var r;
var t;
var a = 100;
var headx;
var discox;
var p;
function setup() {
createCanvas(640, 480)
}
function draw() {
var x = mouseX + (width / 2);
var y = mouseY;
background(230, x, y);
strokeWeight(0);
fill(a, 200, 200);
ellipse(r, t, 20, 20);
ellipse(r * 2, t, 20, 20);
ellipse(r / 2, -t, 20, 20);
ellipse(r, t / 2, 20, 20);
if (mouseIsPressed) {
ellipse(r, t, 50, 50);
r = random(20, 500);
t = random(20, 460);
a = random(1, 250);
}
//disco man
//shirt
fill(255);
rect (headx - 15, 360, 130, 100, 20);
//hair
fill(40);
arc(headx + 50, 250, 200, 200, PI-QUARTER_PI, TWO_PI+QUARTER_PI, CHORD);
//neck
fill(254, 213, 192);
rect(headx + 35, 300, 30, 80, 20);
//face
fill(254, 213, 192)
rect(headx, 250, 100, 100, 20);
//glasses
fill(0)
arc(headx + 30, 290, 30, 30, TWO_PI, PI, CHORD);
arc(headx + 70, 290, 30, 30, TWO_PI, PI, CHORD);
rect (headx + 30, 290, 40, 5);
fill(0, 102, 153, 51);
text("click and drag mouse accross canvas", 5, 480);
if (100 < mouseX & mouseX < (width - 100)) {
headx = mouseX - 50;
}
strokeWeight (0.5)
fill (255, mouseX)
ellipse (p, 50, discox, discox)
if (100 < mouseX & mouseX < (width/2)) {
p = mouseX;
discox = mouseX/3
}
if ((width/2) < mouseX & mouseX < (width - 100)) {
p = mouseX;
discox = (width - mouseX)/3
}
}
Category: Project-03-Dynamic-Drawing
elizabew-Project-03-Dynamic-Drawing
Reflection
Admittedly, I actually did have a more solid idea before coming up with this program — I was planning on creating a sun going up and down, while make a face squint when the sun was all the way up; however I ended up having too much fun playing around with the patterns and the rotation action that I ended up distancing myself from that initial idea. I found it so exciting to see the patterns that would form depending on how fast it is going and where the mouse was on the image. Overall, the final product reminds me of strobe lights or fireworks — and even though it isn’t as obvious as my initial idea, I still think it looks pretty cool.
//Elizabeth Wang
//Section E
//elizabew@andrew.cmu.edu
//Project-03: Dynamic Drawing
var angleSecond = 0;
var angleMiddle = 0;
var angleThird = 0;
var angletriangle = 0;
var colorA = 250;
var colorB = 50;
function setup() {
createCanvas(480, 640);
rectMode(CENTER);
angleMode(DEGREES);
}
function draw() {
background(80, 100, 200);
colorA = mouseX;
colorB = mouseY;
noStroke();
//second from middle
fill(200, 100, colorA); // all colors are random, constantly
push();//rotate
translate(mouseX, mouseY); //moves with mouse
rotate(angleSecond);
translate(0, -140); //location
rect(0, 0, 130, 12);
pop();//rotate
angleSecond += max(min(mouseY/10, 1), mouseX/10); //gets faster as you move right
//middle
fill(50, 100, colorB);
push();//rotate
translate(mouseX, mouseY); //moves with mouse
rotate(angleMiddle);
rect(0, 0, 200, 25);
pop();//rotate
angleMiddle += max(min(mouseY/10, 1), mouseX/10); //gets faster as you move down
//third
fill(230, 60, colorA);
push();
translate(width/2, height/2); //doesn't move with the mouse, constant background
rotate(angleThird);
translate(0, 200);
scale (mouseX/100, mouseY/100); // changed size as it moves
rect(0, 0, 220, 35);
pop();
angleThird += max(min(mouseY/10, 1), mouseX/10);
//third but the other direction
fill(200, 200, colorB);
push();
translate(width/2, height/2);
rotate(-angleThird);
translate(0, 200);
scale (mouseX/100, mouseY/100); // changed size as it moves
rect(0, 0, 220, 35);
pop();
angleThird += max(min(mouseY/10, 1), mouseX/10);
//triangle
fill(250, 150, colorA);
push();
translate(mouseX, mouseY);
rotate(-angletriangle);
translate(0, 20);
triangle(-20, 60, 0, 20, 20, 60);
pop();
angletriangle += max(min(mouseY/10, 1), mouseX/10);
//rotating while also going around
fill(150, 200, colorB);
push();
translate(mouseX, mouseY);
rotate(angleSecond);
translate(0, 200);
rect(0, 0, 300, 40);
pop();
angleSecond += max(min(mouseY/10, 1), mouseX/10);
}
Project 03 – Yugyeong Lee
//Yugyeong Lee
//Section B
//yugyeonl@andrew.cmu.edu
//Project-03
var y = 0;
var spacing = 15;
var r = 7.5;
function setup() {
createCanvas(640, 480);
}
function draw() {
noCursor();
//background color change based on position of mouseX and mouseY
colorR = map(mouseY, 0, height, 180, 255);
colorB = map(mouseX, 0, width, 180, 255);
colorG = map(mouseX, 0, width, 160, 235);
background(colorR, colorG, colorB);
//strokewegith depends on the position of mouseY
m = map(mouseY, 0, height, 1, 5)
strokeWeight(m);
stroke(255);
//array curves with mid points that react to position of mouseX and mouseY
if (mouseX >= width/2) {
for (var i = 0; i < 640; i+= spacing) {
positionX = map(mouseX, 0, width/2, 0, 1);
positionY = map(mouseY, 0, height, 0, 1);
noFill();
beginShape();
curveVertex(i, 0);
curveVertex(i, 0);
curveVertex(positionX*i, height*positionY);
curveVertex(i, height);
curveVertex(i, height);
endShape();
}
}
//array of curves stay straight when mouseX is on left half of canvas
if (mouseX < width/2) {
for (var i = 0; i < 640; i+= spacing) {
line(i, 0, i, height);
}
}
//array of circles
for (var x = 0; x < width + r; x+= spacing) {
for (var y = 0; y < height + r; y+= spacing) {
noStroke();
//the amount of circles which changes size depends on mouseX
var n = map(mouseX, 0, width, 1, 8);
//aray of circles which changes color based on its distance from the Cursor
if (dist(mouseX, mouseY, x, y) < n*r) {
fill(random(0, 255), random(0, 255), 200);
r = 12
}
else {
fill(150);
r = 7.5
}
ellipse(x, y, r, r);
}
}
}
I wanted to create a grid base, interactive graphic that depends heavily on the position of mouseX and mouseY. The array of circles’s sizes and color depend on the distance between position of the cursor and the center point of each circle. The background color also changes based on mouseX and mouseY as well as the array of lines in the background in which the mid point changes value based on location of the cursor as well.
ashleyc1-Section C-Project-03-Dynamic-Drawing
//Ashley Chan
//Section C
//ashleyc1@andrew.cmu.edu
//Project-03-Dynamic-Drawing.
//PARAMETERS THAT I AM CHANGING
//Color
//Ball Size
//Ball angle: rotation of the spiral
//Spacing between balls
//Size of spiral
var angle;
var positionX;
var positionY;
var ballWidth;
var ballHeight;
var isDrawing;
var r;
var g;
var b;
//setup with default values for rgb color values
function setup(/*red = 213, green = 23, blue = 23*/) {
createCanvas(640, 480);
background(0);
//rotate circles based on mouse position
//#gotta love trig
angle = (atan((mouseY - 240) / (mouseX - 320)) / 500);
positionX = 0;
positionY = 0;
ballWidth = 5;
ballHeight = 5;
//as you increment i, draw a circle
for (var i = 0; i < 600; i++) {
fill(r, g, b);
stroke(255);
//As i increases, we draw a ball with increasing width
//untill we reach maximum growth
ballWidth += (30/600) * ((480 - mouseY) / 480);
ballHeight += (30/600) * ((480 - mouseY) / 480);
//start point
push();
translate(width/2, height/2);
rotate(degrees(angle));
ellipse(positionX, positionY, ballWidth, ballHeight);
pop();
drawOut();
}
}
function draw() {
changeColor();
}
function changeColor() {
//if mouse is on right side of canvas, red
if (mouseX > width/2) {
r = map(mouseX, 0, width/2, 500, 213);
g = map(mouseX, 0, width, 100, 32);
b = map(mouseX, 0, width, 135, 255);
}
//if mouse is on left side of canvas, blue
if (mouseX < width/2) {
r = map(mouseX, 0, width/2, 200, 100);
g = map(mouseX, 0, width, 0, 32);
b = map(mouseX, 0, width, 0, 255);
}
//have to call setup again if you want movement
setup(r, g, b);
}
function drawOut() {
//circles drawn outward
positionX = positionX + .5;
positionY = positionY + .5;
//controls the spacing between the balls
angle = angle + (mouseX/ 100000);
}
For this project, I wanted to expand on the spiral drawing exercise we did in lab because I found it a fun shape to play with and thought it would create a cool optical illusion. Although subtle, I am changing several parameters such as color, ball size, ball position and angle as well as the space in between the balls. One surprising result is how much had to tap into old trig knowledge in order to calculate how to rotate the spiral just right.
creyes1-Project-03-Dynamic-Drawing
creyes1-Project-03 Dynamic Drawing
//Christopher Reyes
//Section D
//creyes1@andrew.cmu.edu
//Project-03 (Dynamic Drawing)
var backgroundR = 209
var backgroundG = 153
var backgroundB = 198
var ringSize = 0
var ringColor= 256
function setup() {
createCanvas(640, 480);
angleMode(DEGREES);
}
function draw() {
var colorConstrain = constrain(mouseX, 102, 540)
background(backgroundR, backgroundG, backgroundB);
fromBG = color(209, 153, 198);
toBG = color(199, 211, 183);
backgroundColor = lerpColor(fromBG, toBG, mouseX/width);
background(backgroundColor);
var moonConstrainX = constrain(mouseX, 102, 540)
//Moon, moves in the X direction as mouseX changes
noStroke();
fill(256);
ellipse(moonConstrainX, 80, 64, 64);
//Moon shadow, follows moon at a rate that eventually overtakes it
//Color set to be the same as the background to hide it
fill(backgroundR, backgroundG, backgroundB);
fill(backgroundColor);
ellipse(moonConstrainX*1.30 - 95, 80, 54, 54);
//Mountain ring, changes scale according to mouseX
noFill();
stroke(ringColor);
strokeWeight(5);
ringSize = constrain(mouseX, 100, 545) - 100
ellipse(248, 158, ringSize, ringSize);
//Secondary rings that rotate while revolving around mountain's peak,
//based on mouseX position
var spinConstraint = constrain(mouseX, 102, 540)
push();
translate(248, 158);
rotate(spinConstraint);
arc(248, 158, ringSize, ringSize,
spinConstraint + 5, spinConstraint + 115);
arc(248, 158, ringSize, ringSize,
spinConstraint + 125, spinConstraint + 235);
arc(248, 158, ringSize, ringSize,
spinConstraint + 245, spinConstraint + 355);
pop();
push();
translate(248, 158);
rotate(spinConstraint - 160);
arc(248, 158, ringSize*.75, ringSize*.75,
spinConstraint + 5, spinConstraint + 115);
arc(248, 158, ringSize*.75, ringSize*.75,
spinConstraint + 125, spinConstraint + 235);
arc(248, 158, ringSize*.75, ringSize*.75,
spinConstraint + 245, spinConstraint + 355);
pop();
//Hides rings when they get too small - given same color as background
if (ringSize == 0) {
ringColor = backgroundColor;
} else {
ringColor = 256;
}
noStroke();
//Draws triangles for mountains that change colors as mouseX changes
//Note: For organization, for each mountain, the left side is drawn first
//
//Mountain color variable format: Mount(#)[(L)eft||(R)ight]
//Mountain 1, transitions from pink to blue
fill(232, 151, 168);
fromMount1L = color(232, 151, 168);
toMount1L = color(105, 175, 173);
mount1LColor = lerpColor(fromMount1L, toMount1L, mouseX/width);
fill(mount1LColor);
triangle(111, 226, 57, 416, -3, 416);
fill(206, 122, 137);
fromMount1R = color(206, 122, 137);
toMount1R = color(97, 142, 153);
mount1RColor = lerpColor(fromMount1R, toMount1R, mouseX/width);
fill(mount1RColor);
triangle(111, 226, 57, 416, 189, 416);
//Mountain 2, pink
fill(232, 151, 168);
triangle(41, 254, -13, 444, -73, 444);
fill(206, 122, 137);
triangle(41, 254, -13, 444, 119, 444);
//Mountain 3, pink
fill(232, 151, 168);
triangle(510, 366, 456, 556, 396, 556);
fill(206, 122, 137);
triangle(510, 366, 456, 556, 588, 556);
//Mountain 4, largest, transitions from yellow to navy
fill(229, 225, 163);
fromMount4L = color(229, 225, 163);
toMount4L = color(31, 60, 78);
mount4LColor = lerpColor(fromMount4L, toMount4L, mouseX/width);
fill(mount4LColor);
triangle(248, 158, 154, 480, -111, 480);
fill(214, 192, 123);
fromMount4R = color(214, 192, 123);
toMount4R = color(10, 30, 49);
mount4RColor = lerpColor(fromMount4R, toMount4R, mouseX/width);
fill(mount4RColor);
triangle(248, 158, 154, 480, 591, 480);
//Stray cloud with transparency
fill(256, 125);
quad(210.5, 272, 250.5, 290, 210.5, 308, 170.5, 290);
//Mountain 5, transitions from pink to dark green
fill(232, 151, 168);
fromMount5L = color(232, 151, 168);
toMount5L = color(51, 93, 97);
mount5LColor = lerpColor(fromMount5L, toMount5L, mouseX/width);
fill(mount5LColor);
triangle(193, 290, 139, 480, 79, 480);
fill(206, 122, 137);
fromMount5R = color(206, 122, 137);
toMount5R = color(37, 77, 68);
mount5RColor = lerpColor(fromMount5R, toMount5R, mouseX/width);
fill(mount5RColor);
triangle(193, 290, 139, 480, 271, 480);
//Mountain 6, transitions from blue to pale green
fill(175, 232, 229);
fromMount6L = color(175, 232, 229);
toMount6L = color(170, 191, 156);
mount6LColor = lerpColor(fromMount6L, toMount6L, mouseX/width);
fill(mount6LColor);
triangle(108, 334, 93, 480, -30, 480);
fill(127, 201, 201);
fromMount6R = color(127, 201, 201);
toMount6R = color(130, 171, 142);
mount6RColor = lerpColor(fromMount6R, toMount6R, mouseX/width);
fill(mount6RColor);
triangle(108, 334, 93, 480, 253, 480);
//Mountain 7, transitions from green to light blue
fill(160, 232, 160);
fromMount7L = color(160, 232, 160);
toMount7L = color(105, 175, 173);
mount7LColor = lerpColor(fromMount7L, toMount7L, mouseX/width);
fill(mount7LColor);
triangle(295, 323, 229, 480, 153, 480);
fill(117, 175, 117);
fromMount7R = color(117, 175, 117);
toMount7R = color(97, 142, 153);
mount7RColor = lerpColor(fromMount7R, toMount7R, mouseX/width);
fill(mount7RColor);
triangle(295, 323, 229, 480, 399, 480);
//Clouds with transparency
fill(256, 125);
quad(332.5, 231, 372.5, 249, 332.5, 267, 292.5, 249);
quad(393.5, 217, 433.5, 235, 393.5, 253, 353.5, 235);
quad(298.5, 240, 338.5, 258, 298.5, 276, 258.5, 258);
quad(275.5, 235, 315.5, 253, 275.5, 271, 235.5, 253);
quad(258.5, 214, 298.5, 232, 258.5, 250, 218.5, 232);
quad(313.5, 265, 353.5, 283, 313.5, 301, 273.5, 283);
quad(339.5, 369, 379.5, 387, 339.5, 405, 299.5, 387);
quad(403.5, 258, 443.5, 276, 403.5, 294, 363.5, 276);
quad(353.5, 265, 393.5, 283, 353.5, 301, 313.5, 283);
}
Admittedly, it took me a while to come to this final idea, as a lot of my ideas required code that was out of my current technical abilities, although I do hope to act on them sometime in the future. After mocking up a design in Illustrator, I wanted to have a drawing that transitioned between states rather than an open-ended series of relations. I had some difficulties figuring out exactly how to execute all of the color transitions, but lerpColor(); wound up being the perfect solution, where the color in between the two I specified was determined by the mouse’s X position relative to the width of the canvas, creating the required interval number between 0-1. After finally figuring that out, the rest was a lot of trial and error, playing around with the code in order to see what kind of movement could be created while still making sense composition-wise.
mmiller5_Project-03
var bg = 0;
var angle = 30;
function setup() {
createCanvas(640, 480);
}
function draw() {
//constrain mouse values to be within canvas limits
var mX = constrain(mouseX, 0, width);
var mY = constrain(mouseY, 0, height);
//positional variables for circles and squares
var h1 = height/6;
var h2 = height/2;
var h3 = 5 * height/6;
var w1 = width/8;
var w2 = 3 * width/8;
var w3 = 5 * width/8;
var w4 = 7 * width/8;
var maxD = 3 * width/8;
var minD = width/8;
var diag = dist(w1, h1, w2, h2);
//background gets brighter as mouse moves right
bg = 255 * (mX/width);
background(bg);
fill(255 - bg); //opposite color of background
//enlarge circles as mouse moves near them //row 1
var d1 = constrain(maxD - dist(mX, mY, w1, h1), minD, maxD);
var d2 = constrain(maxD - dist(mX, mY, w2, h1), minD, maxD);
var d3 = constrain(maxD - dist(mX, mY, w3, h1), minD, maxD);
var d4 = constrain(maxD - dist(mX, mY, w4, h1), minD, maxD);
//row 2
var d5 = constrain(maxD - dist(mX, mY, w1, h2), minD, maxD);
var d6 = constrain(maxD - dist(mX, mY, w2, h2), minD, maxD);
var d7 = constrain(maxD - dist(mX, mY, w3, h2), minD, maxD);
var d8 = constrain(maxD - dist(mX, mY, w4, h2), minD, maxD);
//row 3
var d9 = constrain(maxD - dist(mX, mY, w1, h3), minD, maxD);
var d10 = constrain(maxD - dist(mX, mY, w2, h3), minD, maxD);
var d11 = constrain(maxD - dist(mX, mY, w3, h3), minD, maxD);
var d12 = constrain(maxD - dist(mX, mY, w4, h3), minD, maxD);
//circles //row 1
ellipse(w1, h1, d1, d1);
ellipse(w2, h1, d2, d2);
ellipse(w3, h1, d3, d3);
ellipse(w4, h1, d4, d4);
//row 2
ellipse(w1, h2, d5, d5);
ellipse(w2, h2, d6, d6);
ellipse(w3, h2, d7, d7);
ellipse(w4, h2, d8, d8);
//row 3
ellipse(w1, h3, d9, d9);
ellipse(w2, h3, d10, d10);
ellipse(w3, h3, d11, d11);
ellipse(w4, h3, d12, d12);
//square setup
noFill();
strokeWeight(3);
stroke(255 - bg);
rectMode(CENTER);
//squares rotate when mouse moves up and down
angle = (mY / height) * 360;
//left outside column (of squares)
push();
translate(-w1, -h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(-w1, h2);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(-w1, height + h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//first column
push();
translate(w1, h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w1, h3);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//second column
push();
translate(w2, -h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w2, h2);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w2, height + h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//third column
push();
translate(w3, h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w3, h3);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//fourth column
push();
translate(w4, -h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w4, h2);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(w4, height + h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//right outside column
push();
translate(width + w1, h1);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
push();
translate(width + w1, h3);
rotate(radians(angle));
rect(0, 0, diag, diag);
pop();
//center ellipse setup
fill(bg);
noStroke();
var dInner = 30;
//positional variables, use map to restrict center ellipse to inside circle //row 1
var x1 = map(mX, 0, width, w1 - d1/2 + dInner, w1 + d1/2 - dInner);
var y1 = map(mY, 0, height, h1 - d1/2 + dInner, h1 + d1/2 - dInner);
var x2 = map(mX, 0, width, w2 - d2/2 + dInner, w2 + d2/2 - dInner);
var y2 = map(mY, 0, height, h1 - d2/2 + dInner, h1 + d2/2 - dInner);
var x3 = map(mX, 0, width, w3 - d3/2 + dInner, w3 + d3/2 - dInner);
var y3 = map(mY, 0, height, h1 - d3/2 + dInner, h1 + d3/2 - dInner);
var x4 = map(mX, 0, width, w4 - d4/2 + dInner, w4 + d4/2 - dInner);
var y4 = map(mY, 0, height, h1 - d4/2 + dInner, h1 + d4/2 - dInner);
//row 2
var x5 = map(mX, 0, width, w1 - d5/2 + dInner, w1 + d5/2 - dInner);
var y5 = map(mY, 0, height, h2 - d5/2 + dInner, h2 + d5/2 - dInner);
var x6 = map(mX, 0, width, w2 - d6/2 + dInner, w2 + d6/2 - dInner);
var y6 = map(mY, 0, height, h2 - d6/2 + dInner, h2 + d6/2 - dInner);
var x7 = map(mX, 0, width, w3 - d7/2 + dInner, w3 + d7/2 - dInner);
var y7 = map(mY, 0, height, h2 - d7/2 + dInner, h2 + d7/2 - dInner);
var x8 = map(mX, 0, width, w4 - d8/2 + dInner, w4 + d8/2 - dInner);
var y8 = map(mY, 0, height, h2 - d8/2 + dInner, h2 + d8/2 - dInner);
//row 3
var x9 = map(mX, 0, width, w1 - d9/2 + dInner, w1 + d9/2 - dInner);
var y9 = map(mY, 0, height, h3 - d9/2 + dInner, h3 + d9/2 - dInner);
var x10 = map(mX, 0, width, w2 - d10/2 + dInner, w2 + d10/2 - dInner);
var y10 = map(mY, 0, height, h3 - d10/2 + dInner, h3 + d10/2 - dInner);
var x11 = map(mX, 0, width, w3 - d11/2 + dInner, w3 + d11/2 - dInner);
var y11 = map(mY, 0, height, h3 - d11/2 + dInner, h3 + d11/2 - dInner);
var x12 = map(mX, 0, width, w4 - d12/2 + dInner, w4 + d12/2 - dInner);
var y12 = map(mY, 0, height, h3 - d12/2 + dInner, h3 + d12/2 - dInner);
//center ellipses move corresponding to where mouse is //row 1
ellipse(x1, y1, dInner, dInner);
ellipse(x2, y2, dInner, dInner);
ellipse(x3, y3, dInner, dInner);
ellipse(x4, y4, dInner, dInner);
//row 2
ellipse(x5, y5, dInner, dInner);
ellipse(x6, y6, dInner, dInner);
ellipse(x7, y7, dInner, dInner);
ellipse(x8, y8, dInner, dInner);
//row 3
ellipse(x9, y9, dInner, dInner);
ellipse(x10, y10, dInner, dInner);
ellipse(x11, y11, dInner, dInner);
ellipse(x12, y12, dInner, dInner);
}
Oh boy, this took some time, mainly because I was trying to get something to work which never did. I wanted to focus on basic geometric changes, and I’m excited about how it turned out.
rsp1-Section-B-Project-03-Dynamic-Drawing
//Rachel Park
//Section B @ 10:30 AM
//rsp1@andrew.cmu.edu
//Project-03-Dynamic-Drawing
var c = 70;
var triX1 = 307;
var triX2 = 320;
var triX3 = 333;
var triX4 = 305;
var triX5 = 290;
var triX6 = 335;
var triX7 = 350;
var triX8 = 313;
var triX9 = 327;
var triY1 = 230;
var triY2 = 210;
var triY3 = 253;
var triY4 = 235;
var triY5 = 260;
var triY6 = 280;
var triY7 = 268;
function setup() {
createCanvas(640, 480);
}
function draw() {
background(c,60);
//modifying the background fill color
if (mouseX < 640) {
c = mouseX * 0.5;
}
if (mouseX < 0) {
c = mouseX;
}
var m = max(min(mouseX,400),0);
var size = m * (7/8);
var starsize = c;
var n = max(min(400,mouseY),0);
var moonlocation = n * (7/8);
//making the moon
push();
noStroke();
translate(width/4,height/4);
fill(254,255,182);
ellipse(0,moonlocation,50,50);
fill(c);
ellipse(10,moonlocation,45,45);
pop();
//constructing the rocketship + fire blast
push();
noStroke();
fill(255,4,4);
triangle(309 + m * (1/8),triY1-5, 320 + m * (1/8),triY2,
331 + m * (1/8),triY1-5);
fill(215);
triangle(305 + m * (1/8),triY3,305 + m * (1/8),triY4,292 + m * (1/8),triY5);
triangle(335 + m * (1/8),triY3,335 + m * (1/8),triY4,350 + m * (1/8),triY5);
fill(255,80,16);
ellipse(width/2 + m * (1/8),265,15,15);
triangle(320 + m * (1/8),triY6,313 + m * (1/8),triY7,327 + m * (1/8),triY7);
scale(0.5);
fill(255,248,16);
translate(width/2 + m * (1/8),260);
ellipse(width/2 + m * (1/8),265,15,15);
triangle(320 + m * (1/8),triY6,313 + m * (1/8),triY7,327 + m * (1/8),triY7);
pop();
noStroke();
fill(188);
translate(width/2,height/2);
scale(0.25);
rectMode(CENTER);
rect(m * (1/2),0,100,100,10);
// rect(m * (1/2),0,100,100,10);
push();
//rotation of the stars
rotate(frameCount / 90.0);
//small star
push();
noStroke();
fill(mouseX/4, mouseY,mouseX/2);
/*changing the color of the stars
using the mouse movement*/
translate(width-100,height/2);
rotate(frameCount / 100.0);
scale(0.5);
star(0,0,30,50,5);
pop();
//construction of mid-size star
push();
noStroke();
fill(mouseX/8, mouseY,mouseX/2);
translate(width-400, height/2);
rotate(frameCount / -120.0);
star(0,0,30,50,5);
pop();
}
//definition and utilization of variable for the stars
function star(x, y, radius1, radius2, npoints) {
var angle = TWO_PI / npoints;
var halfAngle = angle/2.0;
beginShape();
for (var a = 0; a < TWO_PI; a += angle) {
var sx = x + cos(a) * radius2;
var sy = y + sin(a) * radius2;
vertex(sx, sy);
sx = x + cos(a+halfAngle) * radius1;
sy = y + sin(a+halfAngle) * radius1;
vertex(sx, sy);
}
endShape(CLOSE);
}
This project was actually more difficult than the other projects that we have done this far, at least for me. I started with a simple idea of wanting to create a drawing of a rocket because it allowed for so many different options for using variables that move with the mouse. For this project, I decided to manipulate the movement of the rocket itself, the background color, the location of the moon, and the colors for the stars that are continually rotating around the rocket itself.
In the image below, I show some of the design ideas for the rocket itself, and which directions I initially wanted it to move.
Move the mouse up and down to move the moon, and move the mouse side to side to move the rocket and change the star colors.
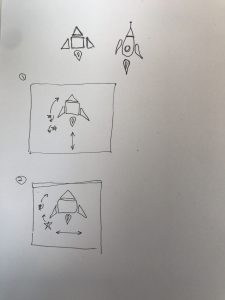
ablackbu-Project-03-Dynamic-Drawing
var flagX = 570;
var color1 = 255;
var color2 = 150;
function setup() {
createCanvas(640, 480);
}
function draw() {
//grass
background(57,181,74);
//sky
fill(102,193,242);
ellipse(320,100,1300,400);
//cloud will move and change color relative to mouseX movement
push();
translate((width / 2) - mouseX, 0);
fill(mouseX - color2);
ellipse(370,100,80,80);
ellipse(450,60,100,100);
ellipse(400,60,60,60);
ellipse(520,100,90,90);
ellipse(450,100,100,100);
ellipse(565,120,50,50);
pop();
//text
push();
textSize(20);
fill(255,mouseX / 2,40);
text("IT'S IN THE HOLE!",mouseX-60,100);
pop() ;
//green
fill(141,198,63);
ellipse(450,400,400,90);
//hole
fill(0);
ellipse(520,400,25,15);
//flag pole
fill(225);
rect(518,200,4, 200);
//flag
fill(200,0,0);
triangle(522,200,522, 250, flagX,225) ;
//ball
fill(color1);
noStroke();
ellipse(mouseX,mouseY,(600 - mouseX) / 7,(600 - mouseX) / 7);
//flag will move when mouse gets close
if(mouseX > 0 & mouseX < 220) {
flagX = 474
}else(flagX = 570);
//ball disappears when it is on top of the hole
if(mouseX > 515 & mouseY < 525 &&
mouseY > 398 && mouseY < 402){
color1 = 0
}else(color1 = 255);
}
First let me explain the drawing I created. The whole golf theme came to me a bit randomly. I was very interested in the pong game we made, so for the dynamic drawing I wanted to make another game. It is supposed to simulate a golfball getting closer to the hole. Once the ball is over the hole it disappears.
The part I found hardest about this project was coming up with an idea. I feel pretty confident with the concepts but just couldn’t get myself to come up with an idea. Once I expanded my thought from just moving patterns, this is what I came up with.
svitoora – 03 Dynamic Gravity Drawings
//
// Supawat Vitoorapakorn
// Svitoora@andrew.cmu.edu
// Section E
//
// Gravity is a point mass dynamic drawing inspired
// from physics, nature, and falling off a skateboard.
// f = G (m1*m2)/d^2
// f = G sum(m)/(d from avg)^2
// World Model Setup /////////////////////////////////////
var w = 480;
var h = 640;
var t = 1; // time variable
var g = .0025; // gravity
var count = 0; // global count of objects
var ball;
var balls = []; // Array of objects
var ball_size = w * .010 // Default object size
var max_count = 30;
var auto_every = 35;
var max_line = 1000; // Prevents crash
// Control ///////////////////////////////////////////////
// Creates new object in system given (x,y)
function ball_create(x, y) {
this.x = x;
this.y = y;
this.vx = 0;
this.vy = 0;
this.r = ball_size;
this.m = 1;
this.alpha = 255
}
// Add new object and delete oldest object
function mouseDragged() {
if (t % 10) {
balls.push(new ball_create(mouseX, mouseY));
}
if (balls.length >= max_count) {
balls.splice(0, 1)
}
}
// Add new object and delete oldest object
function mousePressed() {
balls.push(new ball_create(mouseX, mouseY));
if (balls.length >= max_count) {
balls.splice(0, 1)
}
}
// World /////////////////////////////////////////////////
// Maintain global count of balls
function count_balls(balls) {
count = balls.length;
}
// Maintain global mass of system
function sum_mass(balls) {
sum_m = 0;
for (i in balls) {
sum_m += balls[i].m;
}
return sum_m;
}
// Determines the system's average position X
function average_posX(balls) {
if (balls.length == 0) {
return w / 2;
};
var sum_x = 0;
for (i in balls) {
sum_x += balls[i].x;
}
avg = sum_x / balls.length
return avg;
}
// Determines the system's average position Y
function average_posY(balls) {
if (balls.length == 0) {
return h / 2;
};
var sum_y = 0;
for (i in balls) {
sum_y += balls[i].y;
}
avg = sum_y / balls.length;
return avg
}
// Apply gravity for all objects in the system
function gravity(balls) {
var avg_x = average_posX(balls);
var avg_y = average_posY(balls);
var speed = .1 //0-1 Multuplier for controlling velocity of attratction
for (i in balls) {
d = dist(balls[i].x, balls[i].y, avg_x, avg_y);
ds = map(d,0,w/2,1,0); // used to simulate d^2
// Gravity X
if (balls[i].x > avg_x) {
balls[i].x *= 1 - (g * (count * speed));
} else { balls[i].x *= 1 + (g * (count * speed+ds));}
// Gravity Y
if (balls[i].y > avg_y) {
balls[i].y *= 1 - (g * (count * speed))
} else { balls[i].y *= 1 + (g * (count * speed+ds));}
}
}
// Add object to system in the middle; // Used at setup()
function add_ball(balls) {
balls.push(new ball_create(w / 2, h / 2));
}
// Prevents software from crashing
// from too many objects beyond max_count
function death(balls, t) {
if (balls.length >= max_count) {
balls.splice(0, 1);
for (i in balls) {
balls[i].r *= .99;
}
}
}
// Connects all the object in the system via a line
function draw_line(balls) {
lines = 0
alpha = 255 * .2
if (lines < max_line) {
for (i in balls) {
var x_1 = balls[i].x;
var y_1 = balls[i].y;
for (i in balls) {
var x_2 = balls[i].x;
var y_2 = balls[i].y;
stroke(255, 255, 255, alpha);
line(x_1, y_1, x_2, y_2);
lines += 1;
}
}
}
}
// SETUP
function setup() {
createCanvas(w, h);
g = .0025;
background(50);
balls.length = 0; // Kill all objects in system
add_ball(balls);
}
// Refreshes the systems with new objects
// Removes old objects and add new objects
function auto_refresh(balls, t) {
// Starts refreshing system at 5 objects
// every auto_every interval.
if (t % auto_every == 0 & balls.length > 5) {
balls.splice(0, 1);
}
X = constrain(mouseX, 1, w);
Y = constrain(mouseY, 1, h)
if (t % auto_every == 0) {
balls.push(new ball_create(X, Y));
}
// Resets the system to 8 objects once every 500 ms
// This prevents overload; Array starts at [0]
if (t % 500 == 0 & balls.length > 8) {
balls.length = 7;
}
}
// Draw ////////////////////////////////////////////////////
// Draw all objects in systems mapped by distance from avg
function draw_balls(balls) {
for (ball in balls) {
noStroke();
var avg_x = average_posX(balls);
var avg_y = average_posY(balls);
var d = dist(balls[ball].x, balls[ball].y, avg_x, avg_y);
// Size and Alpha of Ball is a function of distance
var alpha = map(d, balls[ball].r, w / 2, 255, 0);
var size = map(d, 0, w / 2, .5, -1.5) //max to min
fill(255, 255, 255, alpha);
ellipse(balls[ball].x, balls[ball].y,
balls[ball].r * (2 + size),
balls[ball].r * (2 + size));
}
}
// Execute /////////////////////////////////////////////////
function draw() {
background(50);
noStroke();
// Update World
t = t + 1;
count_balls(balls);
auto_refresh(balls, t);
if (balls.length > 1) {
gravity(balls);
}
death(balls, t);
// Draw World
draw_line(balls);
draw_balls(balls);
}
Physics
Gravity is a point mass dynamic drawing inspired from physics, nature, and falling off a skateboard. I wanted to create a systematic representation of mass attracts mass. To do this I was inspired by the physics formula:
The problem with this forumla is that it is meant for physicist dealing with a two body system. In my case, I wanted a forumla that worked with multiple bodies in a system algorithmically. After sketching for a while with equations on the white board, I realized that that the problem I was trying to solve was much simplier than I expected.
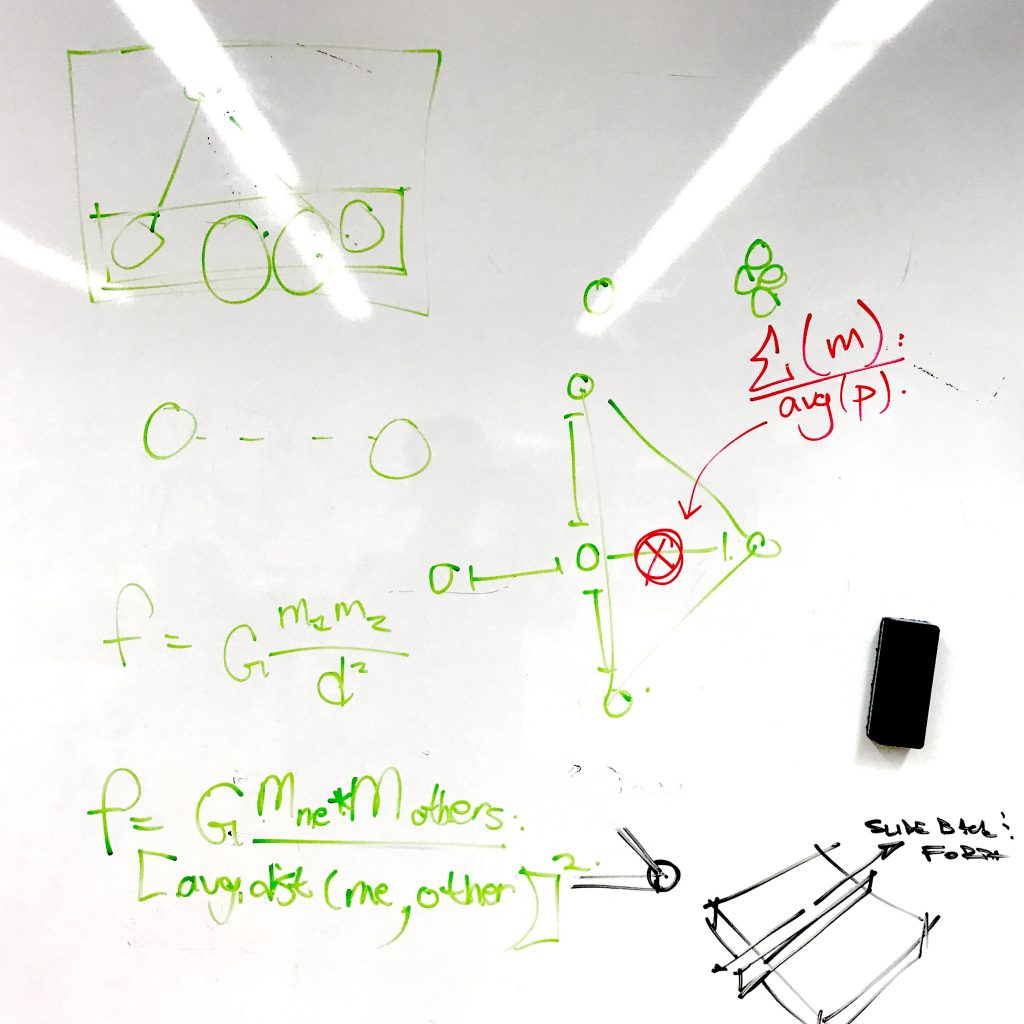
Essentially the problem boils down to averages. If all the object in the system had a mass of 1, then the center of mass of the system would be at the average coordinate position of every point mass. By definition this is the centroid of the system.

After defininig the centroid, I simply make every object in the system moves towards it. To replicate d^2’s effect on speed, I use a position *= and /= function, in addition to a mapping function of speed based off distance away from the centroid.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Apply gravity for all objects in the system function gravity(balls) { var avg_x = average_posX(balls); var avg_y = average_posY(balls); var speed = .1 //0-1 Multuplier for controlling velocity of attratction for (i in balls) { d = dist(balls[i].x, balls[i].y, avg_x, avg_y); ds = map(d,0,w/2,1,0); // used to simulate d^2 // Gravity X if (balls[i].x > avg_x) { balls[i].x *= 1 - (g * (count * speed)); } else { balls[i].x *= 1 + (g * (count * speed+ds));} // Gravity Y if (balls[i].y > avg_y) { balls[i].y *= 1 - (g * (count * speed)) } else { balls[i].y *= 1 + (g * (count * speed+ds));} } } |
Model-View-Controller (MVC)
This programs uses the MVC framework taught in 15-112. Objects are models are added to an array, modfified by the controller, and then representated through view functions. Because of the complexity of this code, I used to object-oriented programming to organize my variables. I didn’t quite understand object-based methods in javascript yet, so I worked around them.
Demo and Project Requirments:
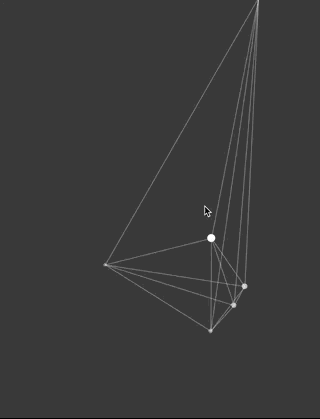
To sastify the project requirement, there are no random or non-deterministic element. Every function is deterministic, therefore given the exact same mouse input the Gravity will create the exact same drawing. The variables that are being altered in how the image is being created are: size, position, opacity, angle, etc.
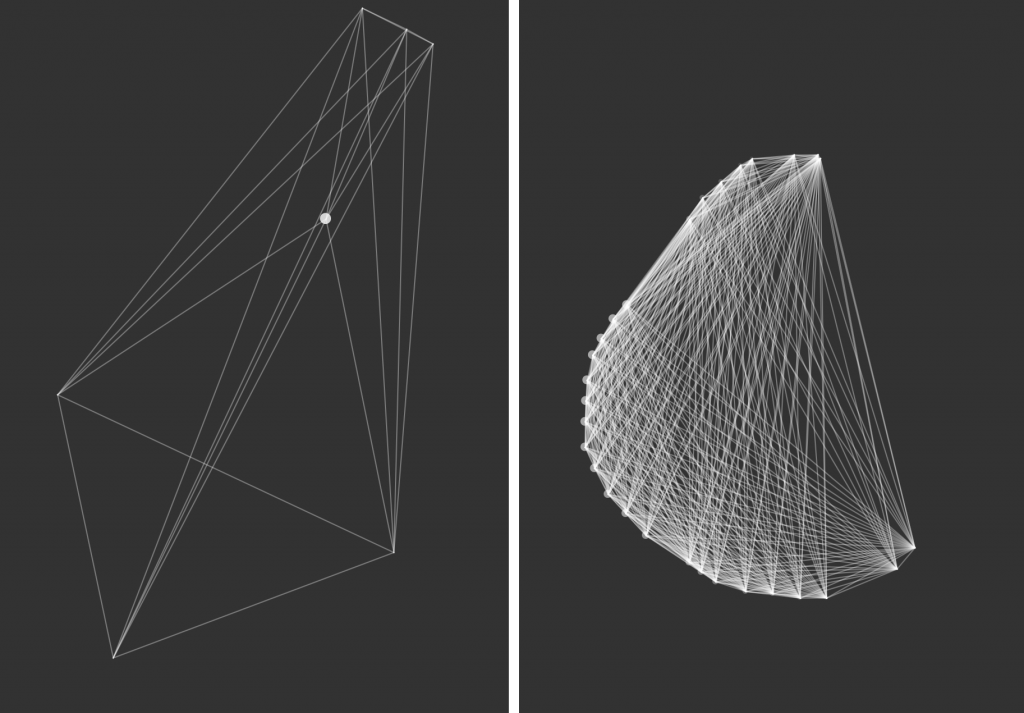
amui1-Project-03-Dynamic-Drawing
Click to change time of day
//Allison Mui
//15-104 Section A
//amui1@andrew.cmu.edu
//Project-03
//variables for cloud
var cloud1x = 50;
var cloud1y = 100;
var cloud2x = 90;
var cloud2y = 90;
var cloud3x = 130;
var cloud3y = 100;
var speed = .30;
var dir = 1;
//variables for rain
var rainY = 110
var rainSpeed = 5;
//variables for bird
var birdHx = 300;
var birdHy = 250;
//variables for easing
var easing = .005;
//variables to switch b/w time of daytime
var day = 0;
function setup() {
createCanvas(640,480);
}
function draw() {
if (day == 0) {
background(151,214,216);
//sun
fill(255,255,0);
ellipse(600,0,225,225);
//flower
//flowerstem
stroke(0,100,0);
strokeWeight(8);
var stemY = constrain(mouseY,350,480);
line(100,stemY,100,480);
//flowerbody
stroke(0);
strokeWeight(0);
fill(0);
//restrict flower height
var flowerY = constrain(mouseY,350,480);
var flowerSize = flowerY*20/175;
fill(255,255,0);
arc(100,flowerY,flowerSize*2-25,flowerSize*2+5,PI,0);
arc(100,flowerY,flowerSize*2-25,flowerSize*2+5,0,PI);
arc(100,flowerY,flowerSize*2+10,flowerSize*2-30,HALF_PI,3*HALF_PI);
arc(100,flowerY,flowerSize*2+10,flowerSize*2-30,3*HALF_PI,HALF_PI);
fill(0);
ellipse(100,flowerY,flowerSize,flowerSize);
//clouds
fill(255);
strokeWeight(0);
//firstcloud
ellipse(cloud1x,cloud1y,50,50);
ellipse(cloud2x,cloud2y,70,80);
ellipse(cloud3x,cloud3y,50,50);
//secondcloud
ellipse(cloud1x+200,cloud1y,50,50);
ellipse(cloud2x+200,cloud2y,70,80);
ellipse(cloud3x+200,cloud3y,50,50);
//thirdcloud
ellipse(cloud1x+400,cloud1y,50,50);
ellipse(cloud2x+400,cloud2y,70,80);
ellipse(cloud3x+400,cloud3y,50,50);
//movecloud
cloud1x += dir*speed;
cloud2x += dir*speed;
cloud3x += dir*speed;
//loopclouds
if (cloud3x > width-25 || cloud1x < 25){
dir = -dir;
}
if (cloud3x + 200 > width-25 || cloud1x+200 < 25){
dir = -dir;
}
if (cloud3x + 400 > width-25 || cloud1x+400 < 25){
dir = -dir;
}
} else{
background(25,25,112);
fill(255);
strokeWeight(0);
//firstcloud
ellipse(cloud1x,cloud1y,50,50);
ellipse(cloud2x,cloud2y,70,80);
ellipse(cloud3x,cloud3y,50,50);
//secondcloud
ellipse(cloud1x+200,cloud1y,50,50);
ellipse(cloud2x+200,cloud2y,70,80);
ellipse(cloud3x+200,cloud3y,50,50);
//thirdcloud
ellipse(cloud1x+400,cloud1y,50,50);
ellipse(cloud2x+400,cloud2y,70,80);
ellipse(cloud3x+400,cloud3y,50,50);
//movecloud
cloud1x += dir*speed;
cloud2x += dir*speed;
cloud3x += dir*speed;
//loopclouds
if (cloud3x > width-25 || cloud1x < 25){
dir = -dir;
}
if (cloud3x + 200 > width-25 || cloud1x+200 < 25){
dir = -dir;
}
if (cloud3x + 400 > width-25 || cloud1x+400 < 25){
dir = -dir;
}
//rain
fill(220,220,220);
ellipse(cloud1x,rainY+10,10,10);
ellipse(cloud2x,rainY+10,10,10);
ellipse(cloud3x,rainY+10,10,10);
ellipse(cloud1x+200,rainY+10,10,10);
ellipse(cloud2x+200,rainY+10,10,10);
ellipse(cloud3x+200,rainY+10,10,10);
ellipse(cloud1x+400,rainY+10,10,10);
ellipse(cloud2x+400,rainY+10,10,10);
ellipse(cloud3x+400,rainY+10,10,10);
rainY += rainSpeed;
if (rainY > height/2 - 40){
rainY = cloud1y + 10;
}
}
//drawbird
//bird flying right
if (mouseX > birdHx) {
var targetX = mouseX;
var distX = mouseX - birdHx;
birdHx += distX*easing;
//where bird wants to travel y
var targetY = mouseY;
var distY = mouseY - birdHy;
birdHy += distY*easing;
//birdhair
stroke(255,255,153);
strokeWeight(5);
line(birdHx-2,birdHy-25,birdHx+3,birdHy-10);
line(birdHx+6,birdHy-25,birdHx-2,birdHy-10);
//birdlegs
strokeWeight(0);
fill(255,140,0);
rect(birdHx-30,birdHy+50,3,10);
rect(birdHx-20,birdHy+50,3,10);
ellipse(birdHx-27,birdHy+60,5,5);
ellipse(birdHx-17,birdHy+60,5,5);
//birdbody
if (mouseY < 100){
fill(255,250,250);
strokeWeight(0);
ellipse(birdHx,birdHy,40,40);
ellipse(birdHx-20,birdHy+30,60,45);
ellipse(birdHx-45,birdHy+20,25,20);
//halo
noFill();
stroke(0,191,255);
strokeWeight(3);
ellipse(birdHx+3,birdHy-35,20,5);
} else {
fill(255,255,153);
strokeWeight(0);
ellipse(birdHx,birdHy,40,40);
ellipse(birdHx-20,birdHy+30,60,45);
ellipse(birdHx-45,birdHy+20,25,20);
}
//birdbeak
strokeWeight(0);
fill(255,140,0);
triangle(birdHx+20,birdHy-2,birdHx+30,birdHy+10,birdHx+16,birdHy+10);
//wing
arc(birdHx-20,birdHy+28, 13, 25, 0, PI+QUARTER_PI, CHORD);
//eye
fill(255);
ellipse(birdHx,birdHy,15,15);
fill(0);
ellipse(birdHx+3,birdHy,8,8);
}
if (mouseX < birdHx) {
//bird flying left
var targetX = mouseX;
var distX = mouseX - birdHx;
birdHx += distX*easing;
//where bird wants to travel y
var targetY = mouseY;
var distY = mouseY - birdHy;
birdHy += distY*easing;
//birdhair
stroke(255,255,153);
strokeWeight(5);
line(birdHx-7,birdHy-25,birdHx-2,birdHy-10);
line(birdHx+3,birdHy-25,birdHx-2,birdHy-10);
//birdlegs
strokeWeight(0);
fill(255,140,0);
rect(birdHx,birdHy+50,3,10);
rect(birdHx+10,birdHy+50,3,10);
ellipse(birdHx,birdHy+60,5,5);
ellipse(birdHx+10,birdHy+60,5,5);
if (mouseY < 100){
fill(255,250,250);
strokeWeight(0);
ellipse(birdHx,birdHy,40,40);
ellipse(birdHx+10,birdHy+30,60,45);
ellipse(birdHx+30,birdHy+20,25,20);
//halo
noFill();
stroke(0,191,255);
strokeWeight(3);
ellipse(birdHx+3,birdHy-35,20,5);
} else {
fill(255,255,153);
strokeWeight(0);
ellipse(birdHx,birdHy,40,40);
ellipse(birdHx+10,birdHy+30,60,45);
ellipse(birdHx+30,birdHy+20,25,20);
}
strokeWeight(0);
//birdbeak
fill(255,140,0);
triangle(birdHx-20,birdHy-2,birdHx-30,birdHy+10,birdHx-16,birdHy+10);
//wing
arc(birdHx+10,birdHy+28, 13, 25, 0, PI+QUARTER_PI, CHORD);
//eye
fill(255);
ellipse(birdHx,birdHy,15,15);
fill(0);
ellipse(birdHx-2,birdHy,8,8);
}
}
function mousePressed() {
if (day == 0) {
day = 1;
} else {
day = 0;
}
}
For this project, I made a little story about a bird/duck that can fly. I had a lot of fun with this project, trying out new ways to incorporate mouseX and mouseY. I first sketched out the background and then started to hardcode in p5js. After that, I changed my objects to be coordinated with mouseX and mouse Y. The most difficult part was coordinating the size of the flower with mouseY. The things I controlled with my mouse was: size of the flower, position of the flower, color of the duck, and direction of the duck.