/*Rachel Park
rsp1@andrew.cmu.edu
Section B @ 10:30AM
Project 06: Abstract Clock*/
var prevSec;
var millisRolloverTime;
function setup() {
createCanvas(620, 620);
noStroke();
millisRolloverTime = 0;
}
function draw() {
background(17,76,98);
// Fetch the current time
var H = hour();
var X = (H%12);//so that the clock becomes a 12hr clock instead of 24 hr
var M = minute();
var S = second();
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
//showing text of time
fill(255);
text(nf(X,2,0) + ':' + nf(M,2,0) + ':' + nf(S,2,0),width/2-30,15);
var hourBarWidth = map(H, 0, 23, 0, width);
var minuteBarWidth = map(M, 0, 59, 0, width);
var secondBarWidth = map(S, 0, 59, 0, width);
// Making the seconds into a smoother transition
var secondsWithFraction = S + (mils / 1000.0);
var secondsWithNoFraction = S;
var secondBarWidthChunky = map(secondsWithNoFraction, 0, 60, 0, width);
var secondBarWidthSmooth = map(secondsWithFraction, 0, 60, 0, width);
//floorbed
fill(250,247,196);
rect(0,height-115,width,200);
//drawing the snail (hour)
noStroke();
fill(207,230,239);
rect(0, 500, hourBarWidth, 10);
fill(234,207,239);
ellipse(hourBarWidth,484,45,45);
fill(155,124,161);
ellipse(hourBarWidth,505,70,10);
fill(155,124,161);
ellipse(hourBarWidth+20,483,5,45);
fill(155,124,161);
ellipse(hourBarWidth+30,483,5,45);
fill(255);
ellipse(hourBarWidth+20,465,15,15);
fill(255);
ellipse(hourBarWidth+30,465,15,15);
fill(25);
ellipse(hourBarWidth+20,465,8,8);
fill(25);
ellipse(hourBarWidth+30,465,8,8);
//drawing fish hook (seconds)
push();
noFill();
strokeWeight(10);
stroke(160);
arc(100,secondBarWidthSmooth,70,70,0,PI);
strokeWeight(5);
arc(135,secondBarWidthSmooth-110,10,10,0,TWO_PI);
strokeWeight(6);
rect(133,secondBarWidthSmooth-100,5,100,10);
stroke(50);
rect(133,0,2,secondBarWidthSmooth-110,5);
pop();
//drawing the fish (minute)
fill(94,229,239);
ellipse(minuteBarWidth,275,100,80);
fill(255);
ellipse(minuteBarWidth+25,250,40,40);
fill(0);
ellipse(minuteBarWidth+35,250,20,20);
fill(94,191,239);
triangle(minuteBarWidth-80,255,minuteBarWidth-80,295,minuteBarWidth-40,270);
//and exclamation point
fill(255);
rect(minuteBarWidth-5,130,10,60,10);
ellipse(minuteBarWidth,210,10,10);
}
For my project, I wanted to make a clock but visually represented in a unique way. Here, I decided to make an instance/ scene of a fish underwater. The snail on the floorbed represents the hours (bc snails are slow anyway), the fish represents the minutes, and the fish hook represents the seconds. The scene here is that the fish is swimming away from the oncoming hook while the snail is just crawling away in a leisurely pace. As seen in the sketch, I initially wanted to use bubbles to represent the seconds, but went ahead and changed my idea to the fish hook instead.
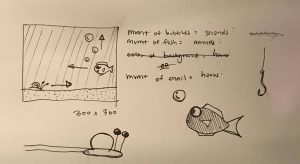
(not sure if it’s just me, but for some reason I have to refresh the page a couple times to see the code work.)