sketch
//Robert Managad
//Section-E
//rmanagad@andrew.cmu.edu
//Project 11 -- Turtle Graphics
var turtleWormA;
function setup() {
createCanvas(400, 400);
}
function draw() {
var mil = millis(); //use of milliseconds an automatic rotation.
background(230, 201, 148);
//turtle drawings below, which vary on length and placement
push();
translate(width/2, height/2);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 100; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width/4, height/4);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.75, height*0.75);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.75, height*0.25);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.25, height*0.75);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.45, height*0.35);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.85, height*0.65);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.15, height*0.45);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.15, height*0.55);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.55, height*0.95);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.60, height*0.65);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.40, height*0.85);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.10, height*0.90);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.40, height*0.60);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.80, height*0.40);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(sin(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.90, height*0.90);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.60, height*0.10);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.95, height*0.15);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
push();
translate(width*0.10, height*0.15);
rotate(mil/500);
turtleWormA = makeTurtle(0, 0);
turtleWormA.setColor(color(47, 24, 57));
turtleWormA.setWeight(3);
for (var i = 0; i < 50; i++) {
turtleWormA.forward(2);
turtleWormA.left(cos(i/5) * mouseY/17);
}
pop();
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
For the Turtle Composition, I wanted to play with modifying turtle paths using trigonometric functions. I also thought about elements in nature that visually present trigonometric functions to incorporate into my work as compositional elements. I came up with a handful of ideas, but ultimately saw compositional harmony with worms popping out of holes in the ground (or armpit hair).
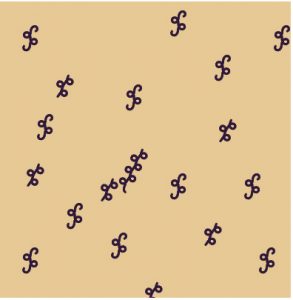
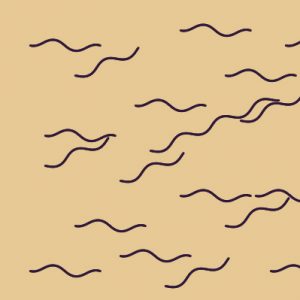