Learning Goals:
- Understand how to recursively call functions.
- Base Case must Terminate
- Recursive Call
- Understand how to debug a recursive program
- Understand depth of a recursive call
- Know the applications of recursion in terms of visualizations
- Fractals
- Organic shapes
- Recursive images
Lab:
- Go over how to draw one tree
- Walkthrough adding two more branches
- Walkthrough adding any number of branches
- Add randomization and/or movement
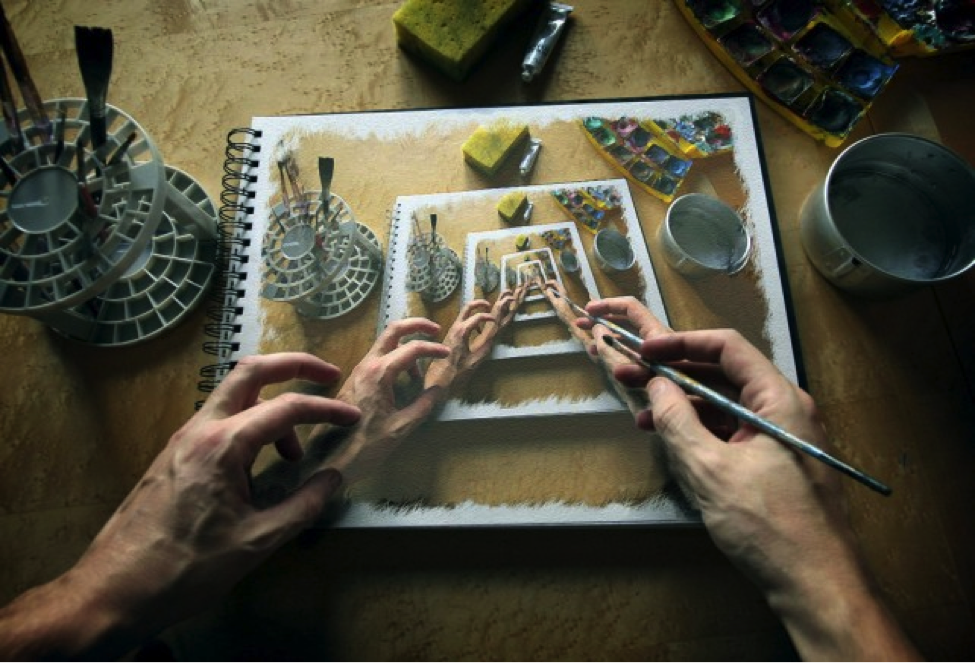
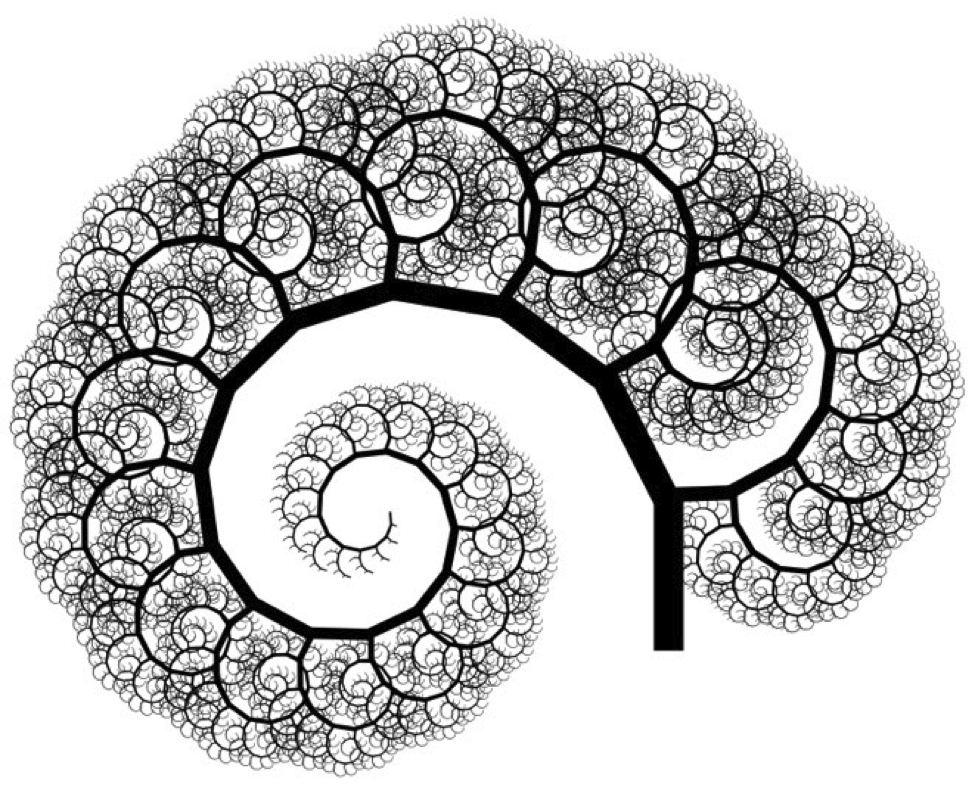
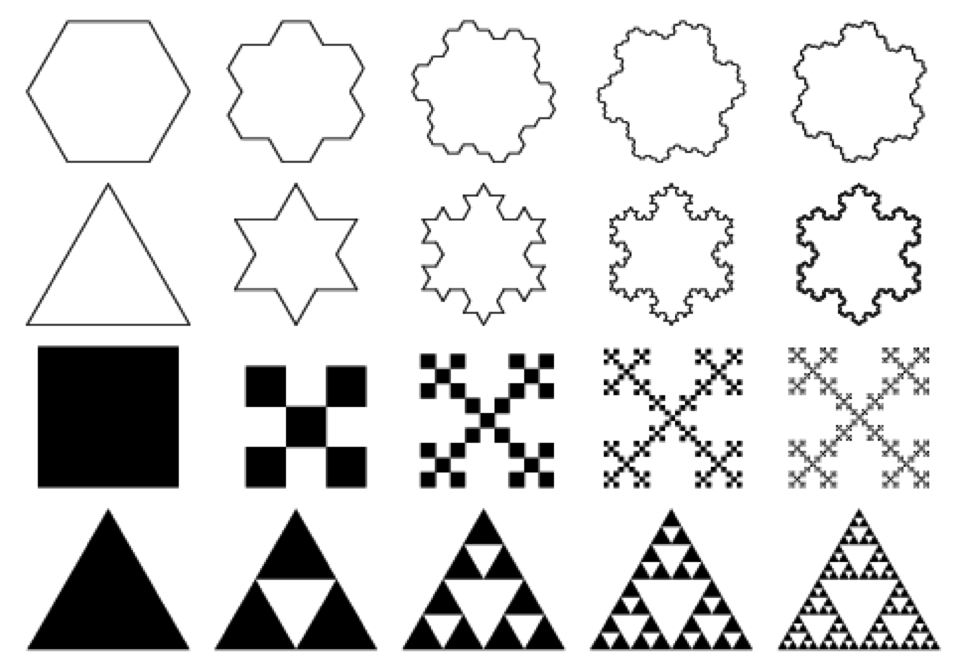
sketch
function setup() {
createCanvas(400, 400);
frameRate(10);
}
function draw() {
background(240);
push();
translate(200, 350);
drawBranch(0, 30);
pop();
}
function drawBranch(depth, len) {
line(0, 0, 0, -len);
push();
translate(0, -len);
drawTree(depth + 1, len);
pop();
}
function drawTree(depth, len) {
if (depth < 8) {
rotate(radians(-10));
drawBranch(depth, len);
rotate(radians(20));
drawBranch(depth, len);
}
}