Initially, I was going to make a generative landscape based on microscopic creatures. Such as what you would see under a microscope. However I later decided to go one layer more, and tried to portray the subatomic environment. For this project, I wanted to create a subatomic generative landscape. So the basis of in the subatomic environment, is the atom. And I had to decide how to create differing forms of atoms. Then I realized that atoms with different sizes also create different elements. I created 3 types of possible moving objects which are different sized atoms, with electrons wizzing around them. Below attached are some of my sketches.
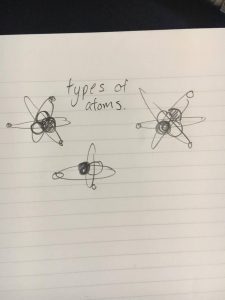
//Alvin Luk
//Section A
//akluk@andrew.cmu.edu
//Project 10
var atoms = [];
function setup() {
createCanvas(480, 480);
// create an initial collection of buildings
for (var i = 0; i < 10; i++){
var rx = random(width);
atoms[i] = makeAtom(rx);
}
frameRate(20);
}
function draw() {
background(0);
push();
stroke(0);
fill(0);
rect(0,0, width, height);
displayStatusString();
updateAndDisplayAtoms();
removeAtomsThatHaveSlippedOutOfView();
addNewAtomsWithSomeRandomProbability();
pop();
}
function updateAndDisplayAtoms(){
// Update the building's positions, and display them.
for (var i = 0; i < atoms.length; i++){
atoms[i].move();
atoms[i].display();
}
}
function removeAtomsThatHaveSlippedOutOfView(){
// If a building has dropped off the left edge,
// remove it from the array. This is quite tricky, but
// we've seen something like this before with particles.
// The easy part is scanning the array to find buildings
// to remove. The tricky part is if we remove them
// immediately, we'll alter the array, and our plan to
// step through each item in the array might not work.
// Our solution is to just copy all the buildings
// we want to keep into a new array.
var atomsToKeep = [];
for (var i = 0; i < atoms.length; i++){
if (atoms[i].x + atoms[i].breadth > 0) {
atomsToKeep.push(atoms[i]);
}
}
atoms = atomsToKeep; // remember the surviving buildings
}
function addNewAtomsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newAtomLikelihood = 0.02;
if (random(0,1) < newAtomLikelihood) {
atoms.push(makeAtom(width));
}
}
// method to update position of building every frame
function atomMove() {
this.x += this.speed;
}
// draw the building and some windows
function objectDisplay() {
var floorHeight = 20;
var particle_size = 20;
var bHeight = this.nFloors * floorHeight;
fill(120);
noStroke(0);
push();
translate(this.x, this.y);
stroke(0);
if (this.breadth == 1){
fill(color(255,0,0));
ellipse(0, 0, particle_size, particle_size);
fill(color(0,0,255));
ellipse(particle_size/4, particle_size/4 , particle_size, particle_size);
}
else if (this.breadth == 2){
fill(color(255,0,0));
ellipse(-particle_size/4, -particle_size/4, particle_size, particle_size);
fill(color(0,0,255));
ellipse(particle_size/4, -particle_size/4 , particle_size, particle_size);
fill(color(255,0,0));
ellipse(-particle_size/4, particle_size/4, particle_size, particle_size);
fill(color(0,0,255));
ellipse(particle_size/4, particle_size/4 , particle_size, particle_size);
}
else{
fill(color(255,0,0));
ellipse(-particle_size/3, particle_size/3, particle_size, particle_size);
fill(color(0,0,255));
ellipse(0, -particle_size/3 , particle_size, particle_size);
ellipse(particle_size/3, particle_size/3, particle_size, particle_size);
}
noStroke();
fill(color(180,180,40));
for (var i = 0; i < this.nFloors; i++) {
ellipse(random(-particle_size,particle_size), random(-particle_size,particle_size), 5, 5);
}
pop();
}
function makeAtom(birthLocationX) {
var bldg = {x: birthLocationX,
y: round(random(0,height)),
breadth: random([1,2,3]),
speed: -1.0,
nFloors: round(random(2,8)),
move: atomMove,
display: objectDisplay}
return bldg;
}
function displayHorizon(){
stroke(0);
line (0,height-50, width, height-50);
}
function displayStatusString(){
noStroke();
fill(0);
var statusString = "# Atoms = " + atoms.length;
text(statusString, 5,20);
}