/*
Jamie Ho
jamieh@andrew.cmu.edu
10:30
Project 10
*/
var clouds = [];
var skies = [];
var sunPos = 0;
var colour = 0;
function setup() {
createCanvas(480, 480);
//to make and store clouds in array
for (var i = 0; i < 10; i++){
var rx = random(width);
clouds[i] = makeClouds(rx);
}
frameRate(10);
}
function draw() {
noStroke();
//environment outside of plane
sky();
theSun(sunPos);
if(sunPos < width){ //condition statement for position of sun
sunPos += 3; //to move sun
colour += 2; //to change colour
} else { //go back to 0
sunPos = 0;
colour = 0;
}
updateAndDisplayClouds();
addNewCloudsChances();
//plane interiors
windows(0);
windows(width*0.7);
planeInterior();
seat(0);
seat(width*0.7);
}
function windows(pos){
fill(200, 200, 200, 50);
rect(pos+width/6, height/4, pos+width/2, height);
ellipseMode(CORNER);
arc(pos+width/6, 0, width/2, width/2, PI, 0);
}
function seat(pos){
noStroke();
fill(150);
rect(pos, height/3, width/8, height, 50); //back rest
fill(200);
rect(pos, height*0.85, width/2, height, 25); //seat
fill(125);
rect(pos+25, height*0.75, width/2.5, 25, 50); //arm rest
ellipseMode(CORNER);
fill(100);
ellipse(pos+25, height*0.75, 25, 25) //arm rest joint
ellipseMode(CENTER);
fill(200);
ellipse(pos+width/8, height/2.5, 18, height/5); //head rest
}
function planeInterior(){
fill(80);
rect(0, height*0.7, width, height);
}
function updateAndDisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].show();
}
}
function addNewCloudsChances() {
// With a very tiny probability, add a new building to the end.
var newCloudChances = 0.01;
if (random(0,1) < newCloudChances) {
clouds.push(makeClouds(width));
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep; // remember the surviving buildings
}
function cloudsMove() {
this.x += this.speed;
}
function cloudsDisplay(){
var gs = random(240, 255); //greyscale
fill(gs, gs, gs, 50);
ellipse(this.x, this.y, this.sizeX, this.sizeY); //big clouds
ellipse(this.x+5, this.y-100, this.sizeX*0.45, this.sizeY*0.25); //small clouds
}
function makeClouds(birthLocationX){
var clouds = {x: birthLocationX, //where it starts
y: random(height*0.35, height*0.7), //vertical position
speed: random(-0.5, -1), //speed of each cloud
breadth: 35, //distance between clouds
sizeX: random(120, 200), //size of ellipse
sizeY: random(60, 100),
move: cloudsMove, //to animate clouds
show: cloudsDisplay}; //to create clouds
return clouds;
}
function sky(){
var factor = 0.5; //factor to decrease rbg values by
for(var i = 0; i<width; i++){
var f = i*factor; //rbg decreases incrementally based on i
fill(230-f, 247-f, 255-f);
skies.push(rect(i, 0, i+1, height*0.7)); //creating sky rectangles
}
}
function theSun(x){
var sSize = 100;
ellipseMode(CORNER);
fill(255+colour, 204+colour, 0+colour, 255-colour/2);
ellipse(x, height/16, sSize, sSize);
}
The hardest part of this project is still understanding objects and keeping track of where those parameters go within all the different functions that are then called in draw. I started the project with drawing what was still, then putting in what moved. However, I think I should have done what moved first as it was in the background and it requires more time to figure out. I wanted to show more to the moving landscape based on the changing time of day during a long flight, but I couldn’t figure out how to do a moving gradient sky and just kept it as a gradient.
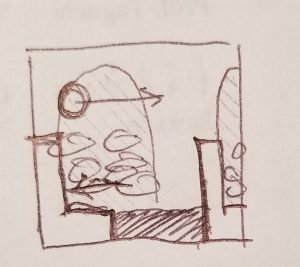