The idea for this project was to try and represent the time of day by paralleling it with the subsequent way someone feels during the day, and match the way they look as a day progresses. The result was a person who looks tired in the morning, starts to liven up around noon and in the afternoon, and then gradually gets tired again and falls asleep. The background also matches the color of the sky as time passes by.
Here are photos of all the different stages: 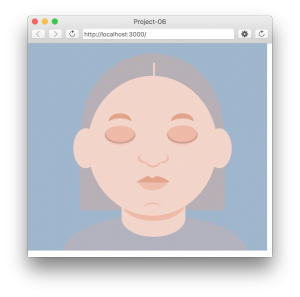
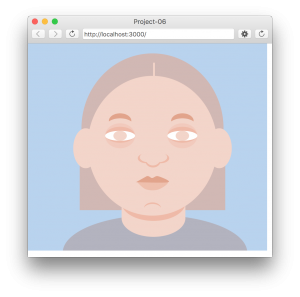
dawn morning
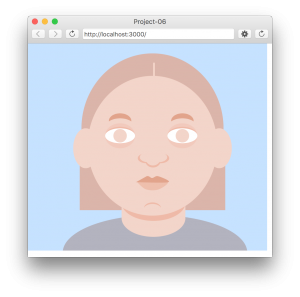
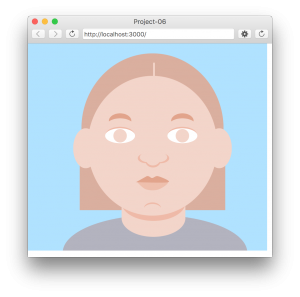
noon afternoon
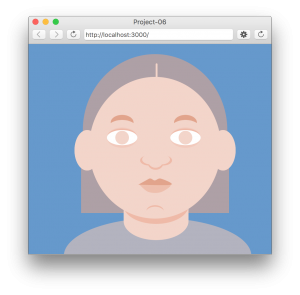
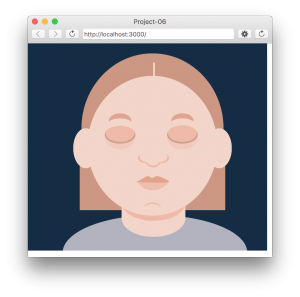
evening dusk
sketch
//Isadora Krsek
//Ikrsek@andrew.cmu.edu
//Section C
//Project 06: Abstract clock
var r = (0);
var g = (0);
var b = (0);
var dawn = (5);
var morning = (7);
var noon = (12);
var afternoon = (13);
var evening = (18);
var dusk = (22);
skinbaseR = (243);
skinbaseG = (213);
skinbaseB = (202);
function setup() {
createCanvas(480,415);
}
function draw() {
var h = hour();
var m = minute();
var s = second();
background(r,g,b);
//draw dawn image if it is dawn
if (dawn <= h & h < morning){
dawnImage();
}
//draw morning image if it is morning
else if (morning <= h & h < noon){
morningImage();
}
//draw noon image if it is noon
else if (noon <= h & h < afternoon){
noonImage();
}
//draw afternoon image if it is afternoon
else if (afternoon <= h & h < evening){
afternoonImage();
}
//draw evening image if it is evening
else if (evening <= h & h < dusk){
eveningImage();
}
//draw dusk image if it is dusk but before midnight
else if (dusk <= h & h < 24 ){
duskImage();
}
//draw dusk after midnight before dawn(separated because p5 tracks hours using military time)
else if (0 <= h < dawn){
duskImage();
}
}
//dawn
function dawnImage() {
r = (159);
g = (182);
b = (205);
var m = minute();
var s = second();
var ms = millis();
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28)
ellipse(252,350,128,75)
//neck shadow
fill(239,186,168)
ellipse(252,318,129,75)
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140)
ellipse(310,155,45,30)
//left eyebrow
ellipse(185,155,45,30)
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,165,55,30)
//left eyebrow light
ellipse(185,165,55,30)
//EYES
//eyebag left
fill(239,186,168,100);
ellipse(185,185,60,40);
//eyebag right
ellipse(310,185,60,40);
//left eyelid
fill(239,186,168)
stroke(207,159,143);
ellipse(185,183,58,32);
noStroke();
ellipse(185,180,58,30);
//right eyelid
stroke(207,159,143)
ellipse(310,183,58,32);
noStroke();
ellipse(310,180,58,32);
//left eye
fill(255)
ellipse(185,185,60,0)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(185,185,27,0)
//right eye
fill(255)
ellipse(310,185,60,0)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,0)
//NOSE
//nose ball shadow
fill(239,186,168)
ellipse(251,236,30,25)
//left nostril shadow
ellipse(238,230,35,20)
//right nostril shadow
ellipse(266,230,35,20)
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(242,228,35,20)
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(262,228,35,20)
//nose ball
ellipse(251,230,30,29)
//CHIN
//chin dark line
fill(239,186,168)
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50)
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
//BLINK
push();
noStroke();
scale(.65,.65);
translate(27,-10);
//blink
if (ms%3===0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
//morning
function morningImage() {
r = (185);
g = (211);
b = (238);
m = minute();
s = second();
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28)
ellipse(252,350,128,75)
//neck shadow
fill(239,186,168)
ellipse(252,318,129,75)
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140)
ellipse(310,155,45,30)
//left eyebrow
ellipse(185,155,45,30)
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,165,55,30)
//left eyebrow light
ellipse(185,165,55,30)
//EYES
//eyebag left
fill(239,186,168,100);
ellipse(185,185,60,50);
//eyebag right
ellipse(310,185,60,50);
//left eyelid
fill(239,186,168)
ellipse(185,183,58,32);
//right eyelid
ellipse(310,183,58,32);
//left eye
fill(255)
ellipse(185,185,60,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(185,185,27,20)
//right eye
fill(255)
ellipse(310,185,60,20)
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,20)
//NOSE
//nose ball shadow
fill(239,186,168)
ellipse(251,236,30,25)
//left nostril shadow
ellipse(238,230,35,20)
//right nostril shadow
ellipse(266,230,35,20)
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(242,228,35,20)
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(262,228,35,20)
//nose ball
ellipse(251,230,30,29)
//CHIN
//chin dark line
fill(239,186,168)
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50)
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
//BLINK
push();
noStroke();
scale(.65,.65);
translate(27,-10);
//sec blink
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
//noon
function noonImage() {
r = (198);
g = (226);
b = (255);
m = minute();
s = second();
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
//neck shadow
fill(239,186,168);
ellipse(252,318,129,75);
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140);
ellipse(310,155,45,30);
//left eyebrow
ellipse(185,155,45,30);
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
//left eyebrow light
ellipse(185,165,55,30);
//EYES
//eyebag left
fill(239,186,168,100);
ellipse(185,185,60,50);
//eyebag right
ellipse(310,185,60,50);
//left eyelid
fill(239,186,168);
ellipse(185,183,58,32);
//right eyelid
ellipse(310,183,58,32);
//left eye
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
//right eye
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,27);
//NOSE
//nose ball shadow
fill(239,186,168);
ellipse(251,236,30,25);
//left nostril shadow
ellipse(238,230,35,20);
//right nostril shadow
ellipse(266,230,35,20);
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
//nose ball
ellipse(251,230,30,29);
//CHIN
//chin dark line
fill(239,186,168);
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(0.65,0.65);
translate(27,-10);
//sec blink
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
//afternoon
function afternoonImage() {
r = (176);
g = (226);
b = (255);
m = minute();
s = second();
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
//neck shadow
fill(239,186,168);
ellipse(252,318,129,75);
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140);
ellipse(310,155,45,30);
//left eyebrow
ellipse(185,155,45,30);
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
//left eyebrow light
ellipse(185,165,55,30);
//EYES
//left eyelid
fill(239,186,168);
ellipse(185,183,58,32);
//right eyelid
ellipse(310,183,58,32);
//left eye
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
//right eye
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,27);
//NOSE
//nose ball shadow
fill(239,186,168);
ellipse(251,236,30,25);
//left nostril shadow
ellipse(238,230,35,20);
//right nostril shadow
ellipse(266,230,35,20);
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
//nose ball
ellipse(251,230,30,29);
//CHIN
//chin dark line
fill(239,186,168);
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(0.65,0.65);
translate(27,-10);
//sec blink
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
//evening
function eveningImage() {
r = (102);
g = (153);
b = (204);
m = minute();
s = second();
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
//neck shadow
fill(239,186,168);
ellipse(252,318,129,75);
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140);
ellipse(310,155,45,30);
//left eyebrow
ellipse(185,155,45,30);
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
//left eyebrow light
ellipse(185,165,55,30);
//EYES
//eyebag left
fill(239,186,168,100);
ellipse(185,185,60,40);
//eyebag right
ellipse(310,185,60,40);
//left eyelid
fill(239,186,168);
ellipse(185,183,58,32);
//right eyelid
ellipse(310,183,58,32);
//left eye
fill(255);
ellipse(185,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,27);
//right eye
fill(255);
ellipse(310,185,60,30);
fill(skinbaseR,skinbaseG,skinbaseB)
ellipse(310,185,27,27);
//NOSE
//nose ball shadow
fill(239,186,168);
ellipse(251,236,30,25);
//left nostril shadow
ellipse(238,230,35,20);
//right nostril shadow
ellipse(266,230,35,20);
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
//nose ball
ellipse(251,230,30,29);
//CHIN
//chin dark line
fill(239,186,168);
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
//sec blink
if (s%4 === 0) {
fill(239,186,168);
ellipse(258,290,90,50);
ellipse(450,290,90,50);
stroke(207,159,143);
strokeWeight(4);
arc(258,290,90,60,0,PI);
arc(450,290,90,60,0,PI);
}
pop();
noStroke();
}
//dusk
function duskImage() {
r = (21);
g = (44);
b = (69);
//shirt
fill(179,179,191);
rect(70,445,360,80);
ellipse(255,419,370,150);
//hair
fill(226,164,140,90); //brown hair 34,20,15
ellipse(250, 170, 285, 300);
rect(104,180,292,154);
//hairline
stroke(skinbaseR,skinbaseG,skinbaseB);
strokeWeight(3.5);
line(253,40,253,100);
//NECK
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
rect(188,320,128,28);
ellipse(252,350,128,75);
//neck shadow
fill(239,186,168);
ellipse(252,318,129,75);
//HEAD
noStroke();
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250, 205, 260, 280);
//right ear
ellipse(384, 210, 50, 80);
//left ear
ellipse(116, 210, 50, 80);
//BROWS
//right eyebrow
fill(226,164,140);
ellipse(310,155,45,30);
//left eyebrow
ellipse(185,155,45,30);
//right eyebrow light
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,165,55,30);
//left eyebrow light
ellipse(185,165,55,30);
//EYES
//eyebag left
fill(239,186,168,100);
ellipse(185,190,60,45);
//eyebag right
ellipse(310,190,60,45);
//left eyelid
fill(239,186,168);
stroke(207,159,143);
ellipse(185,183,58,32);
noStroke();
ellipse(185,180,58,30);
//right eyelid
stroke(207,159,143);
ellipse(310,183,58,32);
noStroke();
ellipse(310,180,58,32);
//left eye
fill(255)
ellipse(185,185,60,0);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(185,185,27,0);
//right eye
fill(255);
ellipse(310,185,60,0);
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(310,185,27,0);
//NOSE
//nose ball shadow
fill(239,186,168);
ellipse(251,236,30,25);
//left nostril shadow
ellipse(238,230,35,20);
//right nostril shadow
ellipse(266,230,35,20);
//left nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(242,228,35,20);
//right nostril
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(262,228,35,20);
//nose ball
ellipse(251,230,30,29);
//CHIN
//chin dark line
fill(239,186,168);
ellipse(250,330,34,23);
//chin
fill(skinbaseR,skinbaseG,skinbaseB);
ellipse(250,333,36,23);
//LIPS
//lower lip
push();
translate(-52,50);
scale(1.2,1.2);
beginShape();
fill(238,191,173);
noStroke();
curveVertex(226,190);
vertex(276,189);
curveVertex(262,202);
curveVertex(241,202);
endShape(CLOSE);
beginShape();
//upper lip
fill(226,164,140);
noStroke();
strokeWeight(1);
curveVertex(224,190);
curveVertex(242,180);
curveVertex(251,183);
curveVertex(261,180);
vertex(278,190);
endShape(CLOSE);
pop();
push();
noStroke();
scale(.65,.65);
translate(27,-10);
}