function setup() {
createCanvas(400, 400);
}
function draw() {
background(255);
var S = second();
var M = minute();
var H = hour();
var mShake = map(S, 0, 60, 0, 1.5);
var shake = random(-mShake, mShake)
expandX = map(M, 0, 60, 0, 100 )
expandY = map(M, 0, 60, 0, 30)
if (H >= 0 & H <= 12){
hourMap = map(H, 0, 12, 0, 255)
}
if (H >= 12 && H <= 24){
hourMap = map(H, 12, 24, 255, 0)
}
beginShape();
fill(hourMap);
noStroke();
curveVertex(91-expandX, 400);
curveVertex(91-expandX, 400);
curveVertex(111-expandX*0.8, 217);
curveVertex(129-expandX*0.65, 96);
curveVertex(178-expandX*0.4, 57-expandY);
curveVertex(218+expandX*0.4, 59-expandY);
curveVertex(270+expandX*0.65, 96);
curveVertex(286+expandX*0.8, 217);
curveVertex(309+expandX, 400);
curveVertex(309+expandX, 400);
endShape();
push();
beginShape();
fill(221, 231, 229)
noStroke();
curveVertex(136, 112);
curveVertex(136, 112);
curveVertex(157, 83);
curveVertex(190, 69);
curveVertex(239, 85);
curveVertex(259, 131);
curveVertex(241, 254);
curveVertex(182, 275);
curveVertex(146, 237);
curveVertex(131, 167);
curveVertex(136, 112);
curveVertex(136, 112);
endShape();
pop();
beginShape();
fill(255)
noStroke();
curveVertex(196, 80);
curveVertex(196, 80);
curveVertex(228, 92);
curveVertex(249, 139);
curveVertex(244, 203);
curveVertex(235, 240);
curveVertex(226, 256);
curveVertex(202, 272);
curveVertex(174, 270);
curveVertex(150, 240);
curveVertex(139, 205);
curveVertex(135, 161);
curveVertex(139, 120);
curveVertex(161, 85);
curveVertex(196, 80);
curveVertex(196, 80);
endShape();
beginShape();
fill(158, 133, 189);
noStroke();
curveVertex(160, 105);
curveVertex(160, 105);
curveVertex(165, 105)
curveVertex(168, 118);
curveVertex(170, 129)
curveVertex(168, 137);
curveVertex(161, 140);
curveVertex(153, 138);
curveVertex(150, 133)
curveVertex(151, 125);
curveVertex(154, 117);
curveVertex(160, 105);
curveVertex(160, 105);
endShape();
beginShape();
fill(158, 133, 189);
noStroke();
curveVertex(230, 105);
curveVertex(230, 105);
curveVertex(237, 112)
curveVertex(245, 131);
curveVertex(242, 139)
curveVertex(236, 141);
curveVertex(230, 140);
curveVertex(225, 136);
curveVertex(225, 123)
curveVertex(226, 113);
curveVertex(230, 105);
curveVertex(230, 105);
endShape();
fill(0);
beginShape();
push();
noStroke();
frameRate(1);
curveVertex(146+shake, 152);
curveVertex(146+shake, 152);
curveVertex(152+shake, 149);
curveVertex(163+shake, 147);
curveVertex(172+shake, 151);
curveVertex(175+shake, 157);
curveVertex(170+shake, 162);
curveVertex(159+shake, 164);
curveVertex(145+shake, 159);
curveVertex(146+shake, 152);
curveVertex(146+shake, 152);
pop();
endShape();
beginShape();
fill(0);
frameRate(1);
noStroke();
curveVertex(233+shake, 147);
curveVertex(233, 147+shake);
curveVertex(249+shake, 149);
curveVertex(254, 159+shake);
curveVertex(244+shake, 164);
curveVertex(232, 164+shake);
curveVertex(224+shake, 159);
curveVertex(222, 154+shake);
curveVertex(225+shake, 150);
curveVertex(233, 147+shake);
curveVertex(233+shake, 147);
endShape();
beginShape();
fill(131);
noStroke();
curveVertex(161, 170);
curveVertex(161, 170);
curveVertex(171, 168);
curveVertex(172, 168);
curveVertex(164, 175);
curveVertex(149, 172);
curveVertex(148, 170);
curveVertex(161, 170);
curveVertex(161, 170);
endShape();
beginShape();
fill(131);
noStroke();
curveVertex(235, 169);
curveVertex(235, 169);
curveVertex(246, 169);
curveVertex(247, 171);
curveVertex(235, 174);
curveVertex(227, 173);
curveVertex(223, 170);
curveVertex(225, 168);
curveVertex(235, 169);
curveVertex(235, 169);
endShape();
beginShape();
fill(158, 133, 189);
noStroke();
curveVertex(151, 188);
curveVertex(151, 188);
curveVertex(153, 182)
curveVertex(157, 180);
curveVertex(169, 185)
curveVertex(170, 210);
curveVertex(170, 218);
curveVertex(168, 226);
curveVertex(165, 226);
curveVertex(158, 214);
curveVertex(151, 188);
curveVertex(151, 188);
endShape();
beginShape();
fill(158, 133, 189);
noStroke();
curveVertex(242, 190);
curveVertex(242, 190);
curveVertex(239, 207)
curveVertex(231, 226);
curveVertex(226, 224)
curveVertex(224, 190);
curveVertex(230, 180);
curveVertex(238, 181);
curveVertex(242, 190);
curveVertex(242, 190);
endShape();
beginShape();
fill(158, 133, 189);
noStroke();
curveVertex(198, 256);
curveVertex(198, 256);
curveVertex(208, 255)
curveVertex(210, 256);
curveVertex(199, 263)
curveVertex(189, 261);
curveVertex(185, 257);
curveVertex(186, 255);
curveVertex(198, 256);
curveVertex(198, 256);
endShape();
if (S>57){
push();
heart(0,0);
heart(77, 0);
pop();
}
var mouthExpandx= 0;
var mouthExpandy= 0;
if (55 < S & S < 60){
mouthExpandx = map(S, 50, 57, 0, 10);
mouthExpandy = map(S, 50, 57, 0, 50);
}
if (S > 59){
mouthExpandx = map(S, 58, 60, 10, 0);
mouthExpandy = map(S, 58, 60, 50, 0);
}
fill(0);
beginShape();
frameRate(10);
noStroke();
curveVertex(197+shake, 237+shake);
curveVertex(197, 237+shake);
curveVertex(211+shake, 236)
curveVertex(218, 239+shake);
curveVertex(220+shake, 244+shake)
curveVertex(214+mouthExpandx, 249+shake+mouthExpandy);
curveVertex(190+shake, 250+mouthExpandy);
curveVertex(179+shake-mouthExpandx, 248+shake+mouthExpandy);
curveVertex(175, 244+shake);
curveVertex(177+shake, 239+shake);
curveVertex(185, 236+shake);
curveVertex(197+shake, 237+shake);
curveVertex(197, 237+shake);
endShape();
fill(120, 18, 20)
arc(198, 329, 186, 54, 0, PI)
fill(239, 59, 57)
ellipse(198, 329, 184, 5)
for (var i = 0; i < S; i++){
frameRate(20);
var shakeX = A[i] + random(-0.5,0.5)
var shakeY = B[i] + random(-0.5 ,0.5)
coin(shakeX, shakeY);
}
}
function coin(x, y) {
push();
translate(x, y);
fill(226, 156, 37);
ellipse(0, 0, 22, 8);
fill(252, 207, 117);
ellipse(0, 0, 19, 5);
fill(255, 232, 192);
ellipse(0, -1, 10, 2);
pop();
}
function heart(x,y) {
beginShape();
noStroke();
translate(x,y);
fill(255, 0 ,0);
curveVertex(160, 161)
curveVertex(160, 161)
curveVertex(157, 157)
curveVertex(155, 152)
curveVertex(158, 151)
curveVertex(159, 154)
curveVertex(160, 152)
curveVertex(162, 150)
curveVertex(165, 151)
curveVertex(163, 157)
curveVertex(160, 161)
curveVertex(160, 161)
endShape();
}
I wanted to create a clock based on a toy that is based on a character from a Ghibli movie. The seconds are the most apparent from the coins, the size of the cloak follows the minute, and the color of the cloak is based on the hour. I wasn’t too concerned with being able to tell time. Overall this was satisfying to make even though there are issues with the smoothness of animation.
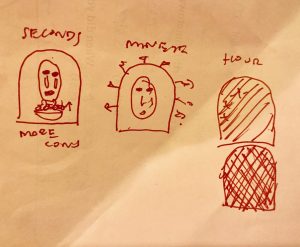