project7
//Fon Euchukanonchai
//15-104 Section A
//keuchuka@andrew.cmu.edu
//Project-07
var nP = 100;
var colorR;
var colorG;
var colorB;
var add = 0;
var plus = 0
var value = 0;
function setup() {
createCanvas(400, 400);
}
function draw() {
//random color values for background
var rColor1 = random(150, 255)
var rColor2= random(200, 255)
// mapping colors to mouseX for the three blobs and blobs that radiate
var mC = map(mouseX, 0, 400, 0, 300)
var bOne = map(mC, 0, 400, 80, 90)
var bTwo = map(mC, 0, 400, 45, 48)
var bThree = map(mC, 0, 400, 90, 100)
var bBehind = map(mC, 0, 200, 0, 100)
//setting background to random colors
background(255, rColor1, rColor2, mouseX/50);
// blobs that radiate
Curve(bBehind, 0+value, 255, 0+value, 255, 10+add, 0, 0, 300, mouseY/130);
Curve(bBehind, 255, 255,0+value, 255, 10+add, 0, 150, 0, mouseY/170);
Curve(bBehind, 0+value, 255, 255, 255, 10+add, 0, 400, 250, mouseY/150);
//blobs
Curve(bOne, 255-value, 255, 255-value, 255, 100-plus, 0, 0, 300, mouseY/140);
Curve(bTwo, 255, 255, 255-value, 255, 100-plus, 0, 150, 0, mouseY/170);
Curve(bThree, 255-value, 255, 255, 255, 100-plus, 0, 400, 250, mouseY/150);
//if mouse is pressed, the whites and colors are flipped
if (mouseIsPressed){
plus = 70;
value = 255
add = 10
} else {
plus = 0
value = 0
add=0
}
}
//function to build blob
function Curve(a, colorR, colorG, colorB, Scolor, trans, sW, mX, mY, ph) {
var x;
var y;
// setting variables to change size, blob shape, and rotation
var b = a / 2.0;
var k = constrain(mouseX, 0, 400)
var h = map(k, 0, width, 0, b-10);
strokeWeight(sW);
stroke(Scolor);
//colors are parametric
fill(colorR, colorG, colorB, trans);
// blobs are variable in size, blob shape, rotation, and location
beginShape();
for (var i = 0; i < nP; i++) {
var t = map(i, 0, nP, 0, TWO_PI);
x = (a + b) * cos(t) - h * cos(ph + t * (a + b) / b);
y = (a + b) * sin(t) - h * sin(ph + t * (a + b) / b);
vertex(mX+ x, mY+ y);
}
endShape();
}
With this project, I wanted to create “blobs” that move in subtle ways. The example that looked like some bacteria inspired this sort of movement, therefore I decided to go with polar curve for my project. I realize that the few parameters I could work with were rotation, size, color, location, and the small changes of the shape. I have the mouse react to some of these parameters so they look like they are changing but in a very subtle, bacteria like way. These blobs also had potential coming together, so I had them change in size to look like they “radiate” color to mix and become a gradient. The last layer was to have the colors flip when the mouse is clicked, but otherwise it works the exact same way. The two states I captured were the colors, as I think this is the main change, most obvious change. But the change of the curve is what gives the sensibility to the whole project.
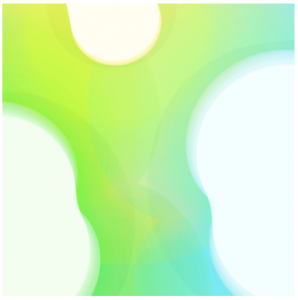
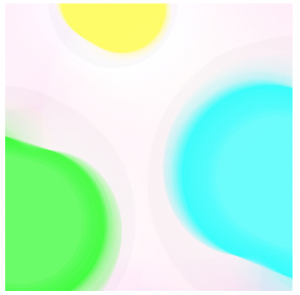